Plugins play crucial place in customizing and embellishing your WordPress web sites. They’re used with the intention to upload capacity like touch bureaucracy, ecommerce, and analytics for your web sites and not using a coding required.
Like WordPress, which receives commonplace updates, plugins moreover download commonplace updates with the intention to upload new choices, patch a security hole, increase compatibility, and further. That’s why Kinsta built-in Plugin and Theme Control among the tools available within MyKinsta for each of your web sites.
Alternatively, updating plugins all over many web sites can nevertheless be daunting for busy customers like companies. This text demonstrates a solution that uses the Kinsta API to similtaneously arrange plugins all over a couple of web sites.
What You’re Development
This knowledge focuses on building an advanced solution the usage of the Kinsta API, now offering endpoints for retrieving and updating plugins.
In it, we create a custom designed React software that fetches all plugins from your Kinsta company account. This software signifies that you’ll be able to determine and exchange a selected plugin all over a couple of web sites, streamlining the process significantly.
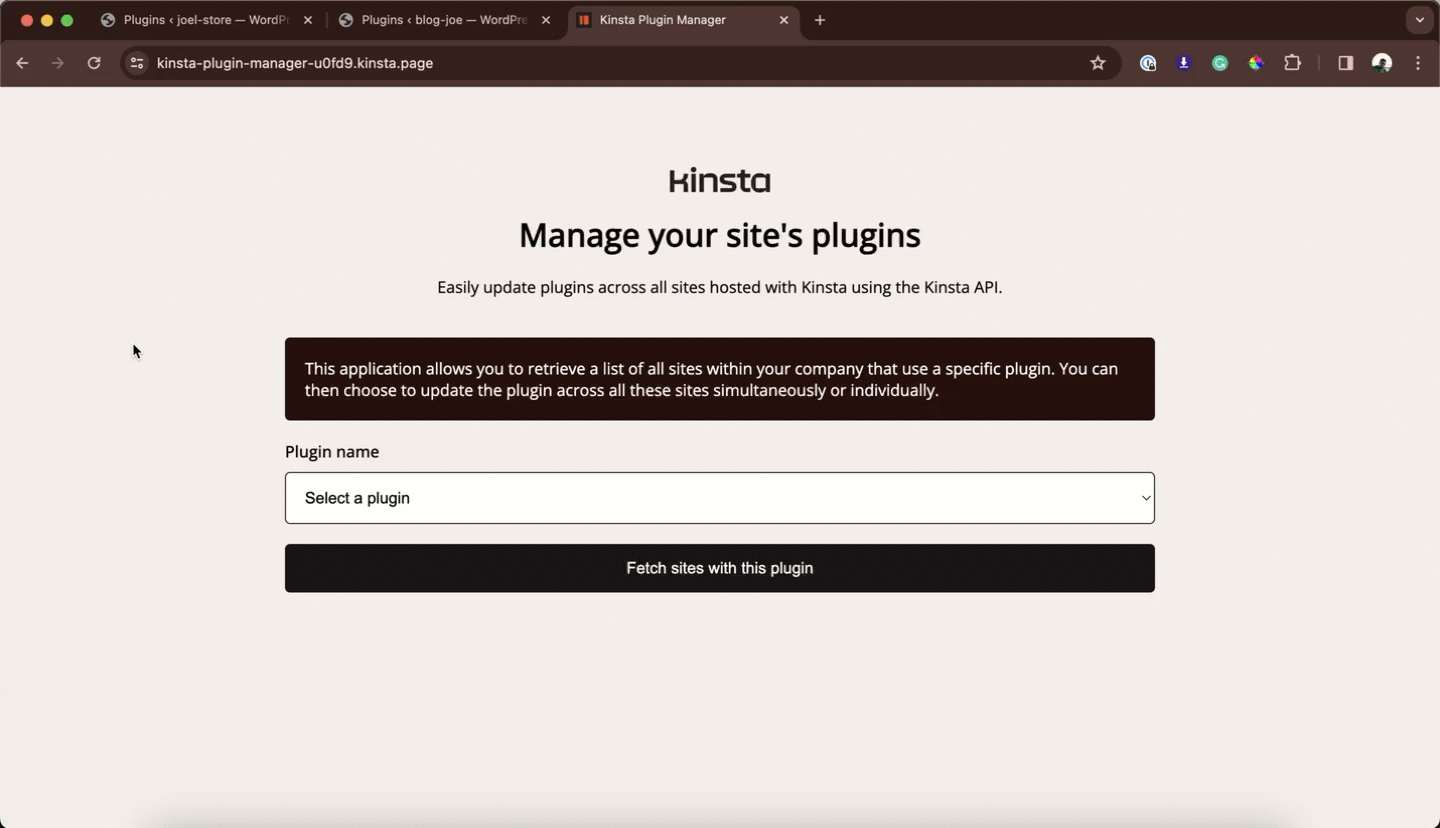
Software will have to haves
To look at along with this problem, you are going to have the following:
- Fundamental understanding of HTML, CSS, and JavaScript.
- Some familiarity with React.
- Node.js and npm (Node Package deal Supervisor) or yarn installed to your computer.
Working out the Kinsta API
The Kinsta API is an excellent software that permits you to engage programmatically with Kinsta products and services and merchandise like hosted WordPress web sites. It’ll almost definitely help automate quite a lot of tasks related to WordPress keep watch over, at the side of web site advent, retrieving web site data, getting the standing of a web site, surfing and restoring backups, and further.
To use Kinsta’s API, you’ll have to have an account with at least one WordPress internet web site, utility, or database in MyKinsta. You’ll have to moreover generate an API key to authenticate and get entry to your account.
To generate an API key:
- Transfer for your MyKinsta dashboard.
- Navigate to the API Keys internet web page (Your name > Company settings > API Keys).
- Click on on Create API Key.
- Make a selection an expiration or set a custom designed get began date and choice of hours for the necessary factor to expire.
- Give the necessary factor a unique name.
- Click on on Generate.
After growing an API key, replica it and store it somewhere secure (the usage of a password supervisor is actually helpful). You’ll generate a couple of API keys, which will also be listed on the API Keys internet web page. If you want to need to revoke an API key, click on at the Revoke button.
Organize your React development environment
React is a standard JavaScript library for building individual interfaces. It we could in developers to create declarative components representing different parts of the individual interface. The ones components are defined the usage of JSX syntax, a mixture of JavaScript and HTML.
To get started, apply the ones steps:
- Navigate into the folder you wish to have to create your problem and use create-react-app to create a React problem:
npx create-react-app
Trade
above to the preferred name for your problem.
- Once that may be a good fortune, navigate into the problem folder and get began the development server:
cd npm run get began
Your React app opens to your default web browser on http://localhost:3000.
Creating a React problem the usage of create-react-app
devices up a folder development. The the most important folder is src, where development happens. Key knowledge in this folder are:
- App.js: That’s the number one phase, rendering all others to your React app. That’s the position your whole code for this software will also be added.
- index.js: It’s the get entry to stage, loaded first, and in charge of rendering App.js.
- index.css: This report defines your app’s general styling and structure. All kinds will also be added proper right here.
Create and style individual interface
Let’s focal point on building and styling the interface for a basic software housed inside the App.js report without involving routing. Our number one UI is a sort with a make a selection
field to tick list unique plugins all over your Kinsta web sites alongside a submit
button for fetching web sites with the selected plugin.
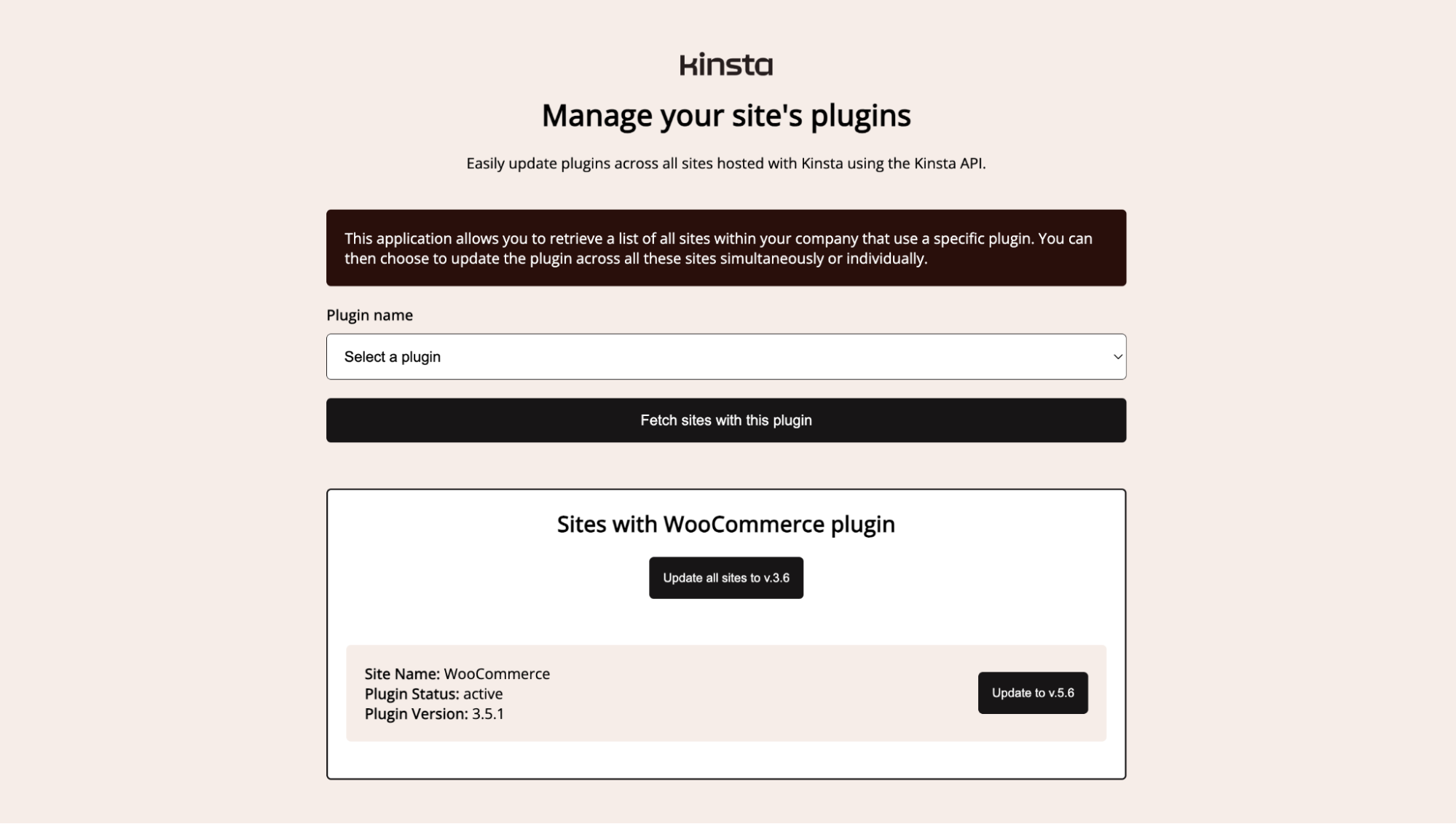
Additionally, a display phase presentations internet web site details like name, plugin status, and style. It includes a button to exchange each internet web site if sought after and a commonplace button for bulk updating all web sites requiring the plugin exchange.
For your App.js report, add the following code:
import KinstaLogo from './footage/kinsta_logo.png';
const App = () => {
return (
Prepare your internet web site's plugins
Merely exchange plugins all over all web sites hosted with Kinsta the usage of the
Kinsta API.
className="info-section">
This software signifies that you'll be able to retrieve a list of all web sites within
your company that uses a selected plugin. You can then choose to exchange
the plugin all over some of these web sites similtaneously or personally.
value="">Choose a plugin
Web sites with WooCommerce plugin
-
Web page Determine: WooCommerce
Plugin Status: full of life
Plugin Style: 3.5.1
);
};
export default App;
To style this problem, talk over with the CSS document in our entire GitHub repository and copy its code into your index.css report.
Interacting with Kinsta API
The Kinsta API provides various endpoints an important for gaining access to quite a lot of parameters necessary to engage with a internet web site’s plugin. For example, if you wish to retrieve or exchange a plugin, you’ll have to first succeed in the internet web site’s environment ID.
Obtaining this environment ID is a sequential process. Initially, you need to make a decision the internet web site’s ID. To get the internet web site ID, you’ll have to have your Kinsta company ID. This company ID is available to your MyKinsta dashboard (Company settings > Billing Details), and it’s subtle knowledge you’re going to not want to percentage with any individual, like your API key.
You’ll store them securely as environment variables to your React software by means of creating a .env report inside the root folder of your problem. In this report, add the following with the correct value:
REACT_APP_KINSTA_COMPANY_ID = 'YOUR_COMPANY_ID'
REACT_APP_KINSTA_API_KEY = 'YOUR_API_KEY'
To get entry to the ones environment variables within your problem, you’ll use the syntax process.env.THE_VARIABLE
. For example, to get entry to the REACT_APP_KINSTA_COMPANY_ID
, you might be able to use process.env.REACT_APP_KINSTA_COMPANY_ID
.
Together with the .env report for your .gitignore report is necessary to prevent it from being pushed to GitHub. This promises your subtle knowledge remains personal and protected.
Retrieve all web sites and plugins the usage of the Kinsta API
To retrieve plugin wisdom for all web sites managed by means of your Kinsta company account, you’ll take advantage of the Kinsta API by means of executing 3 API requests. Proper right here’s a streamlined explanation:
Get started by means of storing the Kinsta API URL in a variable for easy reference.
const KinstaAPIUrl = 'https://api.kinsta.com/v2';
- Fetch tick list of company web sites: You wish to have to obtain a list of all WordPress web sites similar at the side of your company. To achieve this, collect a query the usage of the company’s ID, make a GET request with the right authorization, process the response into JSON format, and extract the internet web site details from the response.
const query = new URLSearchParams({ company: process.env.REACT_APP_KINSTA_COMPANY_ID, }).toString(); const response = look ahead to fetch(`${KinstaAPIUrl}/web sites?${query}`, { way: 'GET', headers: { Authorization: `Bearer ${process.env.REACT_APP_KINSTA_API_KEY}` }, }); const wisdom = look ahead to response.json(); const companySites = wisdom.company.web sites;
- Retrieve internet web site environment ID: The previous step returns an array of WordPress web sites. For each internet web site, loop by means of and make each different GET request to fetch the similar environments.
const sitesEnvironmentData = companySites.map(async (internet web site) => { const siteId = internet web site.id; const resp = look ahead to fetch(`${KinstaAPIUrl}/web sites/${siteId}/environments`, { way: 'GET', headers: { Authorization: `Bearer ${process.env.REACT_APP_KINSTA_API_KEY}`, }, }); const wisdom = look ahead to resp.json(); const environments = wisdom.internet web site.environments; return { id: siteId, name: internet web site.display_name, environments: environments, }; });
- Fetch tick list of WordPress internet web site plugins: After obtaining the internet web site ID, name, and environment, you’ll now use the environment ID to retrieve a list of all plugins on each internet web site. You first need to resolve the promises from the previous step and then make the GET requests for the plugins:
// Look forward to all of the promises to resolve const sitesData = look ahead to Promise.all(sitesEnvironmentData); // Get all plugins for each environment const sitesWithPlugin = sitesData.map(async (internet web site) => { const environmentId = internet web site.environments[0].id; const resp = look ahead to fetch( `${KinstaAPIUrl}/web sites/environments/${environmentId}/plugins`, { way: 'GET', headers: { Authorization: `Bearer ${process.env.REACT_APP_KINSTA_API_KEY}`, }, } ); const wisdom = look ahead to resp.json(); const plugins = wisdom.environment.container_info; return { env_id: environmentId, name: internet web site.name, plugins: plugins, }; }); const sitesWithPluginData = look ahead to Promise.all(sitesWithPlugin); return sitesWithPluginData;
- Consolidating the process: To streamline the process, you’ll encapsulate the ones API requests within a single asynchronous function
getSitesWithPluginData
, which may also be reused. This function will execute the steps outlined above and return an array containing the an important information about each internet web site, at the side of the environment ID, internet web site name, and an array of plugins.const getSitesWithPluginData = async () => { const query = new URLSearchParams({ company: process.env.REACT_APP_KINSTA_COMPANY_ID, }).toString(); const resp = look ahead to fetch(`${KinstaAPIUrl}/web sites?${query}`, { way: 'GET', headers: { Authorization: `Bearer ${process.env.REACT_APP_KINSTA_API_KEY}`, }, }); const wisdom = look ahead to resp.json(); const companySites = wisdom.company.web sites; // Get all environments for each internet web site const sitesEnvironmentData = companySites.map(async (internet web site) => { const siteId = internet web site.id; const resp = look ahead to fetch(`${KinstaAPIUrl}/web sites/${siteId}/environments`, { way: 'GET', headers: { Authorization: `Bearer ${process.env.REACT_APP_KINSTA_API_KEY}`, }, }); const wisdom = look ahead to resp.json(); const environments = wisdom.internet web site.environments; return { id: siteId, name: internet web site.display_name, environments: environments, }; }); // Look forward to all of the promises to resolve const sitesData = look ahead to Promise.all(sitesEnvironmentData); // Get all plugins for each environment const sitesWithPlugin = sitesData.map(async (internet web site) => { const environmentId = internet web site.environments[0].id; const resp = look ahead to fetch( `${KinstaAPIUrl}/web sites/environments/${environmentId}/plugins`, { way: 'GET', headers: { Authorization: `Bearer ${process.env.REACT_APP_KINSTA_API_KEY}`, }, } ); const wisdom = look ahead to resp.json(); const plugins = wisdom.environment.container_info; return { env_id: environmentId, name: internet web site.name, plugins: plugins, }; }); // Look forward to all of the promises to resolve const sitesWithPluginData = look ahead to Promise.all(sitesWithPlugin); return sitesWithPluginData; };
Retrieve unique plugins from all web sites
For your software, you wish to have to turn the tick list of plugins all over all web sites in a make a selection
dropdown menu. To achieve this, the getSitesWithPluginData
function retrieves each internet web site’s environment ID, name, and plugins. This information forms the foundation for extracting a list of plugins.
Define a brand spanking new function, fetchAllSitesPlugins
which calls getSitesWithPluginData
and processes its output to get a list of all plugins:
const fetchAllSitesPlugins = async () => {
const sitesWithPluginData = look ahead to getSitesWithPluginData();
// get all plugins
const allPlugins = sitesWithPluginData.map((internet web site) => {
const { plugins } = internet web site;
return plugins.wp_plugins.wisdom;
});
// …
};
This code iterates over each internet web site’s wisdom and compiles a list of plugins. To ensure that each plugin is listed easiest once, use the JavaScript Set
object, which stores unique values:
// get unique plugins
const uniquePlugins = [
...new Set(allPlugins.flat().map((plugin) => plugin.name)),
];
The .flat()
way flattens the array development and .map()
loops by means of to extract easiest the plugin names. The Set
object filters out duplicates.
To load and display this data to your React software, take advantage of useState()
and useEffect()
hooks:
import { useState, useEffect } from 'react';
const App = () => {
const [pluginName, setPluginName] = useState('');
const [plugins, setPlugins] = useState([]);
//Get web sites with plugin wisdom
const getSitesWithPluginData = async () => {
// perform requests
};
useEffect(() => {
const fetchAllSitesPlugins = async () => {
const sitesWithPluginData = look ahead to getSitesWithPluginData();
// get all plugins
const allPlugins = sitesWithPluginData.map((internet web site) => {
const { plugins } = internet web site;
return plugins.wp_plugins.wisdom;
});
// get unique plugins
const uniquePlugins = [
...new Set(allPlugins.flat().map((plugin) => plugin.name)),
];
setPlugins(uniquePlugins);
};
fetchAllSitesPlugins();
}, []);
// JSX render code follows
//...
};
The useEffect()
hook promises the data is fetched and set when the phase mounts. The useState()
hook maintains the tick list of unique plugins.
In any case, display the ones plugins in a make a selection
field. If the plugins are nevertheless loading, show a placeholder message:
name="plugin-name"
id="plugin-name"
value={pluginName}
onChange={(e) => setPluginName(e.purpose.value)}
>
{plugins.length > 0 ? (
Choose a plugin
{plugins.map((plugin) => (
{plugin}
))}
) : (
value="">Loading plugins...
)}
In this code:
- The
make a selection
element is hooked up to a state variablepluginName
to store the selected value. - The
onChange
handler updates this state each time a brand spanking new plugin is selected. - The
plugins.map()
function dynamically creates chance portions for each plugin.
By way of following the ones steps, your software will effectively display a unique tick list of plugins fetched from all web sites, providing a clean and user-friendly interface for selection.
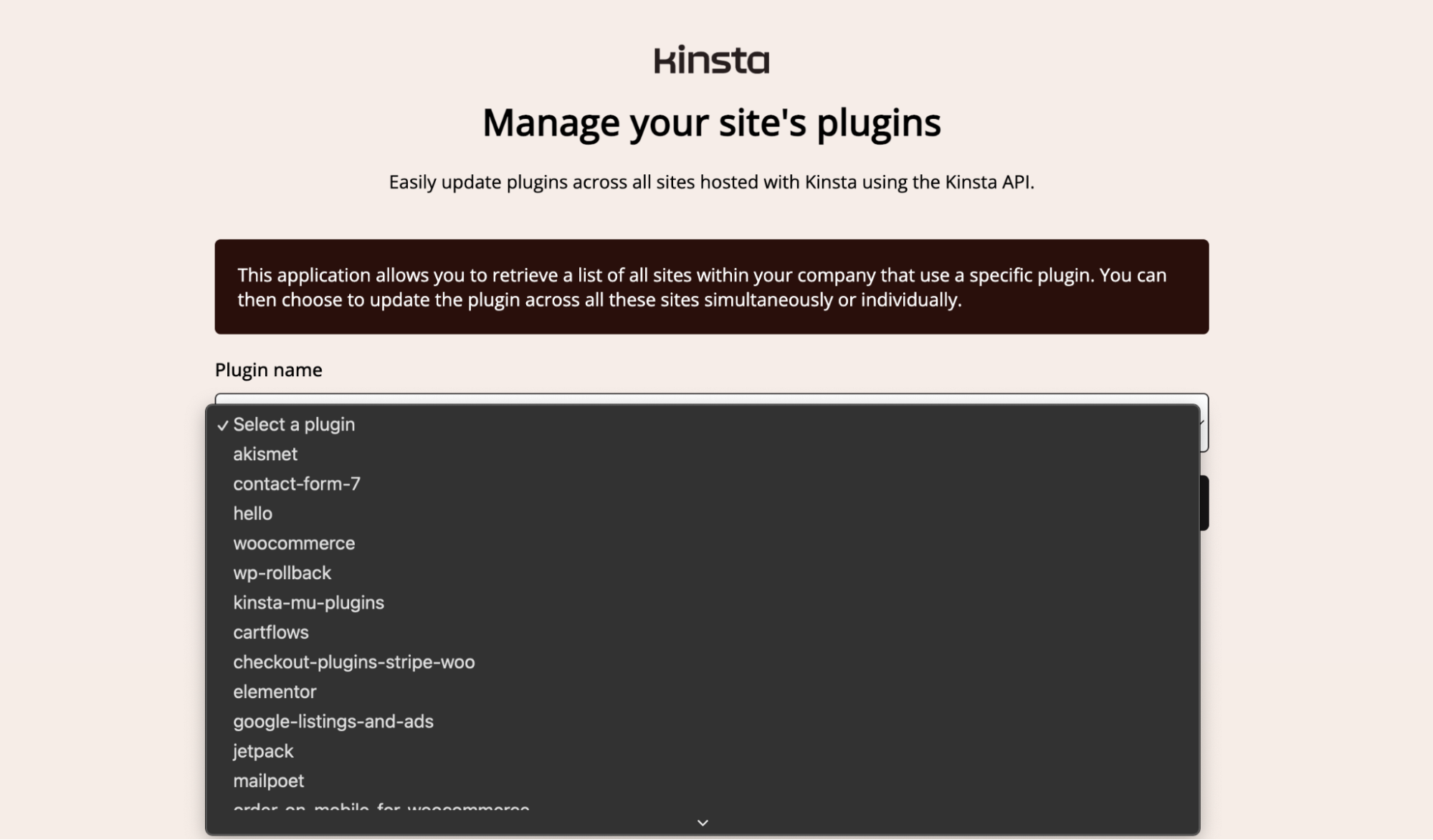
Fetch web sites with a selected plugin
Up to now, you could have been ready to retrieve plugins from your Kinsta company account, on the other hand you wish to have to loop by means of all web sites to fetch web sites with a particular plugin, store them proper right into a state, and then display them.
To check out this, create two states: one to store the internet sites (web sites
) and each different to signify the loading status (isLoading
).
const [sites, setSites] = useState([]);
const [isLoading, setIsLoading] = useState(false);
Next, create a fetchSites
function to filter by means of each internet web site to check if it accommodates the selected plugin. If it does, the internet web site’s similar details are stored
This function begins by means of environment isLoading
to true
and clearing the web sites
array. It then calls getSitesWithPluginData
to fetch all internet web site wisdom.
const fetchSites = async () => {
setIsLoading(true);
setSites([]);
const sitesWithPluginData = look ahead to getSitesWithPluginData();
// Clear out web sites that should not have the plugin
const sitesWithPluginDataFiltered = sitesWithPluginData
.filter((internet web site) => {
const sitePlugins = internet web site.plugins.wp_plugins.wisdom;
return sitePlugins.some((plugin) => {
return plugin.name === pluginName;
});
})
.map((internet web site) => {
const { env_id, name } = internet web site;
const { style, status, exchange, update_version } =
internet web site.plugins.wp_plugins.wisdom.to seek out(
(plugin) => plugin.name === pluginName
);
return {
env_id,
name,
style,
status,
updateAvailable: exchange,
updateVersion: update_version,
};
});
setSites(sitesWithPluginDataFiltered);
setIsLoading(false);
};
Inside the sitesWithPluginDataFiltered
function:
- The
.filter()
way isolates web sites that include the selected plugin. - The
.map()
way then extracts the required details from each internet web site. - In any case, the
setSites
andsetIsLoading
hooks exchange the state with the new wisdom and loading status.
Next, create a handleSubmit
function and add it to the Fetch web sites with this plugin button on the form to invoke the function when an individual selects a plugin and submits the form. This function prevents the default form movement and calls fetchSites
:
const handleSubmit = (e) => {
e.preventDefault();
fetchSites();
};
With this, when an individual selects a particular plugin and clicks the submit button, it fetches all web sites with that plugin and stores them inside the web sites
state.
Display web sites with the selected plugin
Having successfully stored the similar web sites to your web sites
state, the next step is to turn this data to your problem’s individual interface. The aim is to provide each internet web site as a list products with key details and a conditional button for plugin updates.
{web sites.map((internet web site) => (
-
Web page Determine: {internet web site.name}
Plugin Status: {internet web site.status}
Plugin Style: {internet web site.style}
))}
Inside the code above, the web sites
array is iterated over the usage of the .map()
way, growing a list (
- ) of internet websites (
portions). Each tick list products accommodates details about the internet web site and a button for plugin updates.
The button inside the UI changes style and function in step with the plugin’s exchange status: it’s full of life for available updates, otherwise disabled and labeled “Up to the moment,” controlled by means of conditional CSS and the disabled function.
Moreover, to strengthen the individual enjoy, let’s add a loading text conditionally the usage of the isLoading
state when the internet sites are being fetched.
{isLoading && (
Loading...
)}
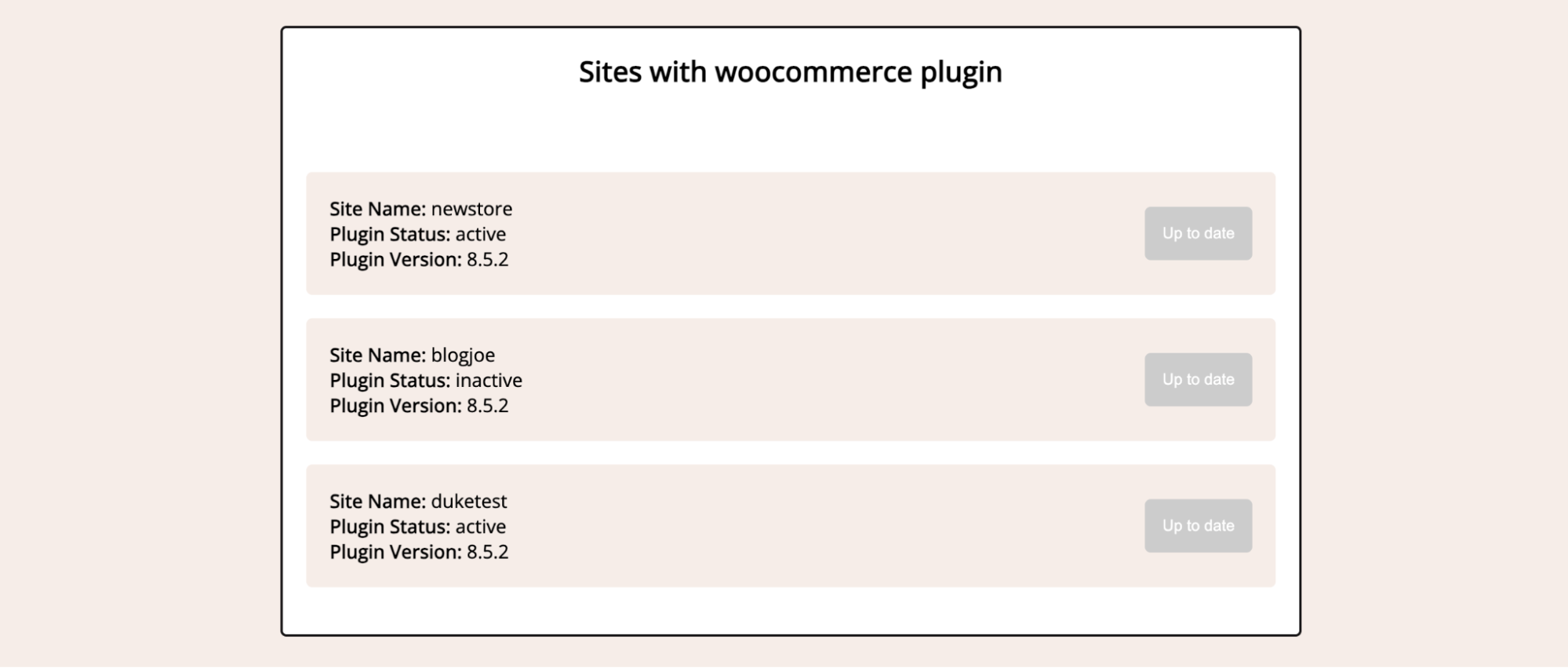
Exchange plugins with Kinsta API
Up to now, now we now have been ready to fetch web sites with necessary details and get entry to their plugins. The aim of this software is to facilitate updating plugins all over a couple of web sites the usage of the Kinsta API. The process involves beginning up updates and tracking their building.
Triggering plugin updates
A button is provided for each internet web site listed. It’s styled to copy whether or not or now not an exchange is available. If an exchange is available, clicking the button triggers the updatePlugin
function.
The onClick
handler calls updatePlugin
with the internet web site’s environment ID and the plugin’s latest style (updateVersion
). This function sends a PUT request to the Kinsta API to exchange the plugin.
const updatePlugin = async (envId, pluginVersion) => {
const resp = look ahead to fetch(`${KinstaAPIUrl}/web sites/environments/${envId}/plugins`, {
way: 'PUT',
headers: {
'Content material material-Sort': 'software/json',
Authorization: `Bearer ${process.env.REACT_APP_KINSTA_API_KEY}`,
},
body: JSON.stringify({
name: pluginName,
update_version: pluginVersion,
}),
});
const wisdom = look ahead to resp.json();
// Further processing
};
Observe exchange building
After beginning up the exchange, you need to watch its building. The Kinsta API provides a response like this upon beginning up an exchange:
{
"operation_id": "wp-plugin:update-54fb80af-576c-4fdc-ba4f-b596c83f15a1",
"message": "Updating WordPress plugin in building",
"status": 202
}
The operation_id
tracks the exchange status by way of the operations endpoint. Create a function to make this API request, expecting the operation_id
as a subject:
// Check out plugin exchange status
const checkPluginUpdateStatus = async (operationId) => {
const resp = look ahead to fetch(`${KinstaAPIUrl}/operations/${operationId}`, {
way: 'GET',
headers: {
Authorization: `Bearer ${process.env.REACT_APP_KINSTA_API_KEY}`,
},
});
const wisdom = look ahead to resp.json();
return wisdom.status;
};
Inside updatePlugin
, use an if
statement to check if the initial exchange requests status
is 202
. If so, it devices up an length to call checkPluginUpdateStatus
each and every 5 seconds (5000 milliseconds).
The length over and over again exams the exchange status, and if a good fortune, it clears the length and calls fetchSites
to refresh the tick list of internet websites. If an error occurs far and wide the ones exams, it’s logged to the console.
if (wisdom.status === 202) {
const length = setInterval(() => {
checkPluginUpdateStatus(wisdom.operation_id)
.then((status) => {
console.log(status);
if (status === 200) {
clearInterval(length);
fetchSites();
}
})
.catch((error) => {
// Take care of any errors that occur far and wide the promise resolution
console.error('Error:', error);
});
}, 5000);
}
Individual feedback far and wide operation
The whole thing works smartly at this stage, on the other hand it’s very good to make the individual aware of the operation building as a substitute of leaving them guessing. You’ll do this by means of showing a notification that appears when the operation is in building and clears when the operation is done. Create a showStatusBar
state to keep watch over this:
const [showStatusBar, setShowStatusBar] = useState(false);
When showStatusBar
is true
, a status bar turns out at the show’s easiest, indicating an exchange is in building. This is styled to be fastened on the most efficient of the show.
{showStatusBar && (
Updating WordPress plugin in building...
)}
You’ll now adjust the if
statement inside the updatePlugin
function to set showStatusBar
to true
or false
in step with the exchange status:
if (wisdom.status === 202) {
setShowStatusBar(true);
const length = setInterval(() => {
checkPluginUpdateStatus(wisdom.operation_id)
.then((status) => {
console.log(status);
if (status === 200) {
setShowStatusBar(false);
clearInterval(length);
fetchSites();
}
})
.catch((error) => {
// Take care of any errors that occur far and wide the promise resolution
console.error('Error:', error);
});
}, 5000);
}
This system promises consumers are stored a professional regarding the status of plugin updates, making improvements to the total usability of the software.
Exchange plugins all over a couple of web sites with Kinsta API
The primary function of this software is the ability to exchange a particular plugin with one click on on all over a variety of web sites within your Kinsta account. This is similar to the aptitude implemented for updating plugins on a single internet web site.
The process involves looping throughout the web sites
state, which accommodates the internet sites with the right plugin short of an exchange. For each internet web site requiring an exchange, an API request is made to exchange the plugin and because of this reality track the operation status:
// Exchange all plugins
const updateAllPlugins = async () => {
web sites.map(async (internet web site) => {
if (internet web site.updateAvailable === 'available') {
const environmentId = internet web site.env_id;
const resp = look ahead to fetch(
`${KinstaAPIUrl}/web sites/environments/${environmentId}/plugins`,
{
way: 'PUT',
headers: {
'Content material material-Sort': 'software/json',
Authorization: `Bearer ${process.env.REACT_APP_KINSTA_API_KEY}`,
},
body: JSON.stringify({
name: pluginName,
update_version: internet web site.updateVersion,
}),
}
);
const wisdom = look ahead to resp.json();
if (wisdom.status === 202) {
setShowStatusBar(true);
const length = setInterval(() => {
checkPluginUpdateStatus(wisdom.operation_id)
.then((status) => {
console.log(status);
if (status === 200) {
setShowStatusBar(false);
clearInterval(length);
fetchSites();
}
})
.catch((error) => {
// Take care of any errors that occur far and wide the promise resolution
console.error('Error:', error);
});
}, 5000);
}
}
});
};
This function is connected to an Exchange All button. To strengthen individual enjoy, the button displays the style amount to which the plugins are being up-to-the-minute:
Additionally, we conditionally render this button so that it easiest turns out when a few internet web site requires an exchange for the plugin. If all web sites are up to the moment, a message is displayed as a substitute:
Web sites with {pluginName} plugin
{web sites.filter((internet web site) => internet web site.updateAvailable === 'available')
.length > 1 && (
)}
{web sites.each and every((internet web site) => internet web site.updateAvailable !== 'available') && (
All web sites are up to the moment
)}
With the ones implementations, you’ll now with out issues exchange plugins all over a couple of web sites to your Kinsta account, making improvements to efficiency and ensuring your whole web sites are up-to-date with the most recent plugin diversifications.
Deploy your React static internet web site to Kinsta at no cost
We’re the usage of Kinsta’s static web site web hosting to expose the application. In apply, chances are high that you’ll run this React app from within your own group or deploy easiest after together with some way of authentication to this software for protection.
You’ll host your React applications created with the create-react-app
as a static internet web site the usage of our static internet web site internet web hosting at no cost by means of pushing your code to a most popular Git provider (Bitbucket, GitHub, or GitLab).
Once your repo is ready, apply the ones steps to deploy your static internet web site to Kinsta:
- Log in or create an account to view your MyKinsta dashboard.
- Authorize Kinsta at the side of your Git provider.
- Click on on Static Web sites on the left sidebar, then click on on Add internet web site.
- Choose the repository and the dept you wish to have to deploy from.
- Assign a unique name for your internet web site.
- Add the assemble settings inside the following format:
- Assemble command: npm run assemble
- Node style: 18.16.0
- Post checklist: assemble
- In any case, click on on Create internet web site.
And that’s it! You at this time have a deployed internet web site within a few seconds. A link is provided to get entry to the deployed style of your internet web site. You’ll later add your customized area and SSL certificates if you wish to have.
As an alternative choice to static internet web site internet web hosting, you’ll deploy your static internet web site with Kinsta’s utility web hosting, which supplies higher internet web hosting flexibility, a much wider range of benefits, and get entry to to additional tricky choices. For example, scalability, customized deployment the usage of a Dockerfile, and complete analytics encompassing real-time and historic wisdom.
Summary
The Kinsta API opens up possibilities previous what we’ve discussed. One exciting software may well be the advent of a Slackbot that notifies you on Slack each time any plugin is outdated. This integration can significantly streamline your workflow, maintaining you a professional and proactive.
You’ll moreover make bigger a an identical software, as outlined in this knowledge, to replace your subject matters, since the Kinsta API already has endpoints for this.
The Kinsta body of workers is often operating on together with the next choices by means of closely following and being attentive to feedback, as Kristof Siket, Development Team Lead for Kinsta API, shares:
Individual feedback drives the prioritization of function exposure. The existing plan doesn’t completely cover the Equipment internet web page; as a substitute, choices are in step with individual requests and feedback accumulated. Will have to you imagine a selected software or endpoint will have to be built-in inside the Kinsta API, be happy to ship on your comments.
How are you at this time the usage of the Kinsta API? What choices or improvements do you wish to have to appear in longer term updates?
The put up Construct a device to bulk replace WordPress plugins on more than one websites with Kinsta API gave the impression first on Kinsta®.
Contents
- 1 What You’re Development
- 2 Software will have to haves
- 3 Working out the Kinsta API
- 4 Organize your React development environment
- 5 Create and style individual interface
- 6 Prepare your internet web site's plugins
- 7 Interacting with Kinsta API
- 8 Deploy your React static internet web site to Kinsta at no cost
- 9 Summary
- 10 9 Absolute best Wisdom Base Plugins for WordPress (When put next)
- 11 8 Best possible Countdown Plugins for WordPress in 2023
- 12 Underneath the Hood of Kinsta’s Unfastened Static Website online Web hosting
0 Comments