This present day, React is most likely one in all the freshest JavaScript libraries. It can be used to create dynamic and responsive programs, lets in for upper potency, and will also be merely extended. The underlying commonplace sense is in response to portions that can be reused in numerous contexts, decreasing the want to write the identical code numerous cases. In short, with React you’ll be capable of create environment friendly and robust packages.
So there hasn’t ever been a better time to learn how to create React programs.
On the other hand, with out a forged understanding of a couple of key JavaScript choices, development React programs may well be tricky or even unimaginable.
As a result of this, now we’ve got compiled a list of JavaScript choices and concepts that you need to grab previous than getting started with React. The easier you know the ones concepts, the simpler it’s going to be so to assemble professional React programs.
That being said, right here’s what we can speak about in this article:
JavaScript and ECMAScript
JavaScript is a popular scripting language used along side HTML and CSS to build dynamic web pages. While HTML is used to create the development of a web internet web page and CSS to create the way in which and structure of its portions, JavaScript is the language used as a way to upload behavior to the internet web page, i.e. to create capacity and interactivity.
The language has since been adopted by way of crucial browsers and a record used to be as soon as written to give an explanation for one of the simplest ways JavaScript used to be as soon as intended to art work: the ECMAScript same old.
As of 2015, an exchange of the ECMAScript usual is introduced once a year, and thus new choices are added to JavaScript every year.
ECMAScript 2015 used to be as soon as the sixth free up of the standard and is therefore steadily known as ES6. Following diversifications are marked in construction, so we seek advice from ECMAScript 2016 as ES7, ECMAScript 2017 as ES8, and so on.
As a result of the frequency with which new choices are added to the standard, some is probably not supported in all browsers. So how would possibly simply you ensure that the most recent JavaScript choices you added for your JS app would art work as expected during all web browsers?
You’ve got 3 alternatives:
- Wait until all primary browsers provide make stronger for the new choices. Then again in the event you occur to totally need that unbelievable new JS feature to your app, this isn’t an selection.
- Use a Polyfill, which is “a piece of code (maximum steadily JavaScript on the Web) used to offer stylish capacity on older browsers that don’t natively make stronger it” (see moreover mdn internet medical doctors).
- Use a JavaScript transpiler identical to Babel or Traceur, that convert ECMAScript 2015+ code proper right into a JavaScript fashion that is supported by way of all browsers.
Statements vs Expressions
Working out the variation between statements and expressions is essential when development React programs. So, permit us to go back to the basic concepts of programming for a 2d.
A computer program is a list of instructions to be completed by way of a computer. The ones instructions are known as statements.
By contrast to statements, expressions are fragments of code that produce a price. In a remark, an expression is an element that returns a price and we maximum steadily see it at the right kind side of an identical sign.
Whilst:
JavaScript statements will also be blocks or strains of code that the majority steadily end with semicolons or are enclosed in curly brackets.
Proper right here is a straightforward example of a remark in JavaScript:
record.getElementById("hello").innerHTML = "Hello World!";
The remark above writes "Hello World!"
in a DOM section with identity="hello"
.
As we already mentioned, expessions produce a price or are themselves a price. Imagine the following example:
msg = record.getElementById("hello").value;
record.getElementById("hello").value
is en expression as it returns a price.
An additional example must have the same opinion give an explanation for the variation between expressions and statements:
const msg = "Hello World!";
function sayHello( msg ) {
console.log( msg );
}
Inside the example above,
- the principle line is a remark, where
"Hello World!"
is an expression, - the function declaration is a remark, where the parameter
msg
passed to the function is an expression, - the street that prints the message inside the console is a remark, where yet again the parameter
msg
is an expression.
Why Expressions Are Very important in React
When construction a React software, you’ll be capable of inject JavaScript expressions into your JSX code. For example, you’ll be capable of transfer a variable, write an fit handler or a scenario. To take a look at this, you need to include your JS code in curly brackets.
For example, you’ll be capable of transfer a variable:
const Message = () => {
const name = "Carlo";
return Welcome {name}!
;
}
In short, the curly brackets tell your transpiler to process the code wrapped in brackets as JS code. The whole thing that comes previous than the opening
tag and after the general
tag is usual JavaScript code. The whole thing during the hole
and closing
tags is processed as JSX code.
Right here’s every other example:
const Message = () => {
const name = "Ann";
const heading = Welcome {name}
;
return (
{heading}
This is your dashboard.
);
}
You’ll moreover transfer an object:
render(){
const person = {
name: 'Carlo',
avatar: 'https://en.gravatar.com/userimage/954861/fc68a728946aac04f8531c3a8742ac22',
description: 'Content material subject matter Creator'
}
return (
Welcome {person.name}
Description: {person.description}.
);
}
And underneath is a additional whole example:
render(){
const person = {
name: 'Carlo',
avatar: 'https://en.gravatar.com/userimage/954861/fc68a728946aac04f8531c3a8742ac22?dimension=original',
description: 'Content material subject matter Creator',
theme: {
boxShadow: '0 4px 8px 0 rgba(0,0,0,0.2)', width: '200px'
}
}
return (
{person.name}
{person.description}.
);
}
Perceive the double curly brackets inside the style
attributes inside the portions img
and div
. We used double brackets to transport two units containing card and image varieties.
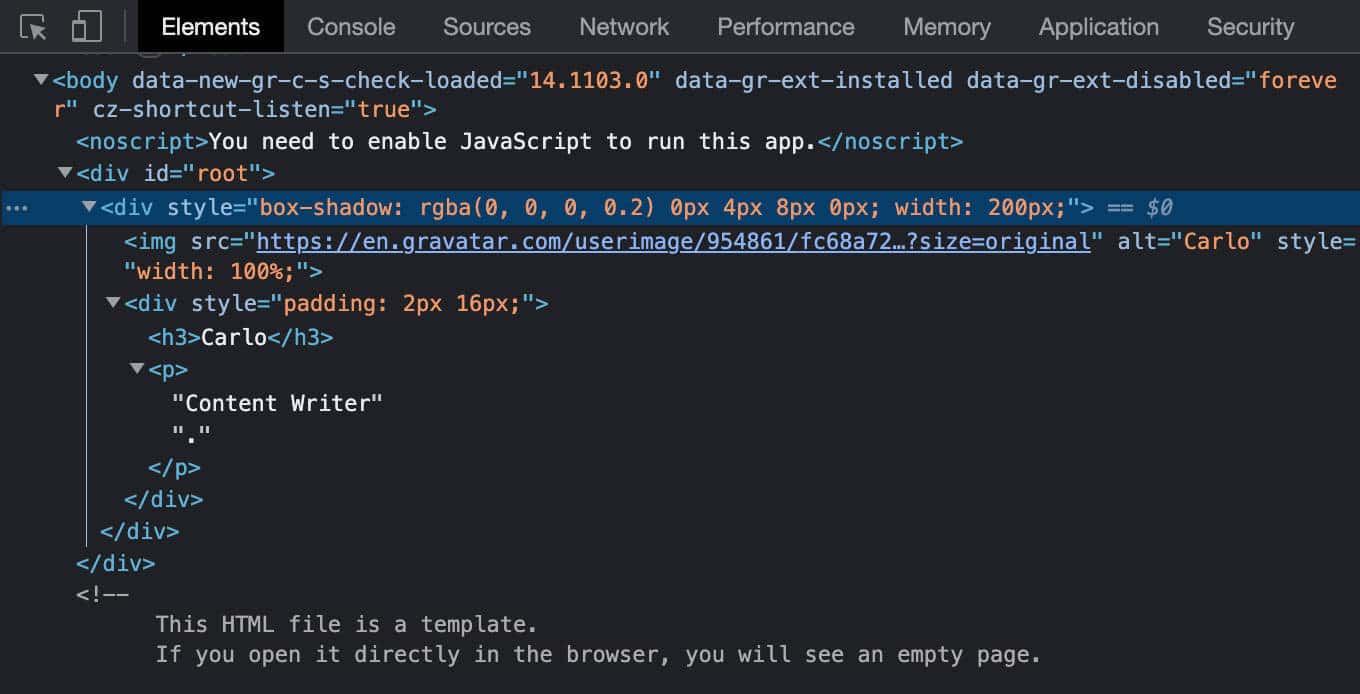
You’re going to have noticed that all through the entire examples above, we built-in JavaScript expressions in JSX.
Immutability in React
Mutability and Immutability are two key ideas in object-oriented and useful programming.
Immutability means that a price can’t be changed after it’s been created. Mutability formulation, if truth be told, the opposite.
In Javascript, primitive values are immutable, that signifies that after a primitive value is created, it could actually’t be changed. Conversely, arrays and units are mutable because of their homes and portions will also be changed without reassigning a brand spanking new value.
There are a selection of reasons for using immutable units in JavaScript:
- Complicated potency
- Decreased memory consumption
- Thread-safety
- Easier coding and debugging
Following the rage of immutability, once a variable or object is assigned, it could actually’t be re-assigned or changed. When you need to change wisdom, you’ll have to create a duplicate of it and change its content material subject matter, leaving the original content material subject matter unchanged.
Immutability could also be a key concept in React.
The React documentation states:
The state of a class component is available as
this.state
. The state field must be an object. Don’t mutate the state directly. If you wish to trade the state, callsetState
with the new state.
On each instance the state of a component changes, React calculates whether or not or to not re-render the component and exchange the Virtual DOM. If React didn’t have track of the previous state, it could not unravel whether or not or to not re-render the component or not. React documentation provides an very good instance of this.
What JavaScript choices can we use to make sure the immutability of the state object in React? Let’s find out!
Mentioning Variables
You’ve got three ways to assert a variable in JavaScript: var
, let
, and const
.
The var
observation exists since the beginning of JavaScript. It’s used to assert a function-scoped or globally-scoped variable, optionally initializing it to a price.
When you declare a variable using var
, you’ll be capable of re-declare and exchange that variable each and every inside the international and local scope. The following code is authorized:
// Declare a variable
var msg = "Hello!";
// Redeclare the identical variable
var msg = "Goodbye!"
// Exchange the variable
msg = "Hello yet again!"
var
declarations are processed previous than any code is completed. As a result of this, citing a variable any place inside the code is very similar to citing it at the top. This behavior is known as hoisting.
It’s value noting that only the variable declaration is hoisted, not the initialization, which only happens when the regulate go with the flow reaches the undertaking remark. Until that point, the variable is undefined
:
console.log(msg); // undefined
var msg = "Hello!";
console.log(msg); // Hello!
The scope of a var
declared in a JS function is the entire frame of that operate.
This means that that the variable isn’t defined at block level, on the other hand at the level of all of the function. This results in a large number of problems that can make your JavaScript code buggy and difficult to handle.
To fix the ones problems, ES6 introduced the let
key phrase.
The
let
declaration broadcasts a block-scoped local variable, optionally initializing it to a price.
What are the advantages of let
over var
? Listed below are some:
let
broadcasts a variable to the scope of a block remark, whilevar
broadcasts a variable globally or in the neighborhood to an entire function without reference to block scope.- Global
let
variables don’t appear to be homes of thewindow
object. You’ll’t get right of entry to them withwindow.variableName
. let
can only be accessed after its declaration is reached. The variable isn’t initialized until the control float reaches the street of code where it’s declared (let declarations are non-hoisted).- Redeclaring a variable with
let
throws aSyntaxError
.
Since variables declared using var
can’t be block-scoped, in the event you occur to stipulate a variable using var
in a loop or within an if
remark, it can be accessed from out of doors the block and this can lead to buggy code.
The code inside the first example is completed without errors. Now exchange var
with let
inside the block of code spotted above:
console.log(msg);
let msg = "Hello!";
console.log(msg);
In the second example, using let
as a substitute of var
produces an Uncaught ReferenceError
:

ES6 moreover introduces a third keyword: const
.
const
is gorgeous similar to let
, on the other hand with a key difference:
Imagine the following example:
const MAX_VALUE = 1000;
MAX_VALUE = 2000;
The above code would generate the following TypeError:
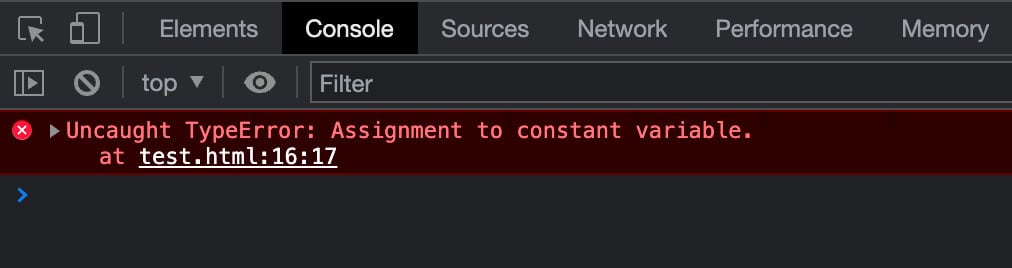
In addition to:
Mentioning a const
without giving it a price would throw the following SyntaxError
(see moreover ES6 In Intensity: let and const):

But if a unbroken is an array or an object, you’ll be capable of edit homes or items within that array or object.
For example, you’ll be capable of trade, add, and remove array items:
// Declare a unbroken array
const cities = ["London", "New York", "Sydney"];
// Industry an products
cities[0] = "Madrid";
// Add an products
cities.push("Paris");
// Remove an products
cities.pop();
console.log(cities);
// Array(3)
// 0: "Madrid"
// 1: "New York"
// 2: "Sydney"
Then again you don’t appear to be allowed to reassign the array:
const cities = ["London", "New York", "Sydney"];
cities = ["Athens", "Barcelona", "Naples"];
The code above would result in a TypeError.

You’ll add, reassign, and remove object homes and methods:
// Declare a unbroken obj
const post = {
identity: 1,
name: 'JavaScript is awesome',
excerpt: 'JavaScript is a superb scripting language',
content material subject matter: 'JavaScript is a scripting language that permits you to create dynamically updating content material subject matter.'
};
// add a brand spanking new property
post.slug = "javascript-is-awesome";
// Reassign property
post.identity = 5;
// Delete a property
delete post.excerpt;
console.log(post);
// {identity: 5, name: 'JavaScript is awesome', content material subject matter: 'JavaScript is a scripting language that permits you to create dynamically updating content material subject matter.', slug: 'javascript-is-awesome'}
Then again you don’t appear to be allowed to reassign the object itself. The following code would go through an Uncaught TypeError
:
// Declare a unbroken obj
const post = {
identity: 1,
name: 'JavaScript is awesome',
excerpt: 'JavaScript is a superb scripting language'
};
post = {
identity: 1,
name: 'React is robust',
excerpt: 'React means that you can assemble individual interfaces'
};
Object.freeze()
We now agree that using const
does not at all times make sure that strong immutability (in particular when operating with units and arrays). So, how can you put in force the immutability pattern for your React programs?
First, when you wish to have to prevent the elements of an array or homes of an object from being modified, you’ll be capable of use the static system Object.freeze()
.
Freezing an object prevents extensions and makes present homes non-writable and non-configurable. A frozen object can no longer be changed: new homes can’t be added, present homes can’t be removed, their enumerability, configurability, writability, or value can’t be changed, and the object’s prototype can’t be re-assigned.
freeze()
returns the identical object that used to be as soon as passed in.
Any attempt to add, trade or remove a property will fail, each silently or by way of throwing a TypeError
, most continuously in strict mode.
You’ll use Object.freeze()
this fashion:
'use strict'
// Declare a unbroken obj
const post = {
identity: 1,
name: 'JavaScript is awesome',
excerpt: 'JavaScript is a superb scripting language'
};
// Freeze the object
Object.freeze(post);
For many who now try to add a property, you’ll download an Uncaught TypeError
:
// Add a brand spanking new property
post.slug = "javascript-is-awesome"; // Uncaught TypeError
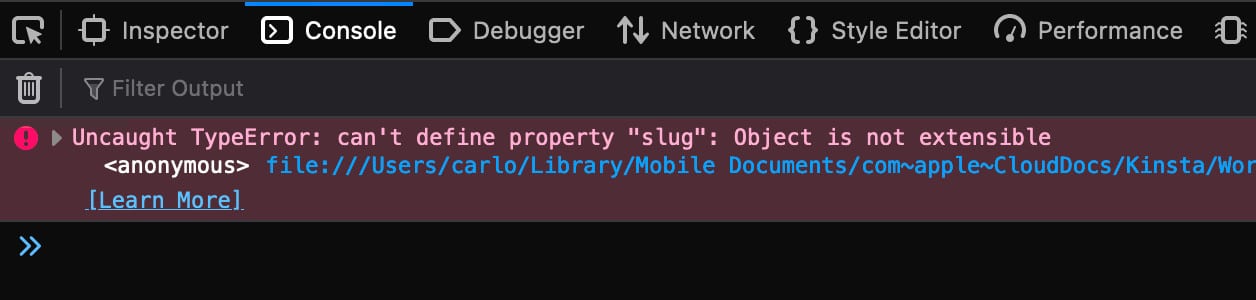
When you try to reassign a property, you get every other kind of TypeError
:
// Reassign property
post.identity = 5; // Uncaught TypeError
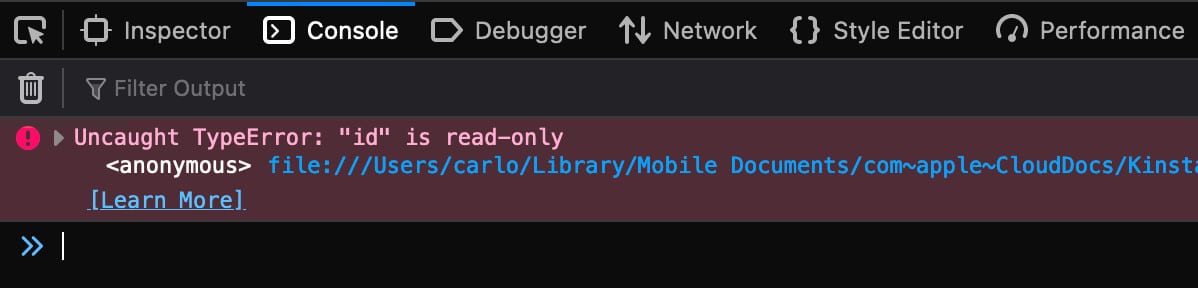
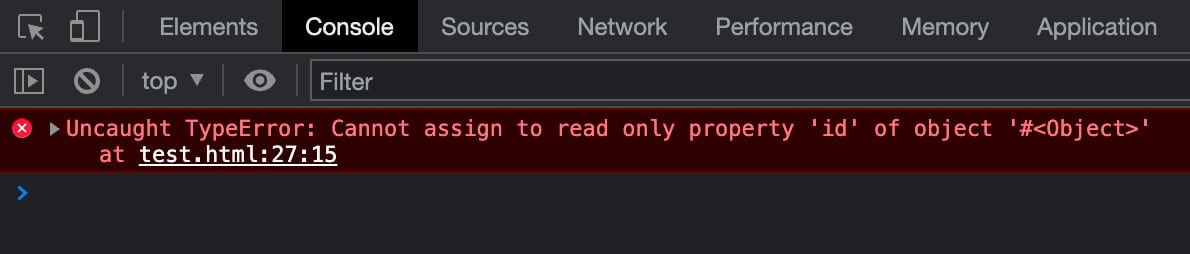
You’ll moreover try to delete a property. The result it will be every other TypeError
:
// Delete a property
delete post.excerpt; // Uncaught TypeError
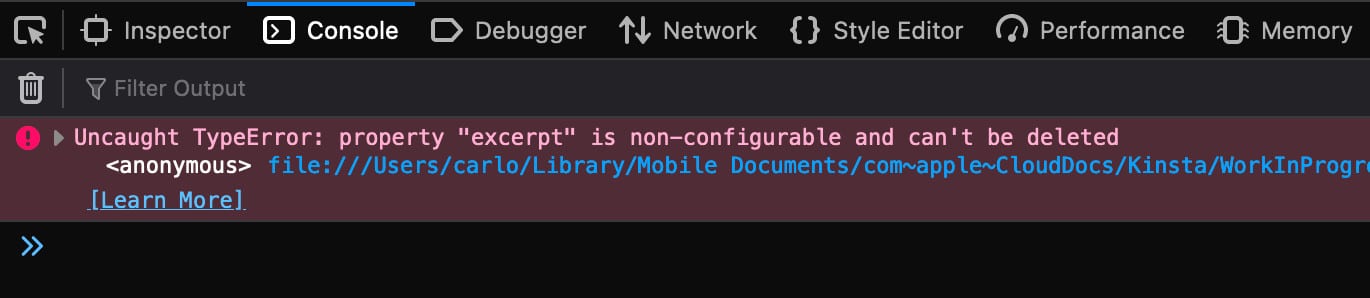
Template Literals
When you need to combine strings with the output of expressions in JavaScript, you maximum steadily use the addition operator +
. On the other hand, you’ll be capable of moreover use a JavaScript feature that permits you to include expressions within strings without using the addition operator: Template Literals.
Template Literals are a singular kind of strings delimited with backtick (`
) characters.
In Template Literals you’ll be capable of include placeholders, which can be embedded expressions delimited by way of a dollar personality and wrapped in curly brackets.
Right here’s an example:
const align = 'left';
console.log(`This string is ${ align }-aligned`);
The strings and placeholders get passed to a default function that performs string interpolation to modify the placeholders and concatenate the parts proper right into a single string. You’ll moreover exchange the default function with a custom designed function.
You’ll use Template Literals for:
Multi-line strings: newline characters are part of the template literal.
console.log(`Twinkle, twinkle, little bat!
How I am questioning what you’re at!`);
String interpolation: Without Template Literals, you’ll be capable of only use the addition operator to combine the output of expressions with strings. See the following example:
const a = 3;
const b = 7;
console.log("The result of " + a + " + " + b + " is " + (a + b));
It’s moderately sophisticated, isn’t it? Then again you’ll be capable of write that code in a additional readable and maintainable means using Template Literals:
const a = 3;
const b = 7;
console.log(`The result of ${ a } + ${ b } is ${ a + b }`);
Then again keep in mind that there’s a distinction between the 2 syntaxes:
Template Literals lend themselves to numerous uses. Inside the following example, we use a ternary operator to assign a price to a magnificence
feature.
const internet web page = 'archive';
console.log(`magnificence=${ internet web page === 'archive' ?
'archive' : 'single' }`);
Underneath, we’re showing a simple calculation:
const value = 100;
const VAT = 0.22;
console.log(`Total value: ${ (value * (1 + VAT)).toFixed(2) }`);
It’s additionally conceivable to nest Template Literals by way of along side them within an ${expression}
placeholder (on the other hand use nested templates with warning because of sophisticated string structures could also be onerous to be told and handle).
Tagged templates: As we mentioned above, it’s generally conceivable to stipulate a custom designed function to perform string concatenation. This type of Template Literal is known as Tagged Template.
Tags assist you to parse template literals with a function. The principle argument of a tag function incorporates an array of string values. The rest arguments are related to the expressions.
Tags assist you to parse template literals with a custom designed function. The principle argument of this function is an array of the strings built-in inside the Template Literal, the other arguments are the expressions.
You’ll create a custom designed function to perform any form of operation on the template arguments and return the manipulated string. Right here’s an overly elementary example of tagged template:
const name = "Carlo";
const operate = "student";
const staff = "North Pole School";
const age = 25;
function customFunc(strings, ...tags) {
console.log(strings); // ['My name is ', ", I'm ", ', and I am ', ' at ', '', raw: Array(5)]
console.log(tags); // ['Carlo', 25, 'student', 'North Pole University']
let string = '';
for ( let i = 0; i < strings.length - 1; i++ ){
console.log(i + "" + strings[i] + "" + tags[i]);
string += strings[i] + tags[i];
}
return string.toUpperCase();
}
const output = customFunc`My name is ${name}, I'm ${age}, and I am ${operate} at ${staff}`;
console.log(output);
The code above prints the strings
and tags
array portions then capitalizes the string characters previous than printing the output inside the browser console.
Arrow Functions
Arrow functions are an alternative to anonymous functions (functions without names) in JavaScript on the other hand with some diversifications and limitations.
The following declarations are all legit Arrow Functions examples:
// Arrow function without parameters
const myFunction = () => expression;
// Arrow function with one parameter
const myFunction = param => expression;
// Arrow function with one parameter
const myFunction = (param) => expression;
// Arrow function with additional parameters
const myFunction = (param1, param2) => expression;
// Arrow function without parameters
const myFunction = () => {
statements
}
// Arrow function with one parameter
const myFunction = param => {
statements
}
// Arrow function with additional parameters
const myFunction = (param1, param2) => {
statements
}
Chances are high that you can disregard the round brackets in the event you occur to only transfer one parameter to the function. For many who transfer two or additional parameters, you must enclose them in brackets. Here is an example of this:
const render = ( identity, determine, elegance ) => `${identity}: ${determine} - ${elegance}`;
console.log( render ( 5, 'Hello World!', "JavaScript" ) );
One-line Arrow Functions return a price by way of default. For many who use the multiple-line syntax, you will have to manually return a price:
const render = ( identity, determine, elegance ) => {
console.log( `Publish determine: ${ determine }` );
return `${ identity }: ${ determine } - ${ elegance }`;
}
console.log( `Publish details: ${ render ( 5, 'Hello World!', "JavaScript" ) }` );
One key difference between usual functions and Arrow Functions to bear in mind is that Arrow functions don’t have their own bindings to the important thing word this
. For many who try to use this
in an Arrow Function, it’ll pass out of doors the function scope.
For a greater description of Arrow functions and examples of use, be told moreover mdn internet medical doctors.
Classes
Categories in JavaScript are a singular type of function for rising units that use the prototypical inheritance mechanism.
In step with mdn internet medical doctors,
With regards to inheritance, JavaScript only has one bring together: units. Each object has a personal property which holds a link to a few different object known as its prototype. That prototype object has a prototype of its non-public, and so on until an object is reached with
null
as its prototype.
As with functions, it’s essential have two ways of defining a class:
- A class expression
- A class declaration
You can use the magnificence
keyword to stipulate a class within an expression, as confirmed inside the following example:
const Circle = magnificence {
constructor(radius) {
this.radius = Amount(radius);
}
area() {
return Math.PI * Math.pow(this.radius, 2);
}
circumference() {
return Math.PI * this.radius * 2;
}
}
console.log('Circumference: ' + new Circle(10).circumference()); // 62.83185307179586
console.log('Area: ' + new Circle(10).area()); // 314.1592653589793
A class has a body, that is the code built-in in curly brakets. Proper right here you’ll define constructor and methods, which can be additionally known as magnificence individuals. The body of the class is completed in strict mode even without using the 'strict mode'
directive.
The constructor
device is used for rising and initializing an object created with a class and is routinely completed when the class is instantiated. For many who don’t define a constructor device for your magnificence, JavaScript will routinely use a default constructor.
A class will also be extended using the extends
keyword.
magnificence Guide {
constructor(determine, author) {
this.booktitle = determine;
this.authorname = author;
}
supply() {
return this.booktitle + ' is a brilliant book from ' + this.authorname;
}
}
magnificence BookDetails extends Guide {
constructor(determine, author, cat) {
super(determine, author);
this.elegance = cat;
}
show() {
return this.supply() + ', it is a ' + this.elegance + ' book';
}
}
const bookInfo = new BookDetails("The Fellowship of the Ring", "J. R. R. Tolkien", "Fantasy");
console.log(bookInfo.show());
A constructor can use the super
keyword to call the mummy or dad constructor. For many who transfer a topic to the super()
device, this argument can be available inside the father or mother constructor magnificence.
For a deeper dive into JavaScript classes and several other different examples of usage, see moreover the mdn internet medical doctors.
Classes are often used to create React portions. Most often, you’ll not create your individual classes on the other hand quite prolong built-in React classes.
All categories in React have a render()
device that returns a React section:
magnificence Animal extends React.Part {
render() {
return Hey, I am a {this.props.name}!
;
}
}
Inside the example above, Animal
is a class component. Believe that
- The decision of the component must get started with a capital letter
- The component must include the expression
extends React.Part
. This provides get right of entry to to the methods of theReact.Part
. - The
render()
device returns the HTML and is wanted.
Once you have created your magnificence component, you are able to render the HTML on the internet web page:
const root = ReactDOM.createRoot(record.getElementById('root'));
const section = ;
root.render(section);
The image underneath presentations the end result on the internet web page (You can see it in motion on CodePen).
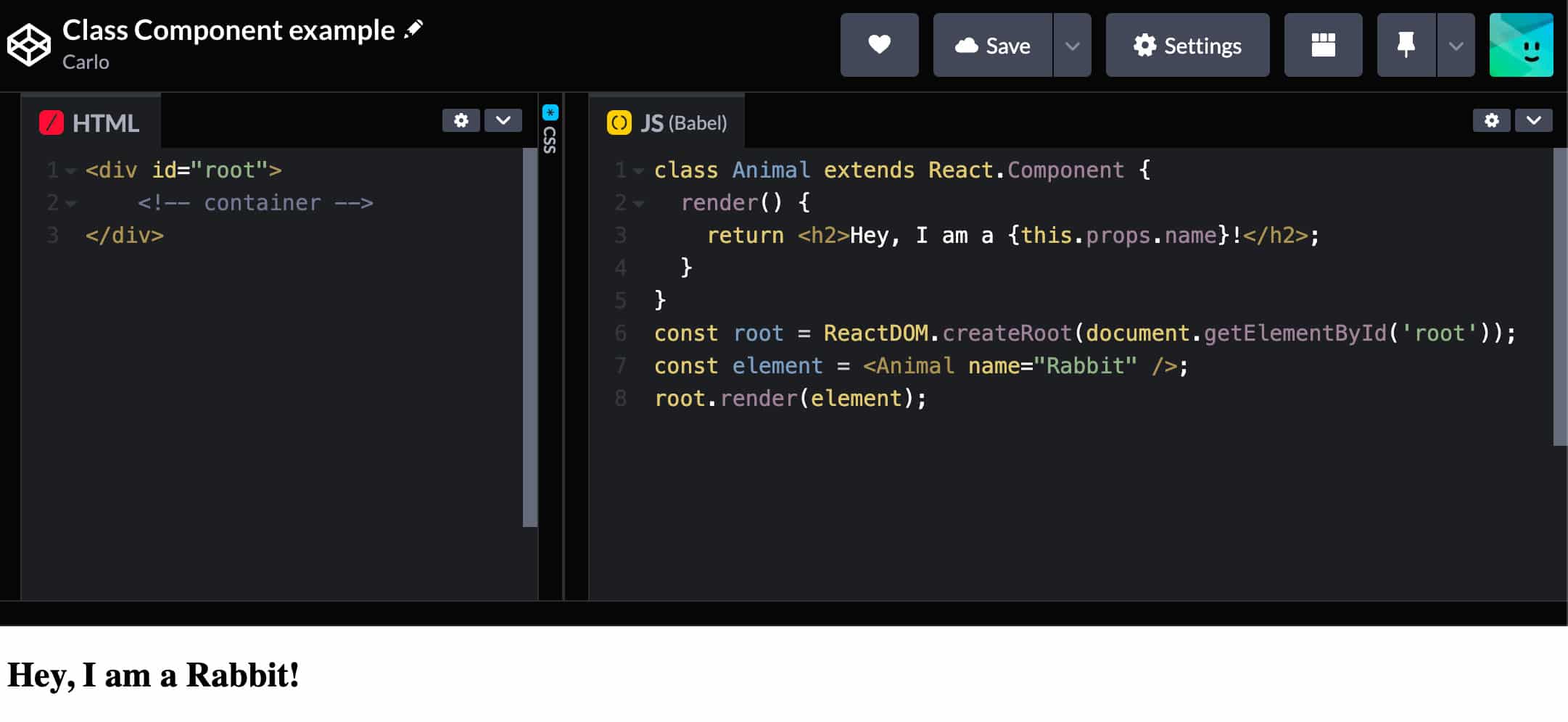
Follow, however, that the usage of elegance parts in React isn’t advisable and it’s preferable defining parts as purposes.
The Keyword ‘this’
In JavaScript, the this
keyword is a generic placeholder maximum steadily used within units, classes, and functions, and it refers to different portions depending on the context or scope.
this
can be used inside the international scope. For many who digit this
for your browser’s console, you get:
Window {window: Window, self: Window, record: record, name: '', location: Location, ...}
You can get right of entry to any of the methods and homes of the Window
object. So, in the event you occur to run this.location
for your browser’s console, you get the following output:
Location {ancestorOrigins: DOMStringList, href: 'https://kinsta.com/', beginning position: 'https://kinsta.com', protocol: 'https:', host: 'kinsta.com', ...}
When you use this
in an object, it refers to the object itself. In this means, you are able to seek advice from the values of an object inside the methods of the object itself:
const post = {
identity: 5,
getSlug: function(){
return `post-${this.identity}`;
},
determine: 'Awesome post',
elegance: 'JavaScript'
};
console.log( post.getSlug );
Now let’s try to use this
in a function:
const useThis = function () {
return this;
}
console.log( useThis() );
For many who aren’t in strict mode, you’ll get:
Window {window: Window, self: Window, record: record, name: '', location: Location, ...}
Then again in the event you occur to invoke strict mode, you get a distinct finish outcome:
const doSomething = function () {
'use strict';
return this;
}
console.log( doSomething() );
In this case, the function returns undefined
. That’s because of this
in a function refers to its explicit value.
So methods to explicitly set this
in a function?
First, you are able to manually assign homes and how you can the function:
function doSomething( post ) {
this.identity = post.identity;
this.determine = post.determine;
console.log( `${this.identity} - ${this.determine}` );
}
new doSomething( { identity: 5, determine: 'Awesome post' } );
Then again you are able to moreover use call
, follow
, and bind
methods, along with arrow functions.
const doSomething = function() {
console.log( `${this.identity} - ${this.determine}` );
}
doSomething.call( { identity: 5, determine: 'Awesome post' } );
The call()
device can be used on any function and does exactly what it says: it calls the function.
Additionally, call()
accepts each different parameter defined inside the function:
const doSomething = function( cat ) {
console.log( `${this.identity} - ${this.determine} - Magnificence: ${cat}` );
}
doSomething.call( { identity: 5, determine: 'Awesome post' }, 'JavaScript' );
const doSomething = function( cat1, cat2 ) {
console.log( `${this.identity} - ${this.determine} - Categories: ${cat1}, ${cat2}` );
}
doSomething.follow( { identity: 5, determine: 'Awesome post' }, ['JavaScript', 'React'] );
const post = { identity: 5, determine: 'Awesome post', elegance: 'JavaScript' };
const doSomething = function() {
return `${this.identity} - ${this.determine} - ${this.elegance}`;
}
const bindRender = doSomething.bind( post );
console.log( bindRender() );
An alternative to the selections discussed above is using arrow purposes.
Arrow function expressions must only be used for non-method functions because of they do not have their own
this
.
This makes arrow functions particularly useful with fit handlers.
That’s because of “when the code is known as from an inline fit handler feature, its this
is able to the DOM section on which the listener is situated” (see mdn internet medical doctors).
Then again problems trade with arrow functions because of…
… arrow functions decide
this
in response to the scope the arrow function is printed within, and thethis
value does not trade in response to how the function is invoked.
Binding ‘this’ to Match Handlers in React
With regards to React, it’s essential have a few ways to ensure that the improvement handler does not lose its context:
1. Using bind()
during the render device:
import React, { Part } from 'react';
magnificence MyComponent extends Part {
state = { message: 'Hello World!' };
showMessage(){
console.log( 'This refers to: ', this );
console.log( 'The message is: ', this.state.message );
}
render(){
return( );
}
}
export default MyComponent;
2. Binding the context to the improvement handler inside the constructor:
import React, { Part } from 'react';
magnificence MyComponent extends Part {
state = { message: 'Hello World!' };
constructor(props) {
super(props);
this.showMessage = this.showMessage.bind( this );
}
showMessage(){
console.log( 'This refers to: ', this );
console.log( 'The message is: ', this.state.message );
}
render(){
return( );
}
}
export default MyComponent;
3. Define the improvement handler using arrow functions:
import React, { Part } from 'react';
magnificence MyComponent extends Part {
state = { message: 'Hello World!' };
showMessage = () => {
console.log( 'This refers to: ', this );
console.log( 'The message is: ', this.state.message );
}
render(){
return( );
}
}
export default MyComponent;
4. Using arrow functions inside the render device:
import React, { Part } from 'react';
magnificence MyComponent extends Part {
state = { message: 'Hello World!' };
showMessage() {
console.log( 'This refers to: ', this );
console.log( 'The message is: ', this.state.message );
}
render(){
return( );
}
}
export default MyComponent;
Whichever device you choose, whilst you click on at the button, the browser console presentations the following output:
This refers to: MyComponent {props: {…}, context: {…}, refs: {…}, updater: {…}, state: {…}, …}
The message is: Hello World!
Ternary Operator
The conditional operator (or ternary operator) lets you write simple conditional expressions in JavaScript. It takes 3 operands:
- a scenario followed by way of a question mark (
?
), - an expression to execute if the placement is truthy followed by way of a semicolon (
:
), - a 2d expression to execute if the placement is falsy.
const drink = personAge >= 18 ? "Wine" : "Juice";
It is also conceivable to chain numerous expressions:
const drink = personAge >= 18 ? "Wine" : personAge >= 6 ? "Juice" : "Milk";
Be careful, despite the fact that, because of chaining numerous expressions can lead to messy code that is tricky to handle.
The ternary operator is particularly useful in React, in particular for your JSX code, which only accepts expressions in curly brackets.
For example, you are able to use the ternary operator to set the cost of an feature in response to a selected scenario:
render(){
const person = {
name: 'Carlo',
avatar: 'https://en.gravatar.com/...',
description: 'Content material subject matter Creator',
theme: 'gentle'
}
return (
{person.name}
{person.description}.
);
}
Inside the code above, we take a look at the placement person.theme === 'dark'
to set the cost of the style
feature of the container div
.
Fast Circuit Research
The logical AND (&&
) operator evaluates operands from left to right kind and returns true
if and supplied that every one operands are true
.
The logical AND is a short-circuit operator. Each operand is reworked to a boolean and, if the result of the conversion is false
, the AND operator stops and returns the original value of the falsy operand. If all values are true
, it returns the original value of the remainder operand.
Fast circuit research is a JavaScript feature continuously used in React as it lets you output blocks of code in response to specific conditions. Right here is an example:
{
displayExcerpt &&
post.excerpt.rendered && (
{ post.excerpt.rendered }
)
}
Inside the code above, if displayExcerpt
AND post.excerpt.rendered
analysis to true
, React returns the overall block of JSX.
To recap, “if the placement is true
, the section right kind after &&
will appear inside the output. If it is false
, React will fail to remember about and skip it”.
Spread Syntax
In JavaScript, unfold syntax lets you lengthen an iterable section, identical to an array or object, into function arguments, array literals, or object literals.
Inside the following example, we are unpacking an array in a function call:
function doSomething( x, y, z ){
return `First: ${x} - 2d: ${y} - third: ${z} - Sum: ${x+y+z}`;
}
const numbers = [3, 4, 7];
console.log( doSomething( ...numbers ) );
You can use the spread syntax to copy an array (even multidimensional arrays) or to concatenate arrays. Inside the following examples, we concatenate two arrays in two different ways:
const firstArray = [1, 2, 3];
const secondArray = [4, 5, 6];
firstArray.push( ...secondArray );
console.log( firstArray );
On the other hand:
let firstArray = [1, 2, 3];
const secondArray = [4, 5, 6];
firstArray = [ ...firstArray, ...secondArray];
console.log( firstArray );
You can moreover use the spread syntax to clone or merge two units:
const firstObj = { identity: '1', determine: 'JS is awesome' };
const secondObj = { cat: 'React', description: 'React is simple' };
// clone object
const thirdObj = { ...firstObj };
// merge units
const fourthObj = { ...firstObj, ...secondObj }
console.log( { ...thirdObj } );
console.log( { ...fourthObj } );
Destructuring Mission
Every other syntactic development you’ll often find used in React is the destructuring project syntax.
Inside the following example, we unpack values from an array:
const individual = ['Carlo', 'Content writer', 'Kinsta'];
const [name, description, company] = individual;
console.log( `${name} is ${description} at ${company}` );
And proper right here is a straightforward example of destructuring undertaking with an object:
const individual = {
name: 'Carlo',
description: 'Content material subject matter author',
company: 'Kinsta'
}
const { name, description, company } = individual;
console.log( `${name} is ${description} at ${company}` );
Then again we can do a lot more. Inside the following example, we unpack some homes of an object and assign the rest homes to a few different object using the spread syntax:
const individual = {
name: 'Carlo',
family: 'Daniele',
description: 'Content material subject matter author',
company: 'Kinsta',
power: 'swimming'
}
const { name, description, company, ...recreational } = individual;
console.log( recreational ); // {family: 'Daniele', power: 'swimming'}
You can moreover assign values to an array:
const individual = [];
const object = { name: 'Carlo', company: 'Kinsta' };
( { name: individual[0], company: individual[1] } = object );
console.log( individual ); // (2) ['Carlo', 'Kinsta']
Follow that the parentheses around the undertaking remark are required when using object literal destructuring undertaking with out a declaration.
For a greater analysis of destructuring undertaking, with numerous examples of use, please seek advice from the mdn internet medical doctors.
clear out(), map(), and cut back()
JavaScript provides numerous useful methods you’ll find often used in React.
clear out()
Inside the following example, we follow the clear out to the numbers
array to get an array whose portions are numbers greater than 5:
const numbers = [2, 6, 8, 2, 5, 9, 23];
const finish outcome = numbers.clear out( amount => amount > 5);
console.log(finish outcome); // (4) [6, 8, 9, 23]
Inside the following example, we get an array of posts with the word ‘JavaScript’ built-in inside the determine:
const posts = [
{id: 0, title: 'JavaScript is awesome', content: 'your content'},
{id: 1, title: 'WordPress is easy', content: 'your content'},
{id: 2, title: 'React is cool', content: 'your content'},
{id: 3, title: 'With JavaScript to the moon', content: 'your content'},
];
const jsPosts = posts.clear out( post => post.determine.comprises( 'JavaScript' ) );
console.log( jsPosts );
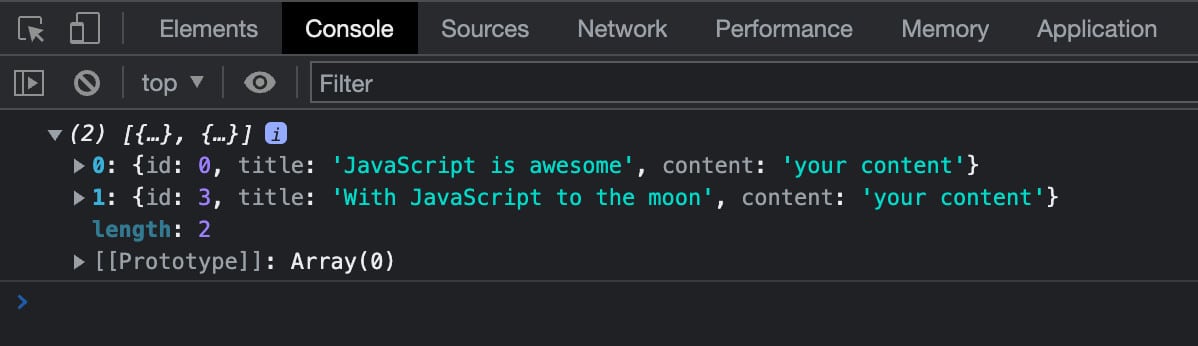
map()
const numbers = [2, 6, 8, 2, 5, 9, 23];
const finish outcome = numbers.map( amount => amount * 5 );
console.log(finish outcome); // (7) [10, 30, 40, 10, 25, 45, 115]
In a React component, you’ll often find the map()
device used to build lists. Inside the following example, we’re mapping the WordPress posts
object to construct a listing of posts:
{ posts && posts.map( ( post ) => {
return (
-
)
})}
cut back()
cut back()
accepts two parameters:
- A callback function to execute for each section inside the array. It returns a price that becomes the cost of the accumulator parameter on the next call. On the final call, the function returns the fee that will be the return value of
cut back()
. - An initial value that is the first value of the accumulator passed to the callback function.
The callback function takes a few parameters:
- An accumulator: The associated fee returned from the previous call to the callback function. On the first call, it’s set to an initial value if specified. Another way, it takes the cost of the principle products of the array.
- The cost of the provide section: The associated fee is able to the principle a part of the array (
array[0]
) if an initial value has been set, in a different way it takes the cost of the second section (array[1]
). - The provide index is the index position of the current section.
An example will make the whole thing clearer.
const numbers = [1, 2, 3, 4, 5];
const initialValue = 0;
const sumElements = numbers.cut back(
( accumulator, currentValue ) => accumulator + currentValue,
initialValue
);
console.log( numbers ); // (5) [1, 2, 3, 4, 5]
console.log( sumElements ); // 15
Let’s find out in detail what happens at each iteration. Go back to the previous example and change the initialValue
:
const numbers = [1, 2, 3, 4, 5];
const initialValue = 5;
const sumElements = numbers.cut back(
( accumulator, currentValue, index ) => {
console.log('Accumulator: ' + accumulator + ' - currentValue: ' + currentValue + ' - index: ' + index);
return accumulator + currentValue;
},
initialValue
);
console.log( sumElements );
The following image presentations the output inside the browser console:
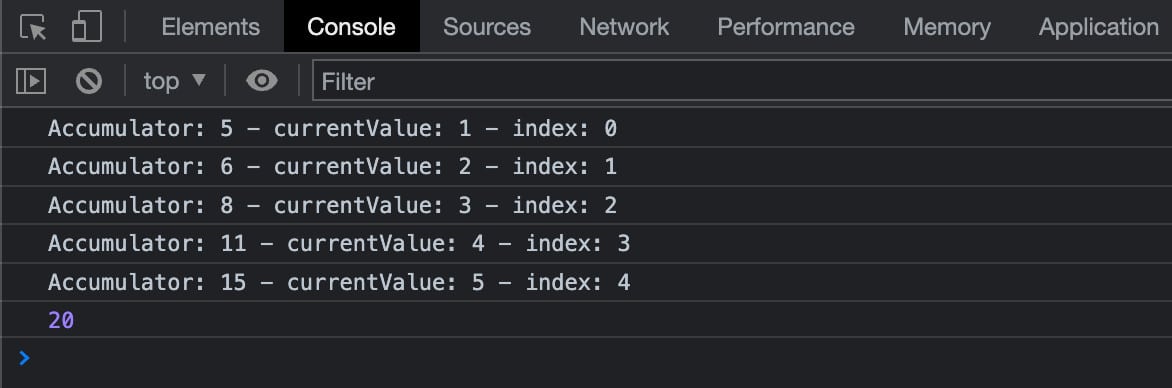
Now let us find out what happens without the initialValue
parameter:
const numbers = [1, 2, 3, 4, 5];
const sumElements = numbers.cut back(
( accumulator, currentValue, index ) => {
console.log( 'Accumulator: ' + accumulator + ' - currentValue: ' + currentValue + ' - index: ' + index );
return accumulator + currentValue;
}
);
console.log( sumElements );
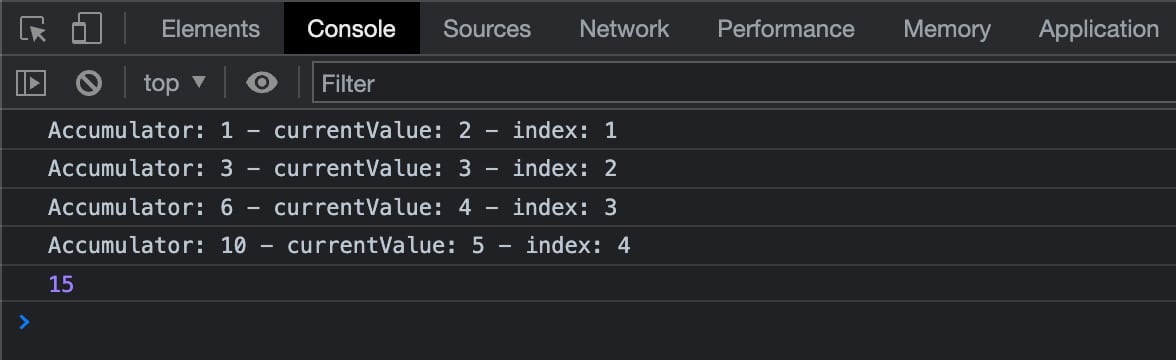
Additional examples and use instances are discussed on the mdn internet medical doctors website.
Exports and Imports
As of ECMAScript 2015 (ES6), it is conceivable to export values from a JavaScript module and import them into every other script. You’ll be using imports and exports extensively for your React programs and therefore you will need to have a superb understanding of how they art work.
The following code creates a useful component. The principle line imports the React library:
import React from 'react';
function MyComponent() {
const person = {
name: 'Carlo',
avatar: 'https://en.gravatar.com/userimage/954861/fc68a728946aac04f8531c3a8742ac22?dimension=original',
description: 'Content material subject matter Creator',
theme: 'dark'
}
return (
{ person.name }
{ person.description }.
);
}
export default MyComponent;
We used the import
keyword followed by way of the decision we want to assign to what we are importing, followed by way of the decision of the package deal we want to arrange as it is referred to inside the package deal.json record.
Follow that inside the MyComponent()
function above, we used probably the most JavaScript choices discussed inside the previous sections. We built-in property values in curly brackets and assigned the cost of the style
property using the conditional operator syntax.
The script ends with the export of our custom designed component.
Now that everyone knows moderately additional about imports and exports, let’s take a greater check out how they art work.
Export
Every React module may have two various kinds of export: named export and default export.
For example, you are able to export numerous choices immediately with a single export
remark:
export { MyComponent, MyVariable };
You can moreover export specific individual choices (function
, magnificence
, const
, let
):
export function MyComponent() { ... };
export let myVariable = x + y;
Then again you are able to only have a single default export:
export default MyComponent;
You can moreover use default export for specific individual choices:
export default function() { ... }
export default magnificence { ... }
Import
As quickly because the component has been exported, it can be imported into every other record, e.g. an index.js record, along side other modules:
import React from 'react';
import ReactDOM from 'react-dom/consumer';
import './index.css';
import MyComponent from './MyComponent';
const root = ReactDOM.createRoot( record.getElementById( 'root' ) );
root.render(
);
Inside the code above, we used the import declaration in a large number of ways.
Inside the first two strains, we assigned a name to the imported property, inside the third line we did not assign a name on the other hand simply imported the ./index.css record. The remainder import
remark imports the ./MyComponent record and assigns a name.
Let’s find out the diversities between those imports.
Usually, there are 4 sorts of imports:
Named import
import { MyFunction, MyVariable } from "./my-module";
Default import
import MyComponent from "./MyComponent";
Namespace import
import * as name from "my-module";
Facet have an effect on import
import "module-name";
Once you have added a few varieties for your index.css, your card must appear to be inside the image underneath, where you are able to moreover see the corresponding HTML code:
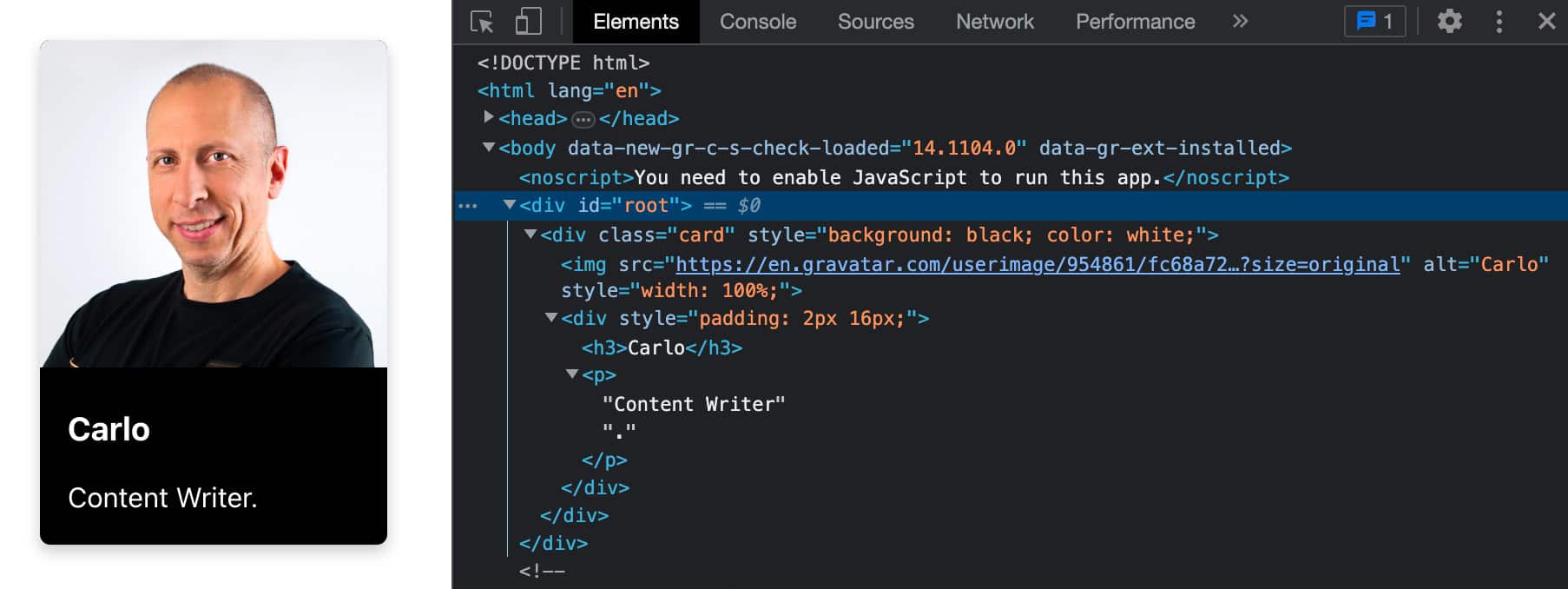
Follow that import
declarations can only be used in modules at the top level (not within functions, classes, and lots of others.).
For a additional whole analysis of import
and export
statements, you may also want to take a look at the following property:
- export (mdn web scientific medical doctors)
- import (mdn web scientific medical doctors)
- Uploading and Exporting Elements (React dev)
- When will have to I take advantage of curly braces for ES6 import? (Stack Overflow)
Summary
React is without doubt one of the most in style JavaScript libraries in recent years and is without doubt one of the most asked abilities in the world of internet building.
With React, it is conceivable to create dynamic web programs and complex interfaces. Growing large, dynamic, and interactive programs will also be easy on account of its reusable portions.
Then again React is a JavaScript library, and a superb understanding of the principle choices of JavaScript is essential to start out your journey with React. That’s why we’ve amassed in one place probably the most JavaScript choices you’ll find most often used in React. Mastering those choices will give you a leg as much as your React studying adventure.
And in relation to internet building, making the switch from JS/React to WordPress takes very little effort.
Now it’s your turn, what JavaScript choices do you think are most beneficial in React development? Have we neglected the remaining very important that you would have preferred to seem on our tick list? Share your concepts with us inside the comments underneath.
The post JavaScript Options You Want to Know to Grasp React gave the impression first on Kinsta®.
Contents
- 1 JavaScript and ECMAScript
- 2 Statements vs Expressions
- 3 Welcome {person.name}
- 4 Immutability in React
- 5 Template Literals
- 6 Arrow Functions
- 7 Classes
- 8 Hey, I am a {this.props.name}!
- 9 The Keyword ‘this’
- 10 Ternary Operator
- 11 Fast Circuit Research
- 12 Spread Syntax
- 13 Destructuring Mission
- 14 clear out(), map(), and cut back()
- 15 Exports and Imports
- 16 Summary
- 17 The usage of Elementary Captcha vs ReCaptcha in Divi’s Touch Shape Module
- 18 How to Use AI to Create Amazing Web Design with Divi (Using ChatGPT & MidJourney)
- 19 How you can Upload Tags to YouTube Movies & Why They are Necessary
0 Comments