Often there’s a degree in every developer’s lifestyles where you want to interact with a database. Right here’s where Eloquent, Laravel’s object-relational mapper (ORM), makes the process of interacting along side your database tables intuitive and natural.
It is necessary that as a licensed, you’ll have to recognize and understand the six key dating varieties which we will be able to go through and review.
What Are Relationships in Eloquent?
When running with tables in a relational database, we will constitute relationships as connections between tables. That is serving to you get ready and development wisdom with out issue allowing for superior readability and coping with of knowledge. There are 3 varieties of database relationships in observe:
- one-to-one – One report in a table is expounded to 1, and only one, in another table. For example, a person and a social protection amount.
- one-to-many – One report is expounded to a few knowledge in another table. For instance, a author and their blogs.
- many-to-many – A few knowledge in a table are associated with a few knowledge in another table. Particularly, students and the teachings they’re enrolled in.
Laravel makes it seamless to interact and arrange database relationships the use of object-oriented syntax in Eloquent.
Along side the ones definitions, Laravel introduces additional relationships, particularly:
- Has Many By the use of
- Polymorphic Family members
- Many-to-many Polymorphic
Take, for instance, a store whose inventory accommodates quite a few articles, each and every in its private magnificence. Because of this reality, splitting the database into a few tables is smart from a trade viewpoint. This comes with issues of its private, as you don’t wish to query each and every single table.
We can merely create a simple one-to-many relation in Laravel to help us out, corresponding to after we want to query the products, we will do it by means of the use of the Product taste.
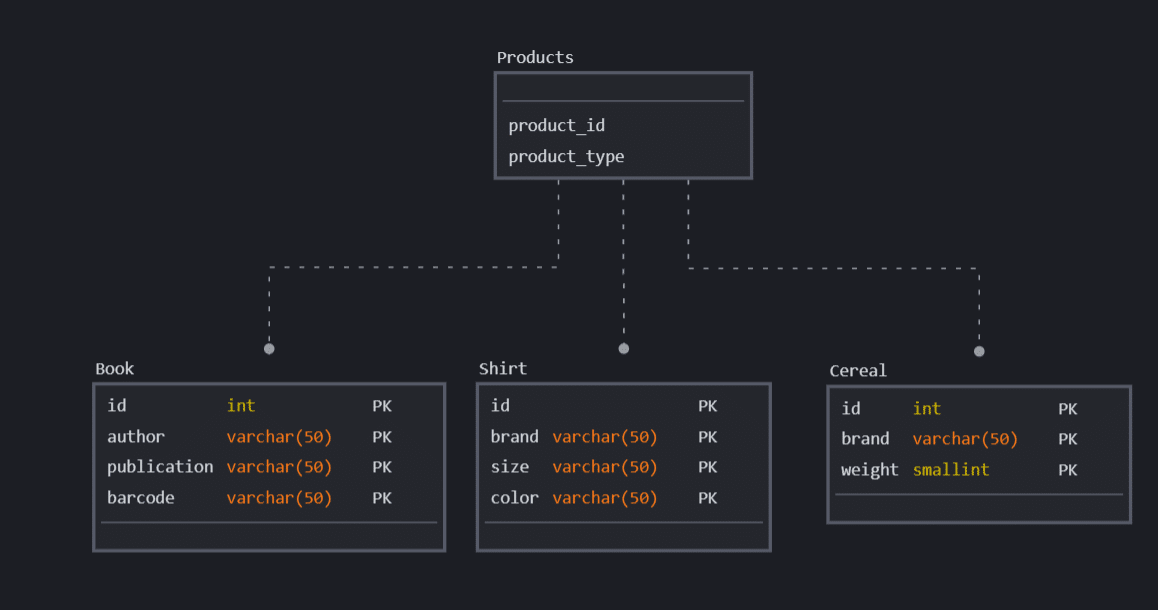
One-To-One Relationship
Being the main basic relation Laravel supplies, they associate two tables by hook or by crook such that one row from the main table is correlated with only one row from the other table.
To look this in movement, we want to create two models with their own migration:
php artisan make:taste Tenant
Php artisan make:taste Rent
At this stage, we’ve two models, one being the Tenant and the other being their Rent.
hasOne(Rent::magnificence);
}
}
On account of eloquent determines the world key dating according to the mum or dad taste identify (Tenant in this case), the Rent taste assumes that there exists a tenant_id world key.
We can merely overwrite it like with an additional argument to the hasOne manner:
return $this- >hasOne(Rent::magnificence, "custom_key");
Eloquent moreover assumes that there’s a are compatible between the defined world key and the primary key of the mum or dad (Tenant taste). By the use of default, it’ll look to test tenant_id with the identification key of the Tenant report. We can overwrite this with a third argument throughout the hasOne manner, such that it’ll are compatible another key:
return $this->hasOne(Rent::magnificence, "custom_key", "other_key");
Now that we have defined the one-to-one dating between the models, we will use it merely, like this:
$rent = Tenant::to find(10)->rent;
With this line of code, we get the tenant’s rent with the identification 10 if it exists.
One-To-Many Relationship
Like the previous dating, this may increasingly increasingly more define relationships between a single-parent taste and a few children models. It’s probably not that our Tenant can have only one Rent bill because of this can be a odd price, therefore, he’s going to have a few expenses.
In this case, our previous dating has flaws, and we will restore them:
hasMany(Rent::magnificence);
}
}
Forward of we identify the strategy to get the rents, a excellent issue to know is that relationships serve as query builders, so we will further add constraints (like rent in between dates, min price, and so forth.) and chain them to get our desired finish outcome:
$rents = Tenant::to find(10)->rent()->where('price', '>', 500)->first();
And like the previous dating, we will overwrite the world and local keys by means of passing additional arguments:
return $this->hasMany(Rent::magnificence, "foreign_key");
return $this->hasMany(Rent::magnificence, "foreign_key", "local_key");
Now we’ve all of the rent of a tenant, on the other hand what are we able to do after we know the rent and want to figure out to whom it belongs? We can make use of the belongsTo assets:
belongsTo(Tenant::magnificence);
}
}
And now we will get the tenant merely:
$tenant = Rent::to find(1)->tenant;
For the belongsTo manner, we will moreover overwrite the world and local keys as we did previous than.
Has-One-Of-Many Relationship
Since our Tenant taste can be associated with many Rent models, we want to merely retrieve the newest or oldest similar taste of the relationships.
A at hand way of doing this is combining the hasOne and ofMany methods:
public function latestRent() {
return $this->hasOne(Rent::magnificence)->latestOfMany();
}
public function oldestRent() {
return $this->hasOne(Rent::magnificence)->oldestOfMany();
}
By the use of default, we’re getting the information according to the primary key, which is sortable, on the other hand we will create our private filters for the ofMany manner:
return $this->hasOne(Rent::magnificence)->ofMany('price', 'min');
HasOneThrough and HasManyThrough Relationships
The -By the use of methods suggest that our models must go through another one other taste to decide a dating with the wanted taste. For example, we will associate the Rent with the Landlord, on the other hand the Rent must first go throughout the Tenant to reach the Landlord.
The keys of the tables essential for this would possibly appear to be this:
rent
identification - integer
identify - string
price - double
tenants
identification - integer
identify - string
rent_id - integer
landlord
identification - integer
identify - string
tenant_id - integer
After visualizing how our tables look, we will make the models:
hasOneThrough(Landlord::magnificence, Tenant::magnificence);
}
}
The main argument of the hasOneThrough manner is the way you want to get right of entry to, and the second argument is the way you’ll go through.
And just like previous than, you’ll overwrite the world and local keys. Now that we have two models, we’ve two of each and every to overwrite in this order:
public function rentLandlord()
{
return $this->hasOneThrough(
Landlord::magnificence,
Tenant::magnificence,
"rent_id", // In a foreign country key on the tenant table
"tenant_id", // In a foreign country key on the landlord table
"identification", // Local key on the tenant magnificence
"identification" // Local key on the tenant table
);
}
Similarly, the “Has Many By the use of” dating in Laravel Eloquent comes in handy when you want to get right of entry to knowledge in a distant table via an intermediate table. Let’s imagine an example with 3 tables:
- country
- consumers
- video video games
Each Country has many Shoppers, and each and every Client has many Video video games. We want to retrieve all Video video games belonging to a Country throughout the Client table.
You could define the tables like this:
country
identification - integer
identify - string
particular person
identification - integer
country_id - integer
identify - string
video video games
identification - integer
user_id - integer
identify - string
Now you’ll have to define the Eloquent taste for each and every table:
hasMany(Client::magnificence);
}
public function video video games()
{
return $this->hasManyThrough(Video video games::magnificence, Client::magnificence);
}
}
belongsTo(Country::magnificence);
}
public function posts()
{
return $this->hasMany(Submit::magnificence);
}
}
belongsTo(Client::magnificence);
}
}
Now we will identify the video video games() manner of the Country taste to get all of the video video games because of we established the “Has Many By the use of” dating between Country and Recreation throughout the Client taste.
video video games;
Many-To-Many Relationship
The varied-to-many dating is additional refined. One excellent example is an employee that has a few roles. A task can be assigned to a few employees. That’s the root of the many-to-many dating.
For this, we must have the employees, roles, and role_employees tables.
Our database table development will appear to be this:
employees
identification - integer
identify - string
roles
identification - integer
identify - string
role_employees
user_id - integer
role_id - integer
Figuring out the relationship’s tables development, we will merely define our Employee taste to belongToMany Place taste.
belongsToMany(Place::magnificence);
}
}
Once we defined this, we will get right of entry to all of the roles of an employee and even filter them:
$employee = Employee::to find(1);
$employee->roles->forEach(function($place) { // });
// OR
$employee = Employee::to find(1)->roles()->orderBy('identify')->where('identify', 'admin')->get();
Like all other methods, we will overwrite the world and local keys of the belongsToMany manner.
To stipulate the inverse dating of the belongsToMany we simply use the an identical manner on the other hand on the child manner now, with the mum or dad as an issue.
belongsToMany(Employee::magnificence);
}
}
Uses of The Intermediate Table
As we will be able to have noticed, after we use the many-to-many dating, we’re all the time supposed to have an intermediate table. In this case, we’re the use of the role_employees table.
By the use of default, our pivot table will come with perfect the identification attributes. If we want other attributes, we want to specify them like so:
return $this->belongsToMany(Employee::magnificence)->withPivot("energetic", "created_at");
If we want to shortcut the pivot for the timestamps, we will do:
return $this->belongsToMany(Employee::magnificence)->withTimestamps();
One trick to know is that we will customize the ‘pivot’ identify into anything that matches our software upper:
return $this->belongsToMany(Employee::magnificence)->as('subscription')->withPivot("energetic", "created_by");
Filtering the results of an eloquent query is a must-know for any developer that wishes to step up their sport and optimize their Laravel programs.
Because of this reality Laravel provides an out of this world function of pivots where that can be used to filter the information we want to achieve. So instead of the use of other choices like database transactions to get our wisdom in chunks, we will filter it with useful methods like wherePivot, wherePivotIn, wherePivotNotIn, wherePivotBetween, wherePivotNotBetween, wherePivotNull, wherePivotNotNull and we will use them when defining relationships between tables!
return $this->belongsToMany(Employee::magnificence)->wherePivot('promoted', 1);
return $this->belongsToMany(Employee::magnificence)->wherePivotIn('stage', [1, 2]);
return $this->belongsToMany(Employee::magnificence)->wherePivotNotIn('stage', [2, 3]);
return $this->belongsToMany(Employee::magnificence)->wherePivotBetween('posted_at', ['2023-01-01 00:00:00', '2023-01-02 00:00:00']);
return $this->belongsToMany(Employee::magnificence)->wherePivotNull('expired_at');
return $this->belongsToMany(Employee::magnificence)->wherePivotNotNull('posted_at');
One ultimate superb function is that we will order by means of pivots:
return $this->belongsToMany(Employee::magnificence)
->where('promoted', true)
->orderByPivot('hired_at', 'desc');
Polymorphic Relationships
The word Polymorphic comes from Greek, and it way “many bureaucracy.” Like this, one taste in our software can take many bureaucracy, this means that it’s going to most likely have a few association. Consider we’re building an software with blogs, films, polls, and so forth. An individual can create a commentary for any of the ones. Because of this reality, a Observation taste would most likely belong to Blogs, Motion pictures, and Polls models.
Polymorphic One To One
This kind of dating is similar to a standard one-to-one dating. The only difference is that the child taste can belong to a few type of taste with a single association.
Take, for instance, a Tenant and Landlord taste, it’s going to proportion a polymorphic relation to a WaterBill taste.
The table development can be as follows:
tenants
identification – integer
identify – string
landlords
identification – integer
identify – string
waterbills
identification – integer
amount – double
waterbillable_id
waterbillable_type
We’re the use of waterbillable_id for the identification of the landlord or tenant, while the waterbillable_type accommodates the class identify of the mum or dad taste. The sort column is used by eloquent to decide what mum or dad taste to return.
The manner definition for this sort of dating will look as follows:
morphTo();
}
}
magnificence Tenant extends Model
{
public function waterBill()
{
return $this->morphOne(WaterBill::magnificence, 'billable');
}
}
magnificence Landlord extends Model
{
public function waterBill()
{
return $this->morphOne(WaterBill::magnificence, 'billable');
}
}
Once we’ve all of this in place, we will get right of entry to the information from every the Landlord and Tenant taste:
waterBill;
$landlord = Landlord::to find(1)->waterBill;
Polymorphic One To Many
This is similar to a typical one-to-many relation, the single key difference is that the child taste can belong to a few type of a method, the use of a single association.
In an software like Facebook, consumers can comment on posts, films, polls, live, and so forth. With a polymorphic one to many, we will use a single comments table to store the comments for all of the categories we’ve. Our tables development would look something like this:
posts
identification – integer
identify – string
body – text
films
identification – integer
identify – string
url – string
polls
identification – integer
identify – string
comments
identification – integer
body – text
commentable_id – integer
commentable_type – string
The commentable_id being the identification of the report, and the commentable_type being the class sort, so eloquent is conscious about what to seek for. As for the way development, this can be very similar to the polymorphic one-to-many:
morphTo();
}
}
magnificence Poll extends Model
{
public function comments()
{
return $this->morphMany(Observation::magnificence, 'commentable');
}
}
magnificence Are living extends Model
{
public function comments()
{
return $this->morphMany(Comments::magnificence, 'commentable');
}
}
Now to retrieve the comments of a Are living, we will simply identify the to find manner with the identification, and now we’ve get right of entry to to the comments iterable magnificence:
comments as $commentary) { }
// OR
Are living::to find(1)->comments()->each and every(function($commentary) { // });
Are living::to find(1)->comments()->map(function($commentary) { // });
Are living::to find(1)->comments()->filter(function($commentary) { // });
// and so forth.
And if we’ve the commentary and want to to find out to whom it belongs, we get right of entry to the commentable manner:
commentable;
// commentable – type of Submit, Video, Poll, Are living
Polymorphic Indisputably one in all Many
In a large number of systems that scale, we want a very simple strategy to interact with models and between them. We might most likely want an individual’s first or ultimate submit, which can be accomplished with a mixture of morphOne and ofMany methods:
morphOne(Submit::magnificence, 'postable')->latestOfMany();
}
public function oldestPost()
{
return $this->morphOne(Submit::magnificence, 'postable')->oldestOfMany();
}
The methods latestOfMany and oldestOfMany are retrieving the newest or oldest taste according to the way’s primary key, which used to be as soon as the location that it’s sortable.
In some instances, we don’t want to sort by means of the ID, in all probability we changed the publishing date of a couple of posts and we want them in that order, no longer by means of their identification.
This can be accomplished by means of passing 2 parameters to the ofMany strategy to help with this. The main parameter is the key that we want to filter by means of, and the second is the sorting manner:
morphOne(Submit::magnificence, "postable")->ofMany("published_at", "max");
}
With this in ideas, it’s possible to construct additional complicated family members for this! Consider we’ve this example. We’re asked to generate an inventory of all provide posts throughout the order they’ve been published. The problem arises once we’ve 2 posts with the an identical published_at price and when posts are scheduled to be posted in the future.
To do this, we will transfer the order in which we want the filters to be carried out to the ofMany manner. This way we order by means of published_at, and in the event that they’re the an identical, we order by means of identification. Secondly, we will apply a query function to the ofMany strategy to exclude all posts which could be scheduled for publishing!
hasOne(Submit::magnificence)->ofMany([
'published_at' => 'max',
'id' => 'max',
], function ($query) {
$query->where('published_at', '<', now());
});
}
Polymorphic Many To Many
The polymorphic many-to-many is slightly additional complicated than the usual one. One now not bizarre scenario is having tags apply to additional assets to your software. For example, on TikTok, we now have tags that can be carried out to Motion pictures, Shorts, Stories, and so forth.
The polymorphic many-to-many allows us to have a single table of tags associated with the Motion pictures, Shorts, and Stories.
The table development is discreet:
films
identification – integer
description – string
stories
identification – integer
description – string
taggables
tag_id – integer
taggable_id – integer
taggable_type – string
With the tables ready, we will make the way and use the morphToMany manner. The program accepts the identify of the way magnificence and the ‘dating identify’:
morphToMany(Tag::magnificence, 'taggable');
}
}
And with this, we will merely define the inverse relation. Everyone knows that for every child taste we want to identify the morphedByMany manner:
morphedByMany(Story::magnificence, 'taggable');
}
public function films()
{
return $this->morphedByMany(Video::magnificence, 'taggable');
}
}
And now, after we get a Tag, we will retrieve all films and stories related to that tag!
stories;
$films = $tag->stories;
Optimize Eloquent for Tempo
When running with Laravel’s Eloquent ORM, it’s the most important to understand how to optimize database queries and cut back the time frame and memory it requires to fetch wisdom. A technique to check out that is by means of enforcing caching to your software.
Laravel provides a flexible caching tool that is helping slightly numerous backends, corresponding to Redis, Memcached, and file-based caching. By the use of caching Eloquent query results, you can reduce the number of database queries, making your software faster and additional precious.
Additionally, you can use Laravel’s question builder to create additional complicated queries, further optimizing your software’s potency.
Summary
In conclusion, Eloquent relationships are an impressive function of Laravel that allows developers to easily art work with similar wisdom. From one-to-one to many-to-many relationships, Eloquent provides a simple and intuitive syntax to stipulate and query the ones relationships.
As a Laravel developer, mastering Eloquent relationships can an excellent deal give a boost to your construction workflow and make your code additional atmosphere pleasant and readable. If you happen to’re excited by learning additional about Laravel, Kinsta has slightly numerous belongings available, along with a educational on getting started with Laravel and an article on Laravel developer salaries.
Kinsta supplies managed web site webhosting solutions that make deploying and managing Laravel programs a breeze.
The submit Laravel Eloquent Relationships: An Complex Information seemed first on Kinsta®.
Contents
- 1 What Are Relationships in Eloquent?
- 2 One-To-One Relationship
- 3 One-To-Many Relationship
- 4 Has-One-Of-Many Relationship
- 5 HasOneThrough and HasManyThrough Relationships
- 6 Many-To-Many Relationship
- 7 Polymorphic Relationships
- 8 Polymorphic One To One
- 9 Polymorphic One To Many
- 10 Polymorphic Indisputably one in all Many
- 11 Polymorphic Many To Many
- 12 Optimize Eloquent for Tempo
- 13 Summary
- 14 How to Upload Divi Theme Builder Templates to Your Divi Cloud
- 15 Migrate To Kinsta and Give Your self a Loose 20% Spice up — Subsidized By means of Stats
- 16 The usage of a Fullwidth Format vs Grid in Divi’s Filterable Portfolio Module
0 Comments