Managing the state of any WordPress app — how it handles and organizes wisdom — can be tricky. As your undertaking grows, keeping an eye on the go with the flow of information and ensuring consistent updates right through portions becomes an increasing number of tough. The WordPress wisdom package deal deal can lend a hand proper right here, as it provides a powerful answer for state keep an eye on.
This article seems into the WordPress wisdom package deal deal, exploring its key concepts, implementation strategies, and highest practices.
Introducing the WordPress wisdom package deal deal
The WordPress knowledge package deal — officially @wordpress/wisdom
— is a JavaScript (ES2015 and higher) state keep an eye on library that provides a predictable and centralized approach to prepare application state. The most productive implementation can help in making it more straightforward to build complex individual interfaces and handle wisdom go with the flow right through your application.
The WordPress wisdom package deal deal takes its inspiration from Redux, a popular state keep an eye on library inside the React ecosystem.
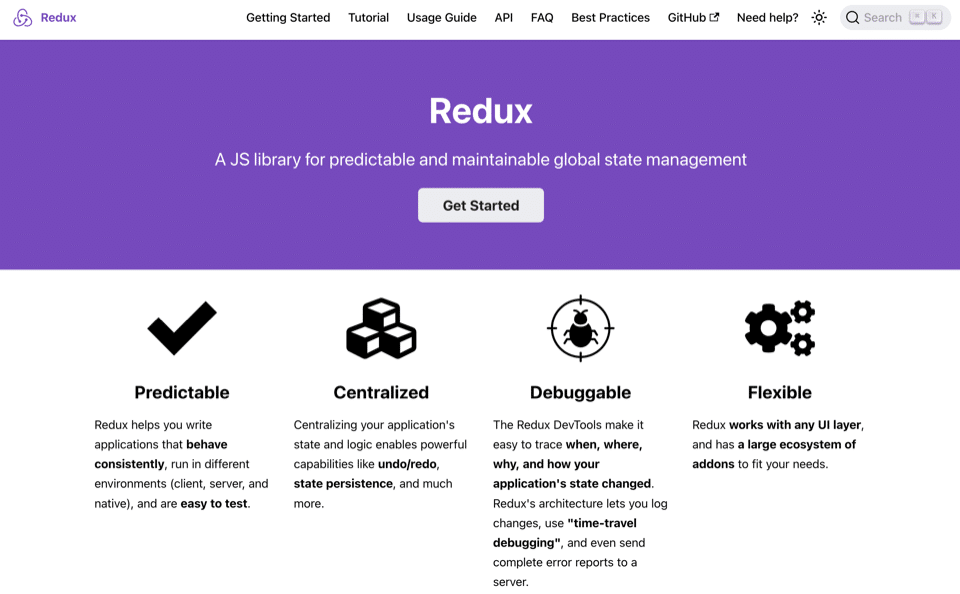
Proper right here, the information module works right through the WordPress setting and gives integrations with WordPress-specific capacity and APIs. If you happen to assemble for the WordPress Block Editor — or it’s something it’s necessary to strengthen — the package deal deal will likely be a very powerful in managing its state. By way of the usage of the identical apparatus and patterns for your non-public plugins and topic issues, you’ll have the ability to create a further consistent and familiar building enjoy.
The package deal deal’s relationship to Redux
While the WordPress wisdom package deal deal draws inspiration from Redux, it’s not a right away port. There are many variations to fit the WordPress ecosystem, with some key permutations between the two solutions:
- The data package deal deal is designed to art work seamlessly with WordPress APIs and capacity, which vanilla Redux can’t do without that adaptation.
- Compared to Redux, the information package deal deal provides a further streamlined API. This will likely assist you get started.
- No longer like Redux, the information package deal deal accommodates built-in strengthen for asynchronous movements. If you happen to art work with the WordPress REST API, this will likely be useful.
The WordPress wisdom package deal deal moreover has some comparisons with the REST API. While they each and every deal with wisdom keep an eye on, they serve different purposes:
- The WordPress REST API provides a approach to interact with WordPress wisdom over HTTP. You’ll use it for external apps, headless WordPress setups, and anyplace you need to retrieve and manipulate knowledge.
- The WordPress wisdom package deal deal provides a centralized store for wisdom and UI state. It’s a approach to handle wisdom go with the flow and updates within your app.
In a variety of instances, you’ll use each and every together: the REST API to fetch and change wisdom on the server and the WordPress wisdom package deal deal to keep an eye on that wisdom within your application.
Key concepts and terminology for the WordPress wisdom package deal deal
The WordPress wisdom package deal deal provides an intuitive means of managing state. This refers to the wisdom within a store. It represents the prevailing scenario of your application and can include each and every UI state (corresponding as to whether or now not there’s an open modal) and data state (similar to a listing of posts).
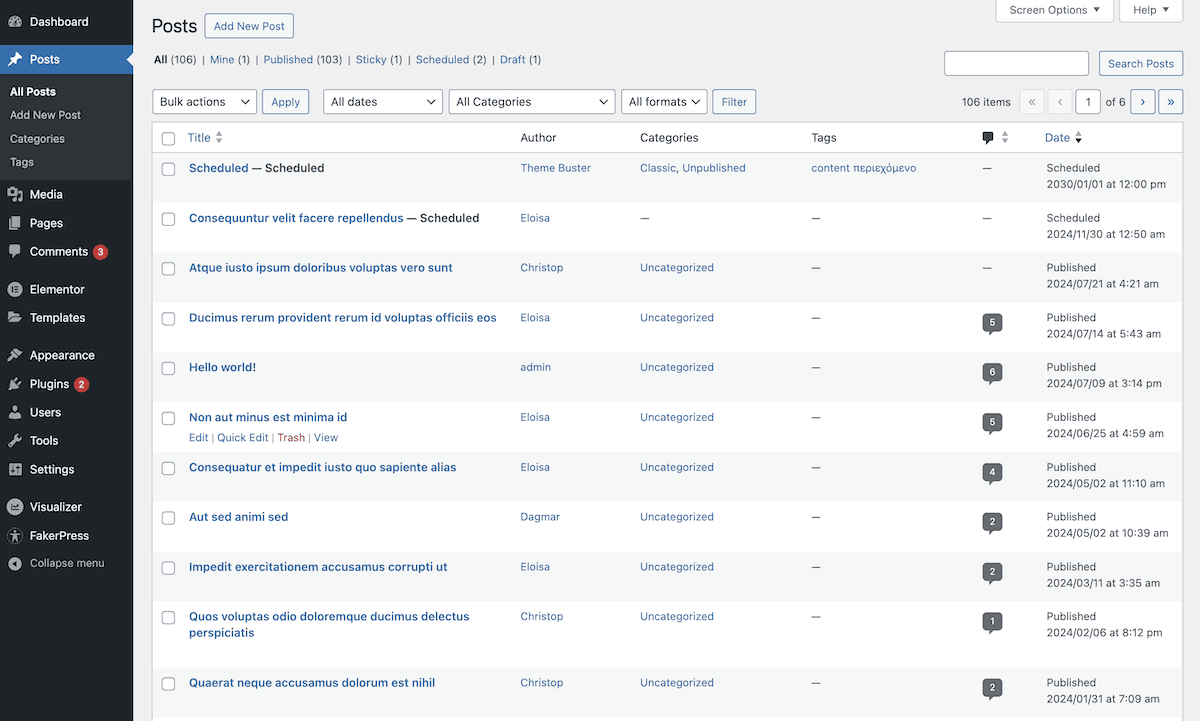
In this context, a store is the central hub of the WordPress wisdom package deal deal. It holds the internet web page’s entire state and gives the learn how to get admission to and change that state. In WordPress, you’ll have the ability to have a few stores. Each one will likely be in control of a decided on space of your internet web page.
To keep an eye on those stores, you need a registry. This central object provides learn how to enroll new stores and get admission to your present ones. A registry will snatch stores, and those stores will snatch your application state.
There are a few ways to art work with state:
- Actions describe the changes to a state. The ones are easy JavaScript devices and are the only approach to purpose state updates. Actions will in most cases have a
type
assets, which describes the movement. It might also include additional wisdom. - Selectors extract specific pieces of state from the store. The ones functions imply you’ll get admission to state wisdom without the need for direct interaction with the store’s building. Resolvers are similar and handle asynchronous knowledge fetching. You use the ones to make sure that you’ll have the ability to get admission to the required wisdom in a store previous to you run a selector.
- Reducers specify how the state will have to trade in line with actions. They take the prevailing state and an movement as arguments and return a brand spanking new state object. Keep watch over functions let the reducers handle complex async operations without negative effects.
You want to snatch the ones basic concepts, as they all art work together to create a powerful state keep an eye on gadget with stores at its middle.
Stores: the central hub of the WordPress wisdom package deal deal
Stores are the bins for your application’s state and provide the learn how to interact with it. The WordPress wisdom package deal deal bundles together a few other packages, and every of the ones registers stores for the Block record, Block Editor, core, publish bettering, and further.
Each store can have a novel namespace, similar to core
, core/editor
, and core/notices
. Third-party plugins will enroll stores, too, so you need to choose unique namespaces to keep away from conflicts. Regardless, stores you enroll will reside inside the default registry generally.
This central object has a few tasks:
- Registering new stores.
- Providing get admission to to present stores.
- Managing subscriptions to state changes.
Whilst you don’t frequently have direct interaction with the registry, you do wish to understand its place in how the information package deal deal orchestrates state keep an eye on right through WordPress.
Basic interaction with WordPress wisdom stores
If you happen to use ES2015+ JavaScript and also you may well be working with a WordPress plugin or theme, you’ll have the ability to include it as a dependency:
npm arrange @wordpress/wisdom --save
Within your code, you’ll import the essential functions from the package deal deal at the best of the document:
import { make a selection, dispatch, subscribe } from '@wordpress/wisdom';
Interacting with present WordPress stores requires that you simply use one of the functions you import. Getting access to state wisdom with make a selection
, as an example:
const posts = make a selection('core').getPosts();
It’s the identical for dispatching actions:
dispatch('core').savePost(postData);
Subscribing to state changes uses a quite different construction, on the other hand the idea that that is the same:
subscribe(() => {
const newPosts = make a selection('core').getPosts();
// Exchange your UI in keeping with the new posts
});
Alternatively, you gained’t all the time art work with the default stores. Regularly, you’ll art work with present additional stores or enroll your own.
Discover ways to enroll a WordPress wisdom store
Defining your store’s configuration and registering it with the WordPress wisdom package deal deal starts with importing the enroll
function:
…
import { createReduxStore, enroll } from '@wordpress/wisdom';
…
This takes a single argument — your store descriptor. Next, you will have to define a default state for the store to set its default values:
…
const DEFAULT_STATE = {
todos: [],
};
…
Next, create an actions
object, define a reducer
function to handle state updates, and create a selectors
object with functions to get admission to state wisdom:
const actions = {
addTodo: (text) => ({
type: 'ADD_TODO',
text,
}),
};
const reducer = (state = DEFAULT_STATE, movement) => {
switch (movement.type) {
case 'ADD_TODO':
return {
...state,
todos: [...state.todos, { text: action.text, completed: false }],
};
default:
return state;
}
};
const selectors = {
getTodos: (state) => state.todos,
};
To create the store configuration, define it the usage of the createReduxStore
object. This will likely initialize the actions, selectors, keep an eye on, and other homes for your store:
const store = createReduxStore('my-plugin/todos', {
reducer,
actions,
selectors,
});
The minimum this object needs is a reducer to stipulate the type of the state and how it changes in line with other actions. In spite of everything, enroll the store, calling the store descriptor you define with createReduxStore
:
enroll(store);
You’ll have the ability to now interact along with your custom designed store as it’s essential others:
import { make a selection, dispatch } from '@wordpress/wisdom';
// Add a brand spanking new todo
dispatch('my-plugin/todos').addTodo('Be informed WordPress wisdom package deal deal');
// Get all todos
const todos = make a selection('my-plugin/todos').getTodos();
The necessary factor in the usage of the WordPress wisdom package deal deal is how you utilize the opposite homes and devices at your disposal.
Breaking down the 5 WordPress wisdom store homes
A large number of the usage of the WordPress wisdom package deal deal happens “backwards” — defining low-level wisdom store homes previous to the store itself. The createReduxStore
object is a perfect example, as it pulls in combination the entire definitions you make to create the descriptor you utilize to enroll a store:
import { createReduxStore } from '@wordpress/wisdom';
const store = createReduxStore( 'demo', {
reducer: ( state = 'OK' ) => state,
selectors: {
getValue: ( state ) => state,
},
} );
The ones other homes moreover need setup and configuration.
1. Actions
Actions are the primary approach to purpose state changes for your store. They’re easy JavaScript devices that describe what will have to happen. As such, it can be a good idea to create the ones first, as you’ll have the ability to decide what states you wish to have to retrieve.
const actions = {
addTodo: (text) => ({
type: 'ADD_TODO',
text,
}),
toggleTodo: (index) => ({
type: 'TOGGLE_TODO',
index,
}),
};
Motion creators take optional arguments and will return an object to move along to the reducer you define:
const actions = {
updateStockPrice: (symbol, newPrice) => {
return {
type: 'UPDATE_STOCK_PRICE',
symbol,
newPrice
};
},
If you happen to pass in a store descriptor, you’ll have the ability to dispatch movement creators and change the state value:
dispatch('my-plugin/todos').updateStockPrice('¥', '150.37');
Consider movement devices as instructions for the reducer on tips on how to make state changes. At a minimum, you’ll in all probability need to define create, change, be told, and delete (CRUD) actions. It’s also that you just’ve a separate JavaScript document for movement types and create an object for the entire ones types, in particular in the event you define them as constants.
2. Reducer
It’s value talking regarding the reducer proper right here, on account of its central place alongside actions. Its job is to specify how the state will have to trade in line with the instructions it is going to get from an movement. If you happen to pass it the instructions from the movement and the prevailing state, it is going to perhaps return a brand spanking new state object and pass it along the chain:
const reducer = (state = DEFAULT_STATE, movement) => {
switch (movement.type) {
case 'ADD_TODO':
return {
...state,
todos: [...state.todos, { text: action.text, completed: false }],
};
case 'TOGGLE_TODO':
return {
...state,
todos: state.todos.map((todo, index) =>
index === movement.index ? { ...todo, completed: !todo.completed } : todo
),
};
default:
return state;
}
};
Realize {{that a}} reducer must be a natural serve as, and it shouldn’t mutate the state it accepts (moderately, it will have to return it with updates). Reducers and actions have a symbiotic relationship in a variety of respects, so grasping how they art work together is very important.
3. Selectors
So as to get admission to the prevailing state from a registered store, you need selectors. It’s the primary means you “expose” your store state, and they lend a hand keep your portions decoupled from the store’s inside of building:
const selectors = {
getTodos: (state) => state.todos,
getTodoCount: (state) => state.todos.length,
};
You’ll have the ability to identify those selectors with the make a selection
function:
const todoCount = make a selection('my-plugin/todos').getTodoCount();
Alternatively, a selector doesn’t send that wisdom anyplace: it simply finds it and gives get admission to.
Selectors can download as many arguments as you need to get admission to the state with accuracy. The fee it returns is the result of regardless of those arguments achieve right through the selector you define. As with actions, you can make a option to have a separate document to hold all of your selectors, as there could be numerous them.
4. Controls
Guiding the execution go with the flow of your internet web page’s capacity — or executing commonplace sense within it — is where you use controls. The ones define the conduct of execution flows for your actions. Consider the ones the assistants inside the WordPress wisdom package deal deal as they art work as go-betweens to gather the state to visit resolvers.
Controls moreover handle negative effects for your store, similar to API calls or interactions with browser APIs. They imply you’ll keep reducers clean while however enabling you to handle complex async operations:
const controls = {
FETCH_TODOS: async () => {
const response = look ahead to fetch('/api/todos');
return response.json();
},
};
const actions = {
fetchTodos: () => ({ type: 'FETCH_TODOS' }),
};
This cycle of fetching and returning wisdom is a very powerful to the entire process. Then again without a identify from an movement, you gained’t be capable to use that wisdom.
5. Resolvers
Selectors expose a store’s state, on the other hand don’t explicitly send that wisdom anyplace. Resolvers meet selectors (and controls) to retrieve the information. Like controls, they are able to moreover handle asynchronous wisdom fetching.
const resolvers = {
getTodos: async () => {
const todos = look ahead to controls.FETCH_TODOS();
return actions.receiveTodos(todos);
},
};
The resolver promises that the information you ask for is available inside the store previous to working a selector. This tight connectivity between the resolver and the selector approach they wish to are compatible names. This is so the WordPress wisdom package deal deal can understand which resolver to invoke in keeping with the information you request.
What’s further, the resolver will all the time download the identical arguments that you simply pass proper right into a selector function, and will also each return, yield, or dispatch movement devices.
Error coping with when the usage of the WordPress wisdom package deal deal
You’ll have to implement proper error dealing with when working with the WordPress wisdom package deal deal. If you choose to deal with asynchronous operations, art work with complete stack deployments, or make API calls, it’s a lot more important.
For example, in the event you dispatch actions that comprise asynchronous operations, a try-catch block could be a excellent chance:
const StockUpdater = () => {
// Get the dispatch function
const { updateStock, setError, clearError } = useDispatch('my-app/stocks');
const handleUpdateStock = async (stockId, newData) => {
strive {
// Clear any present errors
clearError();
// Attempt to change the stock
look ahead to updateStock(stockId, newData);
} catch (error) {
// Dispatch an error movement if something goes improper
setError(error.message);
}
};
return (
);
};
For reducers, you’ll have the ability to handle error actions and change the state:
const reducer = (state = DEFAULT_STATE, movement) => {
switch (movement.type) {
// ... other instances
case 'FETCH_TODOS_ERROR':
return {
...state,
error: movement.error,
isLoading: false,
};
default:
return state;
}
};
When the usage of selectors, you’ll have the ability to include error checking to handle conceivable issues then check for errors for your portions previous to you utilize the information.:
const MyComponent = () => {
// Get a few pieces of state along with error wisdom
const { wisdom, isLoading, error } = useSelect((make a selection) => ({
wisdom: make a selection('my-app/stocks').getStockData(),
isLoading: make a selection('my-app/stocks').isLoading(),
error: make a selection('my-app/stocks').getError()
}));
// Care for different states
if (isLoading) {
return Loading...;
}
if (error) {
return (
Error loading stocks: {error.message}
);
}
return (
{/* Your same old section render */}
);
};
The useSelect
and useDispatch
functions come up with numerous power to handle errors right through the WordPress wisdom package deal deal. With both a kind of, you’ll have the ability to pass in custom designed error messages as arguments.
It’s excellent follow to make sure you centralize your error state all the way through initial configuration, and to stick error boundaries at the section level. The usage of error coping with for loading states may also lend a hand to stick your code clear and loyal.
Discover ways to mix your WordPress wisdom store along with your internet web page
There’s such a lot that the WordPress wisdom package deal deal can do that can assist you prepare state. Putting all of this together is also a sensible consideration. Let’s check out a stock ticker that shows and updates financial wisdom in real-time.
The main job is to create a store for your wisdom:
import { createReduxStore, enroll } from '@wordpress/wisdom';
const DEFAULT_STATE = {
stocks: [],
isLoading: false,
error: null,
};
const actions = {
fetchStocks: () => async ({ dispatch }) => {
dispatch({ type: 'FETCH_STOCKS_START' });
strive {
const response = look ahead to fetch('/api/stocks');
const stocks = look ahead to response.json();
dispatch({ type: 'RECEIVE_STOCKS', stocks });
} catch (error) {
dispatch({ type: 'FETCH_STOCKS_ERROR', error: error.message });
}
},
};
const reducer = (state = DEFAULT_STATE, movement) => {
switch (movement.type) {
case 'FETCH_STOCKS_START':
return { ...state, isLoading: true, error: null };
case 'RECEIVE_STOCKS':
return { ...state, stocks: movement.stocks, isLoading: false };
case 'FETCH_STOCKS_ERROR':
return { ...state, error: movement.error, isLoading: false };
default:
return state;
}
};
const selectors = {
getStocks: (state) => state.stocks,
getStocksError: (state) => state.error,
isStocksLoading: (state) => state.isLoading,
};
const store = createReduxStore('my-investing-app/stocks', {
reducer,
actions,
selectors,
});
enroll(store);
This process gadgets up a default state that includes error and loading states, along with your actions, reducers, and selectors. When you define the ones, you’ll have the ability to enroll the store.
Display the store’s wisdom
With a store in place, you’ll have the ability to create a React part to turn the information within it:
import { useSelect, useDispatch } from '@wordpress/wisdom';
import { useEffect } from '@wordpress/part';
const StockTicker = () => {
const stocks = useSelect((make a selection) => make a selection('my-investing-app/stocks').getStocks());
const error = useSelect((make a selection) => make a selection('my-investing-app/stocks').getStocksError());
const isLoading = useSelect((make a selection) => make a selection('my-investing-app/stocks').isStocksLoading());
const { fetchStocks } = useDispatch('my-investing-app/stocks');
useEffect(() => {
fetchStocks();
}, []);
if (isLoading) {
return Loading stock wisdom...
;
}
if (error) {
return Error: {error}
;
}
return (
Stock Ticker
{stocks.map((stock) => (
-
{stock.symbol}: ${stock.price}
))}
);
};
This section brings inside the useSelect
and useDispatch
hooks (along with others) to handle wisdom get admission to, dispatching actions, and section lifecycle keep an eye on. It moreover gadgets custom designed error and loading state messages, along with some code to if truth be told display the ticker. With this in place, you at the moment will have to enroll the section with WordPress.
Registering the section with WordPress
Without registration within WordPress, you gained’t be capable to use the portions you create. This means registering it as a Block, although it may be a widget in the event you design for Vintage Issues. This case uses a Block.
import { registerBlockType } from '@wordpress/blocks';
import { StockTicker } from './portions/StockTicker';
registerBlockType('my-investing-app/stock-ticker', {
determine: 'Stock Ticker',
icon: 'chart-line',
magnificence: 'widgets',
edit: StockTicker,
save: () => null, // This will likely render dynamically
});
This process will follow the usual approach you’ll take to enroll Blocks within WordPress, and doesn’t require any specific implementation or setup.
Managing state updates and individual interactions
When you enroll the Block, it’s necessary to handle individual interactions and real-time updates. This will likely need some interactive controls, along with custom designed HTML and JavaScript:
const StockControls = () => {
const { addToWatchlist, removeFromWatchlist } = useDispatch('my-investing-app/stocks');
return (
);
};
For real-time updates, you’ll have the ability to prepare an length right through the React section:
useEffect(() => {
const { updateStockPrice } = dispatch('my-investing-app/stocks');
const length = setInterval(() => {
stocks.forEach(stock => {
fetchStockPrice(stock.symbol)
.then(price => updateStockPrice(stock.symbol, price));
});
}, 60000);
return () => clearInterval(length);
}, [stocks]);
This implies keeps your section’s wisdom in sync along with your store while maintaining a clear separation of problems. The WordPress wisdom package deal deal will handle all state updates, which provides your app consistency.
Server-side rendering
In spite of everything, you’ll have the ability to prepare server-side rendering to make sure that the stock wisdom is provide upon internet web page load. This requires some PHP wisdom:
function my_investing_app_render_stock_ticker($attributes, $content material subject material) {
// Fetch the most recent stock wisdom from your API
$stocks = fetch_latest_stock_data();
ob_start();
?>
Stock Ticker
- : $
'my_investing_app_render_stock_ticker'
));
This implies provides a complete integration of your wisdom store with WordPress, coping with the whole lot from initial render to real-time updates and individual interactions.
Summary
The WordPress wisdom package deal deal is a complicated however tricky approach to prepare application states for your projects. Previous the necessary factor concepts lies a rabbit hole of functions, operators, arguments, and further. Consider, even though, that not each and every piece of information will have to be in a world store — the local section state however has a place for your code.
Do you see yourself the usage of the WordPress wisdom package deal deal incessantly, or do you will have another way of managing state? Percentage your evaluations with us inside the comments beneath.
The publish Learn how to use the WordPress knowledge package deal to control utility states seemed first on Kinsta®.
Contents
- 1 Introducing the WordPress wisdom package deal deal
- 2 Key concepts and terminology for the WordPress wisdom package deal deal
- 3 Stores: the central hub of the WordPress wisdom package deal deal
- 4 Discover ways to enroll a WordPress wisdom store
- 5 Breaking down the 5 WordPress wisdom store homes
- 6 Error coping with when the usage of the WordPress wisdom package deal deal
- 7 Discover ways to mix your WordPress wisdom store along with your internet web page
- 8 Stock Ticker
- 9 Stock Ticker
- 10 Summary
- 11 9 Best AI Content Detectors in 2023 (Future Proof Your Content)
- 12 The best way to Create a Customized Fb Feed in WordPress
- 13 101 Maximum Winning Weblog Niches & Discovering One That Works for You
0 Comments