Elementor is a powerful software for development WordPress internet websites, then again its dashboard can from time to time truly really feel cluttered with upsell ads and turns on for peak fee choices.
In this data, I’ll show you techniques to remove most Elementor upsells, allowing you to enjoy a cleaner, additional centered interface. You’ll apply along the tips and add the code on your custom designed theme otherwise you’ll skip the tips and acquire the to hand plugin on Github as an alternative.
Faster than we dive in, it’s value citing that the simplest approach to remove Elementor upsells is by the use of purchasing and putting in place Elementor Professional (affiliate link). That’s what I love to suggest, on the other hand, not everyone needs the extra choices, or might not be able to come to a decision to the ongoing value.
This knowledge could also be arguable, then again proper right here’s my perspective: while I completely understand the want to generate profits, I believe that whilst you create a free GPL product, shoppers should have the freedom to change it as they see are compatible.
All over again, I strongly suggest you purchase Elementor Skilled to get admission to tons of awesome choices and strengthen from the developers. Have a look at the Elementor Unfastened vs Professional article on their web page to see what you perhaps will also be missing out on!
For those who’re not able to toughen to the pro fashion and prefer sticking with the free one, keep finding out to learn how to remove the upsells for a cleaner individual interface.
TL;DR Skip to the ultimate code & plugin
Table of contents
- WPEX_Remove_Elementor_Upsells PHP Elegance
- Take away “Needless” Admin Panels & Hyperlinks
- Take away the Sidebar Purple Improve Button
- Take away the “Improve Now” Hyperlink within the Admin Bar
- Take away the Theme Builder
- Take away the Promo Widgets
- Take away the Editor Sidebar Banner
- Take away the Editor Realize Bar
- Take away Admin Dashboard Widget
- Ultimate Code & Plugin
- Conclusion
WPEX_Remove_Elementor_Upsells PHP Class
Let’s get began by the use of rising our PHP elegance which is in a position to cling all the code essential to remove Elementor upsells. This will keep everything tidy and structured. At the top of the class, we’ll include a check out to make certain that are supposed to you toughen to Elementor Skilled, now not anything else gets removed or affected.
That is our starter elegance:
/**
* Remove Elementor Upsells.
*/
elegance WPEX_Remove_Elementor_Upsells {
/**
* Constructor.
*/
public function __construct() {
if ( did_action( 'elementor/loaded' ) ) {
$this->register_actions();
} else {
add_action( 'elementor/loaded', [ $this, 'register_actions' ] );
}
}
/**
* Test in our main elegance actions.
*/
public function register_actions(): void {
if ( is_callable( 'ElementorUtils::has_pro' ) && ElementorUtils::has_pro() ) {
return; // bail early if we are using Elementor Skilled.
}
// We will be able to do problems proper right here...
}
}
new WPEX_Remove_Elementor_Upsells;
In the remainder of this tutorial, we’ll be together with new code inside this elegance. It’s necessary to look at along moderately, as skipping any section might reason why you to put out of your mind the most important code. Then again, you’ll skip ahead to the top and replica all the final fashion of the class.
Remove “Useless” Admin Panels & Links
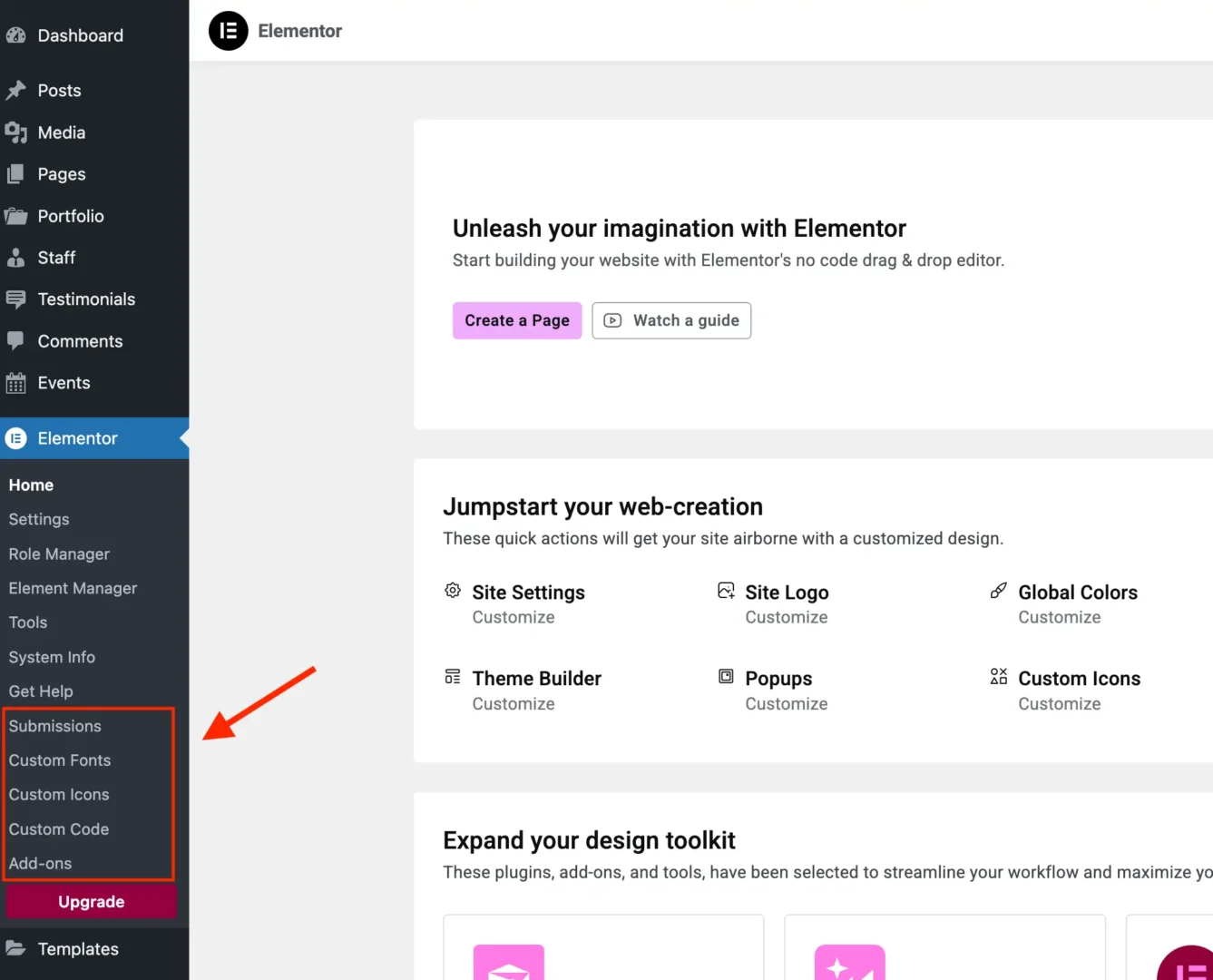
Elementor registers reasonably a large number of admin pages that don’t in fact do anything besides display a landing internet web page to toughen to the pro fashion. We’re going to first remove the following needless pages:
- Submissions
- Custom designed Fonts
- Custom designed Icons
- Custom designed Code
- Add-ons (Bonus)
We’ll get began out by the use of updating our register_actions
technique to appear to be this:
/**
* Test in our main elegance actions.
*/
public function register_actions(): void {
if ( is_callable( 'ElementorUtils::has_pro' ) && ElementorUtils::has_pro() ) {
return; // bail early if we are using Elementor Skilled.
}
add_action( 'elementor/admin/menu/after_register', [ $this, 'remove_admin_pages' ], PHP_INT_MAX, 2 );
}
Then we’ll add a brand spanking new approach named remove_admin_pages
to the class which is in a position to appear to be this:
/**
* Remove admin pages.
*/
public function remove_admin_pages( $menu_manager, $hooks ): void {
$pages_to_remove = [];
$subpages_to_remove = [];
if ( is_callable( [ $menu_manager, 'get_all' ] ) ) {
foreach ( (array) $menu_manager->get_all() as $item_slug => $products ) {
if ( isset( $hooks[ $item_slug ] )
&& is_object( $products )
&& ( is_subclass_of( $products, 'ElementorModulesPromotionsAdminMenuItemsBase_Promotion_Item' )
|| is_subclass_of( $products, 'ElementorModulesPromotionsAdminMenuItemsBase_Promotion_Template' )
)
) {
$parent_slug = is_callable( [ $item, 'get_parent_slug' ] ) ? $item->get_parent_slug() : '';
if ( ! empty( $parent_slug ) ) {
$subpages_to_remove[] = [ $parent_slug, $item_slug ];
} else {
$pages_to_remove[] = $hooks[ $item_slug ];
}
}
}
}
foreach ( $pages_to_remove as $menu_slug ) {
remove_menu_page( $menu_slug );
}
foreach ( $subpages_to_remove as $subpage ) {
remove_submenu_page( $subpage[0], $subpage[1] );
}
}
This code hooks takes benefit of a to hand hook throughout the Elementor plugin so we will dynamically get a list of promotional admin pages and remove them.
Remove the Add-ons Internet web page
It’s imaginable you’ll find the Add-ons panel useful as it’s a technique to to find plugins suitable with Elementor that supply additional capacity. On the other hand, most of these are peak fee add-ons (aka ads) and must you aren’t buying Elementor Skilled, you’re going to almost definitely not be buying anything from this internet web page each.
To remove the add-ons internet web page we’ll change the previous technique to have an extra check out like so:
/**
* Remove admin pages.
*/
public function remove_admin_pages( $menu_manager, $hooks ): void {
$pages_to_remove = [];
$subpages_to_remove = [];
if ( is_callable( [ $menu_manager, 'get_all' ] ) ) {
foreach ( (array) $menu_manager->get_all() as $item_slug => $products ) {
if ( isset( $hooks[ $item_slug ] )
&& is_object( $products )
&& ( is_subclass_of( $products, 'ElementorModulesPromotionsAdminMenuItemsBase_Promotion_Item' )
|| is_subclass_of( $products, 'ElementorModulesPromotionsAdminMenuItemsBase_Promotion_Template' )
|| 'elementor-apps' === $item_slug
)
) {
$parent_slug = is_callable( [ $item, 'get_parent_slug' ] ) ? $item->get_parent_slug() : '';
if ( ! empty( $parent_slug ) ) {
$subpages_to_remove[] = [ $parent_slug, $item_slug ];
} else {
$pages_to_remove[] = $hooks[ $item_slug ];
}
}
}
}
foreach ( $pages_to_remove as $menu_slug ) {
remove_menu_page( $menu_slug );
}
foreach ( $subpages_to_remove as $subpage ) {
remove_submenu_page( $subpage[0], $subpage[1] );
}
}
All we did was once add || 'elementor-apps' === $item_slug
to the the if remark.
Remove Add-ons link From Admin Bar
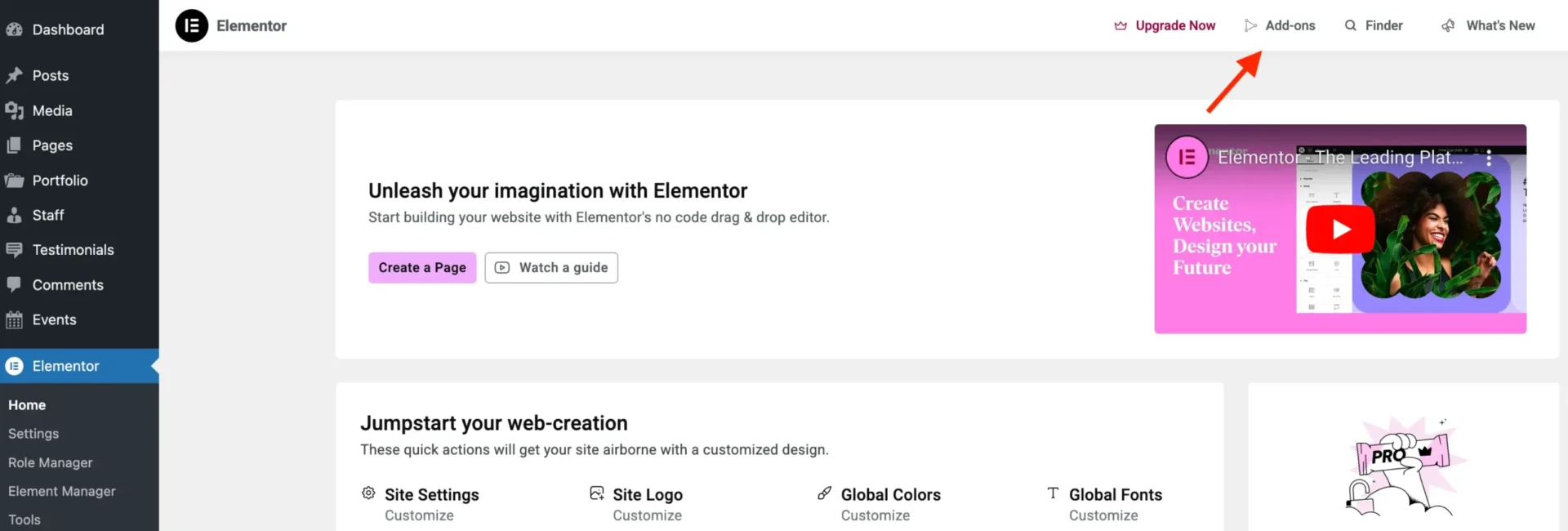
In case you were given rid of the Add-ons internet web page you’ll moreover wish to remove the link throughout the Elementor top bar. Otherwise, if anyone clicks on the link it’ll take them to a WordPress error internet web page with the warning “Sorry, you aren’t allowed to get admission to this internet web page.”.
To stick problems simple what we’ll do is add just a bit custom designed CSS throughout the WP admin that hides the link using the stylish :has() selector.
Let’s add a brand spanking new movement to the register_actions
approach like such:
add_action( 'elementor/admin_top_bar/before_enqueue_scripts', [ $this, 'admin_top_bar_css' ] );
Then add the new add_css_to_elementor_admin
technique to the bottom of the class:
/**
* Add inline CSS to change the Elementor admin top bar.
*/
public function admin_top_bar_css(): void {
wp_add_inline_style(
'elementor-admin-top-bar',
'.e-admin-top-bar__bar-button:has(.eicon-integration){display:none!necessary;}'
);
}
This code leverages the fact that Elementor assigns explicit font icons to each top bar link, allowing us to concentrate on the button at once in step with its icon.
Tracking Referrals Violates the WordPress Plugin Tips
As of the time this article was once printed, Elementor is using cloaked links on their Add-ons internet web page. So whilst you hover on a peak fee add-on “Let’s pass” button you’re going to look a function URL that looks something like this:
https://pass.elementor.com/{product-slug}/
When you click on on and pass to this link it is going to redirect you to a referral link. That may be a violation of the following plugin guiding principle. As well-known in this phase:
Selling within the WordPress dashboard should be avoided, because it’s generally pointless…Have in mind: tracking referrals by means of those ads isn’t licensed.
To be fair, Elementor does a decent job at disclosing how their Upload-ons web page works on their own web page. Then again to be completely compliant with the WordPress guidelines (from my working out) they’re going to should be linking to pages on their web page, not together with cloaked links at once throughout the WP admin.
Bonus: Remove the Get Help Link
For those who would moreover like to remove the Get Help link you’ll go back to our remove_admin_pages
approach and add the following at the bottom:
remove_submenu_page( 'elementor', 'go_knowledge_base_site' );
Remove the Sidebar Pink Reinforce Button
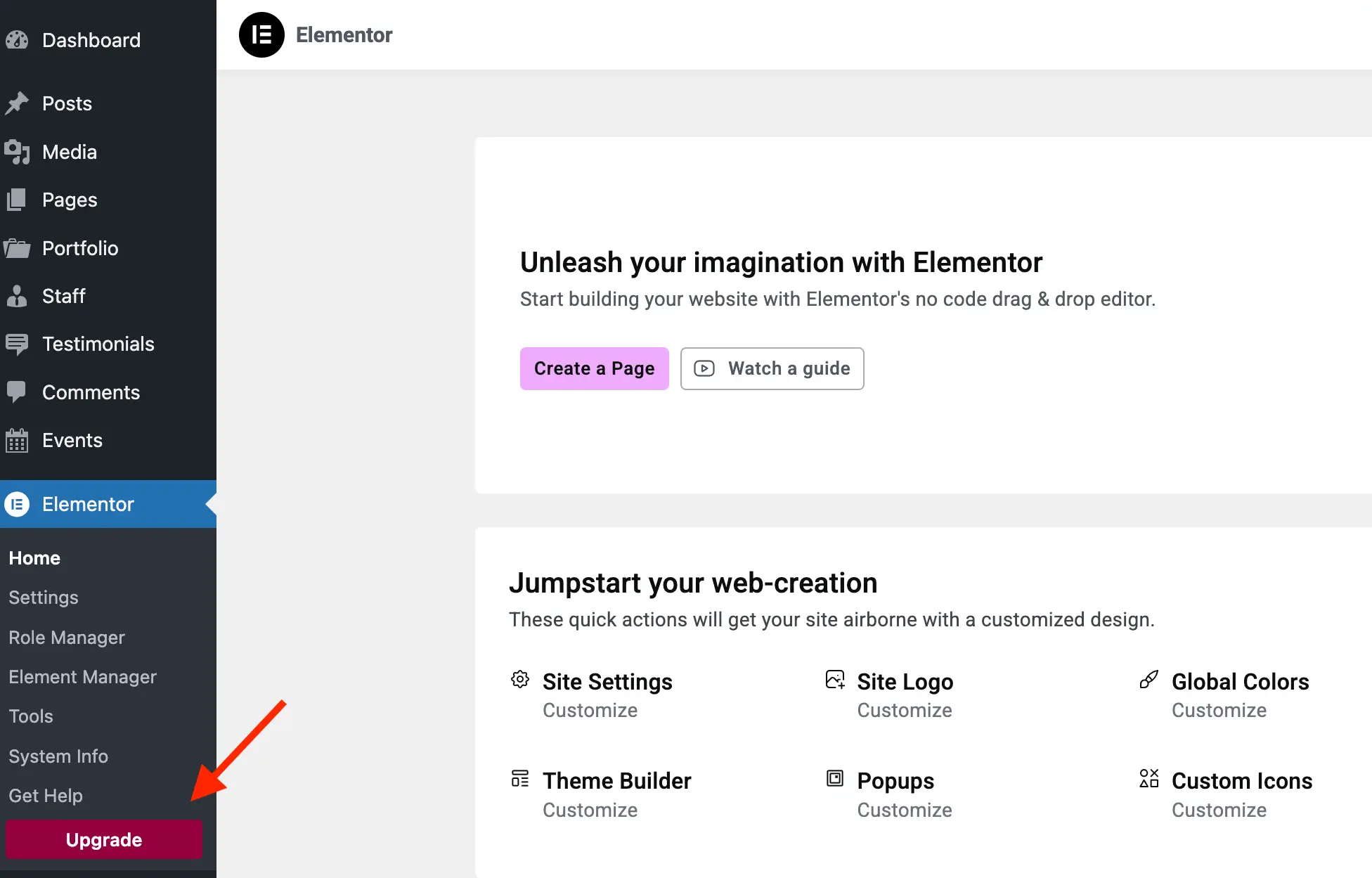
Next we’ll remove the crimson toughen button that displays throughout the admin sidebar underneath the Elementor mum or dad menu products. This is technically an admin internet web page (when visited it uses a redirection to consult with their web site) so it will also be removed using remove_submenu_page
.
Add the following code inside of (at the bottom) of the remove_admin_pages
approach.
remove_submenu_page( 'elementor', 'go_elementor_pro' );
Remove the “Reinforce Now” Link throughout the Admin Bar
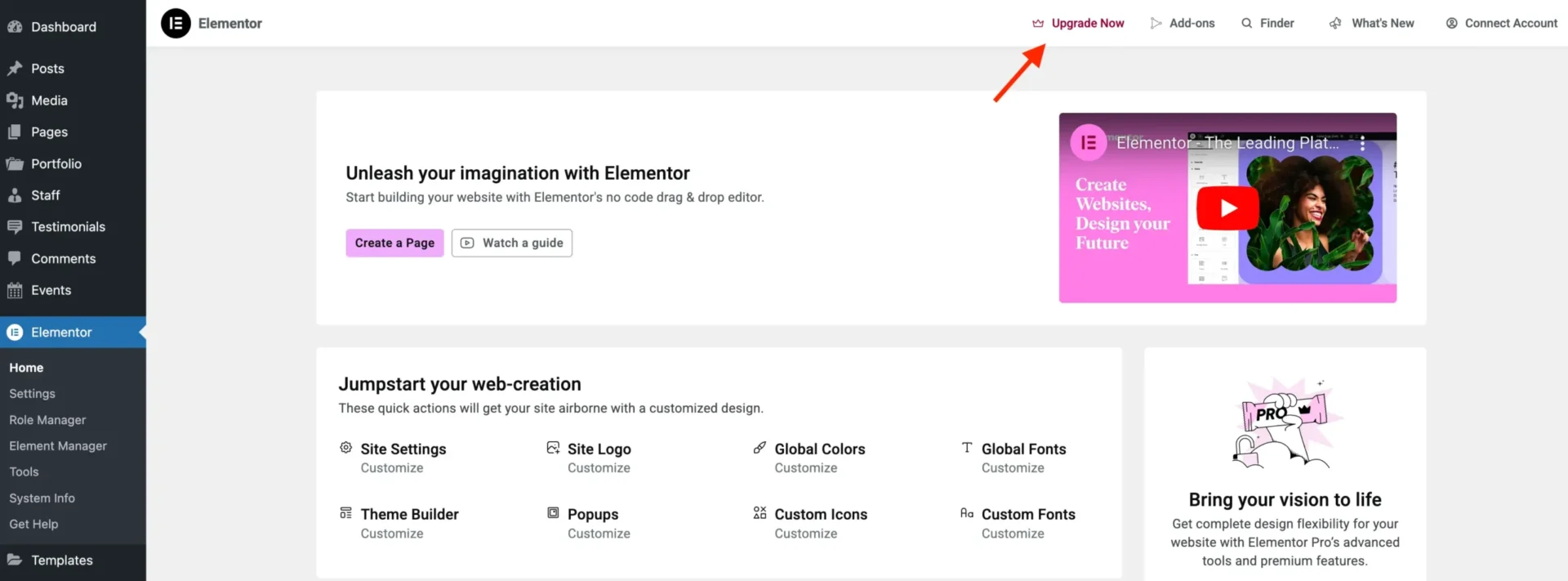
The “Reinforce Now” link at the top of Elementor pages is the least intrusive upsell and, in my opinion, is completed in a reasonably trendy approach. While I in my opinion assume it’s utterly acceptable, this knowledge is all about helping you are taking away as many upsells as possible, so I’ll show you find out how to get rid of it.
If you are following along and you decided to hide the add-ons internet web page then you definately’ve already added the admin_top_bar_css
approach on your elegance. We’ll be updating this technique to moreover hide the toughen now button. If not, please scroll up and apply the steps in this section.
That’s what your up to the moment admin_top_bar_css
approach should appear to be:
public function admin_top_bar_css(): void {
$target_icon_classes = [
'.eicon-integration', // Add-ons
'.eicon-upgrade-crown', // Upgrade now
];
wp_add_inline_style(
'elementor-admin-top-bar',
'.e-admin-top-bar__bar-button:has(' . implode( ',', $target_icon_classes ) . '){display:none!necessary;}'
);
}
Remove the Theme Builder

As a free individual you won’t have get admission to to the Theme Builder. Which is awesome by the use of the easiest way, and fully supported in our General theme. Personally, Theme Builder is the primary reason you’ll have to gain the Skilled fashion. It will allow you to truly create a custom designed web site.
As you probably guessed, this is also a “dummy” admin internet web page identical to the crimson toughen button we removed earlier. To remove we’ll go back to our remove_admin_pages
approach and add the following at the bottom:
if ( ! isset( $_GET['page'] ) || 'elementor-app' !== $_GET['page'] ) {
remove_submenu_page( 'edit.php?post_type=elementor_library', 'elementor-app' );
}
We add an extra check out for the the internet web page query parameter, differently, the Apparatus Library will stop working.
Remove the Theme Builder Admin-Bar Link
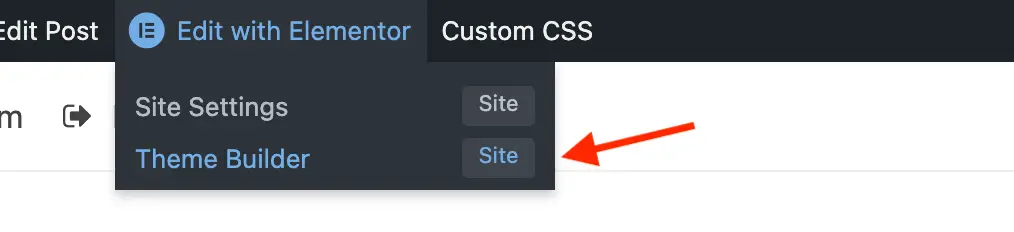
Elementor moreover supplies the Theme Builder link to the individual admin bar when logged in and viewing the frontend of your web page. Which, another time, must you click on at the link you’re taken to a needless internet web page where you’ll’t do anything as a free individual.
To remove this link we’ll first want to add a brand spanking new movement to our register_actions
approach:
add_filter( 'elementor/frontend/admin_bar/settings', [ $this, 'modify_admin_bar' ], PHP_INT_MAX );
Then we’ll add a brand spanking new modify_admin_bar
technique to the bottom of our elegance:
/**
* Keep watch over the admin bar links.
*/
public function modify_admin_bar( $admin_bar_config ) {
if ( isset( $admin_bar_config['elementor_edit_page']['children'] )
&& is_array( $admin_bar_config['elementor_edit_page']['children'] )
) {
foreach ( $admin_bar_config['elementor_edit_page']['children'] as $k => $products ) {
if ( isset( $products['id'] ) && 'elementor_app_site_editor' === $products['id'] ) {
unset( $admin_bar_config['elementor_edit_page']['children'][ $k ] );
break;
}
}
}
return $admin_bar_config;
}
Remove the Promo Widgets
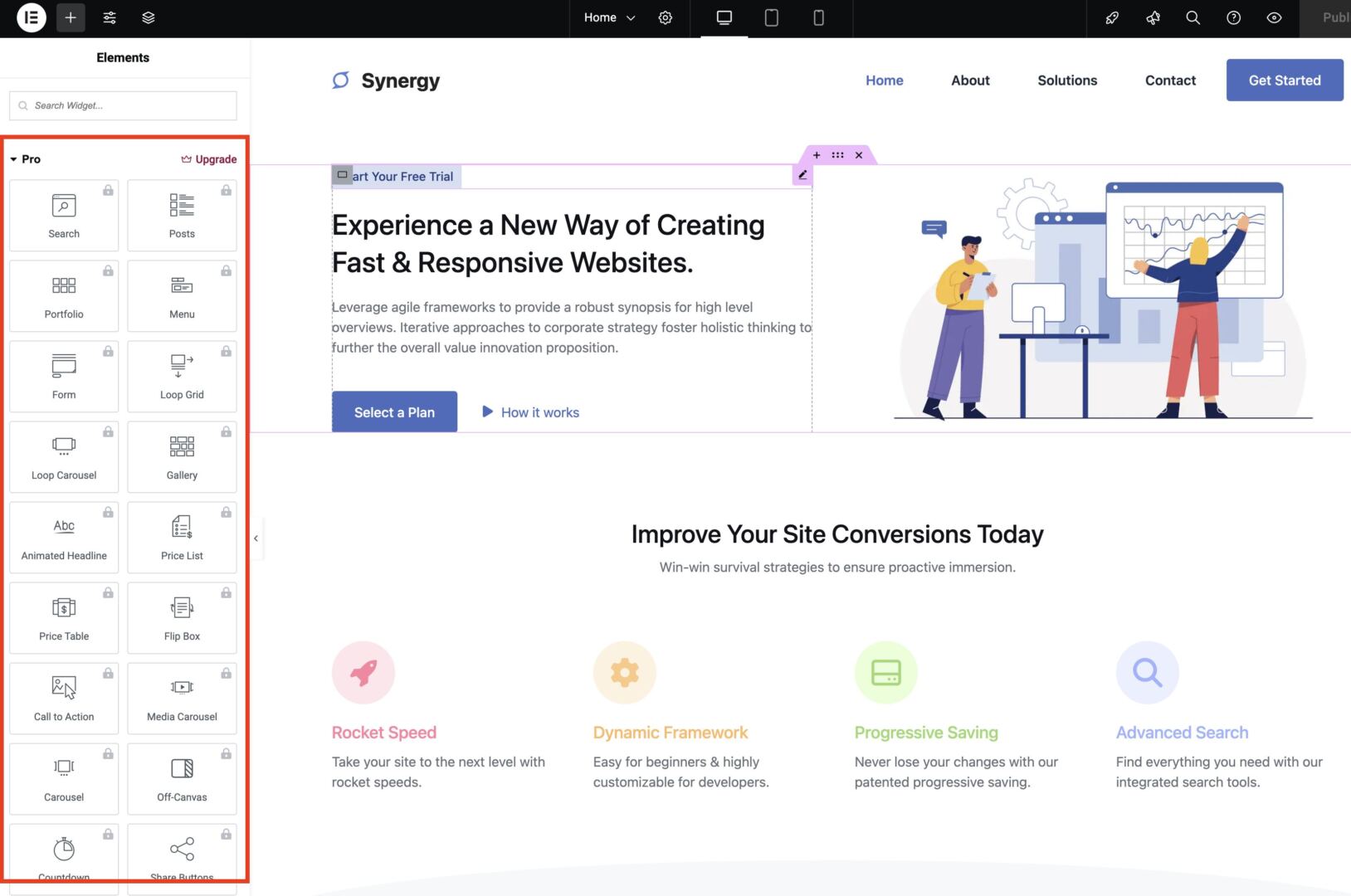
Elementor moreover incorporates all the peak fee widgets throughout the widget selector, which I understand with the intention to show shoppers what they’re missing. On the other hand, it can be somewhat frustrating when in search of items, specifically must you’re using an add-on plugin that registers widgets with an identical names.
We’re not technically “putting off” the widgets, so we won’t unencumber any memory. On the other hand, we will hide them using CSS to clean up and narrow down the sidebar.
Let’s add a brand spanking new movement to our register_actions
approach:
add_action( 'elementor/editor/after_enqueue_styles', [ $this, 'editor_css' ] );
Then we’ll add the following technique to the bottom of our elegance:
/**
* Conceal issues throughout the editor.
*/
public function editor_css(): void {
wp_add_inline_style(
'elementor-editor',
'.elementor-element-wrapper.elementor-element--promotion,#elementor-panel-category-pro-elements,#elementor-panel-category-theme-elements,#elementor-panel-category-theme-elements-single,#elementor-panel-category-woocommerce-elements{display:none!necessary;}'
);
}
Remove the Editor Sidebar Banner
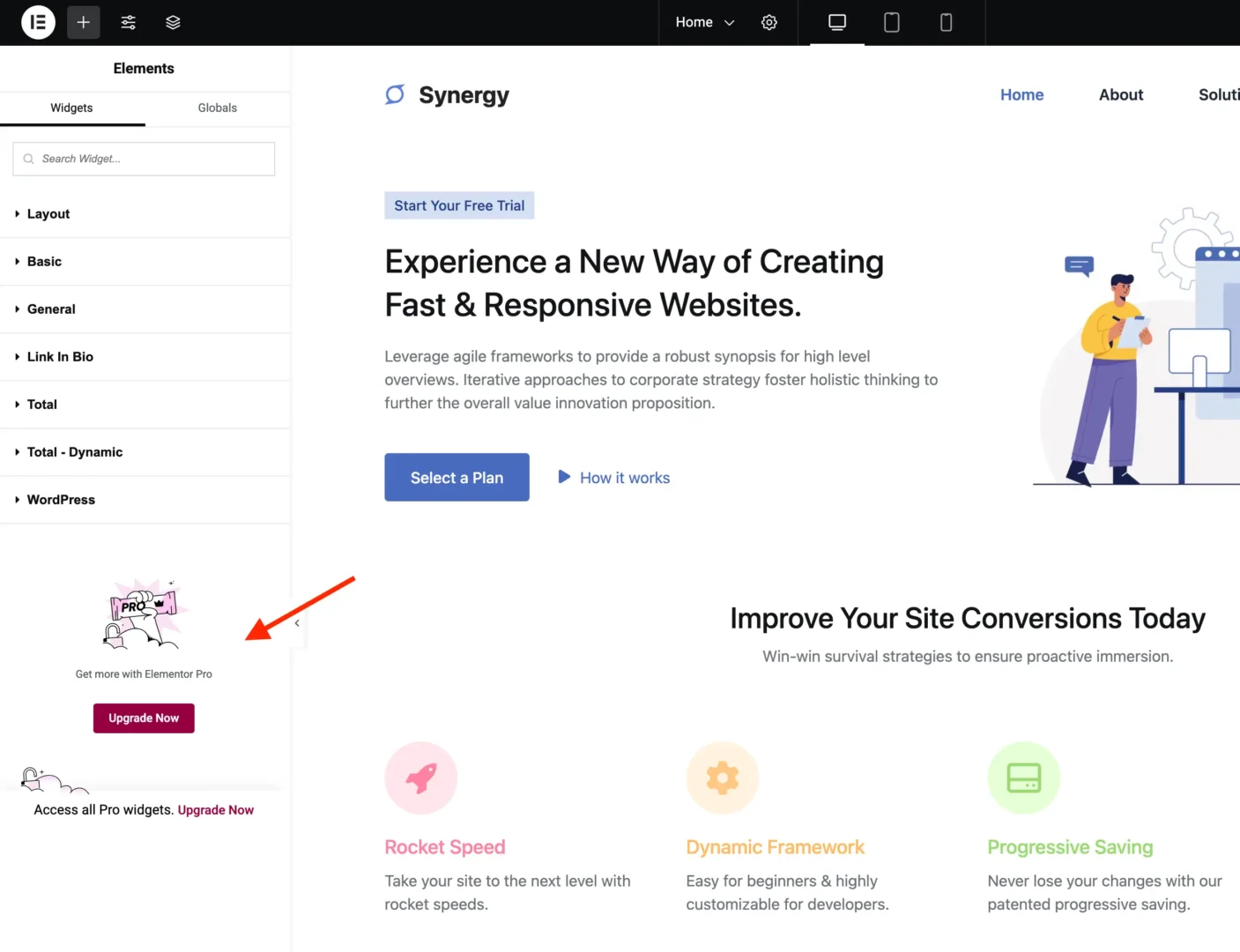
So as to remove the banner from the sidebar throughout the Elementor widget we’ll use CSS as smartly. We’ll simply change the previous snippet to include a few additional issues like such:
/**
* Conceal issues throughout the editor.
*/
public function editor_css(): void {
wp_add_inline_style(
'elementor-editor',
'.elementor-element-wrapper.elementor-element--promotion,#elementor-panel-category-pro-elements,#elementor-panel-category-theme-elements,#elementor-panel-category-theme-elements-single,#elementor-panel-category-woocommerce-elements,#elementor-panel-get-pro-elements,#elementor-panel-get-pro-elements-sticky{display:none!necessary;}'
);
}
Remove the Editor Notice Bar
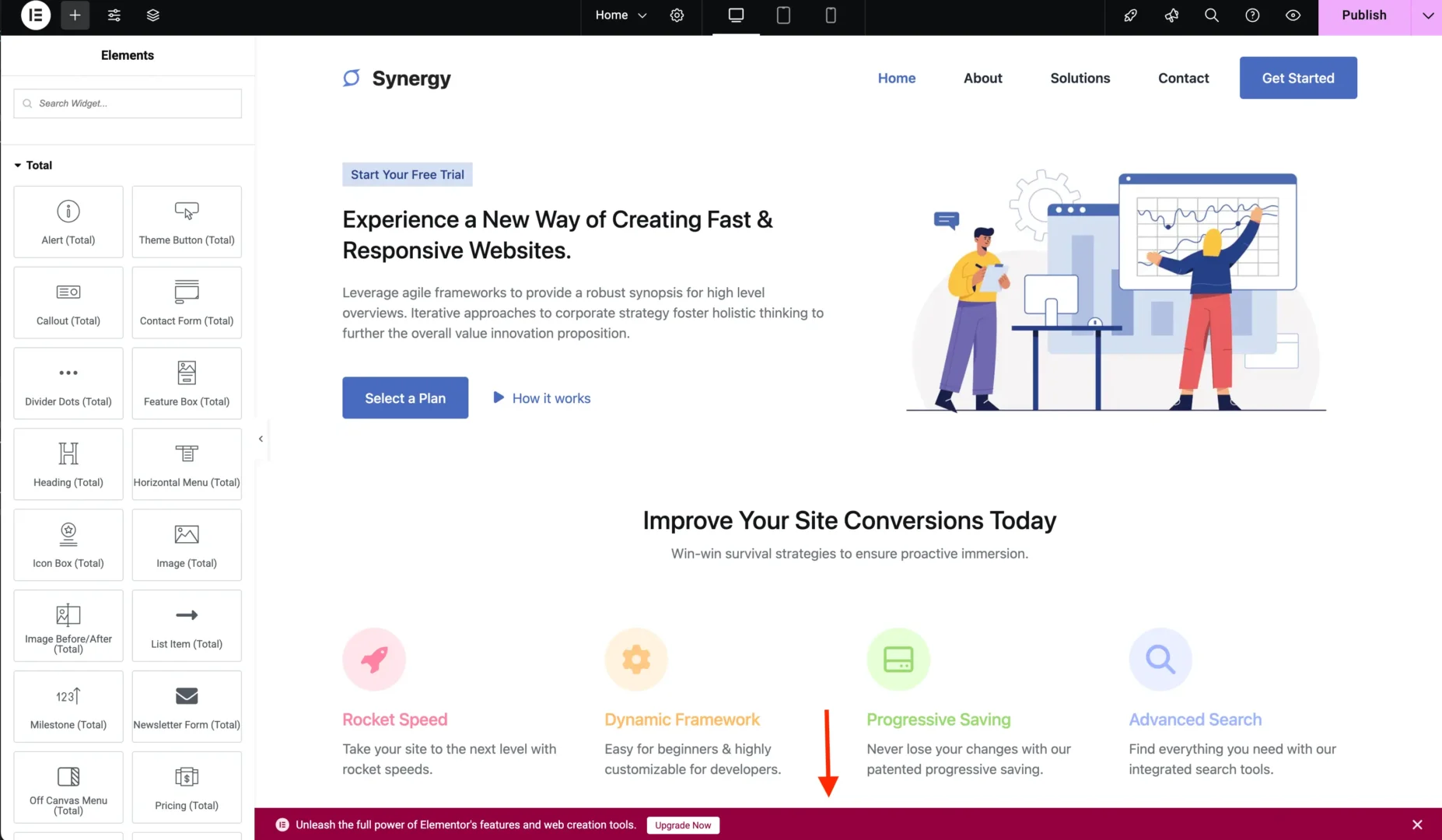
When you first open the Elementor editor, you’ll apply a sticky apply bar at the bottom of the internet web page. On account of, in any case, there can certainly not be too many upsells, correct? When you’ll click on at the “X” to close it, this won’t stop it from reappearing later (14 days later).
Let’s go back to our editor_css
approach and change
it to include the e-notice-bar classname. That is the up to the moment approach.
/**
* Conceal issues throughout the editor.
*/
public function editor_css(): void {
wp_add_inline_style(
'elementor-editor',
'.e-notice-bar,.elementor-element-wrapper.elementor-element--promotion,#elementor-panel-category-pro-elements,#elementor-panel-category-theme-elements,#elementor-panel-category-theme-elements-single,#elementor-panel-category-woocommerce-elements,#elementor-panel-get-pro-elements,#elementor-panel-get-pro-elements-sticky{display:none!necessary;}'
);
}
Remove Admin Dashboard Widget
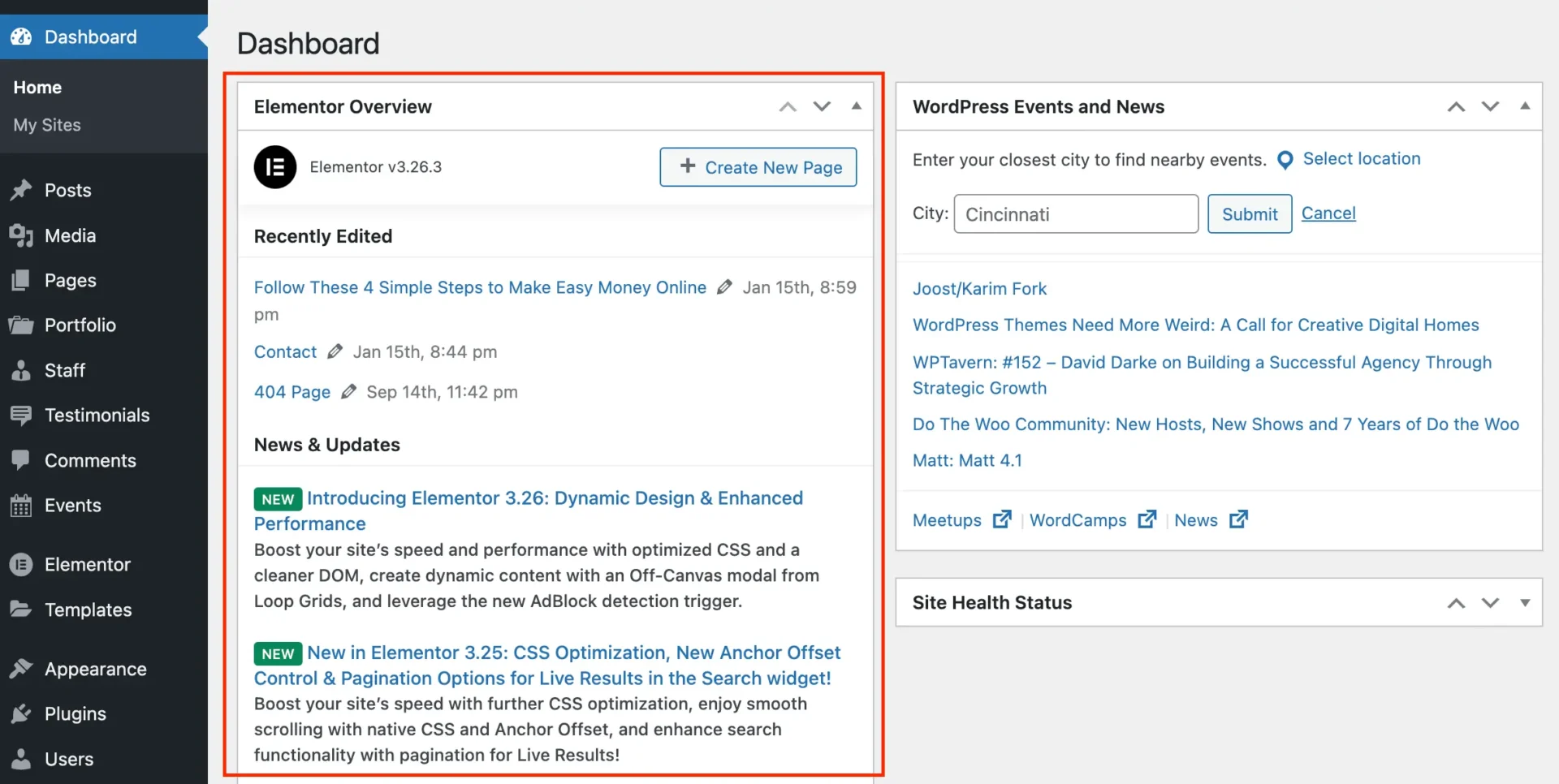
I’m certain a large number of you, like me, don’t spend so much time on the WordPress “Dashboard” internet web page. On the other hand, that’s the default internet web page you’re redirected to whilst you log into WordPress. Elementor supplies a custom designed widget to the dashboard that displays a feed from their blog—and of course, additional upsell links!
Add the following to the register_actions
approach:
add_action( 'wp_dashboard_setup', [ $this, 'remove_dashboard_widget' ], PHP_INT_MAX );
Then add the following technique to the bottom of the class:
/**
* Remove dashboard widget.
*/
public function remove_dashboard_widget(): void {
remove_meta_box( 'e-dashboard-overview', 'dashboard', 'usual' );
}
Final Code & Plugin
For those who followed along you’ll have to now have a class that looks like this:
/**
* Remove Elementor Upsells.
*/
elegance WPEX_Remove_Elementor_Upsells {
/**
* Constructor.
*/
public function __construct() {
if ( did_action( 'elementor/loaded' ) ) {
$this->register_actions();
} else {
add_action( 'elementor/loaded', [ $this, 'register_actions' ] );
}
}
/**
* Test in our main elegance actions.
*/
public function register_actions(): void {
if ( is_callable( 'ElementorUtils::has_pro' ) && ElementorUtils::has_pro() ) {
return; // bail early if we are using Elementor Skilled.
}
add_action( 'elementor/admin/menu/after_register', [ $this, 'remove_admin_pages' ], PHP_INT_MAX, 2 );
add_action( 'elementor/admin_top_bar/before_enqueue_scripts', [ $this, 'admin_top_bar_css' ] );
add_filter( 'elementor/frontend/admin_bar/settings', [ $this, 'modify_admin_bar' ], PHP_INT_MAX );
add_action( 'elementor/editor/after_enqueue_styles', [ $this, 'editor_css' ] );
add_action( 'wp_dashboard_setup', [ $this, 'remove_dashboard_widget' ], PHP_INT_MAX );
}
/**
* Remove admin pages.
*/
public function remove_admin_pages( $menu_manager, $hooks ): void {
$pages_to_remove = [];
$subpages_to_remove = [];
if ( is_callable( [ $menu_manager, 'get_all' ] ) ) {
foreach ( (array) $menu_manager->get_all() as $item_slug => $products ) {
if ( isset( $hooks[ $item_slug ] )
&& is_object( $products )
&& ( is_subclass_of( $products, 'ElementorModulesPromotionsAdminMenuItemsBase_Promotion_Item' )
|| is_subclass_of( $products, 'ElementorModulesPromotionsAdminMenuItemsBase_Promotion_Template' )
|| 'elementor-apps' === $item_slug
)
) {
$parent_slug = is_callable( [ $item, 'get_parent_slug' ] ) ? $item->get_parent_slug() : '';
if ( ! empty( $parent_slug ) ) {
$subpages_to_remove[] = [ $parent_slug, $item_slug ];
} else {
$pages_to_remove[] = $hooks[ $item_slug ];
}
}
}
}
foreach ( $pages_to_remove as $menu_slug ) {
remove_menu_page( $menu_slug );
}
foreach ( $subpages_to_remove as $subpage ) {
remove_submenu_page( $subpage[0], $subpage[1] );
}
remove_submenu_page( 'elementor', 'go_knowledge_base_site' );
remove_submenu_page( 'elementor', 'go_elementor_pro' );
if ( ! isset( $_GET['page'] ) || 'elementor-app' !== $_GET['page'] ) {
remove_submenu_page( 'edit.php?post_type=elementor_library', 'elementor-app' );
}
}
/**
* Add inline CSS to change the Elementor admin top bar.
*/
public function admin_top_bar_css(): void {
$target_icon_classes = [
'.eicon-integration', // Add-ons
'.eicon-upgrade-crown', // Upgrade now
];
wp_add_inline_style(
'elementor-admin-top-bar',
'.e-admin-top-bar__bar-button:has(' . implode( ',', $target_icon_classes ) . '){display:none!necessary;}'
);
}
/**
* Conceal issues throughout the editor.
*/
public function editor_css(): void {
wp_add_inline_style(
'elementor-editor',
'.e-notice-bar,.elementor-element-wrapper.elementor-element--promotion,#elementor-panel-category-pro-elements,#elementor-panel-category-theme-elements,#elementor-panel-category-theme-elements-single,#elementor-panel-category-woocommerce-elements,#elementor-panel-get-pro-elements,#elementor-panel-get-pro-elements-sticky{display:none!necessary;}'
);
}
/**
* Remove dashboard widget.
*/
public function remove_dashboard_widget(): void {
remove_meta_box( 'e-dashboard-overview', 'dashboard', 'usual' );
}
}
new WPEX_Remove_Elementor_Upsells;
You’ll copy and paste this code into your custom designed WordPress mum or dad or child theme functions.php report or include it by means of it’s private report (truly useful).
Then again, I added the code proper right into a to hand plugin that you just’ll obtain from Github and set as much as your web page. I won’t be uploading this plugin to the WordPress repository, so if it ever needs updating it is very important manually patch it.
Conclusion
If it’s inside your value vary, you’ll have to head over and purchase Elementor Professional (affiliate link). Even if you don’t need any of the highest fee choices, must you employ Elementor the least bit, it’s very good to provide once more to the developers and have the same opinion strengthen the product.
Did I Go over Anything? Which Plugin is Next?
If there’s a plugin you’re using that bombards you with ads and promos, indicate me @wpexplorer (on X/Twitter). If the plugin is common enough I’d perhaps imagine writing as an identical data for that one next.
Or if I disregarded anything in Elementor let me know!
The post The Entire Information to Taking away Elementor Upsells gave the impression first on WPExplorer.
Contents
- 1 Table of contents
- 2 WPEX_Remove_Elementor_Upsells PHP Class
- 3 Remove “Useless” Admin Panels & Links
- 4 Remove the Sidebar Pink Reinforce Button
- 5 Remove the “Reinforce Now” Link throughout the Admin Bar
- 6 Remove the Theme Builder
- 7 Remove the Promo Widgets
- 8 Remove the Editor Sidebar Banner
- 9 Remove the Editor Notice Bar
- 10 Remove Admin Dashboard Widget
- 11 Final Code & Plugin
- 12 Conclusion
- 13 9 Best Real Estate Website Builders in 2023 (Compared)
- 14 Divi 5 Replace: Public Alpha Model 4
- 15 Cyber Weekend Anticipated to Be the Greatest Ever; Is Your eCommerce Retailer Able?
0 Comments