Creating dashboards for visualizing SQL wisdom has been a comparable name for for a long time. By contrast, technologies for building web apps trade somewhat regularly, so developers meet this unchangeable name for differently annually.
In this article, I’ve decided to share my latest professional enjoy making a web app for visualizing wisdom from a database.
Choosing technologies
Probably the most prerequisites I had was once to use MySQL. Then again, I might simply freely choose the rest of my toolkit, at the side of a framework.
React has at all times been trendy among web developers for building rich individual interfaces. I’ve moreover had a super enjoy the usage of React in my previous duties and decided to refresh my skills with it.
On the other hand React isn’t in truth a framework anymore. Learning for the duration of the React documentation, I’ve came upon that any further, duties should be created the usage of one of the following React-based frameworks:
- Subsequent.js
- Remix
- Gatsby
- Expo (for React Local)
Consistent with the React group of workers, the usage of a framework from the start promises that the choices used by most web techniques are built-in, similar to routing, HTML generation, wisdom fetching, and so on.
In this manner, when your challenge reaches the aim when such choices are sought after, you do not need to seek for additional libraries and configure them for your challenge. As a substitute, all choices are already available for you, making building so much smoother. You’ll see further details on the React website online.
This left me with one question…
Which framework should I use?
As with the whole thing in this international, there’s no commonplace React framework that matches every need.
I’ve decided to prevent on Subsequent.js for my challenge, and right here’s why:
- Next.js is positioned as a default go-to React framework.
- The most recent Next.js 13 was once developed in close collaboration with the React group of workers, so the framework fulfills the staff’s imaginative and prescient of a super full-stack React framework.
- Next.js is a part of Jamstack building construction. Jamstack web websites are known for their SEO-friendliness, individual enjoy, pace, and serve as.
- Next.js is helping server-side rendering and static website online era, which is in a position to dramatically increase the serving pace of your web app. You’ll moreover mark explicit React parts to be rendered on the consumer side, making Next.js very flexible. Proper right here you’ll learn when to make use of server and shopper elements.
- Next.js 13 is now the usage of the fetch API, so there’s no want to at all times declare the getServersideProps and getStaticProps methods. Learn extra about knowledge fetching in Subsequent.js 13.
- Next.js 13 introduces numerous exciting new choices and number one improvements! Learn extra information about adjustments in Subsequent.js 13.
The framework is chosen, however it’s not enough. We moreover need apparatus for connecting to MySQL and visualizing its wisdom.
Choosing wisdom visualization apparatus
You should somewhat consider which third-party apparatus to use in your challenge since there are such a lot of alternatives and not every thought to be considered one of them would perhaps suit your needs. For me, the perfect imaginable alternatives had been Flexmonster and Recharts.
Both a type of apparatus are flexible, difficult, and easy to use.
- Flexmonster is basically a pivot table and we could in working with different datasets, similar to MySQL. It can also be integrated with slightly numerous frameworks, at the side of Next.js! Flexmonster’s documentation even provides a devoted educational for integrating this software with Subsequent.js. Flexmonster is a paid instrument, however it supplies a 30-day trial, which was once more than enough for me to get conversant in the product and come to a decision if it suits my use cases.
- Recharts is a charting library with a wide array of built-in charts and customization alternatives. The library pairs in particular well with Next.js techniques as it uses React’s component-based construction. Recharts is a free instrument introduced beneath the MIT license, which is price making an allowance for.
My deciding factor was once the simple setup of Flexmonster, Recharts, and their conversation. While Flexmonster connects to the MySQL dataset, processes its wisdom, and visualizes the guidelines inside the pivot table, Recharts can use the processed wisdom to visualize it in charts.
If you happen to’re wondering whether or not or now not the ones apparatus fit your challenge, check out the best possible equipment for knowledge visualization in React article. It covers in great part the opposite alternatives to be had in the marketplace, their professionals and cons, along with their use cases.
Now that the preparations are entire let’s create the actual app!
Create a Next.js challenge
First, a elementary Next.js app should be created with the following command:
.main { -next-app next-sql --ts --app && cd next-sql
Understand the --ts
and --app
arguments. They permit TypeScript and the new App Router feature, which this instructional assumes in more instructions.
You’ll also be given turns on to permit other choices, similar to ESLint or Tailwind CSS. For simplicity, solution No. However while you already know what you’re doing and the ones choices are very important for your challenge – feel free to permit them.
Moreover, it’s very best imaginable to remove unnecessary wisdom from the newly created challenge. For the wishes of this instructional, we won’t need the public/
folder so it’s imaginable you’ll delete it. While you think it’s imaginable you’ll need this folder for various reasons, feel free to leave it where it’s.
In addition to, trade the app/internet web page.tsx document, so it has the following contents:
"use consumer" import sorts from './internet web page.module.css' export default function Area() { return (); }
It’s important that the internet web page is marked as a Shopper Part on account of we can add choices that can be used best when the internet web page is rendered on the consumer side.
After all, the unnecessary sorts should be deleted from the app/internet web page.module.css document so it has the following content material subject material:
.main { display: flex; flex-direction: column; justify-content: space-between; align-items: center; padding: 6rem; min-height: 100vh; }
Embed wisdom visualization apparatus
Next, we want to add Flexmonster and Recharts to our newly created challenge. Let’s get began with Flexmonster.
Embedding Flexmonster
First, arrange the Flexmonster CLI:
npm arrange -g flexmonster-cli
Next, download the Flexmonster wrapper for React:
flexmonster add react-flexmonster
Now let’s add Flexmonster to our internet web page:
- Import Flexmonster sorts into the app/world.css document in your challenge:
@import "flexmonster/flexmonster.css";
- Create the PivotWrapper Shopper Part (e.g., app/PivotWrapper.tsx) that may wrap FlexmonsterReact.Pivot:
"use consumer" import * as React from "react"; import * as FlexmonsterReact from "react-flexmonster"; import Flexmonster from "flexmonster"; sort PivotProps = Flexmonster.Params & { pivotRef?: React.ForwardedRef; }; const PivotWrapper: React.FC = ({ pivotRef, ...params }) => { return ( ); }; export default PivotWrapper;
- Import the PivotWrapper into your Subsequent.js web page (e.g., app/internet web page.tsx) as a dynamic element with out SSR:
import dynamic from "next/dynamic"; const PivotWrapper = dynamic(() => import("@/app/PivotWrapper"), { ssr: false, loading: () => "Loading Flexmonster..." });
The SSR is disabled on account of Flexmonster uses the window object, so it may possibly’t be rendered on a server.
- Within the an identical internet web page document, create an additional part for ref forwarding (e.g.,
ForwardRefPivot
):import * as React from "react"; import { Pivot } from "react-flexmonster"; const ForwardRefPivot = React.forwardRef( (props, ref?: React.ForwardedRef) => );
- Inside the internet web page part (e.g., Area), create an empty ref object (e.g.,
pivotRef
):export default function Area() { const pivotRef: React.RefObject = React.useRef(null); }
- Then insert the
ForwardRefPivot
part from step 4 and move the ref object as its prop:export default function Area() { const pivotRef: React.RefObject = React.useRef(null); return (
Now Flexmonster is ready to be used. Let’s switch to Recharts.
Embedding Recharts
Get began by the use of putting in place Recharts with the following command:
npm arrange recharts
Then, import the LineChart part with its child parts and insert them after the pivot table:
import { LineChart, Line, CartesianGrid, YAxis, XAxis } from "recharts"; export default function Area() { const pivotRef: React.RefObject = React.useRef(null); return (); }
Technically, we’ve added Recharts to our internet web page, on the other hand we want to fill it with wisdom. Let’s first create interfaces that may describe wisdom for Recharts:
interface RechartsDataObject { [key: string]: any; } interface RechartsData { wisdom: RechartsDataObject[]; xName: string; lineName: string; }
Then, we want to create a variable that may take hold of the Recharts wisdom. On the other hand take note, our wisdom will come from Flexmonster, which is in a position to trade at runtime. So we’d like some way of tracking changes to this knowledge. That’s where React states come in handy. Add the following code to your internet web page part:
export default function Area() { // Flexmonster instance ref const pivotRef: React.RefObject = React.useRef(null); // Recharts wisdom const [chartsData, setChartsData] = React.useState({ wisdom: [], xName: "", lineName: "" }); // Subscribe on Recharts wisdom changes React.useEffect(() => { console.log("Charts wisdom changed!"); }, [chartsData]); // The rest of the code }
Now, the guidelines for Recharts will probably be stored inside the chartsData variable, and on account of the useState
and useEffects
React Hooks, we can know when it’s changed.
In spite of everything, we want to tell Recharts to use chartsData as its provide of information by the use of together with the following props:
Inside the next segment, let’s fill the chartsData with the guidelines from Flexmonster so our pivot table and charts are synced.
Connecting Flexmonster and Recharts
Inside the app/internet web page.tsx document, let’s create a function that transforms the guidelines from Flexmonster so it can be accepted by the use of Recharts:
import { GetDataValueObject } from "flexmonster"; function prepareDataFunction(rawData: Flexmonster.GetDataValueObject): RechartsData | null { // If there's no wisdom, return null if (!rawData.wisdom.period) return null; // Initialize chartsData object const chartsData: RechartsData = { wisdom: [], xName: rawData.meta["r0Name" as keyof typeof rawData.meta], lineName: rawData.meta["v0Name" as keyof typeof rawData.meta] }; // Become Flexmonster wisdom so it can be processed by the use of Recharts // The principle rawData ingredient is skipped because it incorporates a grand general value, not sought after for our charts for (let i = 1, dataObj, chartDataObj: RechartsDataObject; i < rawData.wisdom.period; i++) { dataObj = rawData.wisdom[i]; chartDataObj = {}; chartDataObj[chartsData.xName] = dataObj["r0" as keyof typeof dataObj]; chartDataObj[chartsData.lineName] = dataObj["v0" as keyof typeof dataObj]; chartsData.wisdom.push(chartDataObj); } return chartsData; }
To stick the educational simple, prepareFunction
returns best wisdom for the principle row and measure. If you want to display wisdom for another field or measure, switch this field or measure to the principle position the usage of the Box Record in Flexmonster.
Watch this video to see how it works:
Now, let’s add a function for chart drawing inside the internet web page part itself (e.g., Area). The function will identify the previously created prepareFunction and exchange the chartsData state to reason re-rendering:
export default function Area() { const pivotRef: React.RefObject = React.useRef(null); const [chartsData, setChartsData] = React.useState({ wisdom: [], xName: "", lineName: "" }); React.useEffect(() => { console.log("Charts wisdom changed!"); }, [chartsData]); const drawChart = (rawData: GetDataValueObject) => { const chartsData = prepareDataFunction(rawData); if (chartsData) { setChartsData(chartsData); } } }
All that’s left is to tell Flexmonster to use this function when its wisdom is changed. Add the following props to the ForwardRefPivot
part:
<ForwardRefPivot ref={pivotRef} toolbar={true} reportcomplete={() => { pivotRef.provide?.flexmonster.off("reportcomplete"); pivotRef.provide?.flexmonster.getData({}, drawChart, drawChart); }} licenseKey="XXXX-XXXX-XXXX-XXXX-XXXX" />
Proper right here, we’ve subscribed to the reportcomplete
match to take hold of when Flexmonster is in a position to provide wisdom. When the improvement is brought on, we immediately unsubscribe from it and use the getData
way to tell Flexmonster where to move the guidelines and what to do when it’s up-to-the-minute. In our case, we can use the drawChart
function for every purposes.
Moreover, understand the licenseKey
prop. It is going to need to contain a novel trial key that may allow us to connect to Recharts. You can get this sort of key by the use of contacting the Flexmonster staff. Upon getting the necessary factor, paste it somewhat than XXXX-XXXX-XXXX-XXXX-XXXX
.
Flexmonster and Recharts nowadays are attached, on the other hand they are nevertheless empty! Let’s restore it by the use of connecting to a database!
Connecting to MySQL
Since Recharts gets wisdom from Flexmonster, best the latter should be hooked as much as the database. Fortunately, Flexmonster provides a ready-to-use solution – Flexmonster Information Server. It is a server software, so all aggregations are performed on the server side, which saves the browser’s belongings and results in quicker wisdom visualization. This fits utterly into the Next.js philosophy of putting heavy artwork on the server (see the SSG and SSR choices).
We can arrange the Data Server the usage of the previously installed Flexmonster CLI:
flexmonster add fds -r
As quickly because the command is finished – Flexmonster Admin Panel will open. It is a instrument for configuring the Data Server and its connections, additionally known as indexes. Let’s configure one for our MySQL database.
- Open the Indexes tab and click on on Add New Index:
- Under the Establish and Kind fields, enter the connection’s identify (e.g., next-sql) and make a selection the Database sort:
- The Database sort should be set to MySQL by the use of default. Enter the Connection string and the Query for your database beneath the respective fields. For this instructional, I have hosted a development database on the freedb.tech provider. Be at liberty to use this connection string and query:
Connection string:
Server=sql.freedb.tech;Port=3306;Uid=freedb_dkflbvbh;pwd=NpkyU?jYv!2Zn&B;Database=freedb_next_fm
Query:
SELECT CONCAT(first_name,' ',last_name) AS full_name,salary,TIMESTAMPDIFF(YEAR, birthday, CURDATE()) AS age FROM consumers
The Refresh time indicates how perpetually the guidelines should be refreshed (0 manner the guidelines is not refreshed).
When all the configurations are set, hit Create to establish the connection to the database:
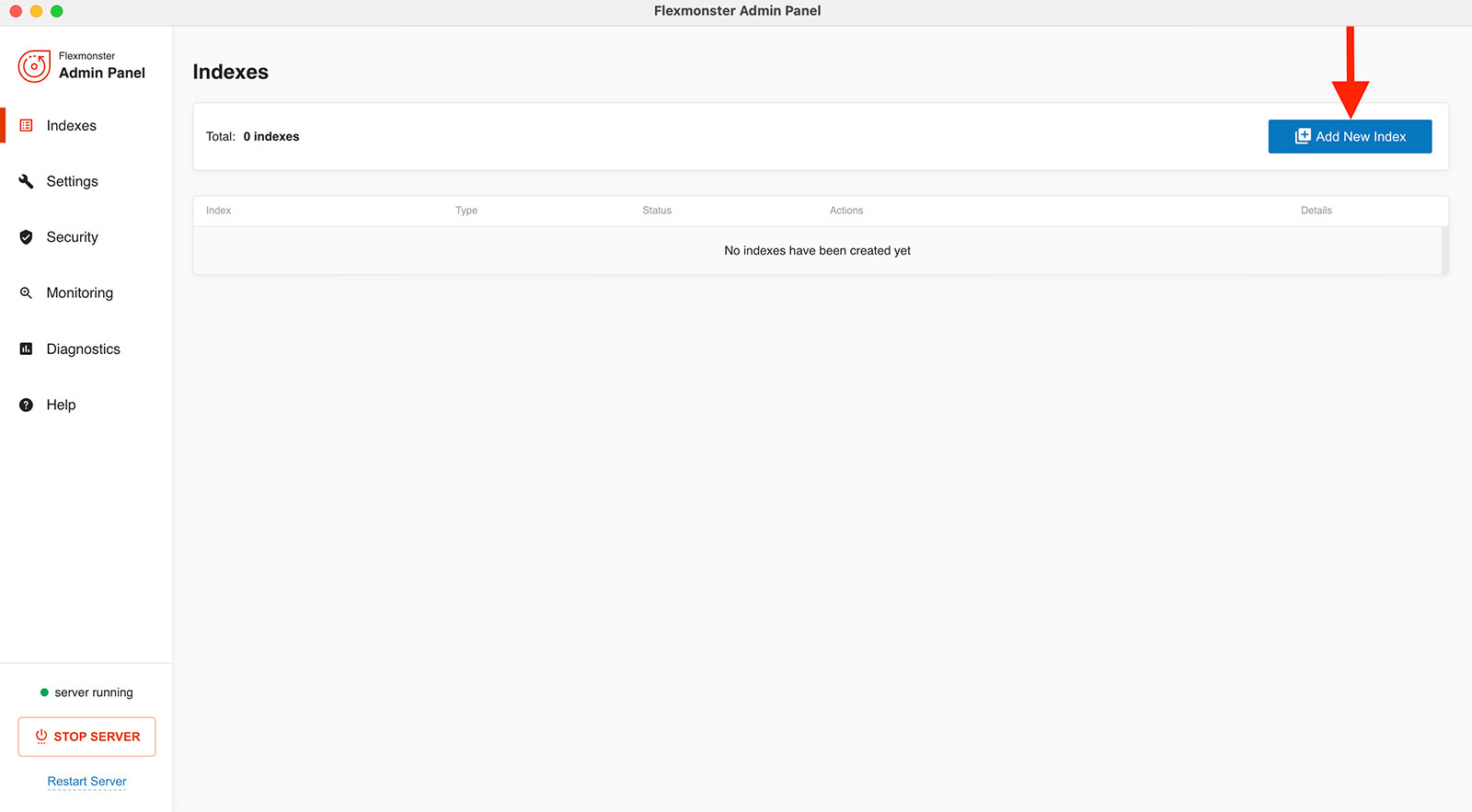
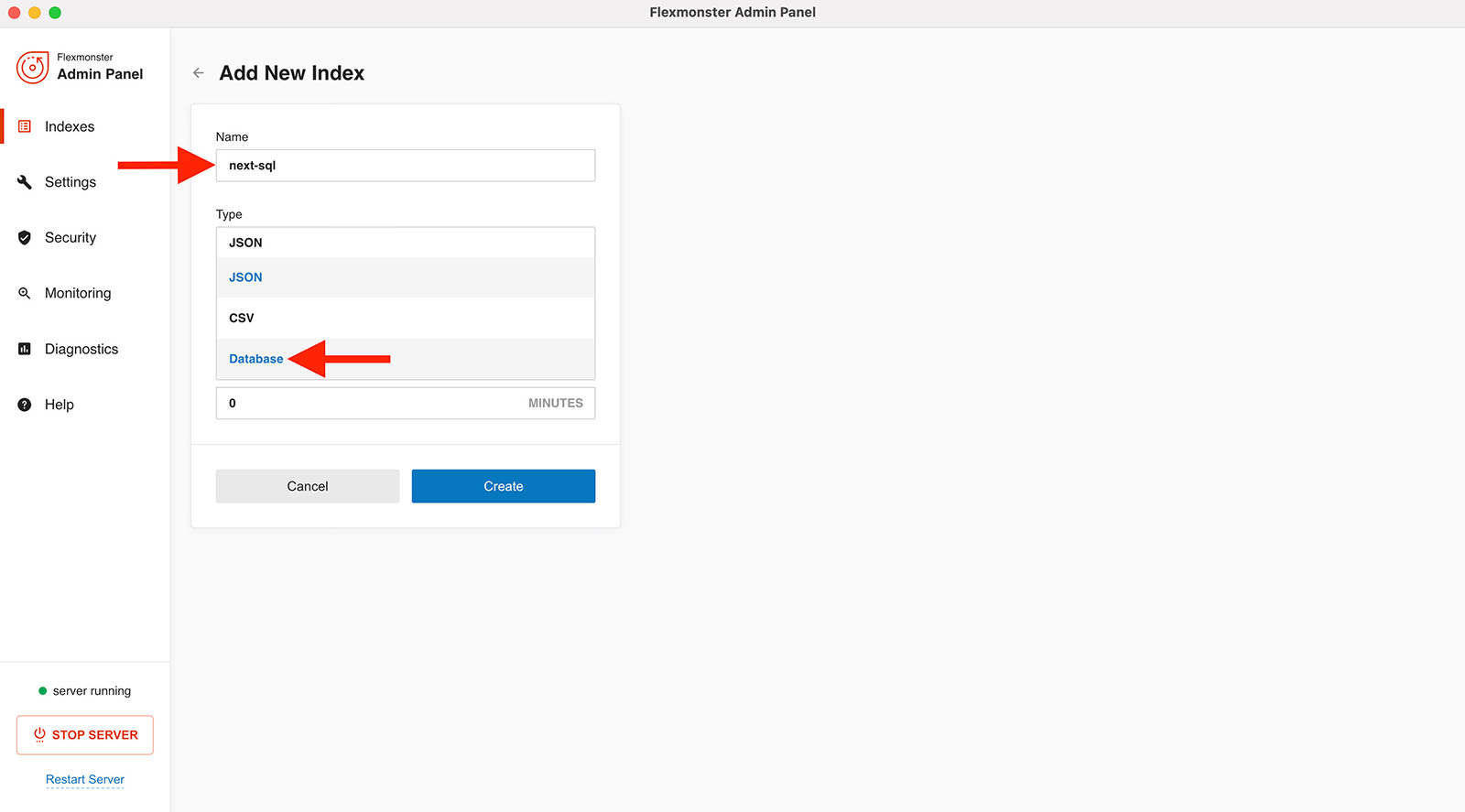
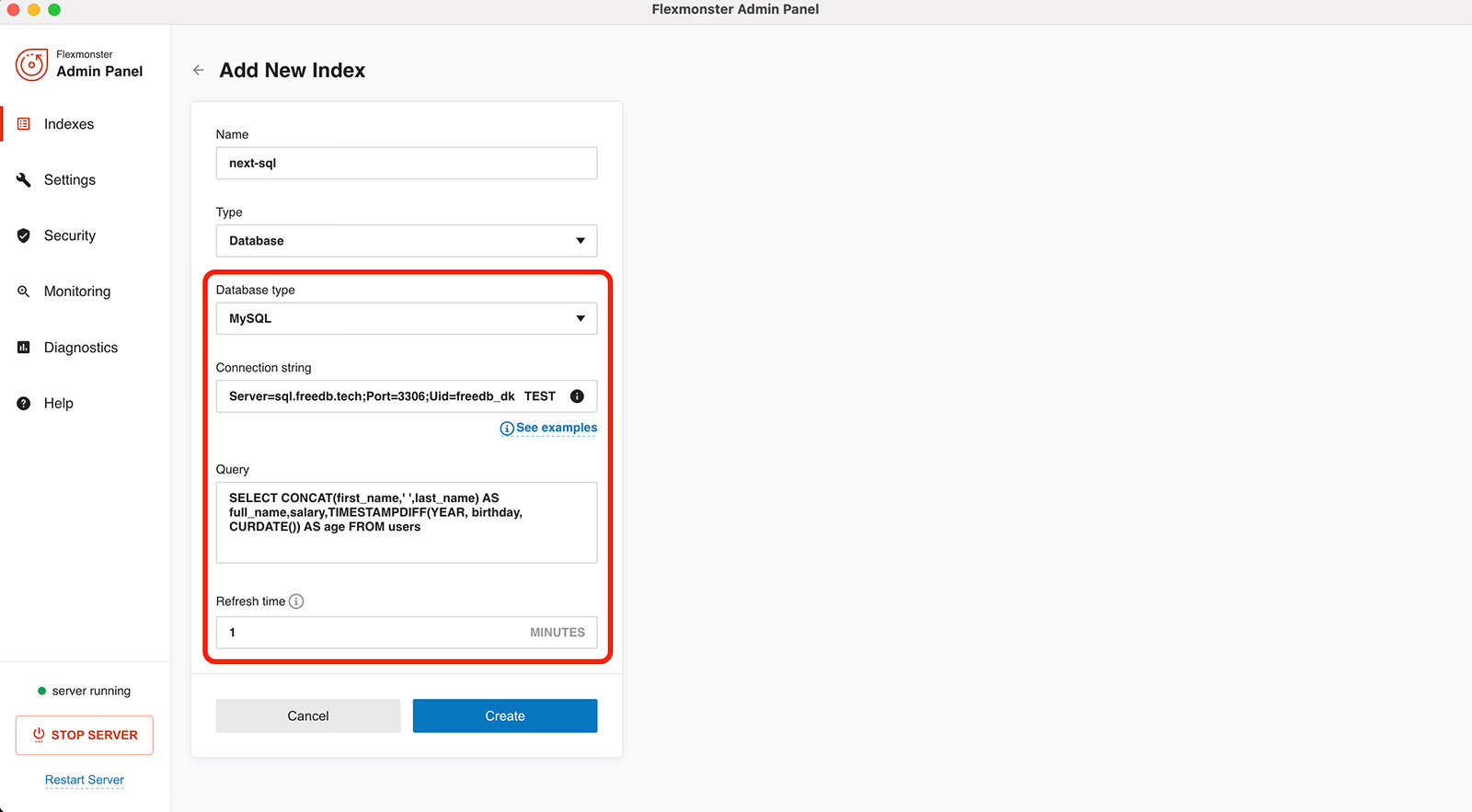
Now, all that’s left is to visualize the guidelines.
Visualize the guidelines inside the pivot table and charts
To visualize wisdom waiting by the use of the Data Server, we want to create a Flexmonster record.
A report is used to predefine configurations for Flexmonster Pivot. Our hobby lies inside the knowledge supply configuration for SQL databases.
We can represent our report as an object (e.g., report) that incorporates the following wisdom:
const report = { dataSource: { sort: "api", url: "http://localhost:9500", index: "next-sql" } };
Let’s duvet what every belongings stands for:
-
dataSource
incorporates wisdom provide configurations for the pivot table part.For simplicity, I’ve specified best the desired properties to visualize our wisdom. There are many further alternatives described in Flexmonster medical doctors.
-
dataSource.sort
specifies the type of the guidelines provide.In our case, it’s going to need to be set to “api“.
-
dataSource.url
incorporates the Data Server’s URL where the client will send requests for wisdom.Given that consumer and the server run on the an identical gadget, we can use the localhost care for. The port is ready to
9500
for the reason that Data Server uses it by the use of default. You can run the Information Server on some other port. -
dataSource.index
identifies the dataset created inside the Data Server.We’ve named it “next-sql” partially 3 of this instructional, so let’s specify it proper right here.
Now, let’s move the previously created report as a ForwardRefPivot
prop:
<ForwardRefPivot ref={pivotRef} toolbar={true} report={report} reportcomplete={() => { pivotRef.provide?.flexmonster.off("reportcomplete"); pivotRef.provide?.flexmonster.getData({}, drawChart, drawChart); }} licenseKey="XXXX-XXXX-XXXX-XXXX-XXXX" />
Conclusion
Hooray, our Next.js wisdom visualization app is ready and can also be started with this command:
npm run assemble && npm get began
You can moreover get this educational mission on GitHub. Be at liberty to use it as a starting point when developing your NEXT app (pun meant)!
The submit Making a Subsequent.js Dashboard for SQL Information Visualization seemed first on Hongkiat.
Supply: https://www.hongkiat.com/blog/nextjs-dashboard-sql-visualization/
Contents
- 0.1 Related posts:
- 1 Using Clamp() In Divi 5 To Create Fluid Responsive Typography
- 2 How To Upload CAPTCHA Coverage to WordPress
- 3 Zapier’s Head of Paid Advertisements on Storytelling, AI-Centered Advertisements, and Why He is All-...
0 Comments