In stylish tool development, microservices have emerged as a pivotal construction, enabling scalability, flexibility, and atmosphere pleasant keep watch over of complex tactics.
Microservices are small, impartial techniques that perform particular tasks, bearing in mind flexible deployment and scaling. This modular approach to tool design loosens the coupling between parts, improving flexibility and manageability during development.
The object provides an overview of microservices, their capacity, and their creation the usage of Python. It moreover demonstrates deploying your microservices to Kinsta the usage of a Dockerfile.
What Are Microservices?
Microservices are impartial, self sustaining services and products and merchandise within an device, each and every addressing particular business needs. They be in contact by means of lightweight APIs or message brokers, forming a whole machine.
No longer like monolithic tactics that scale only according to name for, microservices empower scaling specific particular person high-traffic parts. This construction facilitates easy fault keep watch over and feature updates, countering monolithic boundaries.
There are an a variety of benefits to the usage of microservices, similar to:
- Flexibility and scalability — Decoupling specific particular person services and products and merchandise permits you to build up the selection of nodes running an instance of a chosen supplier experiencing over the top web page guests.
- Code modularity — Each and every supplier can use a discrete technology stack, this means that you’ll choose the best development apparatus for each and every one.
Alternatively, some challenging eventualities accompany microservice architectures:
- Monitoring multiple services and products and merchandise — Monitoring specific particular person services and products and merchandise in a machine becomes tricky as instances of a chosen supplier are deployed and distributed right through a variety of nodes. This factor is especially evident all the way through neighborhood failures or other machine issues.
- Price — Developing microservice techniques may also be significantly dearer than construction monolithic tactics on account of the costs associated with managing multiple services and products and merchandise. Each and every supplier requires its private infrastructure and belongings, which can develop into pricey — in particular when scaling up the machine.
How To Design a Microservice The use of Python
Now that you understand the benefits of the usage of a microservice construction, it’s time to build one with Python.
For this situation, assume you want to build an ecommerce web device. The internet web page has a variety of parts, along side the product catalog, a list of orders, and a charge processing machine and logs, each and every of which you need to implement as an impartial supplier. Additionally, you need to determine a service-to-service dialog strategy to transfer data between the ones services and products and merchandise, similar to HTTP, effectively.
Let’s assemble a microservice the usage of Python to control a product catalog. The microservice will fetch product data from a specified provide and return the ideas in JSON construction.
Must haves
To observe this educational, you should definitely have:
- Familiarity with Flask
- Python, Postman Consumer, and Docker Desktop installed on your tool
1. Create Your Problem
- To get started, create a folder for your enterprise known as flask-microservice and provide checklist into the enterprise’s checklist.
- Next, run
python3 --version
to confirm that Python is installed on your computer appropriately. - Arrange
virtualenv
to create an isolated development setting for the Flask microservice thru running the command beneath:pip3 arrange virtualenv
- Create a virtual setting thru running the following:
virtualenv venv
- In the end, flip at the virtual setting the usage of some of the essential following directions according to your computer’s running machine:
# House home windows: .venvScriptsactivate # Unix or macOS: provide venv/bin/activate
2. Set Up a Flask Server
Inside the root checklist, create a prerequisites.txt record and add the ones dependencies.
flask
requests
Run the pip3
command on your terminal to place within the dependencies.
pip arrange -r prerequisites.txt
Next, create a brand spanking new folder inside the root checklist and name it services and products and merchandise. Inside this folder, create a brand spanking new record, products.py, and add the code beneath to organize a Flask server.
import requests
import os
from flask import Flask, jsonify
app = Flask(__name__)
port = int(os.environ.get('PORT', 5000))
@app.route("/")
def area():
return "Hello, this is a Flask Microservice"
if __name__ == "__main__":
app.run(debug=True, host="0.0.0.0", port=port)
Inside the code above, a basic Flask server is able up. It initializes a Flask app, defines a single route for the root URL ("/"
), and when accessed, displays the message "Hello, this is a Flask Microservice"
. The server runs on a specified port, purchased from an environment variable or defaults to port 5000
, and starts in debugging mode, making it in a position to handle incoming requests.
3. Define the API Endpoints
With the server configured, create an API endpoint for a microservice that fetches product data from a publicly to be had API. Add this code to the products.py record:
BASE_URL = "https://dummyjson.com"
@app.route('/products', methods=['GET'])
def get_products():
response = requests.get(f"{BASE_URL}/products")
if response.status_code != 200:
return jsonify({'error': response.json()['message']}), response.status_code
products = []
for product in response.json()['products']:
product_data = {
'id': product['id'],
'title': product['title'],
'logo': product['brand'],
'price': product['price'],
'description': product['description']
}
products.append(product_data)
return jsonify({'data': products}), 200 if products else 204
The code above creates an /products
endpoint inside the Flask server. When accessed by means of a GET request, it fetches product data from a dummy API. If successful, it processes the retrieved data, extracts product details, and returns the ideas in a JSON construction. In case of errors or no available data, it responds with an appropriate error message and status code.
4. Test the Microservice
At this stage, you’ve got successfully organize a simple microservice. To start out out the supplier, spin up the improvement server, which is in a position to get began running at http://localhost:5000
.
flask --app services and products and merchandise/products run
You then’ll make a GET
request to the /products
endpoint the usage of the Postman client, you’ll have to see a an identical response to the screenshot beneath.
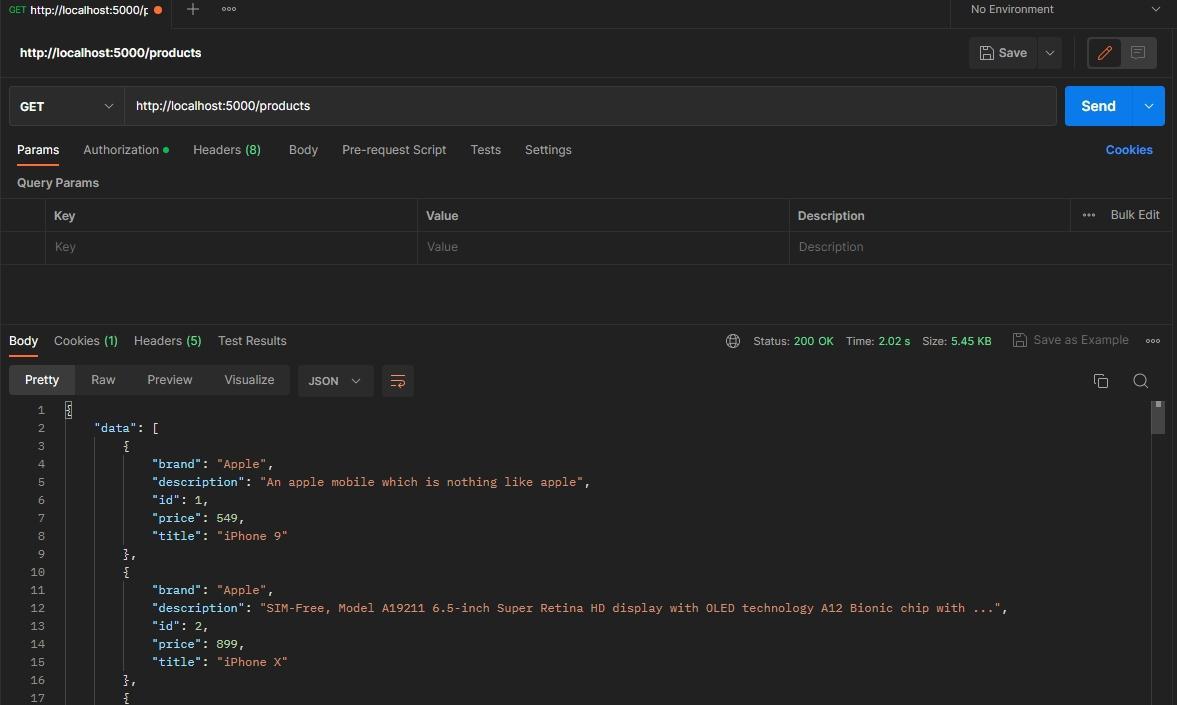
GET
request in Postman.How To Implement Authentication and Authorization in a Python Microservice
When construction microservices, it’s essential to implement tough security measures similar to authentication and authorization. Securing your microservice promises that most straightforward licensed shoppers can get entry to and use the supplier, protecting subtle data and preventing malicious attacks.
One environment friendly method for implementing protected authentication and authorization in microservices is JSON Web Tokens (JWTs).
JWT is a extensively used open usual that provides a safe and atmosphere pleasant approach of transmitting authentication knowledge between clients and servers. They’re compact, encrypted, and digitally signed tokens you move alongside HTTP requests. When you include a JWT with each and every request, the server can in short take a look at a shopper’s identity and permissions.
To implement JWT authentication inside the microservice, do the following:
- Add Python’s
pyjwt
package deal to your prerequisites.txt record and reinstall the dependencies the usage ofpip arrange -r prerequisites.txt
. - Given that supplier doesn’t have a loyal database, create a shoppers.json record inside the root checklist of your enterprise to store a list of licensed shoppers. Paste the code beneath inside the record:
[ { "id": 1, "username": "admin", "password": "admin" } ]
- Then, on your services and products and merchandise/products.py record, replace the import statements with the following:
import requests from flask import Flask, jsonify, request, make_response import jwt from functools import wraps import json import os from jwt.exceptions import DecodeError
You may well be importing the ones modules to handle HTTP requests, create a Flask app, arrange JSON data, implement JWT-based authentication, and handle exceptions, enabling numerous choices right through the Flask server.
- Add the following code beneath the Flask app instance creation to generate a secret key that will probably be used to sign the JWT tokens.
app.config['SECRET_KEY'] = os.urandom(24)
- To verify JWTs, create a decorator function and add the following code above the API routes on your Flask server code. This decorator function will authenticate and validate shoppers previous to they get entry to protected routes.
def token_required(f): @wraps(f) def decorated(*args, **kwargs): token = request.cookies.get('token') if no longer token: return jsonify({'error': 'Authorization token is missing'}), 401 check out: data = jwt.decode(token, app.config['SECRET_KEY'], algorithms=["HS256"]) current_user_id = data['user_id'] aside from DecodeError: return jsonify({'error': 'Authorization token is invalid'}), 401 return f(current_user_id, *args, **kwargs) return decorated
This decorator function tests incoming HTTP requests for a JWT authorization token, which must be inside the request headers or cookies. If the token is missing or invalid, the decorator sends an
unauthorized status code
message as a response.Conversely, if a valid token is supply, the decorator extracts the shopper ID after interpreting it. This process safeguards the protected API endpoints thru granting get entry to most straightforward to licensed shoppers.
- Define an API endpoint for client authentication the usage of the code beneath.
with open('shoppers.json', 'r') as f: shoppers = json.load(f) @app.route('/auth', methods=['POST']) def authenticate_user(): if request.headers['Content-Type'] != 'device/json': return jsonify({'error': 'Unsupported Media Type'}), 415 username = request.json.get('username') password = request.json.get('password') for client in shoppers: if client['username'] == username and client['password'] == password: token = jwt.encode({'user_id': client['id']}, app.config['SECRET_KEY'],algorithm="HS256") response = make_response(jsonify({'message': 'Authentication successful'})) response.set_cookie('token', token) return response, 200 return jsonify({'error': 'Invalid username or password'}), 401
To authenticate and authorize shoppers, the
/auth
API endpoint tests the credentials inside thePOST
request’s JSON payload towards the report of allowed shoppers. If the credentials are reliable, it generates a JWT token the usage of the shopper’s ID and the app’s secret key and devices the token as a cookie inside the response. Shoppers can now use this token to make subsequent API requests.After creating the
/auth
endpoint, use Postman to send an HTTPPOST
request tohttp://localhost:5000/auth
. Inside the request body, include the credentials of the mock admin client you created.Postman request showing the request body. If the request succeeds, the API will generate a JWT token, set it in Postman’s cookies, and send an authenticated just right fortune response.
- In the end, change the
GET
API endpoint to check for and take a look at the JWT token the usage of the code beneath:@app.route('/products', methods=['GET']) @token_required def get_products(current_user_id): headers = {'Authorization': f'Bearer {request.cookies.get("token")}'} response = requests.get(f"{BASE_URL}/products", headers=headers) if response.status_code != 200: return jsonify({'error': response.json()['message']}), response.status_code products = [] for product in response.json()['products']: product_data = { 'id': product['id'], 'title': product['title'], 'logo': product['brand'], 'price': product['price'], 'description': product['description'] } products.append(product_data) return jsonify({'data': products}), 200 if products else 204
How To Containerize Python Microservices With Docker
Docker is a platform that packages techniques and their dependencies in an isolated development setting. Packaging microservices in packing containers streamlines their deployment and keep watch over processes in servers as each and every supplier runs and executes independently in its container.
To containerize the microservice, you will have to create a Docker image from a Dockerfile that specifies the dependencies required to run the application in a container. Create a Dockerfile inside the root checklist of your enterprise and add the ones instructions:
FROM python:3.9-alpine
WORKDIR /app
COPY prerequisites.txt ./
RUN pip arrange -r prerequisites.txt
COPY . .
EXPOSE 5000
CMD ["python", "./services/products.py"]
Faster than construction the image, evaluation the ones directions:
FROM
— Instructs Docker which base image to use. A base image is a pre-built instance containing the tool and dependencies to run the Flask device in a container.WORKDIR
— Devices the specified checklist right through the container for the reason that working checklist.COPY prerequisites.txt ./
— Copies the dependencies inside the prerequisites.txt record into the container’s prerequisites.txt record.RUN
— Runs the specified command to place within the dependencies the image requires.COPY . .
— Copies the entire information from the enterprise’s root checklist to the working checklist right through the container.EXPOSE
— Specifies the port where the container can pay consideration for requests. Alternatively, Docker doesn’t post the port to the host tool.CMD
— Specifies the default command to execute when the container starts.
Next, add a .dockerignore record inside the root checklist of your enterprise to specify the information that the Docker image must exclude. Proscribing the image contents will scale back its final size and similar assemble time.
/venv
/services and products and merchandise/__pycache__/
.gitignore
Now, run the command beneath to build the Docker image:
docker assemble -t flask-microservice .
In the end, once the image builds, you’ll run the microservice in a Docker container the usage of the following command:
docker run -p 5000:5000 flask-microservice
This command gets began a Docker container running the microservice and expose port 5000 on the container to port 5000 on the host tool, allowing you to make HTTP requests from your web browser or Postman the usage of the URL http://localhost:5000
.
Deploy Python Microservices With Kinsta
Kinsta supplies managed hosting solutions for web techniques and databases — you’ll seamlessly deploy and arrange your Python microservices and backend APIs in a producing setting.
Observe the ones steps to configure your Flask microservice for deployment with MyKinsta:
- First, create a brand spanking new Procfile inside the root checklist and add the code beneath. It specifies the command to run the Flask microservice on Kinsta’s Gunicorn WSGI HTTP Server for Python techniques.
web: gunicorn services and products and merchandise.wsgi
- For your prerequisites.txt record, add the Gunicorn dependency:
gunicorn==20.1.*
- Next, create a brand spanking new services and products and merchandise/wsgi.py record and add the code beneath.
from services and products and merchandise.products import app as device if __name__ == "__main__": device.run()
- Create a .gitignore record inside the enterprise root folder and add the following:
services and products and merchandise/__pycache__ venv
- In the end, create a brand spanking new repository on GitHub and push your enterprise information.
Once your repo is in a position, observe the ones steps to deploy the Flask microservice to Kinsta:
- Log in or create an account to view your MyKinsta dashboard.
- Authorize Kinsta at the side of your Git provider (Bitbucket, GitHub, or GitLab).
- Click on on Applications on the left sidebar, then click on on Add device.
- On the dashboard, click on on on Add Supplier, and choose Software.
- Select the repository and the dep. you need to deploy from.
- Assign a novel name to your app and choose a data center location.
- To configure the Assemble setting, choose the selection to use a Dockerfile to construct the container symbol.
- Provide the path to your Dockerfile and the context.
- In the end, evaluation other knowledge and click on on Create device.
Test the Microservice
As quickly because the deployment process is successful, click on at the provided URL to test the microservice thru making HTTP requests in Postman. Make a GET
request to the root endpoint.
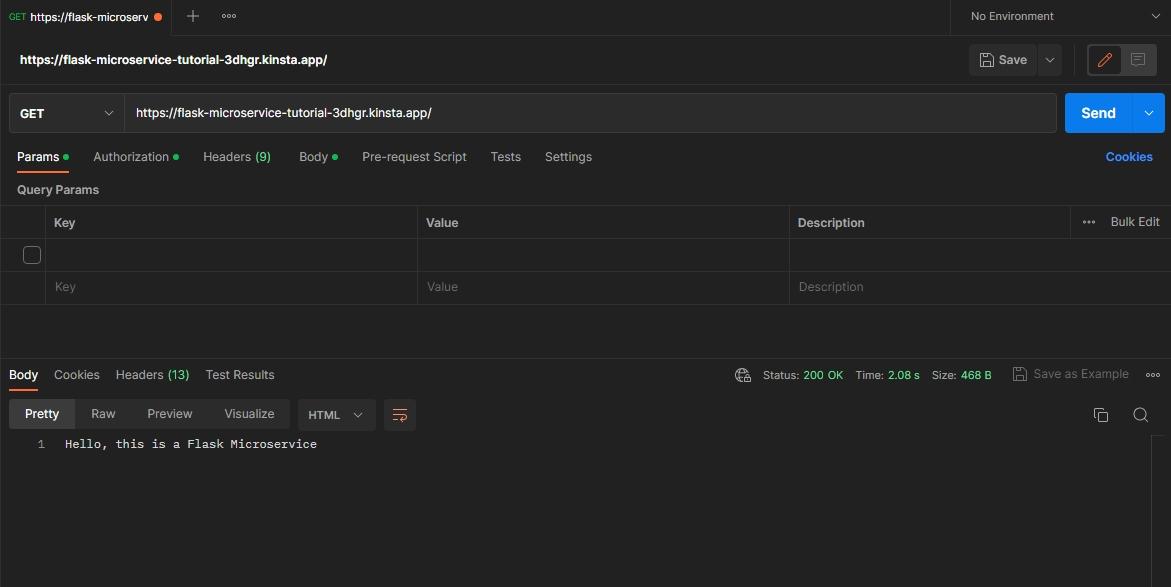
GET
request to the microservice’s product
endpoint.To authenticate and generate a JWT token, send a POST
request to the /auth
API endpoint, passing the admin credentials inside the body of the request.
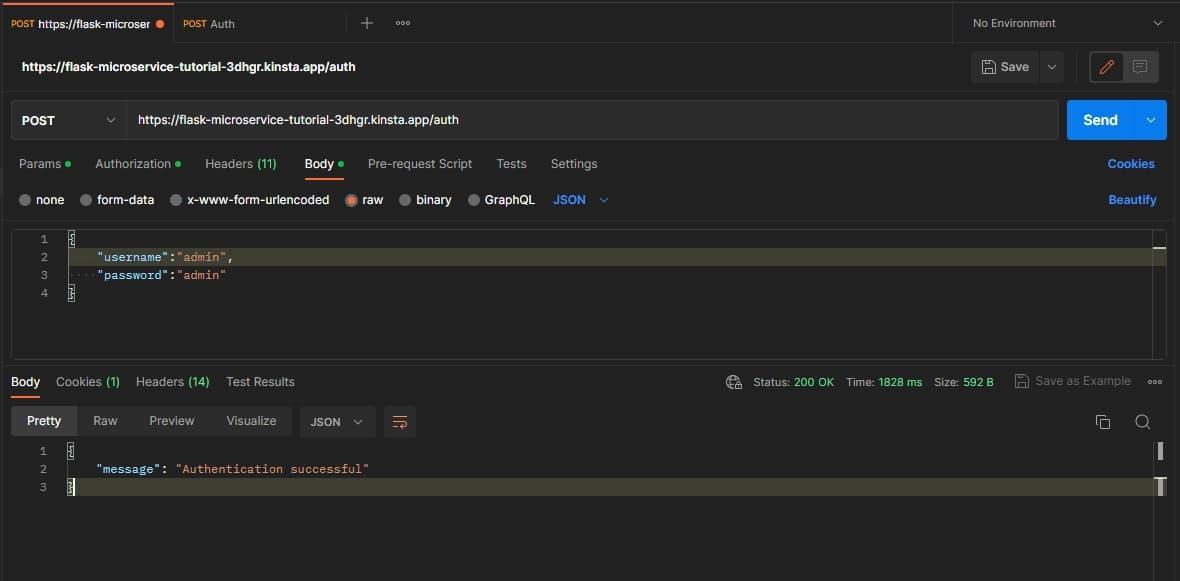
POST
request to microservice auth
endpoint.After all, after successfully getting authenticated, make a GET
request to the /products
endpoint to fetch data.
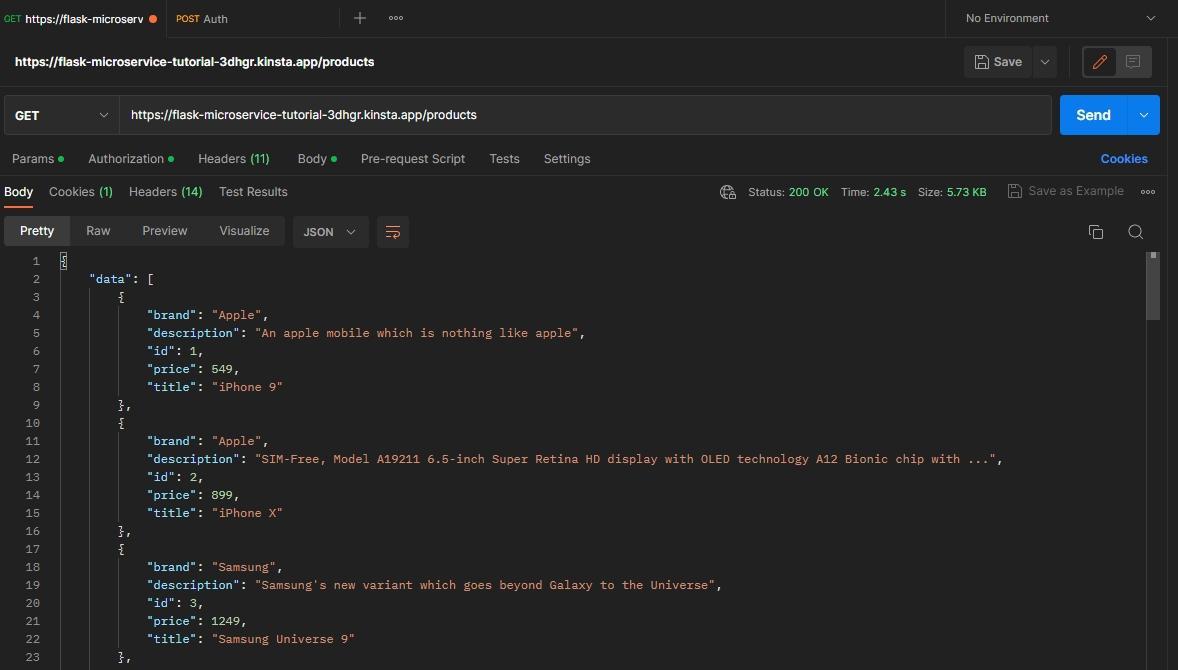
GET
request to a microservice products
endpoint.Summary
As techniques increase in size and complexity, adopting construction patterns that allow tool tactics to scale without straining available belongings is an important.
Microservices construction supplies scalability, development flexibility, and maintainability, making it more uncomplicated in an effort to arrange complex techniques.
Kinsta simplifies the process of hosting your microservices. It permits you to with out issues use your hottest database and very easily host each and every your software and database by means of a unified dashboard.
The submit How To Construct and Deploy Microservices With Python gave the impression first on Kinsta®.
Contents
- 1 What Are Microservices?
- 2 How To Design a Microservice The use of Python
- 3 How To Implement Authentication and Authorization in a Python Microservice
- 4 How To Containerize Python Microservices With Docker
- 5 Deploy Python Microservices With Kinsta
- 6 Summary
- 7 Native Cloud Backups Upload-on Educational: Easy methods to Make the Maximum of It
- 8 Being a Virtual Author: The whole thing You Wish to Know [+ Tips to Get Started]
- 9 5 Seamless Examples of Divi AI-Generated Web pages (& Their Activates)
0 Comments