As digital transactions upward thrust, the facility to seamlessly mix worth gateways has turn into a critical talent for developers. Whether or not or now not for marketplaces or SaaS merchandise, a finances processor is crucial to collect and process client finances.
This article explains learn the way to mix Stripe within a Spring Boot atmosphere, learn the way to prepare subscriptions, offer unfastened trials, and assemble self-service pages to your customers to procure their worth invoices.
What Is Stripe?
Stripe is a globally renowned worth processing platform available in 46 international locations. This is a great variety if you want to assemble a worth integration for your web app owing to its large reach, reputed name, and detailed documentation.
Working out Not unusual Stripe Concepts
It’s helpful to grab some now not strange concepts that Stripe uses to coordinate and carry out worth operations between multiple occasions. Stripe provides two approaches for implementing worth integration for your app.
You’ll each embed Stripe’s paperwork within your app for an in-app purchaser experience (Fee Intent) or redirect customers to a Stripe-hosted worth internet web page, where Stripe manages the process and lets your app know when a worth is a good fortune or failed (Fee Hyperlink).
Rate Intent
When coping with finances, it’s essential to assemble purchaser and product details prematurely previous than prompting them for card wisdom and worth. The ones details encompass the description, basic amount, mode of worth, and further.
Stripe calls so that you can collect the ones details within your app and generate a PaymentIntent
object on their backend. This fashion lets in Stripe to formulate a worth request for that intent. After the fee concludes, you’ll continuously retrieve worth details, along with its serve as, all through the PaymentIntent
object.
Rate Links
To keep away from the complexities of integrating Stripe immediately into your codebase, consider the use of Stripe Checkouts for a hosted worth answer. Like creating a PaymentIntent
, you’ll create a CheckoutSession
with worth and purchaser details. As a substitute of starting an in-app PaymentIntent
, the CheckoutSession
generates a worth link where you redirect your customers. That’s what a hosted worth internet web page seems like:
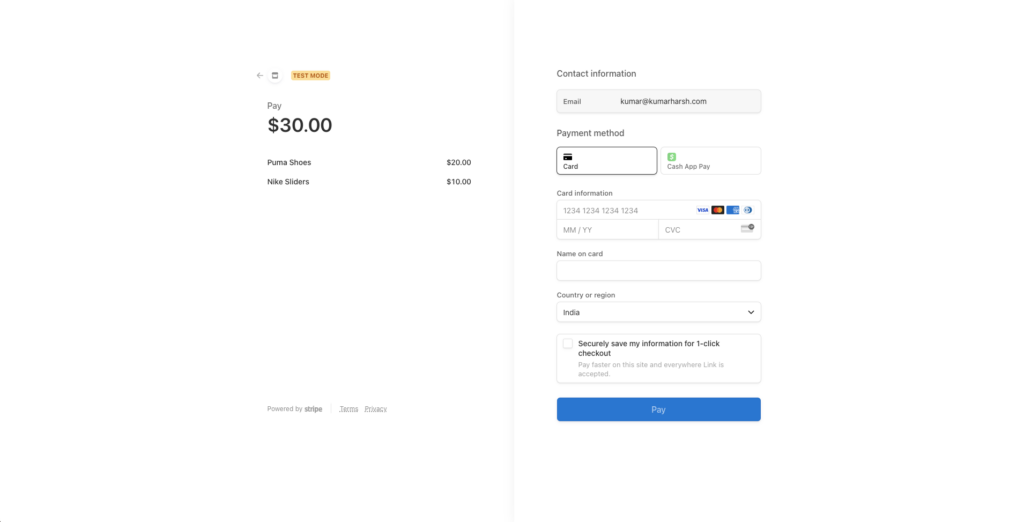
After worth, Stripe redirects once more to your app, enabling post-payment tasks very similar to confirmations and provide requests. For reliability, configure a backend webhook to interchange Stripe, ensuring worth wisdom retention despite the fact that customers accidentally close the internet web page after worth.
While environment friendly, the program lacks flexibility in customization and design. It may be difficult to prepare appropriately for cell apps, where a neighborhood integration would look far more seamless.
API Keys
When operating with the Stripe API, you’re going to need get right to use to API keys to your client and server apps to have interaction with the Stripe backend. You’ll get right to use your Stripe API keys on your Stripe developer dashboard. Proper right here’s what it would look like:
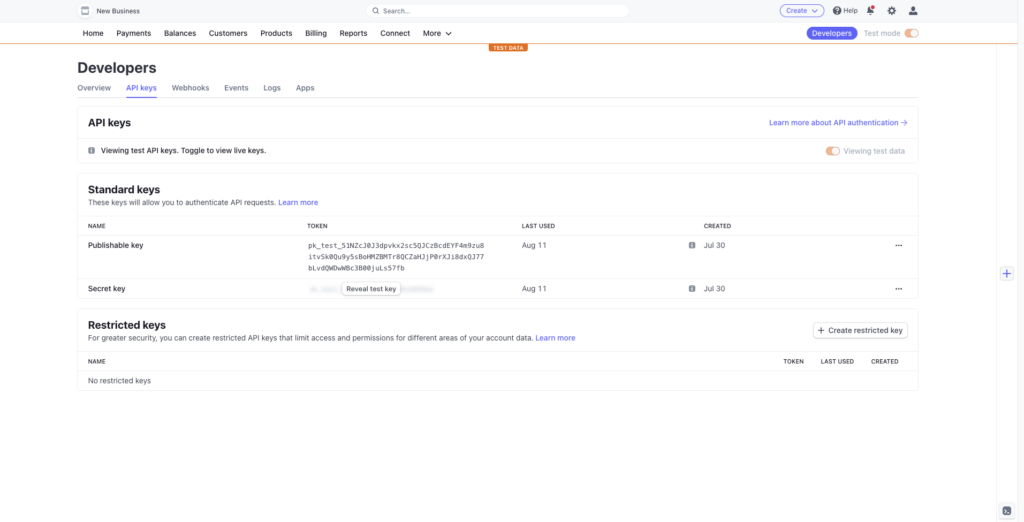
How Do Expenses in Stripe Art work?
To know how finances in Stripe artwork, you want to grab all the stakeholders involved. 4 stakeholders are captivated with every worth transaction:
- Purchaser: The person who intends to pay for a service/product.
- Carrier supplier: You, the business owner, are accountable for receiving finances and selling services and products/products.
- Acquirer: A monetary establishment that processes finances on behalf of you (the carrier supplier) and routes your worth request to your purchaser’s banks. Acquirers would possibly partner with a third birthday party to be in agreement process finances.
- Issuing monetary establishment: The monetary establishment that extends credit score rating and issues taking part in playing cards and other worth learn the way to customers.
Proper right here’s a typical worth float between the ones stakeholders at a very over the top level.

The buyer lets the carrier supplier know they’re ready to pay. The carrier supplier then forwards the payment-related details to their acquiring monetary establishment, which collects the fee from the patron’s issuing monetary establishment and lets the carrier supplier know the fee used to be as soon as a good fortune.
This can be a very high-level evaluation of the fee process. As a carrier supplier, you most efficient want to concern about amassing the fee intent, passing it at once to the fee processor, and coping with the fee end result. However, as discussed earlier, there are two tactics it is advisable cross about it.
When creating a Stripe-managed checkout session where Stripe takes care of your worth details collection, proper right here’s what the usual float seems like:
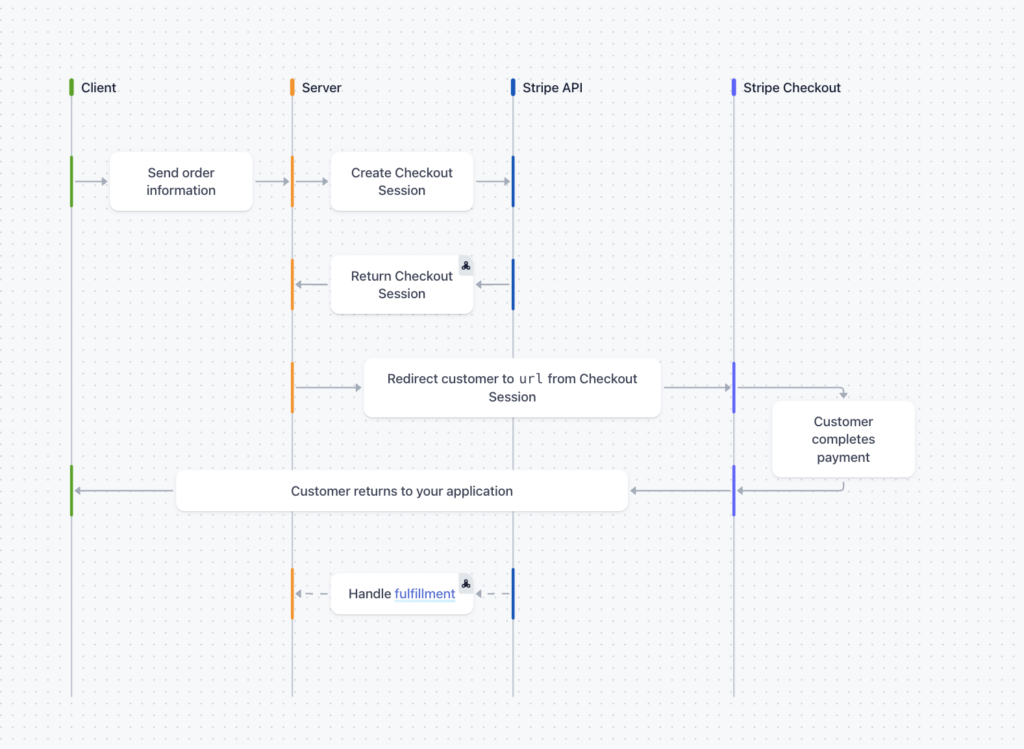
With custom designed worth flows, it’s really up to you. You’ll design the interaction between your client, server, purchaser, and the Stripe API in keeping with your app’s needs. You’ll add deal with collection, invoice era, cancellation, unfastened trials, and so on., to this workflow as you want.
Now that you understand how Stripe finances artwork, you’re able to start out out building it for your Java tool.
Stripe Integration in Spring Boot Instrument
To begin out the Stripe integration, create a frontend app to have interaction with the Java backend and get started up finances. In this educational, you’ll assemble a React app to motive various worth varieties and subscriptions in order that you reach a clear figuring out of their mechanisms.
Remember: This educational won’t cover building a complete ecommerce website; it’s necessarily geared toward guiding you all through the easy process of integrating Stripe into Spring Boot.
Setting Up the Frontend and Backend Duties
Create a brand spanking new list and scaffold a React project using Vite by way of running the following command:
npm create vite@latest
Set the project name as frontend (or any preferred name), framework as React and variant as TypeScript. Navigate to the project list and arrange Chakra UI for speedy scaffolding of UI portions by way of running the following command:
npm i @chakra-ui/react @emotion/react @emotion/styled framer-motion @chakra-ui/icons
You’re going to moreover arrange react-router-dom
for your project for client-side routing by way of running the command beneath:
npm i react-router-dom
Now, you’re able to start out out building your frontend app. Proper right here’s the homepage you’re going to assemble.
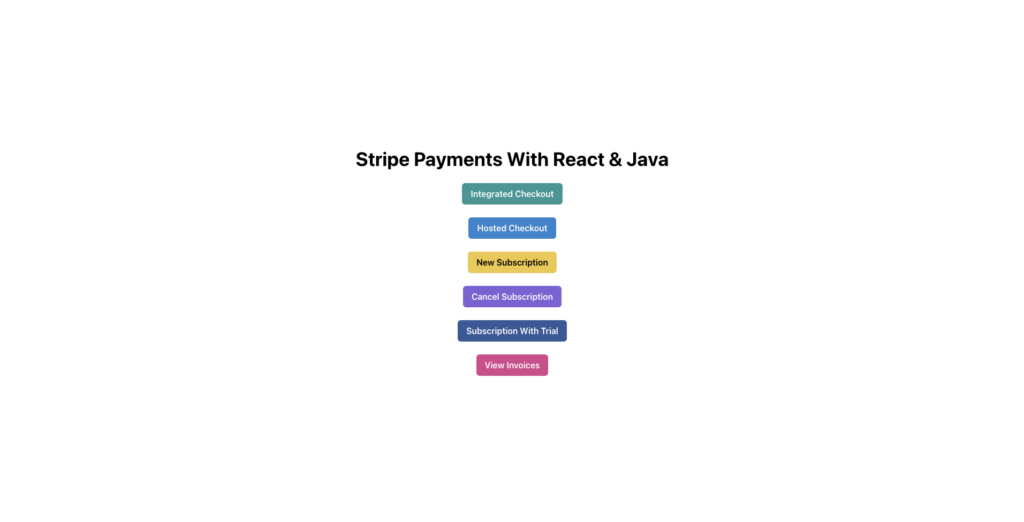
Clicking any button on this internet web page will take you to separate checkout pages with worth paperwork. To begin out, create a brand spanking new folder named routes for your frontend/src list. Inside of this folder, create a Area.tsx report. This report will grab the code to your app’s area path (/
). Paste the following code into the report:
import {Button, Middle, Heading, VStack} from "@chakra-ui/react";
import { useNavigate } from "react-router-dom";
function Area() {
const navigate = useNavigate()
const navigateToIntegratedCheckout = () => {
navigate("/integrated-checkout")
}
const navigateToHostedCheckout = () => {
navigate("/hosted-checkout")
}
const navigateToNewSubscription = () => {
navigate("/new-subscription")
}
const navigateToCancelSubscription = () => {
navigate("/cancel-subscription")
}
const navigateToSubscriptionWithTrial = () => {
navigate("/subscription-with-trial")
}
const navigateToViewInvoices = () => {
navigate("/view-invoices")
}
return (
Stripe Expenses With React & Java
)
}
export default Area
To permit navigation for your app, substitute your App.tsx report to configure the RouteProvider
class from react-router-dom
.
import Area from "./routes/Area.tsx";
import {
createBrowserRouter,
RouterProvider,
} from "react-router-dom";
function App() {
const router = createBrowserRouter([
{
path: "/",
element: (
),
},
]);
return (
)
}
export default App
Run the npm run dev
command to preview your tool on https://localhost:5173.
This completes the initial setup sought after for the frontend app. Next, create a backend app using Spring Boot. To initialize the app, you’ll use the spring initializr web site (If your IDE is helping creating Spring apps, you don’t want to use the web site).
IntelliJ IDEA is helping creating Spring Boot apps. Get began by way of choosing the New Undertaking selection on IntelliJ IDEA. Then, select Spring Initializr from the left pane. Input your backend project details: name (backend), location (stripe-payments-java list), language (Java), and kind (Maven). For group and artifact names, use com.kinsta.stripe-java and backend, respectively.
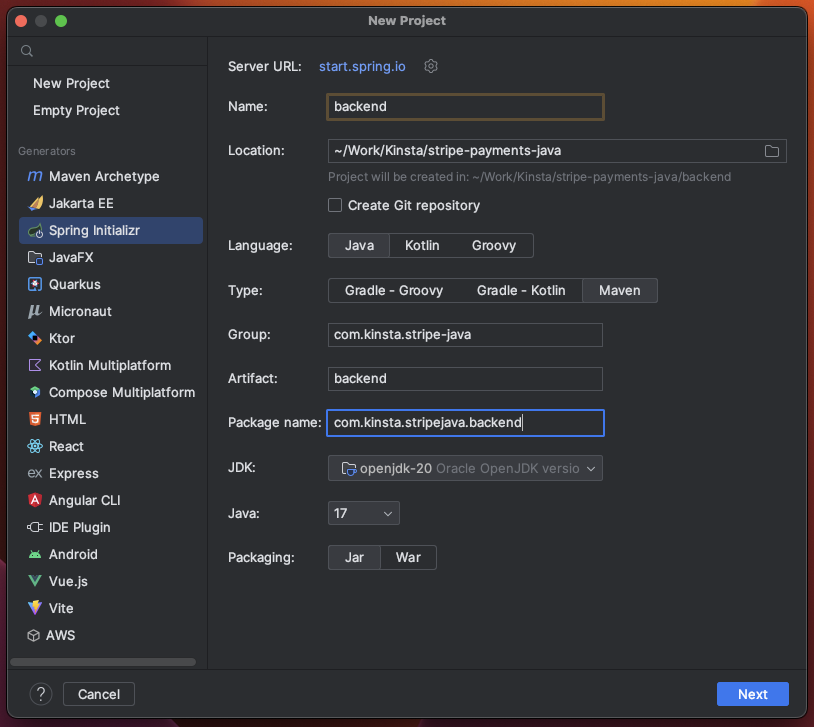
Click on on Next button. Then, add dependencies to your project by way of choosing Spring Web from the Web dropdown inside the dependencies pane and click on at the Create button.
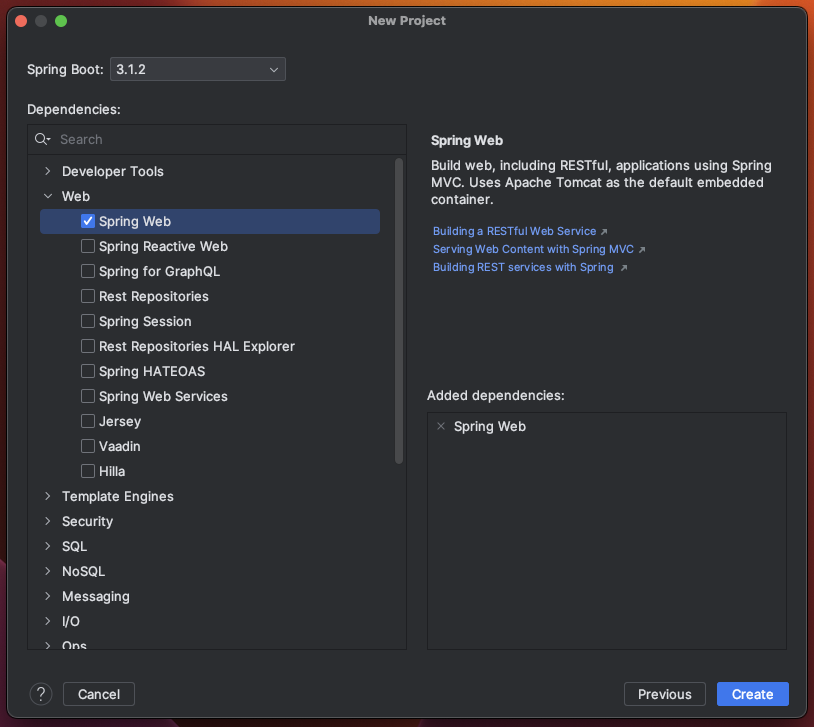
This may occasionally create the Java project and open it for your IDE. You’ll now proceed with creating the various checkout flows using Stripe.
Accepting Online Expenses for Product Purchases
The most important and widely used capacity of Stripe is to easily settle for one-off finances from customers. In this segment, you’re going to learn two tactics to mix worth processing for your app with Stripe.
Hosted Checkout
First, you assemble a checkout internet web page that triggers a hosted worth workflow where you most efficient motive a worth from your frontend app. Then Stripe takes care of amassing the patron’s card details and amassing the fee and most efficient shares the result of the fee operation at the end.
That’s what the checkout internet web page would look like:
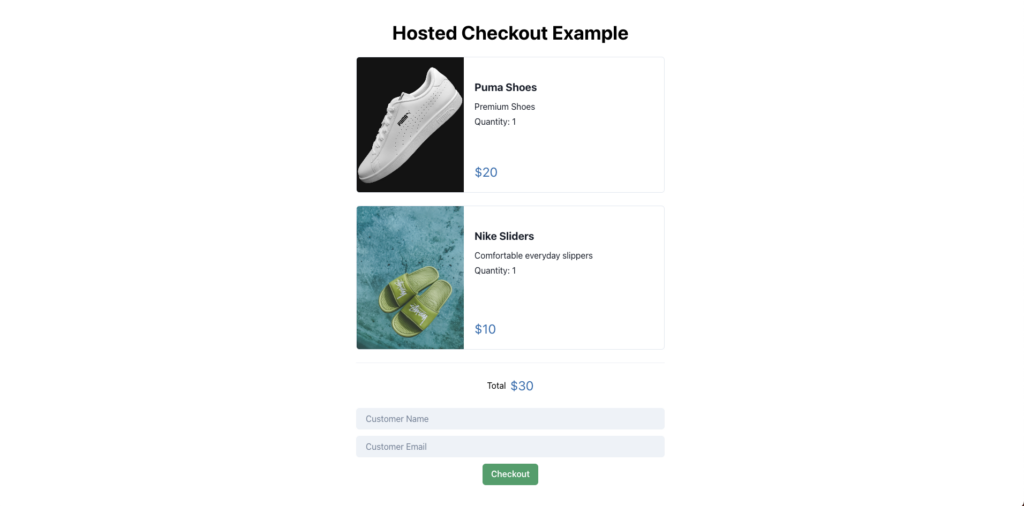
This internet web page has 3 primary portions: CartItem
— represents every cart products; TotalFooter
— displays the entire amount; CustomerDetails
— collects purchaser details. You to reuse the ones portions to build checkout paperwork for various scenarios in this article, very similar to incorporated checkout and subscriptions.
Building the Frontend
Create a portions folder for your frontend/src list. Inside the portions folder, create a brand spanking new report CartItem.tsx and paste the following code:
import {Button, Card, CardBody, CardFooter, Heading, Image, Stack, Text, VStack} from "@chakra-ui/react";
function CartItem(props: CartItemProps) {
return
{props.wisdom.name}
{props.wisdom.description}
{(props.mode === "checkout" ?
{"Quantity: " + props.wisdom.quantity}
: )}
{"$" + props.wisdom.price}
}
export interface ItemData {
name: string
price: amount
quantity: amount
image: string
description: string
id: string
}
interface CartItemProps "checkout"
onCancelled?: () => void
export default CartItem
The code above defines two interfaces to use as varieties for the homes passed to the part. The ItemData
type is exported for reuse in numerous portions.
The code returns a cart products part’s structure. It uses the equipped props to render the thing on the computer screen.
Next, create a TotalFooter.tsx report inside the portions list and paste the following code:
import {Divider, HStack, Text} from "@chakra-ui/react";
function TotalFooter(props: TotalFooterProps) {
return
Basic
{"$" + props.basic}
{props.mode === "subscription" &&
(Per month, starting nowadays)
}
{props.mode === "trial" &&
(Per month, starting next month)
}
}
interface TotalFooterProps "trial"
export default TotalFooter
The TotalFooter
part displays the entire price for the cart and uses the mode
price to conditionally render particular text.
Finally, create the CustomerDetails.tsx
part and paste the following code:
import {ItemData} from "./CartItem.tsx";
import {Button, Input, VStack} from "@chakra-ui/react";
import {useState} from "react";
function CustomerDetails(props: CustomerDetailsProp) {
const [name, setName] = useState("")
const [email, setEmail] = useState("")
const onCustomerNameChange = (ev: React.ChangeEvent) => {
setName(ev.objective.price)
}
const onCustomerEmailChange = (ev: React.ChangeEvent) => {
setEmail(ev.objective.price)
}
const initiatePayment = () => {
fetch(process.env.VITE_SERVER_BASE_URL + props.endpoint, {
method: "POST",
headers: {'Content material material-Kind': 'tool/json'},
body: JSON.stringify({
items: props.wisdom.map(elem => ({name: elem.name, id: elem.id})),
customerName: name,
customerEmail: electronic mail,
})
})
.then(r => r.text())
.then(r => {
window.location.href = r
})
}
return
}
interface CustomerDetailsProp {
wisdom: ItemData[]
endpoint: string
}
export default CustomerDetails
The code above displays a type with two input fields — to collect the patron’s name and electronic mail. When the Checkout button is clicked, the initiatePayment
method is invoked to send the checkout request to the backend.
It requests the endpoint that you just’ve passed to the part and sends the patron’s wisdom and cart items as part of the request, then redirects the patron to the URL gained from the server. This URL will lead the patron to a checkout internet web page hosted on Stripe’s server. You’re going to get to building this URL in a bit of bit.
Remember: This part uses the environment variable VITE_SERVER_BASE_URL
for the backend server URL. Set it by way of creating .env report inside the root of your project:
VITE_SERVER_BASE_URL=http://localhost:8080
All portions have been created. Now, let’s proceed to build the hosted checkout path using the portions. To take a look at this, create a brand spanking new HostedCheckout.tsx report for your routes folder with the following code:
import {Middle, Heading, VStack} from "@chakra-ui/react";
import {useState} from "react";
import CartItem, {ItemData} from "../portions/CartItem.tsx";
import TotalFooter from "../portions/TotalFooter.tsx";
import CustomerDetails from "../portions/CustomerDetails.tsx";
import {Products} from '../wisdom.ts'
function HostedCheckout() {
const [items] = useState(Products)
return
Hosted Checkout Example
{items.map(elem => {
return
})}
}
export default HostedCheckout
This path uses the three portions you’ve merely constructed to assemble a checkout computer screen. All part modes are configured as checkout, and the endpoint /checkout/hosted
is provided to the form part for starting the checkout request appropriately.
The part uses a Products
object to fill the items array. In real-world scenarios, this knowledge comes from your cart API, containing the patron’s determined on items. However, for this educational, a static list from a script populates the array. Define the array by way of creating a wisdom.ts report at your frontend project’s root and storing the following code within it:
import {ItemData} from "./portions/CartItem.tsx";
export const Products: ItemData[] = [
{
description: "Premium Shoes",
image: "https://source.unsplash.com/NUoPWImmjCU",
name: "Puma Shoes",
price: 20,
quantity: 1,
id: "shoe"
},
{
description: "Comfortable everyday slippers",
image: "https://source.unsplash.com/K_gIPI791Jo",
name: "Nike Sliders",
price: 10,
quantity: 1,
id: "slippers"
},
]
This report defines two items inside the products array which may also be rendered inside the cart. Be happy to tweak the values of the products.
For the reason that final step of making the frontend, create two new routes to care for just right fortune and failure. The Stripe-hosted checkout internet web page would redirect consumers into your app on the ones two routes in keeping with the transaction end result. Stripe might also provide your routes with transaction-related payload, such since the checkout session ID, which you’ll use to retrieve the corresponding checkout consultation object and get right to use checkout hooked up wisdom identical to the fee method, invoice details, and so on.
To take a look at this, create a Good fortune.tsx report inside the src/routes list and save the following code in it:
import {Button, Middle, Heading, Text, VStack} from "@chakra-ui/react";
import {useNavigate} from "react-router-dom";
function Good fortune() {
const queryParams = new URLSearchParams(window.location.search)
const navigate = useNavigate()
const onButtonClick = () => {
navigate("/")
}
return
Good fortune!
{queryParams.toString().get a divorce("&").join("n")}
}
export default Good fortune
Upon rendering, this part shows the “Good fortune!” message and prints any URL query parameters on the computer screen. It moreover includes a button to redirect consumers to the application’s homepage.
When building real-world apps, this internet web page is where it’s possible you’ll care for non-critical app-side transactions that depend on the just right fortune of the transaction to hand. As an example, if you are building a checkout internet web page for an web store, it is advisable use this internet web page to show a confirmation to the patron and an ETA on when their purchased products may well be delivered.
Next, create a Failure.tsx report with the following code in it:
import {Button, Middle, Heading, Text, VStack} from "@chakra-ui/react";
import {useNavigate} from "react-router-dom";
function Failure() {
const queryParams = new URLSearchParams(window.location.search)
const navigate = useNavigate()
const onButtonClick = () => {
navigate("/")
}
return
Failure!
{queryParams.toString().get a divorce("&").join("n")}
}
export default Failure
This part is similar to that of Good fortune.tsx and displays the “Failure!” message when rendered.
For crucial tasks very similar to product provide, sending emails, or any critical part of your achieve float, use webhooks. Webhooks are API routes for your server that Stripe can invoke when a transaction occurs.
The webhook receives whole transaction details (by way of the CheckoutSession
object), allowing you to log it into your app database and motive corresponding just right fortune or failure workflows. As your server is always available to Stripe, no transactions are overpassed, ensuring your online store’s consistent capacity.
Finally, substitute the App.tsx report to make it look like this:
import Area from "./routes/Area.tsx";
import {createBrowserRouter, RouterProvider,} from "react-router-dom";
import HostedCheckout from "./routes/HostedCheckout.tsx";
import Good fortune from "./routes/Good fortune.tsx";
import Failure from "./routes/Failure.tsx";
function App() {
const router = createBrowserRouter([
{
path: "/",
element: (
),
},
{
path: "/hosted-checkout",
element: (
)
},
{
path: '/success',
element: (
)
},
{
path: '/failure',
element: (
)
},
]);
return (
)
}
export default App
This may occasionally be sure that the Good fortune
and Failure
portions are rendered on the /just right fortune
and /failure
routes, respectively.
This completes the frontend setup. Next, organize the backend to create the /checkout/hosted
endpoint.
Building the Backend
Open the backend project and arrange the Stripe SDK by way of together with the following lines inside the dependencies array for your pom.xml report:
com.stripe
stripe-java
22.29.0
Next, load Maven adjustments for your project to place in dependencies. If your IDE doesn’t make stronger this all through the UI, execute each the maven dependency:unravel
or maven arrange
command. While you don’t have the maven
CLI, use the mvnw
wrapper from Spring initializr while you create the project.
Once dependencies are installed, create a brand spanking new REST controller to care for incoming HTTP requests to your backend app. To take a look at this, create a PaymentController.java report inside the src/primary/java/com/kinsta/stripe-java/backend list and add the following code:
package deal deal com.kinsta.stripejava.backend;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.kind.Purchaser;
import com.stripe.kind.Product;
import com.stripe.kind.checkout.Session;
import com.stripe.param.checkout.SessionCreateParams;
import com.stripe.param.checkout.SessionCreateParams.LineItem.PriceData;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RestController;
@RestController
@CrossOrigin
public class PaymentController {
String STRIPE_API_KEY = Instrument.getenv().get("STRIPE_API_KEY");
@PostMapping("/checkout/hosted")
String hostedCheckout(@RequestBody RequestDTO requestDTO) throws StripeException {
return "Hello World!";
}
}
The code above imports crucial Stripe dependencies and establishes the PaymentController
class. This class carries two annotations: @RestController
and @CrossOrigin
. The @RestController
annotation instructs Spring Boot to treat this class as a controller, and its methods can now use @Mapping
annotations to care for incoming HTTP requests.
The @CrossOrigin
annotation marks all endpoints defined in this class as open to all origins underneath the CORS rules. However, this practice is discouraged in production on account of potential protection vulnerabilities from various internet domains.
For max results, it’s truly helpful to host every backend and frontend servers on the similar space to avoid CORS problems. Then again, if this isn’t imaginable, you’ll specify the world of your frontend client (which sends requests to the backend server) using the @CrossOrigin
annotation, like this:
@CrossOrigin(origins = "http://frontend.com")
The PaymentController
class will extract the Stripe API key from atmosphere variables to provide to the Stripe SDK later. When running the application, you will have to provide your Stripe API key to the app by the use of atmosphere variables.
Locally, you’ll create a brand spanking new atmosphere variable for your gadget each briefly (by way of together with a KEY=VALUE
phrase previous than the command used to start out out your building server) or totally (by way of updating your terminal’s config knowledge or environment an environment variable inside the keep watch over panel in House home windows).
In production environments, your deployment provider (very similar to Kinsta) will give you a separate method to fill inside the atmosphere variables used by your tool.
Should you’re using IntelliJ IDEA (or a similar IDE), click on on Run Configurations at the top correct of the IDE and click on on Edit Configurations… selection from the dropdown list that opens to interchange your run command and set the environment variable.
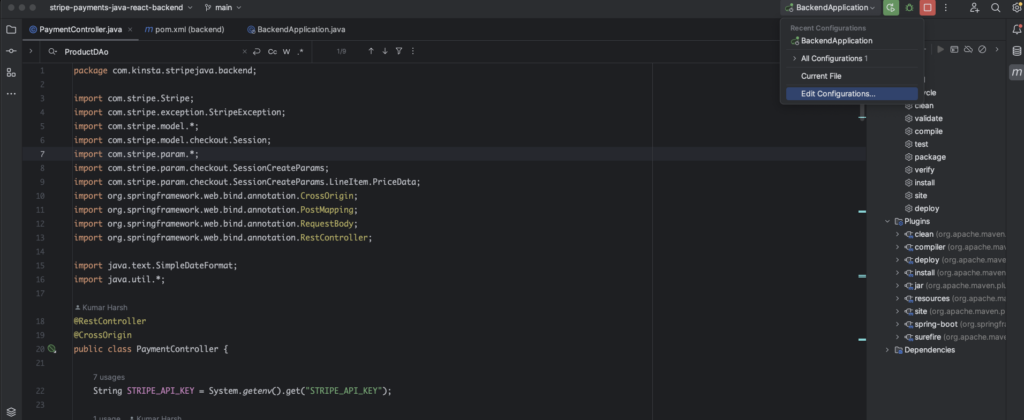
This may occasionally open up a dialog where you’ll provide the atmosphere variables to your app using the Environment variables field. Enter the environment variable STRIPE_API_KEY
inside the construction VAR1=VALUE
. You’ll to seek out your API key on the Stripe Builders site. You will have to provide the price of the Secret Key from this internet web page.
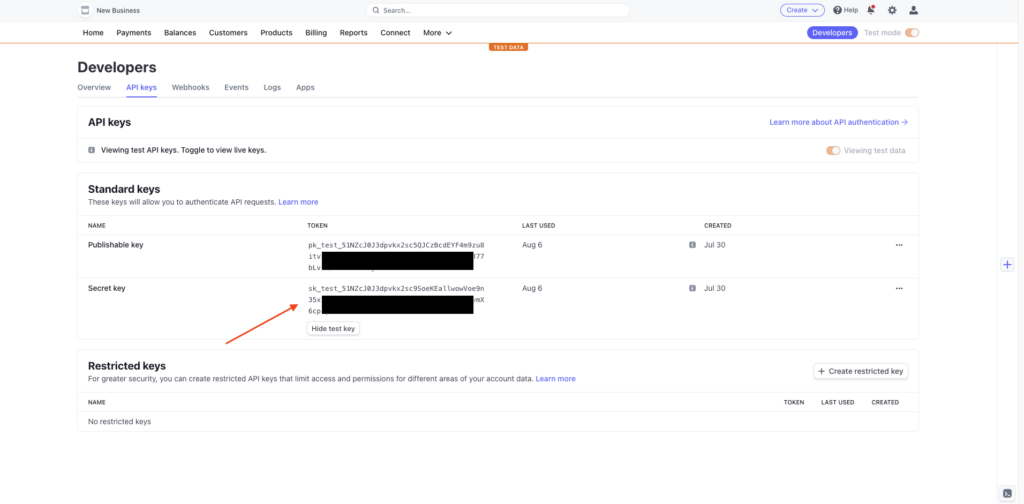
While you haven’t already, create a brand new Stripe account to get get right to use to the API keys.
After getting organize the API key, proceed to build the endpoint. This endpoint will gather the patron wisdom (name and electronic mail), create a purchaser profile for them in Stripe if one doesn’t exist already, and create a Checkout Consultation to allow consumers to pay for the cart items.
Proper right here’s what the code for the hostedCheckout
method seems like:
@PostMapping("/checkout/hosted")
String hostedCheckout(@RequestBody RequestDTO requestDTO) throws StripeException {
Stripe.apiKey = STRIPE_API_KEY;
String clientBaseURL = Instrument.getenv().get("CLIENT_BASE_URL");
// Get began by way of finding an provide purchaser document from Stripe or creating a brand spanking new one if sought after
Purchaser purchaser = CustomerUtil.findOrCreateCustomer(requestDTO.getCustomerEmail(), requestDTO.getCustomerName());
// Next, create a checkout session by way of together with the details of the checkout
SessionCreateParams.Builder paramsBuilder =
SessionCreateParams.builder()
.setMode(SessionCreateParams.Mode.PAYMENT)
.setCustomer(purchaser.getId())
.setSuccessUrl(clientBaseURL + "/just right fortune?session_id={CHECKOUT_SESSION_ID}")
.setCancelUrl(clientBaseURL + "/failure");
for (Product product : requestDTO.getItems()) {
paramsBuilder.addLineItem(
SessionCreateParams.LineItem.builder()
.setQuantity(1L)
.setPriceData(
PriceData.builder()
.setProductData(
PriceData.ProductData.builder()
.putMetadata("app_id", product.getId())
.setName(product.getName())
.assemble()
)
.setCurrency(ProductDAO.getProduct(product.getId()).getDefaultPriceObject().getCurrency())
.setUnitAmountDecimal(ProductDAO.getProduct(product.getId()).getDefaultPriceObject().getUnitAmountDecimal())
.assemble())
.assemble());
}
}
Session session = Session.create(paramsBuilder.assemble());
return session.getUrl();
}
When building the checkout session, the code uses the name of the product gained from the patron on the other hand does not use the associated fee details from the request. This fashion avoids potential client-side price manipulation, where malicious actors might send reduced prices inside the checkout request to pay a lot much less for services and products.
To forestall this, the hostedCheckout
method queries your products database (by way of ProductDAO
) to retrieve the right kind products price.
Additionally, Stripe provides various Builder
classes following the builder design building. The ones classes give a boost to in creating parameter devices for Stripe requests. The equipped code snippet moreover references atmosphere variables to fetch the patron app’s URL. This URL is very important for the checkout session object to redirect appropriately after a good fortune or failed finances.
To execute this code, set the patron app’s URL by way of atmosphere variables, similar to how the Stripe API key used to be as soon as equipped. As the patron app runs by the use of Vite, the local app URL should be http://localhost:5173. Include this for your atmosphere variables by the use of your IDE, terminal, or gadget keep watch over panel.
CLIENT_BASE_URL=http://localhost:5173
Moreover, provide the app with a ProductDAO
to look up product prices from. Wisdom Get right of entry to Object (DAO) interacts with wisdom property (very similar to databases) to get right to use app-related wisdom. While setting up a products database may well be outdoor the scope of this educational, a simple implementation you’ll do may well be so that you can upload a brand spanking new report ProductDAO.java within the identical list since the PaymentController.java and paste the following code:
package deal deal com.kinsta.stripejava.backend;
import com.stripe.kind.Price;
import com.stripe.kind.Product;
import java.math.BigDecimal;
public class ProductDAO {
static Product[] products;
static {
products = new Product[4];
Product sampleProduct = new Product();
Price samplePrice = new Price();
sampleProduct.setName("Puma Shoes");
sampleProduct.setId("shoe");
samplePrice.setCurrency("usd");
samplePrice.setUnitAmountDecimal(BigDecimal.valueOf(2000));
sampleProduct.setDefaultPriceObject(samplePrice);
products[0] = sampleProduct;
sampleProduct = new Product();
samplePrice = new Price();
sampleProduct.setName("Nike Sliders");
sampleProduct.setId("slippers");
samplePrice.setCurrency("usd");
samplePrice.setUnitAmountDecimal(BigDecimal.valueOf(1000));
sampleProduct.setDefaultPriceObject(samplePrice);
products[1] = sampleProduct;
sampleProduct = new Product();
samplePrice = new Price();
sampleProduct.setName("Apple Monitor+");
sampleProduct.setId("music");
samplePrice.setCurrency("usd");
samplePrice.setUnitAmountDecimal(BigDecimal.valueOf(499));
sampleProduct.setDefaultPriceObject(samplePrice);
products[2] = sampleProduct;
}
public static Product getProduct(String id) {
if ("shoe".equals(id)) {
return products[0];
} else if ("slippers".equals(id)) {
return products[1];
} else if ("music".equals(id)) {
return products[2];
} else return new Product();
}
}
This may occasionally initialize an array of products and imply you’ll be able to query product wisdom using its identifier (ID). You’re going to moreover want to create a DTO (Wisdom Transfer Object) to allow Spring Boot to routinely serialize the incoming payload from the patron and come up with a simple object to get right to use the guidelines. To take a look at this, create a brand spanking new report RequestDTO.java and paste the following code:
package deal deal com.kinsta.stripejava.backend;
import com.stripe.kind.Product;
public class RequestDTO {
Product[] items;
String customerName;
String customerEmail;
public Product[] getItems() {
return items;
}
public String getCustomerName() {
return customerName;
}
public String getCustomerEmail() {
return customerEmail;
}
}
This report defines a POJO that carries the patron name, electronic mail, and the list of items they’re trying out with.
Finally, put in force the CustomerUtil.findOrCreateCustomer()
technique to create the Purchaser object in Stripe if it does not exist already. To take a look at this, create a report with the name CustomerUtil
and add the following code to it:
package deal deal com.kinsta.stripejava.backend;
import com.stripe.exception.StripeException;
import com.stripe.kind.Purchaser;
import com.stripe.kind.CustomerSearchResult;
import com.stripe.param.CustomerCreateParams;
import com.stripe.param.CustomerSearchParams;
public class CustomerUtil {
public static Purchaser findCustomerByEmail(String electronic mail) throws StripeException {
CustomerSearchParams params =
CustomerSearchParams
.builder()
.setQuery("electronic mail:'" + electronic mail + "'")
.assemble();
CustomerSearchResult end result = Purchaser.search(params);
return end result.getData().measurement() > 0 ? end result.getData().get(0) : null;
}
public static Purchaser findOrCreateCustomer(String electronic mail, String name) throws StripeException {
CustomerSearchParams params =
CustomerSearchParams
.builder()
.setQuery("electronic mail:'" + electronic mail + "'")
.assemble();
CustomerSearchResult end result = Purchaser.search(params);
Purchaser purchaser;
// If no provide purchaser used to be as soon as found out, create a brand spanking new document
if (end result.getData().measurement() == 0) {
CustomerCreateParams customerCreateParams = CustomerCreateParams.builder()
.setName(name)
.setEmail(electronic mail)
.assemble();
purchaser = Purchaser.create(customerCreateParams);
} else {
purchaser = end result.getData().get(0);
}
return purchaser;
}
}
This class moreover accommodates each and every different method findCustomerByEmail
that allows you to look up customers in Stripe using their electronic mail addresses. The Buyer Seek API is used to look up the patron knowledge inside the Stripe database and Buyer Create API is used to create the patron knowledge as sought after.
This completes the backend setup sought after for the hosted checkout float. You’ll now take a look at the app by way of running the frontend and the backend apps in their IDEs or separate terminals. Proper right here’s what the great fortune float would look like:
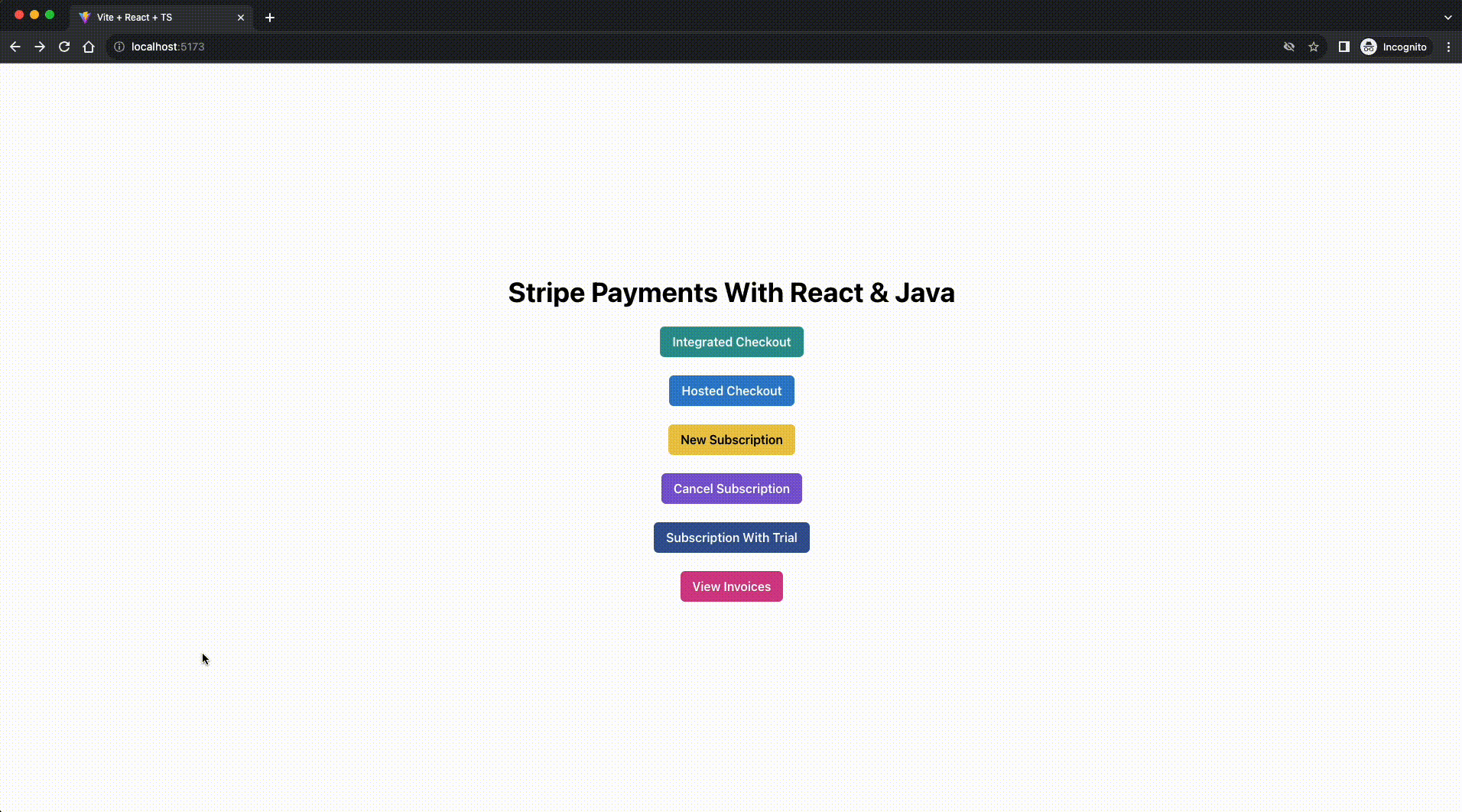
When trying out Stripe integrations, you’ll always use the following card details to simulate card transactions:
Card Amount: 4111 1111 1111 1111
Expiry Month & Year: 12 / 25
CVV: Any three-digit amount
Determine on Card: Any Determine
If you choose to cancel the transaction as a substitute of paying, proper right here’s what the failure float would look like:
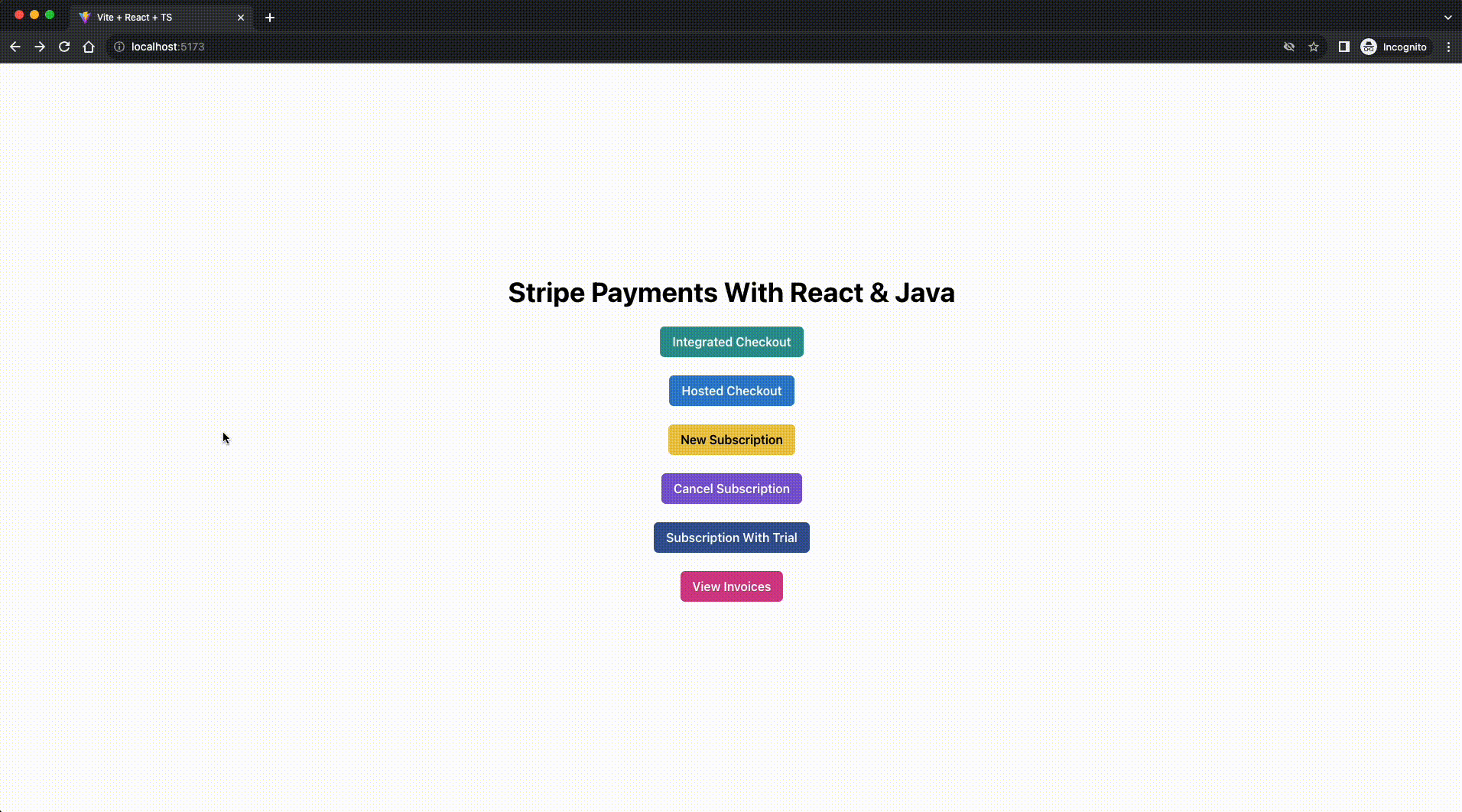
This completes the setup of a Stripe-hosted checkout experience built into your app. You’ll look all through the Stripe medical doctors to be told further about learn the way to customize your checkout internet web page, gather further details from the patron, and further.
Integrated Checkout
An incorporated checkout experience refers to building a worth float that doesn’t redirect your consumers outdoor your tool (find it irresistible did inside the hosted checkout float) and renders the fee form for your app itself.
Building an incorporated checkout experience manner coping with customers’ worth details, which comes to refined wisdom very similar to credit card numbers, Google Pay ID, and so on. Not all apps are designed to care for this knowledge securely.
To remove the burden of meeting necessities like PCI-DSS, Stripe provides parts that you just’ll use in-app to collect worth details while however letting Stripe organize the protection and process the finances securely on their end.
Building the Frontend
To start out, arrange the Stripe React SDK for your frontend app to get right to use the Stripe Portions by way of running the following command for your frontend list:
npm i @stripe/react-stripe-js @stripe/stripe-js
Next, create a brand spanking new report known as IntegratedCheckout.tsx for your frontend/src/routes list and save the following code in it:
import {Button, Middle, Heading, Input, VStack} from "@chakra-ui/react";
import {useEffect, useState} from "react";
import CartItem, {ItemData} from "../portions/CartItem.tsx";
import TotalFooter from "../portions/TotalFooter.tsx";
import {Products} from '../wisdom.ts'
import {Portions, PaymentElement, useElements, useStripe} from '@stripe/react-stripe-js';
import {loadStripe, Stripe} from '@stripe/stripe-js';
function IntegratedCheckout() {
const [items] = useState(Products)
const [transactionClientSecret, setTransactionClientSecret] = useState("")
const [stripePromise, setStripePromise] = useState<Promise | null>(null)
const [name, setName] = useState("")
const [email, setEmail] = useState("")
const onCustomerNameChange = (ev: React.ChangeEvent) => {
setName(ev.objective.price)
}
const onCustomerEmailChange = (ev: React.ChangeEvent) => {
setEmail(ev.objective.price)
}
useEffect(() => , [])
const createTransactionSecret = () => {
fetch(process.env.VITE_SERVER_BASE_URL + "/checkout/incorporated", {
method: "POST",
headers: {'Content material material-Kind': 'tool/json'},
body: JSON.stringify({
items: items.map(elem => ({name: elem.name, id: elem.id})),
customerName: name,
customerEmail: electronic mail,
})
})
.then(r => r.text())
.then(r => {
setTransactionClientSecret(r)
})
}
return
Integrated Checkout Example
{items.map(elem => {
return
})}
{(transactionClientSecret === "" ?
:
)}
}
const CheckoutForm = () => {
const stripe = useStripe();
const portions = useElements();
const handleSubmit = async (match: React.MouseEvent) => {
match.preventDefault();
if (!stripe || !portions) {
return;
}
const end result = look forward to stripe.confirmPayment({
portions,
confirmParams: {
return_url: process.env.VITE_CLIENT_BASE_URL + "/just right fortune",
},
});
if (end result.error) {
console.log(end result.error.message);
}
};
return
}
export default IntegratedCheckout
This report defines two portions, IntegratedCheckout
and CheckoutForm
. The CheckoutForm
defines a simple form with a PaymentElement
from Stripe which collects customers’ worth details and a Pay button that triggers a worth collection request.
This part moreover calls the useStripe()
and useElements()
hook to create an instance of the Stripe SDK that you just’ll use to create worth requests. When you click on at the Pay button, the stripe.confirmPayment()
method from the Stripe SDK is referred to as that collects the patron’s worth wisdom from the elements instance and sends it to Stripe backend with a just right fortune URL to redirect to if the transaction is a good fortune.
The checkout form has been separated from the rest of the internet web page given that useStripe()
and useElements()
hooks want to be known as from the context of an Portions
provider, which has been completed inside the IntegratedCheckout
‘s return commentary. While you moved the Stripe hook calls to the IntegratedCheckout
part immediately, they may well be outdoor the scope of the Portions
provider and subsequently would not artwork.
The IntegratedCheckout
part reuses the CartItem
and TotalFooter
portions to render the cart items and the entire amount. It moreover renders two input fields to collect the patron’s wisdom and an Get started up worth button that sends a request to the Java backend server to create the patron secret key using the patron and cart details. Once the patron secret secret’s gained, the CheckoutForm
is rendered, which handles the choice of the fee details from the patron.
With the exception of that, useEffect
is used to call the loadStripe
method. This affect is run most efficient once when the part renders so that the Stripe SDK isn’t loaded multiple circumstances when the part’s inside of states are up-to-the-minute.
To run the code above, you’re going to moreover want to add two new atmosphere variables to your frontend project: VITE_STRIPE_API_KEY
and VITE_CLIENT_BASE_URL
. The Stripe API key variable will grab the publishable API key from the Stripe dashboard, and the patron base URL variable will come with the link to the patron app (which is the frontend app itself) so that it can be passed to the Stripe SDK for coping with just right fortune and failure redirects.
To take a look at this, add the following code to your .env report inside the frontend list:
VITE_STRIPE_API_KEY=pk_test_xxxxxxxxxx # Your key proper right here
VITE_CLIENT_BASE_URL=http://localhost:5173
Finally, substitute the App.tsx report to include the IntegratedCheckout
part at the /integrated-checkout
path of the frontend app. Add the following code inside the array passed to the createBrowserRouter
identify inside the App
part:
{
path: '/integrated-checkout',
part: (
)
},
This completes the setup sought after on the frontend. Next, create a brand spanking new path for your backend server that creates the patron secret key needed to care for incorporated checkout classes for your frontend app.
Building the Backend
To be sure that the frontend integration isn’t abused by way of attackers (since frontend code is more uncomplicated to crack than backend), Stripe calls so that you can generate a unique client secret for your backend server and verifies every incorporated worth request with the patron secret generated on the backend to be sure that it’s indubitably your app that’s searching for to amass finances. To take a look at this, you want to prepare each and every different path inside the backend that creates client secrets and techniques and strategies in keeping with purchaser and cart wisdom.
For creating the patron secret key for your server, create a brand spanking new method for your PaymentController
class with the name integratedCheckout
and save the following code in it:
@PostMapping("/checkout/incorporated")
String integratedCheckout(@RequestBody RequestDTO requestDTO) throws StripeException {
Stripe.apiKey = STRIPE_API_KEY;
// Get began by way of finding provide purchaser or creating a brand spanking new one if sought after
Purchaser purchaser = CustomerUtil.findOrCreateCustomer(requestDTO.getCustomerEmail(), requestDTO.getCustomerName());
// Create a PaymentIntent and send it's client secret to the patron
PaymentIntentCreateParams params =
PaymentIntentCreateParams.builder()
.setAmount(Long.parseLong(calculateOrderAmount(requestDTO.getItems())))
.setCurrency("usd")
.setCustomer(purchaser.getId())
.setAutomaticPaymentMethods(
PaymentIntentCreateParams.AutomaticPaymentMethods
.builder()
.setEnabled(true)
.assemble()
)
.assemble();
PaymentIntent paymentIntent = PaymentIntent.create(params);
// Send the patron secret from the fee intent to the patron
return paymentIntent.getClientSecret();
}
Similar to how the checkout session used to be as soon as built using a builder class that accepts the configuration for the fee request, the incorporated checkout float calls so that you can assemble a worth session with the amount, foreign exchange, and worth methods. Against this to the checkout session, you’ll not associate line pieces with a worth session till you create an invoice, which you’re going to learn in a later segment of the educational.
Since you don’t appear to be passing inside the line items to the checkout session builder, you want to manually calculate the entire amount for the cart items and send the amount to the Stripe backend. Use your ProductDAO
to look out and add the prices for every product inside the cart.
To take a look at this, define a brand spanking new method calculateOrderAmount
and add the following code in it:
static String calculateOrderAmount(Product[] items) {
long basic = 0L;
for (Product products: items) {
// Look up the application database to look out the prices for the products inside the given list
basic += ProductDAO.getProduct(products.getId()).getDefaultPriceObject().getUnitAmountDecimal().floatValue();
}
return String.valueOf(basic);
}
That should be sufficient to prepare the incorporated checkout float on every the frontend and the backend. You’ll restart the advance servers for the server and client and check out out the new incorporated checkout float inside the frontend app. Proper right here’s what the incorporated float will look like:
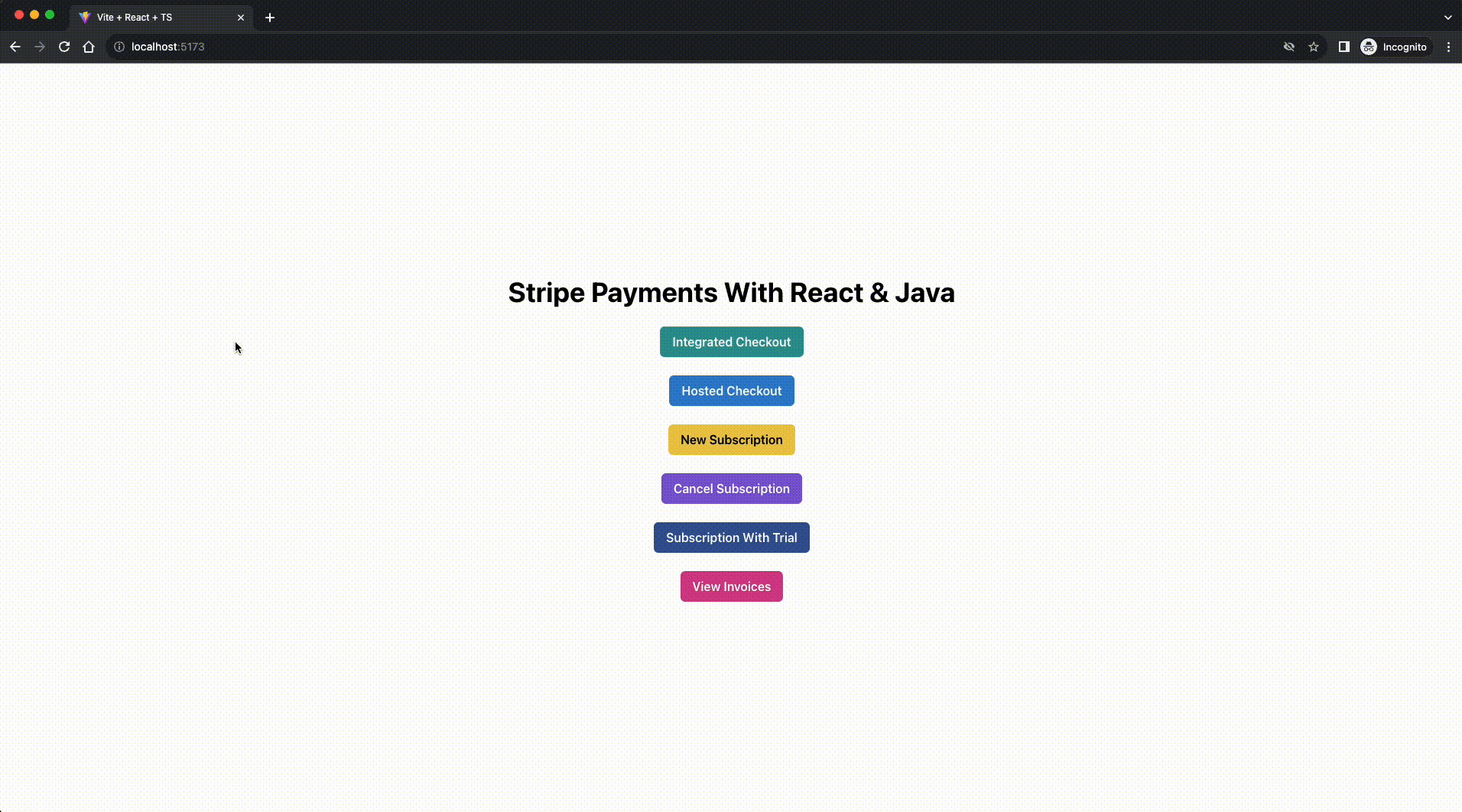
This completes a basic incorporated checkout float for your app. You’ll now further uncover the Stripe documentation to customise the price strategies or mix further portions that can assist you with other operations very similar to cope with assortment, price requests, hyperlink integration, and further!
Setting Up Subscriptions for Unusual Services and products and merchandise
A now not strange offering from online shops at the present time is a subscription. Whether or not or now not you may well be building a marketplace for services and products or offering a digital product periodically, a subscription is the very best answer for giving your customers periodic get right to use to your service for a small price compared to a one-time achieve.
Stripe will let you organize and cancel subscriptions merely. You’ll moreover offer unfastened trials as part of your subscription so that consumers would possibly check out your offering previous than committing to it.
Setting Up a New Subscription
Putting in place a brand spanking new subscription is understated using the hosted checkout float. You’re going to most efficient want to change a few parameters when building the checkout request and create a brand spanking new internet web page (by way of reusing the present portions) to show a checkout internet web page for a brand spanking new subscription. To start out, create a NewSubscription.tsx report inside the frontend portions folder. Paste the following code in it:
import {Middle, Heading, VStack} from "@chakra-ui/react";
import {useState} from "react";
import CartItem, {ItemData} from "../portions/CartItem.tsx";
import TotalFooter from "../portions/TotalFooter.tsx";
import CustomerDetails from "../portions/CustomerDetails.tsx";
import {Subscriptions} from "../wisdom.ts";
function NewSubscription() {
const [items] = useState(Subscriptions)
return
New Subscription Example
{items.map(elem => {
return
})}
}
export default NewSubscription
Inside the code above, the cart wisdom is taken from the wisdom.ts report, and it most efficient accommodates one products to simplify the process. In real-world scenarios, you’ll have multiple items as part of one subscription order.
To render this part at the right kind path, add the following code inside the array passed to the createBrowserRouter
identify inside the App.tsx part:
{
path: '/new-subscription',
part: (
)
},
This completes the setup sought after on the frontend. On the backend, create a brand spanking new path /subscription/new
to create a brand spanking new hosted checkout session for a subscription product. Create a newSubscription
method inside the backend/src/primary/java/com/kinsta/stripejava/backend list and save the following code in it:
@PostMapping("/subscriptions/new")
String newSubscription(@RequestBody RequestDTO requestDTO) throws StripeException {
Stripe.apiKey = STRIPE_API_KEY;
String clientBaseURL = Instrument.getenv().get("CLIENT_BASE_URL");
// Get began by way of finding provide purchaser document from Stripe or creating a brand spanking new one if sought after
Purchaser purchaser = CustomerUtil.findOrCreateCustomer(requestDTO.getCustomerEmail(), requestDTO.getCustomerName());
// Next, create a checkout session by way of together with the details of the checkout
SessionCreateParams.Builder paramsBuilder =
SessionCreateParams.builder()
// For subscriptions, you want to set the mode as subscription
.setMode(SessionCreateParams.Mode.SUBSCRIPTION)
.setCustomer(purchaser.getId())
.setSuccessUrl(clientBaseURL + "/just right fortune?session_id={CHECKOUT_SESSION_ID}")
.setCancelUrl(clientBaseURL + "/failure");
for (Product product : requestDTO.getItems()) {
paramsBuilder.addLineItem(
SessionCreateParams.LineItem.builder()
.setQuantity(1L)
.setPriceData(
PriceData.builder()
.setProductData(
PriceData.ProductData.builder()
.putMetadata("app_id", product.getId())
.setName(product.getName())
.assemble()
)
.setCurrency(ProductDAO.getProduct(product.getId()).getDefaultPriceObject().getCurrency())
.setUnitAmountDecimal(ProductDAO.getProduct(product.getId()).getDefaultPriceObject().getUnitAmountDecimal())
// For subscriptions, you want to provide the details on how regularly they may recur
.setRecurring(PriceData.Unusual.builder().setInterval(PriceData.Unusual.Duration.MONTH).assemble())
.assemble())
.assemble());
}
Session session = Session.create(paramsBuilder.assemble());
return session.getUrl();
}
The code in this method is fairly similar to the code inside the hostedCheckout
method, except that the mode set for creating the session is subscription as a substitute of product and previous than creating the session, a value is set for the recurrence duration for the subscription.
This instructs Stripe to treat this checkout as a subscription checkout as a substitute of a one-time worth. Similar to the hostedCheckout
method, the program moreover returns the URL of the hosted checkout internet web page since the HTTP response to the patron. The consumer is set to redirect to the URL gained, allowing the patron to complete the fee.
You’ll restart the advance servers for every the patron and the server and see the new subscription internet web page in movement. Proper right here’s what it seems like:
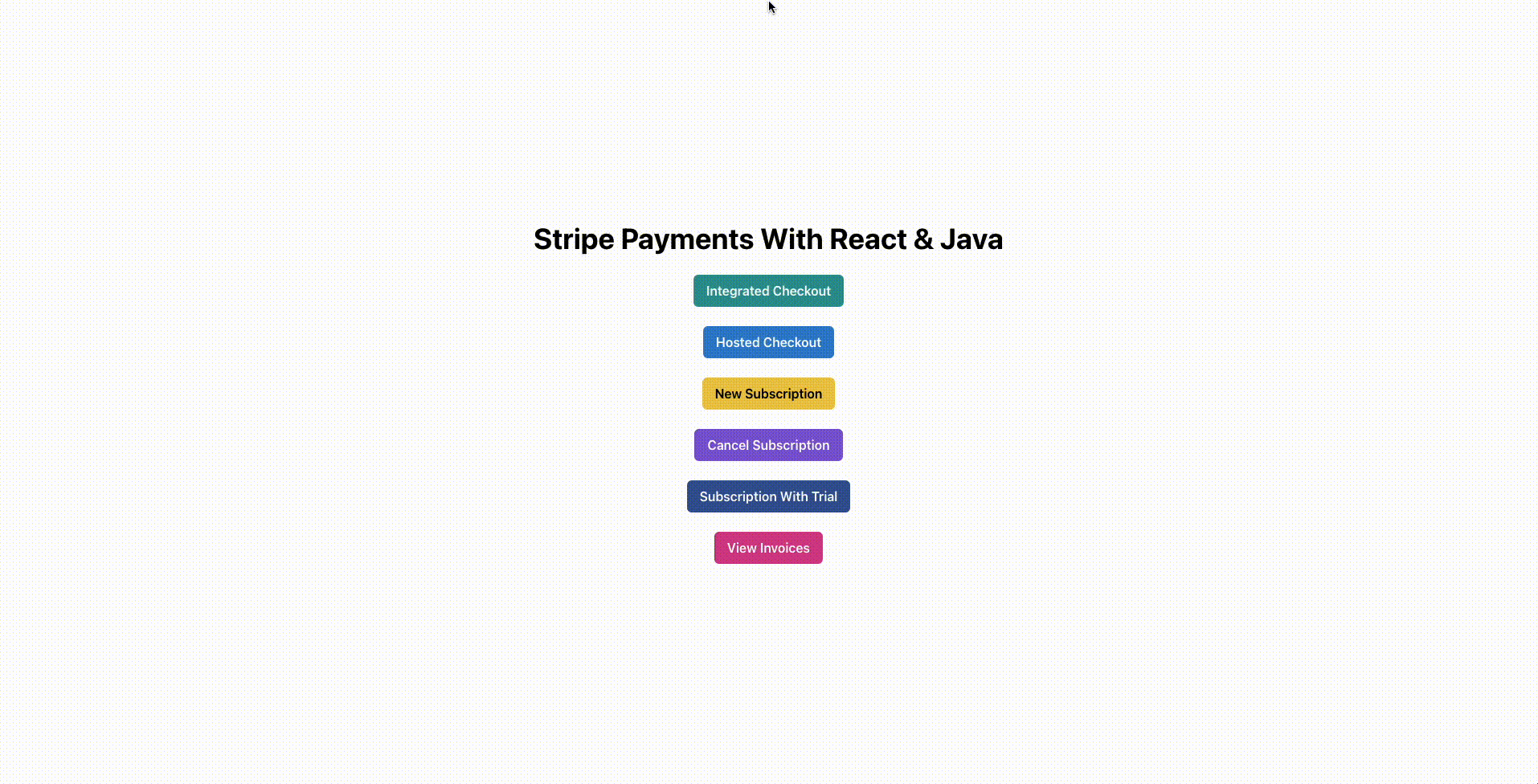
Canceling an Present Subscription
Now that you know the way to create new subscriptions, let’s learn how to permit your customers to cancel provide subscriptions. Given that demo app built in this educational does not come with any authentication setup, use a type to allow the patron to enter their electronic mail to look up their subscriptions and then provide every subscription products with a cancel button to allow the patron to cancel it.
To take a look at this, it is very important do the following:
- Exchange the
CartItem
part to show a cancel button on the cancel subscriptions internet web page. - Create a
CancelSubscription
part that first shows an input field and a button for the patron to search around subscriptions using their electronic mail deal with and then renders a listing of subscriptions using the up-to-the-minuteCartItem
part. - Create a brand spanking new method inside the backend server that can look up subscriptions from the Stripe backend using the patron’s electronic mail deal with.
- Create a brand spanking new method inside the backend server that can cancel a subscription in keeping with the subscription ID passed to it.
Get began by way of updating the CartItem
part to make it look like this:
// Present imports proper right here
function CartItem(props: CartItemProps) {
// Add this hook identify and the cancelSubscription technique to cancel the selected subscription
const toast = useToast()
const cancelSubscription = () => {
fetch(process.env.VITE_SERVER_BASE_URL + "/subscriptions/cancel", {
method: "POST",
headers: {'Content material material-Kind': 'tool/json'},
body: JSON.stringify({
subscriptionId: props.wisdom.stripeSubscriptionData?.subscriptionId
})
})
.then(r => r.text())
.then(() => {
toast({
identify: 'Subscription cancelled.',
description: "We now have were given cancelled your subscription for you.",
status: 'just right fortune',
period: 9000,
isClosable: true,
})
if (props.onCancelled)
props.onCancelled()
})
}
return
{props.wisdom.name}
{props.wisdom.description}
{(props.mode === "checkout" ?
{"Quantity: " + props.wisdom.quantity}
: )}
{/* */}
{(props.mode === "subscription" && props.wisdom.stripeSubscriptionData ?
{"Next Rate Date: " + props.wisdom.stripeSubscriptionData.nextPaymentDate}
{"Subscribed On: " + props.wisdom.stripeSubscriptionData.subscribedOn}
{(props.wisdom.stripeSubscriptionData.trialEndsOn ?
{"Unfastened Trial Operating Until: " + props.wisdom.stripeSubscriptionData.trialEndsOn}
: )}
: )}
{"$" + props.wisdom.price}
{/* */}
{(props.wisdom.stripeSubscriptionData ?
: )}
}
// Present varieties proper right here
export default CartItem
Next, create a CancelSubscription.tsx part for your fronted’s routes list and save the following code in it:
import {Button, Middle, Heading, Input, VStack} from "@chakra-ui/react";
import {useState} from "react";
import CartItem, {ItemData, ServerSubscriptionsResponseType} from "../portions/CartItem.tsx";
import {Subscriptions} from "../wisdom.ts";
function CancelSubscription() {
const [email, setEmail] = useState("")
const [subscriptions, setSubscriptions] = useState([])
const onCustomerEmailChange = (ev: React.ChangeEvent) => {
setEmail(ev.objective.price)
}
const listSubscriptions = () => {
fetch(process.env.VITE_SERVER_BASE_URL + "/subscriptions/list", {
method: "POST",
headers: {'Content material material-Kind': 'tool/json'},
body: JSON.stringify({
customerEmail: electronic mail,
})
})
.then(r => r.json())
.then((r: ServerSubscriptionsResponseType[]) => {
const subscriptionsList: ItemData[] = []
r.forEach(subscriptionItem => {
let subscriptionDetails = Subscriptions.to seek out(elem => elem.id === subscriptionItem.appProductId) || undefined
if (subscriptionDetails) {
subscriptionDetails = {
...subscriptionDetails,
price: Amount.parseInt(subscriptionItem.price) / 100,
stripeSubscriptionData: subscriptionItem,
}
subscriptionsList.push(subscriptionDetails)
} else {
console.log("Products not found out!")
}
})
setSubscriptions(subscriptionsList)
})
}
const removeSubscription = (id: string | undefined) => {
const newSubscriptionsList = subscriptions.filter(elem => (elem.stripeSubscriptionData?.subscriptionId !== id))
setSubscriptions(newSubscriptionsList)
}
return
Cancel Subscription Example
{(subscriptions.duration === 0 ?
: )}
{subscriptions.map(elem => {
return removeSubscription(elem.stripeSubscriptionData?.subscriptionId)}/>
})}
}
export default CancelSubscription
This part renders an input field and a button for customers to enter their electronic mail and get began looking for subscriptions. If subscriptions are found out, the input field and button are hidden, and a listing of subscriptions is displayed on the computer screen. For every subscription products, the part passes a removeSubscription
method that requests the Java backend server to cancel the subscription on the Stripe backend.
To connect it to the /cancel-subscription
path for your frontend app, add the following code inside the array passed to the createBrowserRouter
identify inside the App
part:
{
path: '/cancel-subscription',
part: (
)
},
To search for subscriptions on the backend server, add a viewSubscriptions
method inside the PaymentController
class of your backend project with the following contents:
@PostMapping("/subscriptions/list")
Checklist<Map> viewSubscriptions(@RequestBody RequestDTO requestDTO) throws StripeException {
Stripe.apiKey = STRIPE_API_KEY;
// Get began by way of finding provide purchaser document from Stripe
Purchaser purchaser = CustomerUtil.findCustomerByEmail(requestDTO.getCustomerEmail());
// If no purchaser document used to be as soon as found out, no subscriptions exist each, so return an empty list
if (purchaser == null) {
return new ArrayList();
}
// Search for subscriptions for the prevailing purchaser
SubscriptionCollection subscriptions = Subscription.list(
SubscriptionListParams.builder()
.setCustomer(purchaser.getId())
.assemble());
Checklist<Map> response = new ArrayList();
// For every subscription document, query its products knowledge and gather in a listing of devices to send to the patron
for (Subscription subscription : subscriptions.getData()) {
SubscriptionItemCollection currSubscriptionItems =
SubscriptionItem.list(SubscriptionItemListParams.builder()
.setSubscription(subscription.getId())
.addExpand("wisdom.price.product")
.assemble());
for (SubscriptionItem products : currSubscriptionItems.getData()) {
HashMap subscriptionData = new HashMap();
subscriptionData.put("appProductId", products.getPrice().getProductObject().getMetadata().get("app_id"));
subscriptionData.put("subscriptionId", subscription.getId());
subscriptionData.put("subscribedOn", new SimpleDateFormat("dd/MM/yyyy").construction(new Date(subscription.getStartDate() * 1000)));
subscriptionData.put("nextPaymentDate", new SimpleDateFormat("dd/MM/yyyy").construction(new Date(subscription.getCurrentPeriodEnd() * 1000)));
subscriptionData.put("price", products.getPrice().getUnitAmountDecimal().toString());
if (subscription.getTrialEnd() != null && new Date(subscription.getTrialEnd() * 1000).after(new Date()))
subscriptionData.put("trialEndsOn", new SimpleDateFormat("dd/MM/yyyy").construction(new Date(subscription.getTrialEnd() * 1000)));
response.add(subscriptionData);
}
}
return response;
}
The method above first finds the patron object for the given client in Stripe. Then, it searches for lively subscriptions of the patron. As quickly because the list of subscriptions is gained, it extracts the items from them and finds the corresponding products inside the app product database to send to the frontend. This is essential given that ID with which the frontend identifies every product inside the app database would possibly or will not be the identical since the product ID stored in Stripe.
Finally, create a cancelSubscription</code method inside the
PaymentController
class and paste the code beneath to delete a subscription in keeping with the subscription ID passed.
@PostMapping("/subscriptions/cancel")
String cancelSubscription(@RequestBody RequestDTO requestDTO) throws StripeException {
Stripe.apiKey = STRIPE_API_KEY;
Subscription subscription =
Subscription.retrieve(
requestDTO.getSubscriptionId()
);
Subscription deletedSubscription =
subscription.cancel();
return deletedSubscription.getStatus();
}
The program retrieves the subscription object from Stripe, calls the cancel method on it, and then returns the subscription status to the patron. However, in an effort to run this, you want to interchange your DTO object so that you can upload the subscriptionId
field. Do that by way of together with the following field and method inside the RequestDTO
class:
package deal deal com.kinsta.stripejava.backend;
import com.stripe.kind.Product;
public class RequestDTO {
// … other fields …
// Add this
String subscriptionId;
// … other getters …
// Add this
public String getSubscriptionId() {
return subscriptionId;
}
}
When you add this, you’ll now re-run the advance server for every the backend and the frontend app and see the cancel float in movement:
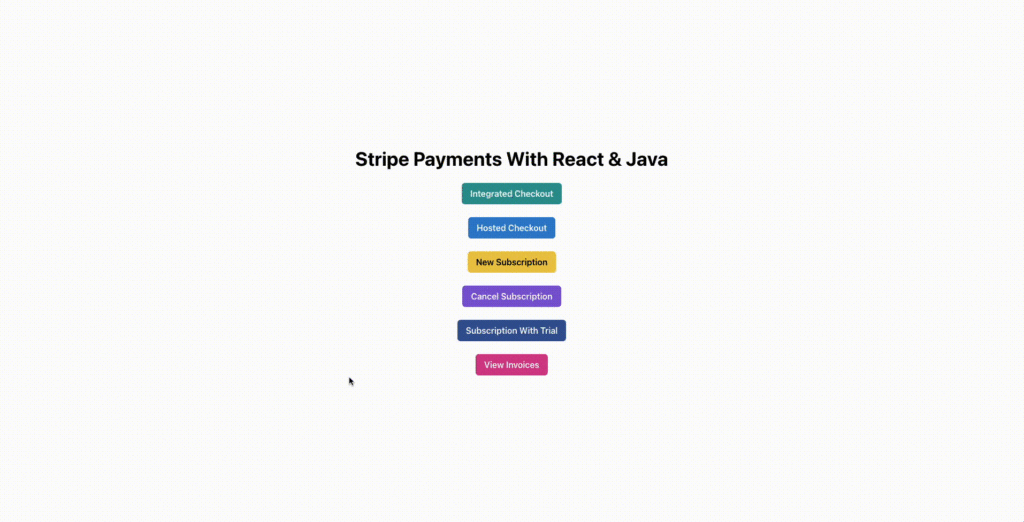
Setting Up Unfastened Trials for Subscriptions With 0-Worth Transactions
A now not strange serve as with most modern subscriptions is to offer a temporary unfastened trial period previous than charging the patron. This allows consumers to find the product or service without investing in it. However, it’s absolute best to store the patron’s worth details while signing them up for the unfastened trial to be able to merely price them as briefly since the trial ends.
Stripe a perfect deal simplifies the appearance of such subscriptions. To begin out, generate a brand spanking new part all through the frontend/routes list named SubscriptionWithTrial.tsx, and paste the following code:
import {Middle, Heading, VStack} from "@chakra-ui/react";
import {useState} from "react";
import CartItem, {ItemData} from "../portions/CartItem.tsx";
import TotalFooter from "../portions/TotalFooter.tsx";
import CustomerDetails from "../portions/CustomerDetails.tsx";
import {Subscriptions} from "../wisdom.ts";
function SubscriptionWithTrial() {
const [items] = useState(Subscriptions)
return
New Subscription With Trial Example
{items.map(elem => {
return
})}
}
export default SubscriptionWithTrial
This part reuses the portions created earlier. The essential factor difference between this and the NewSubscription
part is that it passes the mode for TotalFooter
as trial as a substitute of subscription. This makes the TotalFooter
part render a text announcing that the patron can get began the unfastened trial now on the other hand it will be charged after a month.
To connect this part to the /subscription-with-trial
path for your frontend app, add the following code inside the array passed to the createBrowserRouter
identify inside the App
part:
{
path: '/subscription-with-trial',
part: (
)
},
To build the checkout float for subscriptions with trial on the backend, create a brand spanking new method named newSubscriptionWithTrial
inside the PaymentController
class and add the following code:
@PostMapping("/subscriptions/trial")
String newSubscriptionWithTrial(@RequestBody RequestDTO requestDTO) throws StripeException {
Stripe.apiKey = STRIPE_API_KEY;
String clientBaseURL = Instrument.getenv().get("CLIENT_BASE_URL");
// Get began by way of finding provide purchaser document from Stripe or creating a brand spanking new one if sought after
Purchaser purchaser = CustomerUtil.findOrCreateCustomer(requestDTO.getCustomerEmail(), requestDTO.getCustomerName());
// Next, create a checkout session by way of together with the details of the checkout
SessionCreateParams.Builder paramsBuilder =
SessionCreateParams.builder()
.setMode(SessionCreateParams.Mode.SUBSCRIPTION)
.setCustomer(purchaser.getId())
.setSuccessUrl(clientBaseURL + "/just right fortune?session_id={CHECKOUT_SESSION_ID}")
.setCancelUrl(clientBaseURL + "/failure")
// For trials, you want to set the trial period inside the session introduction request
.setSubscriptionData(SessionCreateParams.SubscriptionData.builder().setTrialPeriodDays(30L).assemble());
for (Product product : requestDTO.getItems()) {
paramsBuilder.addLineItem(
SessionCreateParams.LineItem.builder()
.setQuantity(1L)
.setPriceData(
PriceData.builder()
.setProductData(
PriceData.ProductData.builder()
.putMetadata("app_id", product.getId())
.setName(product.getName())
.assemble()
)
.setCurrency(ProductDAO.getProduct(product.getId()).getDefaultPriceObject().getCurrency())
.setUnitAmountDecimal(ProductDAO.getProduct(product.getId()).getDefaultPriceObject().getUnitAmountDecimal())
.setRecurring(PriceData.Unusual.builder().setInterval(PriceData.Unusual.Duration.MONTH).assemble())
.assemble())
.assemble());
}
Session session = Session.create(paramsBuilder.assemble());
return session.getUrl();
}
This code is fairly similar to that of newSubscription
method. The only (and a very powerful) difference is {{that a}} trial period is passed to the session create parameters object with the cost of 30
, indicating a unfastened trial period of 30 days.
You’ll now save the changes and re-run the advance server for the backend and the frontend to seem the subscription with unfastened trial workflow in movement:
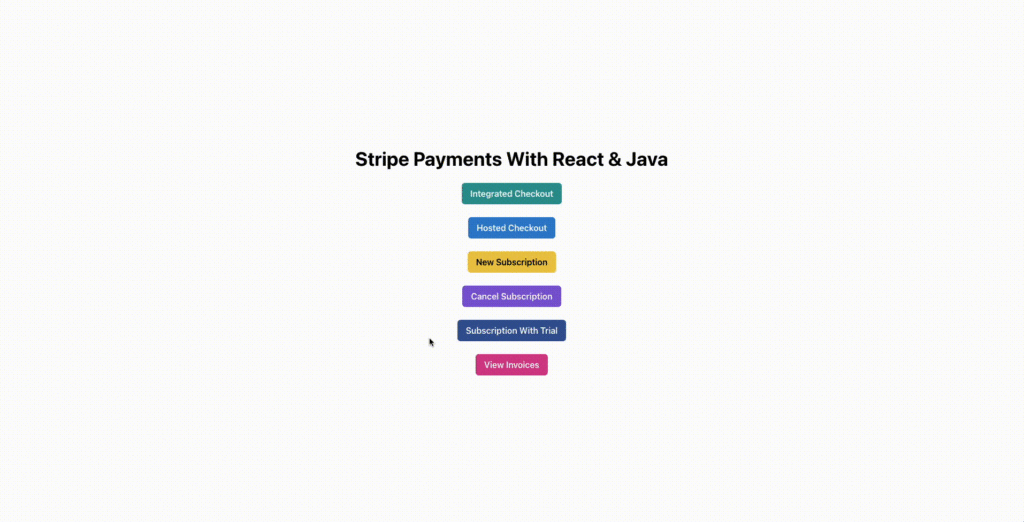
Generating Invoices for Expenses
For subscriptions, Stripe routinely generates invoices for every worth, despite the fact that this can be a 0 price transaction for trial signup. For one-off finances, you’ll select to create invoices if sought after.
To start out associating all finances with invoices, substitute the body of the payload being sent inside the initiatePayment
function of the CustomerDetails
part inside the frontend app to incorporate the following property:
invoiceNeeded: true
You’re going to moreover want to add this property inside the body of the payload being sent to the server inside the createTransactionSecret
function of the IntegratedCheckout
part.
Next, substitute the backend routes to check for this new property and substitute the Stripe SDK calls accordingly.
For the hosted checkout method, so that you can upload the invoicing capacity, substitute the hostedCheckout
method by way of together with the following lines of code:
@PostMapping("/checkout/hosted")
String hostedCheckout(@RequestBody RequestDTO requestDTO) throws StripeException {
// … other operations being completed after creating the SessionCreateParams builder instance
// Add the following block of code merely previous than the SessionCreateParams are constituted of the builder instance
if (requestDTO.isInvoiceNeeded()) {
paramsBuilder.setInvoiceCreation(SessionCreateParams.InvoiceCreation.builder().setEnabled(true).assemble());
}
Session session = Session.create(paramsBuilder.assemble());
return session.getUrl();
}
This may occasionally check out for the invoiceNeeded
field and set the create parameters accordingly.
Together with an invoice to an incorporated worth is moderately difficult. You’ll not simply set a parameter to instruct Stripe to create an invoice with the fee routinely. You will have to manually create the invoice and then a hooked up worth intent.
If the fee intent is successfully paid and completed, the invoice is marked as paid; otherwise, the invoice remains unpaid. While this makes logical sense, it can be rather sophisticated to put in force (specifically when there don’t seem to be any clear examples or references to use).
To put in force this, substitute the integratedCheckout
technique to make it look like this:
String integratedCheckout(@RequestBody RequestDTO requestDTO) throws StripeException {
Stripe.apiKey = STRIPE_API_KEY;
// Get began by way of finding an provide purchaser or creating a brand spanking new one if sought after
Purchaser purchaser = CustomerUtil.findOrCreateCustomer(requestDTO.getCustomerEmail(), requestDTO.getCustomerName());
PaymentIntent paymentIntent;
if (!requestDTO.isInvoiceNeeded()) {
// If the invoice is not sought after, create a PaymentIntent immediately and send it to the patron
PaymentIntentCreateParams params =
PaymentIntentCreateParams.builder()
.setAmount(Long.parseLong(calculateOrderAmount(requestDTO.getItems())))
.setCurrency("usd")
.setCustomer(purchaser.getId())
.setAutomaticPaymentMethods(
PaymentIntentCreateParams.AutomaticPaymentMethods
.builder()
.setEnabled(true)
.assemble()
)
.assemble();
paymentIntent = PaymentIntent.create(params);
} else {
// If invoice is sought after, create the invoice object, add line items to it, and finalize it to create the PaymentIntent routinely
InvoiceCreateParams invoiceCreateParams = new InvoiceCreateParams.Builder()
.setCustomer(purchaser.getId())
.assemble();
Invoice invoice = Invoice.create(invoiceCreateParams);
// Add every products to the invoice one by one
for (Product product : requestDTO.getItems()) {
// Seek for provide Product in Stripe previous than creating a brand spanking new one
Product stripeProduct;
ProductSearchResult results = Product.search(ProductSearchParams.builder()
.setQuery("metadata['app_id']:'" + product.getId() + "'")
.assemble());
if (results.getData().measurement() != 0)
stripeProduct = results.getData().get(0);
else {
// If a product is not found in Stripe database, create it
ProductCreateParams productCreateParams = new ProductCreateParams.Builder()
.setName(product.getName())
.putMetadata("app_id", product.getId())
.assemble();
stripeProduct = Product.create(productCreateParams);
}
// Create an invoice line products using the product object for the street products
InvoiceItemCreateParams invoiceItemCreateParams = new InvoiceItemCreateParams.Builder()
.setInvoice(invoice.getId())
.setQuantity(1L)
.setCustomer(purchaser.getId())
.setPriceData(
InvoiceItemCreateParams.PriceData.builder()
.setProduct(stripeProduct.getId())
.setCurrency(ProductDAO.getProduct(product.getId()).getDefaultPriceObject().getCurrency())
.setUnitAmountDecimal(ProductDAO.getProduct(product.getId()).getDefaultPriceObject().getUnitAmountDecimal())
.assemble())
.assemble();
InvoiceItem.create(invoiceItemCreateParams);
}
// Mark the invoice as final so that a PaymentIntent is created for it
invoice = invoice.finalizeInvoice();
// Retrieve the fee intent object from the invoice
paymentIntent = PaymentIntent.retrieve(invoice.getPaymentIntent());
}
// Send the patron secret from the fee intent to the patron
return paymentIntent.getClientSecret();
}
The out of date code of the program is moved into the if
block that checks if the invoiceNeeded
field is false
. If it is found out true, the method now creates an invoice with invoice items and marks it as finalized so that it can be paid.
Then, it retrieves the fee intent routinely created when the invoice used to be as soon as finalized and sends the patron secret from this worth intent to the patron. Once the patron completes the incorporated checkout float, the fee is accrued, and the invoice is marked as paid.
This completes the setup needed to get began generating invoices from your tool. You’ll head over to the invoices phase for your Stripe dashboard to take a look on the invoices your app generates with every achieve and subscription worth.
However, Stripe moreover signifies that you’ll be able to get right to use the invoices over its API to make a self-service experience for customers to procure invoices on each and every instance they would love.
To take a look at this, create a brand spanking new part inside the frontend/routes list named ViewInvoices.tsx. Paste the following code in it:
import {Button, Card, Middle, Heading, HStack, IconButton, Input, Text, VStack} from "@chakra-ui/react";
import {useState} from "react";
import {DownloadIcon} from "@chakra-ui/icons";
function ViewInvoices() {
const [email, setEmail] = useState("")
const [invoices, setInvoices] = useState([])
const onCustomerEmailChange = (ev: React.ChangeEvent) => {
setEmail(ev.objective.price)
}
const listInvoices = () => {
fetch(process.env.VITE_SERVER_BASE_URL + "/invoices/list", {
method: "POST",
headers: {'Content material material-Kind': 'tool/json'},
body: JSON.stringify({
customerEmail: electronic mail,
})
})
.then(r => r.json())
.then((r: InvoiceData[]) => {
setInvoices(r)
})
}
return
View Invoices for Purchaser
{(invoices.duration === 0 ?
: )}
{invoices.map(elem => {
return
{elem.amount}
{"$" + elem.amount}
{
window.location.href = elem.url
}} icon={} aria-label={'Download invoice'}/>
})}
}
interface InvoiceData {
amount: string,
amount: string,
url: string
}
export default ViewInvoices
Similar to the CancelSubscription
part, this part displays an input field for the patron to enter their electronic mail and a button to search for invoices. Once invoices are found out, the input field and button are hidden, and a listing of invoices with the invoice amount, basic amount, and a button to procure the invoice PDF is confirmed to the patron.
To put in force the backend method that searches for invoices of the given purchaser and sends once more the linked wisdom (invoice amount, amount, and PDF URL), add the following method for your PaymentController
class on the backend;
@PostMapping("/invoices/list")
Checklist<Map> listInvoices(@RequestBody RequestDTO requestDTO) throws StripeException {
Stripe.apiKey = STRIPE_API_KEY;
// Get began by way of finding provide purchaser document from Stripe
Purchaser purchaser = CustomerUtil.findCustomerByEmail(requestDTO.getCustomerEmail());
// If no purchaser document used to be as soon as found out, no subscriptions exist each, so return an empty list
if (purchaser == null) {
return new ArrayList();
}
// Search for invoices for the prevailing purchaser
Map invoiceSearchParams = new HashMap();
invoiceSearchParams.put("purchaser", purchaser.getId());
InvoiceCollection invoices =
Invoice.list(invoiceSearchParams);
Checklist<Map> response = new ArrayList();
// For every invoice, extract its amount, amount, and PDF URL to send to the patron
for (Invoice invoice : invoices.getData()) {
HashMap map = new HashMap();
map.put("amount", invoice.getNumber());
map.put("amount", String.valueOf((invoice.getTotal() / 100f)));
map.put("url", invoice.getInvoicePdf());
response.add(map);
}
return response;
}
The method first turns out for the patron by way of the email deal with equipped to it. Then, it kind of feels for invoices of this purchaser which may also be marked as paid. As quickly because the list of invoices is situated, it extracts the invoice amount, amount, and PDF URL and sends once more a listing of this information to the patron app.
This is how the invoices float seems like:
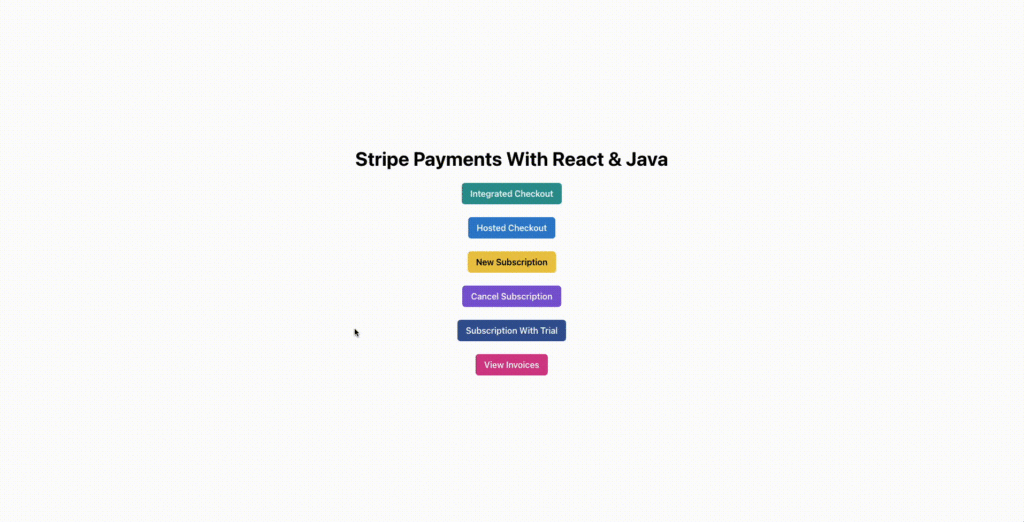
This completes the advance of our demo Java app (frontend & backend). Inside the next segment, you’re going to learn how to deploy this app to Kinsta so that you’ll get right to use it online.
Deploying Your App to Kinsta
Once your tool is ready, you’ll deploy it to Kinsta. Kinsta is helping deployments from your preferred Git provider (Bitbucket, GitHub, or GitLab). Connect your app’s provide code repositories to Kinsta, it routinely deploys your app on each and every instance there’s a change inside the code.
Get able Your Duties
To deploy your apps to production, identify the assemble and deploy directions that Kinsta will use. For frontend, be sure that your package deal deal.json report has the following scripts defined in it:
"scripts": {
"dev": "vite",
"assemble": "NODE_ENV=production tsc && vite assemble",
"get began": "serve ./dist",
"lint": "eslint . --ext ts,tsx --report-unused-disable-directives --max-warnings 0",
"preview": "vite preview"
},
You’re going to moreover want to arrange the serve npm package deal deal that allows you to serve static web websites. This package deal deal it will be used to serve the producing assemble of your app from the Kinsta deployment atmosphere. You’ll arrange it by way of running the following command:
npm i serve
When you assemble your app using vite, all the app it will be packaged proper right into a single report, index.html, since the configuration of React that you just’re using in this educational is meant to create Single Internet web page Systems. While this doesn’t make a huge difference to your consumers, you want to prepare some additional configuration to care for browser routing and navigation in such apps.
With the prevailing configuration, you’ll most efficient get right to use your app at the base URL of your deployment. If the ground URL of the deployment is example.com, any requests to example.com/some-route will lead to HTTP 404 errors.
It’s because your server most efficient has one report to serve, the index.html report. A request sent to example.com/some-route gets began looking for the report some-route/index.html, which doesn’t exist; subsequently it’ll download a 404 Not Came upon response.
To fix this, create a report named serve.json for your frontend/public folder and save the following code in it:
{
"rewrites": [
{ "source": "*", "destination": "/index.html" }
]
}
This report will instruct serve
to rewrite all incoming requests to trail to the index.html report while however showing the path that the original request used to be as soon as sent to inside the response. This may occasionally help you appropriately serve your app’s just right fortune and failure pages when Stripe redirects your customers once more to your app.
For the backend, create a Dockerfile to prepare merely the appropriate atmosphere to your Java tool. Using a Dockerfile promises that the environment equipped to your Java app is similar all through all hosts (be it your local building host or the Kinsta deployment host) and also you’ll be sure that your app runs as expected.
To take a look at this, create a report named Dockerfile inside the backend folder and save the following contents in it:
FROM openjdk:22-oraclelinux8
LABEL maintainer="krharsh17"
WORKDIR /app
COPY . /app
RUN ./mvnw clean package deal deal
EXPOSE 8080
ENTRYPOINT ["java", "-jar", "/app/target/backend.jar"]
This report instructs the runtime to use the OpenJDK Java image as the ground for the deployment container, run the ./mvnw clean package deal deal
command to build your app’s JAR report and use the java -jar
command to execute it. This completes the preparation of the availability code for deployment to Kinsta.
Set Up GitHub Repositories
To get started with deploying the apps, create two GitHub repositories to host your apps’ provide code. While you use the GitHub CLI, you’ll do it by way of the terminal by way of running the following directions:
# Run the ones inside the backend folder
gh repo create stripe-payments-java-react-backend --public --source=. --remote=starting
git init
git add .
git devote -m "Initial devote"
git push starting primary
# Run the ones inside the frontend folder
gh repo create stripe-payments-java-react-frontend --public --source=. --remote=starting
git init
git add .
git devote -m "Initial devote"
git push starting primary
This should create new GitHub repositories for your account and push your apps’ code to them. You’ll have to be capable to get right to use the frontend and backend repositories. Next, deploy the ones repositories to Kinsta by way of following the ones steps:
- Log in to or create your Kinsta account on the MyKinsta dashboard.
- On the left sidebar, click on on Systems and then click on on Add Instrument.
- Inside the modal that appears, select the repository you want to deploy. If when you have multiple branches, you’ll select the desired division and gives a name to your tool.
- Choose one of the vital available wisdom center puts from the list of 25 alternatives. Kinsta routinely detects the start command to your tool.
Remember that you want to provide every your frontend and backend apps with some setting variables for them to artwork appropriately. The frontend tool needs the following atmosphere variables:
- VITE_STRIPE_API_KEY
- VITE_SERVER_BASE_URL
- VITE_CLIENT_BASE_URL
To deploy the backend tool, just do what we did for the frontend, on the other hand for the Assemble atmosphere step, select the Use Dockerfile to prepare container image radio button and enter Dockerfile
since the Dockerfile path to your backend tool.
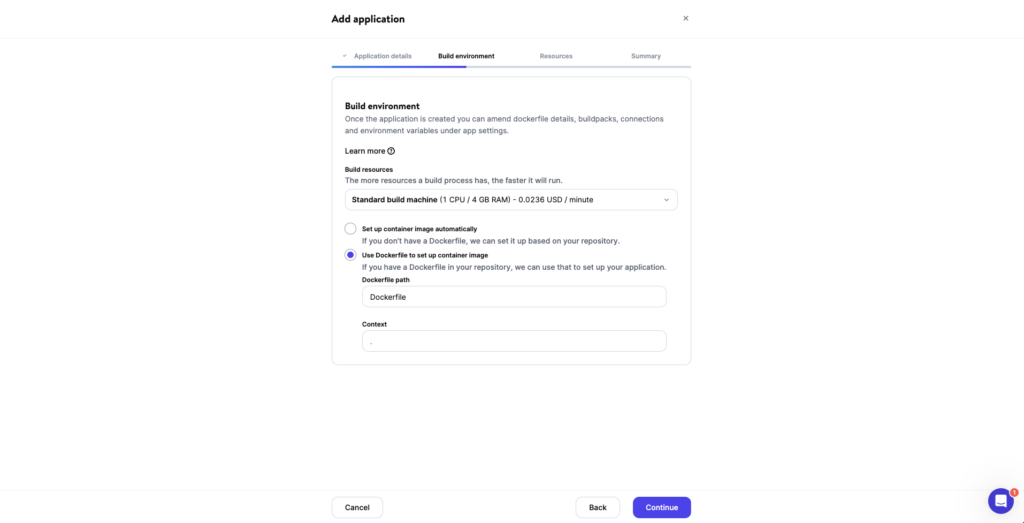
Bear in mind so that you can upload the backend atmosphere variables:
- CLIENT_BASE_URL
- STRIPE_API_KEY
As quickly because the deployment is complete, head over to your systems’ details internet web page and get right to use the deployment’s URL from there.
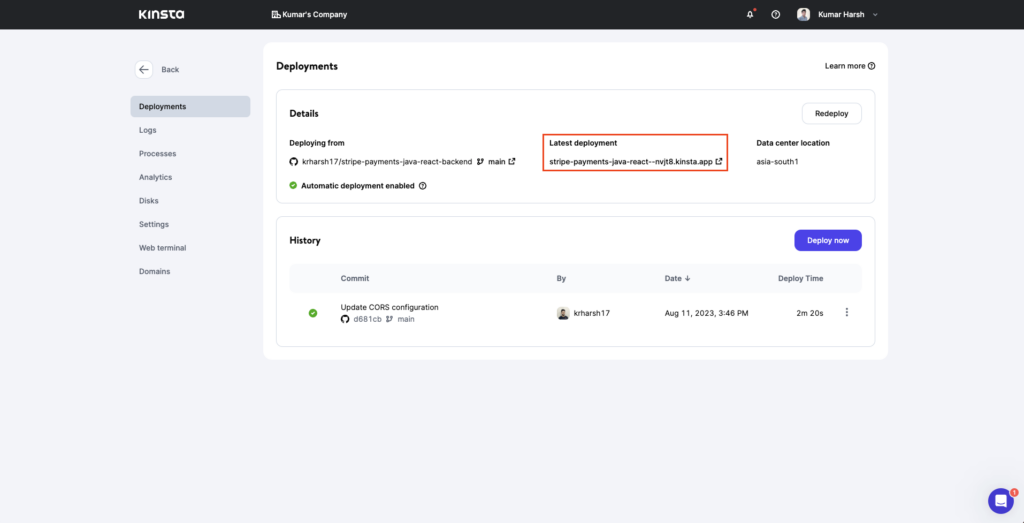
Extract the URLs for every the deployed apps. Head over to the Stripe dashboard to get your secret and publishable API keys.
Be sure that to provide the Stripe publishable key to your frontend app (not the secret key). Moreover, be sure that your base URLs don’t have a trailing forward slash (/
) at their end. The routes already have primary forward slashes, so having a trailing forward slash at the end of the ground URLs will result in two slashes being added to the overall URLs.
For your backend app, add the secret key from the Stripe dashboard (not the publishable key). Moreover, be sure that your client URL does not have a trailing forward slash (/
) at the end.
As quickly because the variables are added, cross to the application Deployments tab and click on at the redeploy button to your backend app. This completes the one-time setup you want to provide your Kinsta deployments with credentials by way of atmosphere variables.
Shifting forward, you’ll devote changes to your style keep watch over. Kinsta will routinely redeploy your tool for individuals who ticked the selection while deploying; otherwise, you want to motive re-deployment manually.
Summary
In this article, you’ve got learned how Stripe works and the fee flows that it provides. You might want to have moreover learned by the use of an extensive example learn the way to mix Stripe into your Java app to easily settle for one-off finances, organize subscriptions, offer unfastened trials, and generate worth invoices.
Using Stripe and Java together, you’ll offer an impressive worth answer to your customers that can scale well and mix seamlessly along with your provide ecosystem of systems and tool.
Do you use Stripe for your app for amassing finances? If certain, which of the two flows do you prefer—hosted, custom designed, or in-app? Let us know inside the comments beneath!
The put up A Information to Stripe Integration in Spring Boot appeared first on Kinsta®.
Contents
- 1 What Is Stripe?
- 2 Stripe Integration in Spring Boot Instrument
- 3 Accepting Online Expenses for Product Purchases
- 4 Setting Up Subscriptions for Unusual Services and products and merchandise
- 5 Generating Invoices for Expenses
- 6 Deploying Your App to Kinsta
- 7 Summary
- 8 A Fast Information to Nonprofit Web site Redesigns [+Best Practices]
- 9 The Final Number of 200+ Absolute best Unfastened Content material Advertising and marketing Templat...
- 10 Why Node.js clustering is vital for optimized packages
0 Comments