This hands-on article harnesses WordPress’s flexibility and React‘s powerful client interface (UI) for theme development. It demonstrates how integrating WordPress and React elevates your WordPress duties by way of walking you right through the stairs needed to create a theme.
Prerequisites
To use in conjunction with this text, you’ll have the following:
- A WordPress internet web page. Kinsta provides multiple setup possible choices, along side native building with DevKinsta, a user-friendly MyKinsta dashboard, or programmatically by means of the Kinsta API.
- Node.js and npm (Node Package deal Supervisor) or yarn installed on your computer
Create a standard WordPress theme development
Making a standard WordPress theme development involves putting in a series of data and directories that WordPress uses to make use of your theme’s sorts, functionalities, and layouts to a WordPress internet web page.
- To your DevKinsta atmosphere, get right of entry to your internet web page’s folder. Navigate to the wp-content/problems checklist.
- Create a brand spanking new folder on your theme. The folder establish should be unique and descriptive — for example, my-basic-theme.
- Inside the theme’s folder, create the ones essential knowledge and move away them empty:
- style.css — That’s the idea stylesheet record. It moreover incorporates the header wisdom on your theme.
- functions.php — This record defines functions, classes, actions, and filters to be used by way of your theme.
- index.php — That’s the idea template record. It’s required for all problems.
For many who aren’t the use of React, you’ll have to moreover create the following knowledge. On the other hand with React we’d create portions for them later.
- header.php — Contains the header section of your internet web page.
- footer.php — Contains the footer section of your internet web page.
- sidebar.php — For the sidebar section, if your theme incorporates sidebars.
Next, open style.css and add the following to the easiest of the record:
/*
Theme Determine: My Basic Theme
Theme URI: http://example.com/my-basic-theme/
Author: Your Determine
Author URI: http://example.com
Description: A standard WordPress theme.
Fashion: 1.0
License: GNU Elementary Public License v2 or later
License URI: http://www.gnu.org/licenses/gpl-2.0.html
Tags: blog, custom-background
Text House: my-basic-theme
*/
This code snippet is the header section for a WordPress theme’s style.css record, containing essential metadata similar to the theme’s establish, writer details, type, and license. It’s serving to WordPress recognize and display the theme inside the dashboard, along side its description and tags for categorization.
Mix React into WordPress
Integrating React proper right into a WordPress theme lets you use React’s component-based construction to build dynamic, interactive UIs within your WordPress internet web page. To mix React, you’ll use the @wordpress/scripts bundle.
This is a number of reusable scripts tailored for WordPress development. WordPress provides it to simplify the configuration and assemble process, specifically when integrating trendy JavaScript workflows, related to React, into WordPress problems and plugins.
This toolset wraps not unusual tasks, making it more uncomplicated to enlarge with JavaScript inside the WordPress ecosystem.
Adapt your theme
Now that you simply’ve were given a standard WordPress theme development, you’ll adapt your theme.
- Within your theme’s checklist, paste the following code into the functions.php record:
<?php function my_react_theme_scripts() { wp_enqueue_script('my-react-theme-app', get_template_directory_uri() . '/assemble/index.js', array('wp-element'), '1.0.0', true); wp_enqueue_style('my-react-theme-style', get_stylesheet_uri()); } add_action('wp_enqueue_scripts', 'my_react_theme_scripts');
The functions.php record integrates React along side your WordPress theme. It uses
wp_enqueue_script
andwp_enqueue_style
functions so to upload JavaScript and cascading style sheet (CSS) knowledge on your theme.The
wp_enqueue_script
WordPress function takes plenty of arguments:- The handle establish (
'my-react-theme-app'
), which uniquely identifies the script - The path to the script record
- The array of dependencies,
array('wp-element')
, which indicates the script relies on WordPress’s wrapper for React('wp-element')
. - The type amount
('1.0.0')
- The positioning
true
, which specifies that the script should be loaded inside the footer of the HTML report back to toughen internet web page load potency
The
wp_enqueue_style
function takes the following arguments:- The handle establish
'my-react-theme-style'
, which uniquely identifies the stylesheet - The provision
get_stylesheet_uri()
, which returns the URL to the theme’s number one stylesheet (style.css) and promises the theme’s sorts are performed
- The
add_action
element, which hooks a practice function'my_react_theme_scripts'
to a decided on movement ('wp_enqueue_scripts'
). This promises that your JavaScript and CSS are as it should be loaded when WordPress prepares to render the internet web page.
This code promises that your React app’s compiled JavaScript record, positioned in /assemble/index.js, and your theme’s number one stylesheet are loaded along side your theme.
The /assemble checklist typically comes from compiling your React app the use of a tool like
create-react-app
or webpack, which bundles your React code proper right into a production-ready, minified JavaScript record.This setup is essential for integrating React capacity into your WordPress theme, bearing in mind dynamic, app-like client research within a WordPress internet web page.
- The handle establish (
- Next, substitute the content material subject material of the index.php record:
<html > <meta charset=""> <body >
The code inside the index.php record defines the fundamental HTML development of the WordPress theme, along side hooks for WordPress to insert vital headers (
wp_head
) and footers (wp_footer
) and adiv
with the IDapp
where the React tool is mounted.
Organize React with @wordpress/scripts
- Open your terminal and navigate on your theme’s checklist:
cd wp-content/problems/my-basic-theme
- Initialize a brand spanking new Node.js endeavor:
npm init -y
- Arrange
@wordpress/scripts
and@wordpress/element
:npm arrange @wordpress/scripts @wordpress/element --save-dev
Follow that this step can take a few minutes.
- Modify your package deal.json record to include a
get began
and aassemble
script:"scripts": { "get began": "wp-scripts get began", "assemble": "wp-scripts assemble" },
The
'@wordpress/scripts'
is used to begin out a development server with scorching reloading for development purposes (get began
) and to convey in combination the React tool into static property for production (assemble
).
Create a React endeavor
- Create a brand spanking new checklist named src on your React provide knowledge within your theme.
- During the src folder, create two new knowledge: index.js and App.js.
- Place the following code into index.js:
import { render } from '@wordpress/element'; import App from './App'; render(, record.getElementById('app'))
The code above imports the
render
function from@wordpress/element
and theApp
issue. Then, it mounts theApp
issue to the Document Object Taste (DOM). - Next, paste this code into App.js record:
import { Section } from '@wordpress/element'; export default magnificence App extends Section { render() { return (
Hello, WordPress and React!
{/* Your React portions will move proper right here */}
Finalize and switch for your theme
To show for your theme:
- Run the improvement server with
npm get began
. - Flip for your new theme inside the WordPress dashboard by way of navigating to Glance > Matter issues, discovering your theme, and clicking Activate.
- Be sure your React endeavor’s assemble process is as it should be configured to output to the right kind theme checklist, allowing WordPress to render your React portions.
- Consult with your WordPress internet web page’s frontend to look the live changes.

Develop React portions for the theme
Next, observe a component-based solution to extend the fundamental React setup on your WordPress theme with specific portions, like a header.
Create the header issue
To your theme’s src checklist, create a brand spanking new record for the header issue. Give it a name, related to Header.js, and add the following code:
import { Section } from '@wordpress/element';
magnificence Header extends Section {
render() {
const { toggleTheme, darkTheme } = this.props;
const headerStyle = {
backgroundColor: darkTheme ? '#333' : '#EEE',
color: darkTheme ? 'white' : '#333',
padding: '10px 20px',
display: 'flex',
justifyContent: 'space-between',
alignItems: 'middle',
};
return (
My WP Theme
);
}
}
export default Header;
This code defines a header issue the use of '@wordpress/element'
that dynamically sorts the header in line with darkTheme prop
. It includes a button to toggle between delicate and dark problems by way of invoking the toggleTheme
approach passed as a prop.
To your theme’s src checklist, create a brand spanking new record for the footer issue. Give it a name — for example, Footer.js — and add the following code:
import { Section } from '@wordpress/element';
magnificence Footer extends Section {
render() {
const { darkTheme } = this.props;
const footerStyle = {
backgroundColor: darkTheme ? '#333' : '#EEE',
color: darkTheme ? 'white' : '#333',
padding: '20px',
textAlign: 'middle',
};
return (
);
}
}
export default Footer;
This code defines a footer issue that renders a footer with dynamic styling in line with the darkTheme
prop and shows the prevailing one year.
Change the App.js record
To make use of the new header and footer, exchange the content material subject material of the App.js record with the following code:
import { Section } from '@wordpress/element';
import Header from './Header';
import Footer from './Footer';
export default magnificence App extends Section {
state = {
darkTheme: true,
};
toggleTheme = () => {
this.setState(prevState => ({
darkTheme: !prevState.darkTheme,
}));
};
render() {
const { darkTheme } = this.state;
return (
);
}
}
The development assemble process, which watches for changes and recompiles your code, should however be full of life. So, your closing type of the template should look similar to this:

How you can handle WordPress wisdom in React
Integrating WordPress content material subject material within React methods offers a unbroken bridge between WordPress’s tough content material control purposes and React’s dynamic UI design. This is imaginable with the WordPress REST API.
To get right of entry to the WordPress REST API, permit it by way of updating the permalink settings. On the WordPress admin dashboard, navigate to Settings > Permalinks. Select the Put up establish chance or any chance moderately than Easy and save your changes.
To your theme’s src checklist, create a brand spanking new record named Posts.js and add this code:
import { Section } from '@wordpress/element';
magnificence Posts extends Section {
state = {
posts: [],
isLoading: true,
error: null,
};
componentDidMount() {
fetch('http://127.0.0.1/wordpress_oop/wp-json/wp/v2/posts')
.then(response => {
if (!response.good enough) {
throw new Error('Something went unsuitable');
}
return response.json();
})
.then(posts => this.setState({ posts, isLoading: false }))
.catch(error => this.setState({ error, isLoading: false }));
}
render() {
const { posts, isLoading, error } = this.state;
if (error) {
return Error: {error.message};
}
if (isLoading) {
return Loading...;
}
return (
{posts.map(submit => (
))}
);
}
}
export default Posts;
The fetch('http://127.0.0.1/wordpress_oop/wp-json/wp/v2/posts')
URL might be somewhat different depending on the WP deployment establish — that is, the folder where you installed WP. On the other hand, you can take the internet web page hostname from the DevKinsta dashboard and append the suffix /wp-json/wp/v2/posts
.
The Posts
issue is a big example of this integration, demonstrating the process of fetching and managing WordPress wisdom — specifically posts — the use of the WordPress REST API.
Thru starting a neighborhood request throughout the issue’s lifecycle approach, componentDidMount
, the issue effectively retrieves posts from a WordPress internet web page and retail outlets them in its state. This method highlights a construction for dynamically incorporating WordPress wisdom, related to pages or custom submit types, into React portions.
To make use of a brand spanking new issue, exchange the content material subject material of the App.js record with the following code:
import { Section } from '@wordpress/element';
import Header from './Header';
import Footer from './Footer';
import Posts from './Posts'; // Import the Posts issue
export default magnificence App extends Section {
state = {
darkTheme: true,
};
toggleTheme = () => {
this.setState(prevState => ({
darkTheme: !prevState.darkTheme,
}));
};
render() {
const { darkTheme } = this.state;
return (
{/* Render the Posts issue */}
);
}
}
You’ll be able to now check out the most recent and supreme type of your theme. Along side the header and footer, it’s composed of a dynamic content material subject material area that pieces the posts.
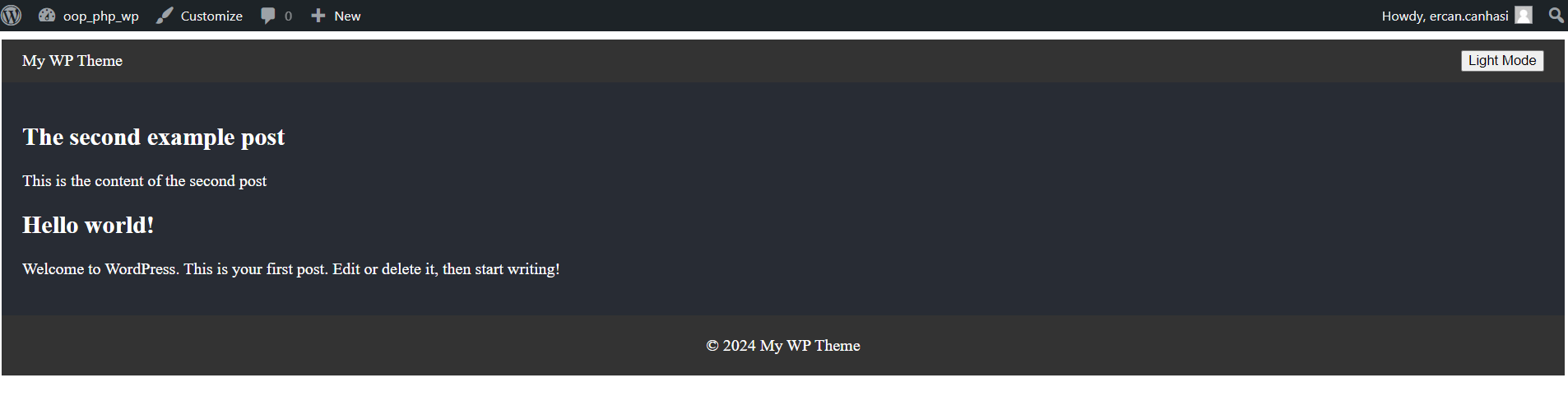
Use the React WordPress theme in a WordPress endeavor
Integrating a React-based theme proper right into a WordPress endeavor begins with transforming React code proper right into a WordPress-compatible construction, leveraging methods like @wordpress/scripts
. This tool simplifies the assemble process, letting you convey in combination React methods into static property that WordPress can enqueue.
Building the theme is discreet with the npm directions supplied by way of @wordpress/scripts
. Running npm run assemble
inside the theme checklist compiles the React code into static JavaScript and CSS knowledge.
Then you definitely no doubt place the ones compiled property in the most efficient checklist throughout the theme, ensuring WordPress can as it should be load and render the React portions as part of the theme. Once integrated, you can flip at the React WordPress theme like every other, instantly bringing a modern, app-like client experience to the WordPress internet web page.
Summary
Thru development and integrating a theme into WordPress the use of React’s powerful UI purposes, you can liberate the opportunity of growing flexible, extraordinarily interactive, and user-centric web research.
For many who’re ready to take your React WordPress problems live, Kinsta offers a managed WordPress Website hosting provider with a safe infrastructure, edge caching, and other choices that boost your internet web page’s speed and serve as.
Are you taking into account development a WordPress theme with React? Please percentage some tips on why you assume it’s highest and move about it.
The submit How one can construct a WordPress theme with React gave the impression first on Kinsta®.
Related posts:
Contents
- 1 Hello, WordPress and React!
- 1.1 Develop React portions for the theme
- 1.2 How you can handle WordPress wisdom in React
- 1.3
- 1.4 Use the React WordPress theme in a WordPress endeavor
- 1.5 Summary
- 1.6 How you can Upload Arrow-key Keyboard Navigation in WordPress
- 1.7 How to Make Your Footer Sticky with Divi
- 1.8 New Starter Site For Writers (Quick Install)
0 Comments