Through the years, our services and products at Kinsta have all the time been handled manually by way of the MyKinsta dashboard. On the other hand, with the introduction of the Kinsta API and the continuing release of latest API endpoints, you’ll step up your sport by way of developing a personalized solution to engage with Kinsta services and products. One such manner is making a Slackbot to observe and arrange movements like site introduction.
What You’re Development
This instructional explains learn the way to build a Slackbot (or Slack application) that interacts with the Kinsta API to retrieve wisdom and delivers it as real-time messages to a delegated Slack channel the use of the Slack API Incoming Webhooks.
To reach this, you’ll create a Node.js application with the Specific framework to create a UI for WordPress site introduction and mix it with the Kinsta API. The applying uses a type to collect configuration details on your WordPress site and then sends a real-time exchange regarding the site’s wisdom and details on learn the way to check its operation status to the specified Slack channel.

Should haves
To look at along side this mission, you’ll have the following:
- Elementary knowledge of JavaScript and Node.js
- Node.js type 12 or higher
- npm (Node Bundle Supervisor) installed to your computer
- A Slack workspace
Setting Up the Development Surroundings
To get started, create a brand spanking new record on your application and initialize it with npm:
mkdir my-express-app
cd my-express-app
npm init -y
After operating the npm init -y
command, a brand spanking new package deal.json file could be created in your mission’s record with default values. This file incorporates crucial information about your mission and its dependencies.
Next, arrange the crucial dependencies on your mission. The following dependencies are a very powerful:
- ejs: EJS (Embedded JavaScript) is a templating engine that allows you to generate dynamic HTML content material materials with JavaScript.
- specific: Explicit is a snappy and minimalist web application framework for Node.js. It simplifies building web applications and APIs by way of providing a very powerful choices like routing, middleware enhance, and coping with HTTP requests and responses.
- express-ejs-layouts: Explicit EJS layouts is an extension for Explicit that permits the use of layouts or templates to handle a continuing building all the way through a couple of views.
To position in the ones dependencies, run the command below:
npm arrange ejs explicit express-ejs-layouts
Additionally, you’ll need to arrange the following dev dependencies that can assist you assemble and try your Node.js mission:
- nodemon: A valuable instrument that robotically restarts your Node.js application each time file changes throughout the record is detected, ensuring a streamlined construction workflow.
- dotenv: This zero-dependency module plays a crucial serve as in loading setting variables from a .env file.
To position in the ones dev dependencies, run the command below:
npm arrange -D nodemon dotenv
Once your package deal.json is initialized and all dependencies are installed, create a brand spanking new file, e.g. app.js.
touch app.js
Proper right here’s a default setup on your app.js file, where you import crucial modules and set it to run on a decided on port:
// Import required modules
const explicit = require('explicit');
const app = explicit();
// Organize your routes and middleware proper right here
// ...
// Get began the server to pay attention on the specified port
app.listen(process.env.PORT || 3000, () => {
console.log(`Server is operating on port $`);
});
To run your Node.js application, execute the command:
node app.js
On the other hand, operating an application like this means manually restarting it every time you’re making changes in your mission. To overcome this inconvenience, use nodemon
, which you already installed. Configure it in your package deal.json file by way of creating a script command:
"scripts": {
"dev": "nodemon app.js"
},
Now, run your Node.js application with automatic restarts the use of the following command:
npm run dev
Getting Started With Explicit and EJS Templating
In this instructional, you’re building a Node.js application that can display content material materials on the browser. To reach this, explicit.js is used as your web framework, and EJS (Embedded JavaScript) as your templating engine.
To set EJS as your view engine, add the following line in your app.js file. This may occasionally an increasing number of make it conceivable to run .ejs
data:
// Use EJS for the reason that view engine
app.set('view engine', 'ejs');
Now that Explicit is configured with EJS, define your routes. In web applications, routes unravel how the application responds to different HTTP requests (related to GET or POST) and specify the actions to be taken when a decided on URL is accessed.
For example, create a route that can load a decided on internet web page when an individual navigates to the index internet web page (/
). To try this, use the GET request method.
// Define a route for the homepage
app.get('/', (req, res) => {
// Proper right here, you can specify what to do when any person accesses the homepage
// For example, render an EJS template or send some HTML content material materials
});
Inside the above code, when an individual accesses the index of your application the server will execute the callback function specified as the second parameter. Inside this callback function, you’ll handle the common-sense to render an EJS template or send some HTML content material materials to be displayed on the homepage.
You’ll use the res.render()
solution to render an EJS template or use res.send()
to send simple HTML content material materials.
app.get('/', (req, res) => {
res.send('Hello Global');
});
While you run your application, “Hello Global” could be displayed on the index internet web page.
EJS Templating
This instructional focuses on the common-sense and incorporates starter data, in order that you don’t have to worry about growing templates from scratch. Follow the ones steps to get started:
- Get right to use the template on GitHub to create a brand spanking new repository.
- Check out the selection Include all branches all the way through repository introduction.
- As quickly because the repository is created, clone the mission in your computer the use of Git.
- To get right to use the starter code, switch to the starter-files division in your local repository.
Inside the starter code, we have two primary folders: public and views. The public folder holds all static belongings (CSS data and photographs). They’re added to the template as static data:
// Static data
app.use(explicit.static('/public'));
app.use('/css', explicit.static(__dirname + '/public/css'));
app.use('/footage', explicit.static(__dirname + '/public/footage'));
Inside the views folder, you are going to have the layout.ejs file and two folders: pages and partials. The layout.ejs file holds the full layout of this mission, in order that you don’t have to duplicate some regimen code for all pages. Import the express-ejs-layouts
library into the app.js file, then configure it:
// Import
const expressEjsLayouts = require('express-ejs-layouts');
// Configure
app.use(expressEjsLayouts);
The pages folder incorporates the route data (index.ejs and operation.ejs), while the partials folder incorporates components (header.ejs and footer.ejs). Add them to the layout like this:
Internet web page Builder
While you run your Node.js utility, the UI will load, alternatively you need to be able to upload commonplace sense to this application to send the form wisdom to the Kinsta API and send information about the site to Slack when the operation starts.
Getting Started With Slack Incoming Webhooks
Slack Incoming Webhooks provide a simple strategy to send messages from external applications to Slack. To use Slack Incoming Webhooks, create and configure a Slack application, then copy your Webhook URL for sending messages to Slack programmatically.
Setting Up a Slack App and Obtaining Webhook URL
Create a brand spanking new Slack application by way of following the ones steps:
- Navigate to the Slack API dashboard.
- Click on at the Create New App button, which is in a position to open a modal.
- Make a selection the From Scratch approach to get began building your app from the ground up.
- Provide a name on your Slack app, for example, Kinsta Bot.
- Next, choose the workspace where you want to place within the app and click on at the Create App button.
Once your Slack application is created, you’ll permit incoming Webhooks by way of navigating to Choices and selecting Incoming Webhooks. Toggle the switch to permit Incoming Webhooks on your app.
Scroll all of the method right down to the Webhook URLs for Your Workspace segment and click on on Add New Webhook to Workspace. You’ll be prompted to select a channel where the messages could be sent. Make a selection the desired channel and click on on Authorize.
After authorization, you’ll be provided with a Webhook URL for the selected channel. This URL is what you’ll use to send messages to Slack programmatically. That’s what a Webhook URL turns out like:
https://hooks.slack.com/services and products/T00000000/B00000000/XXXXXXXXXXXXXXXXXXXXXXXX
This Webhook is specific to a single individual and a single channel. Keep it safe, as it acts as an authentication token on your app. You’ll store the unique codes after /services and products/
in your .env file. You’ll also be prompted to reinstall the app in your workspace for the changes to take affect.
Sending Messages to Slack With Node.js and Kinsta API
Now that your Node.js application’s interface is in a position up and the Slackbot has been successfully created (along side your WebHook URL), it’s time to handle the common-sense.
Fetch Form Knowledge in Node.js
On the index internet web page, you are going to have a type that can send wisdom to the Kinsta API to create a brand spanking new WordPress site. To make this art work, you need to create a POST request from the index internet web page. Be sure that your selection has a method POST
, and the input fields have a determine
feature, which could be used throughout the app.js file.
app.post('/', (req, res) => {
// Perform the desired operation with the form wisdom
});
To retrieve wisdom from a type in Node.js, you’ll need to use the following middleware:
app.use(explicit.json());
app.use(explicit.urlencoded({ extended: true }));
Now, you’ll get right to use your selection values the use of req.body.[form field name]
. For example, req.body.displayName
provides you with the display determine submitted at some point of the variability. Let’s log all the selection wisdom:
app.post('/', (req, res) => {
console.log(req.body);
});
While you run your code, the form wisdom could be displayed after you fill out the form and click on at the Post button.

Internet web page Creation With Kinsta API in Node.js
To create a WordPress site with the Kinsta API in Node.js, you’ll use the fetch()
method, which is now supported and performs effectively throughout the newest Node.js variations.
To perform any operation with the Kinsta API, you need to create an API key. To generate an API key:
- Move in your MyKinsta dashboard.
- Navigate to the API Keys internet web page (Your determine > Company settings > API Keys).
- Click on on Create API Key.
- Choose an expiration date or set a custom designed get began date and number of hours for the necessary factor to expire.
- Give the necessary factor a singular determine.
- Click on on Generate.
Take into accout to copy the generated API key and store it securely, because it’ll most simple be visible at this 2nd. For this mission, create a .env file in your root record and save the API key as KINSTA_API_KEY
.
Additionally, to create a WordPress site the use of the Kinsta API, you’ll need your Company ID (which can be found in MyKinsta beneath Company > Billing Details > Company ID). Store this ID throughout the .env file as well, so that you’ll get right to use the ones setting variables through process.env
. To permit this capacity, you should definitely configure the dotenv
dependency at the top of your app.js file like this:
require('dotenv').config();
To proceed with creating a WordPress site at some point of the Kinsta API, send a POST request to the /websites
endpoint, with the crucial wisdom supplied throughout the req.body
object:
const KinstaAPIUrl = 'https://api.kinsta.com/v2';
app.post('/', (req, res) => {
const createSite = async () => {
const resp = stay up for fetch(`${KinstaAPIUrl}/web pages`, {
method: 'POST',
headers: {
'Content material material-Type': 'application/json',
Authorization: `Bearer ${process.env.REACT_APP_KINSTA_API_KEY}`,
},
body: JSON.stringify({
company: process.env.REACT_APP_KINSTA_COMPANY_ID,
display_name: req.body.displayName,
house: req.body.location,
install_mode: 'new',
is_subdomain_multisite: false,
admin_email: req.body.piece of email,
admin_password: req.body.password,
admin_user: req.body.username,
is_multisite: false,
site_title: req.body.siteTitle,
woocommerce: false,
wordpressseo: false,
wp_language: 'en_US',
}),
});
const wisdom = stay up for resp.json();
console.log(wisdom);
};
createSite();
});
By way of operating the code above, you’ll create a brand spanking new WordPress site with Kinsta API. Then again this isn’t the principle serve as. The serve as is to send a message to Slack containing information about the site when the site introduction operation is a luck.
Send a Message to Slack With the Incoming Webhook URL
To try this, create an If remark to check the API request response status. If it is 202
, it manner “site introduction has started” and also you’ll send a message to Slack the use of the incoming Webhooks URL. To reach this, you’ll use your most popular HTTP request library (e.g. Axios) or solution to send a POST request to Slack. Let’s use the fetch()
method:
if (wisdom.status === 202) {
fetch(
`https://hooks.slack.com/services and products/${process.env.SLACK_WEBHOOK_ID}`,
{
method: 'POST',
headers: {
'Content material material-Type': 'application/json',
},
body: JSON.stringify({
text: 'Hello, world.',
}),
}
);
}
Run the code above and fill out the site introduction selection. If the process is a luck, a message could be sent to Slack immediately.

Customizing Slack Messages
The example above sends a basic text message, alternatively Slack Incoming Webhooks enhance much more than simple text. You’ll customize your messages to include attachments, links, footage, buttons, and further.
A method you’ll customize Slack messages is by way of the use of the Slack block Package Builder. The Block Bundle is a UI framework supplied by way of Slack that allows you to assemble rich and interactive messages with somewhat a large number of content material materials portions.
For this instructional, right here’s a block created with the block package deal builder to construction the message accurately and add some values from the form and the site introduction response:
const message = {
blocks: [
{
type: 'section',
text: {
type: 'mrkdwn',
text: `Hello, your new site (${req.body.displayName}) has started building. It takes minutes to build. You can check the operation status intermittently via https://site-builder-nodejs-xvsph.kinsta.app/operation/${req.body.displayName}/${data.operation_id}.`,
},
},
{
type: 'divider',
},
{
type: 'section',
text: {
type: 'mrkdwn',
text: "_Here are your site's details:_",
},
},
{
type: 'section',
text: {
type: 'mrkdwn',
text: `1. *Site URL:* http://${req.body.displayName}.kinsta.cloud/n2. *WP Admin URL:* http://${req.body.displayName}.kinsta.cloud/wp-admin/`,
},
},
],
};
In this code, you create a message object containing an array of blocks. Each block represents a decided on segment of the Slack message and can have different types of content material materials.
- Section Block: This block kind is used to turn a work of text. You use the
kind: 'segment'
to suggest it’s a work block. Inside the segment block, the text property is used withkind: 'mrkdwn'
to specify that the text content material materials will have to be interpreted as Markdown construction. The actual text content material materials is supplied throughout the text property, and we use template literals to include dynamic values from the form and the site introduction response, related toreq.body.displayName
andwisdom.operation_id
. - Divider Block: This block kind is used to be able to upload a horizontal line to separate sections of the message. We use
kind: 'divider'
to create the divider block.
When this message is shipped to Slack the use of the Incoming Webhook, it’ll generate a visually attention-grabbing and informative message in your Slack channel. The message will include dynamic values from the form (identical to the site’s determine) and data from the site introduction response, making it a very customized and custom designed message.
To send this tradition designed message, trade the article throughout the body of the fetch()
with the contents of the message variable:
if (wisdom.status === 202) {
fetch(
`https://hooks.slack.com/services and products/${process.env.SLACK_WEBHOOK_ID}`,
{
method: 'POST',
headers: {
'Content material material-Type': 'application/json',
},
body: JSON.stringify(message),
}
);
}
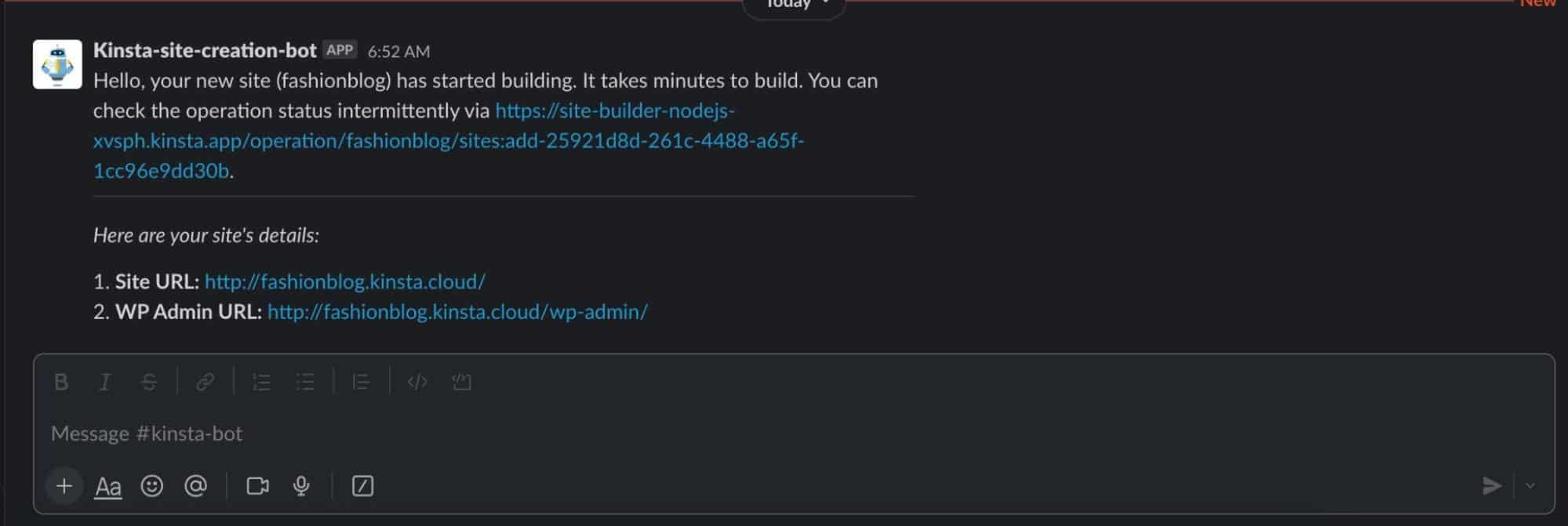
Handle Internet web page Creation Operation With Kinsta API
Inside the message sent to Slack, a link is created that has the operation ID and display determine. You’ll create a brand spanking new route for the Operations internet web page to use this data to check the operation status.
In Explicit, you’ll get right to use URL parameters with the req
parameter. For example, to get the operation ID, you employ req.params.operationId
.
const KinstaAPIUrl = 'https://api.kinsta.com/v2';
app.get('/operation/:displayName/:operationId', (req, res) => {
const checkOperation = async () => {
const operationId = req.params.operationId;
const resp = stay up for fetch(`${KinstaAPIUrl}/operations/${operationId}`, {
method: 'GET',
headers: {
Authorization: `Bearer ${process.env.REACT_APP_KINSTA_API_KEY}`,
},
});
const wisdom = stay up for resp.json();
res.render('pages/operation', {
operationID: req.params.operationId,
displayName: req.params.displayName,
operationMessage: wisdom.message,
});
};
checkOperation();
});
With the code above, clicking the link in Slack will make a request to the Kinsta API to check your site’s operation status. Change the operation.ejs file to be able to upload dynamic wisdom:
Check out Internet web page Operation Status
Check out the status of your site tools operation by way of the id. Be happy to duplicate
the ID and take a look at in few seconds.
<input class="form-control" value="" readOnly />
..
If message above means that "Operation has successfully finished", use
the links below to get right to use your WP admin and the site itself.
<a
href="http://.kinsta.cloud/wp-admin/"
objective="_blank"
rel="noreferrer"
class="detail-link"
>
Open WordPress admin
<a
href="http://.kinsta.cloud/"
objective="_blank"
rel="noreferrer"
class="detail-link"
>
Open URL
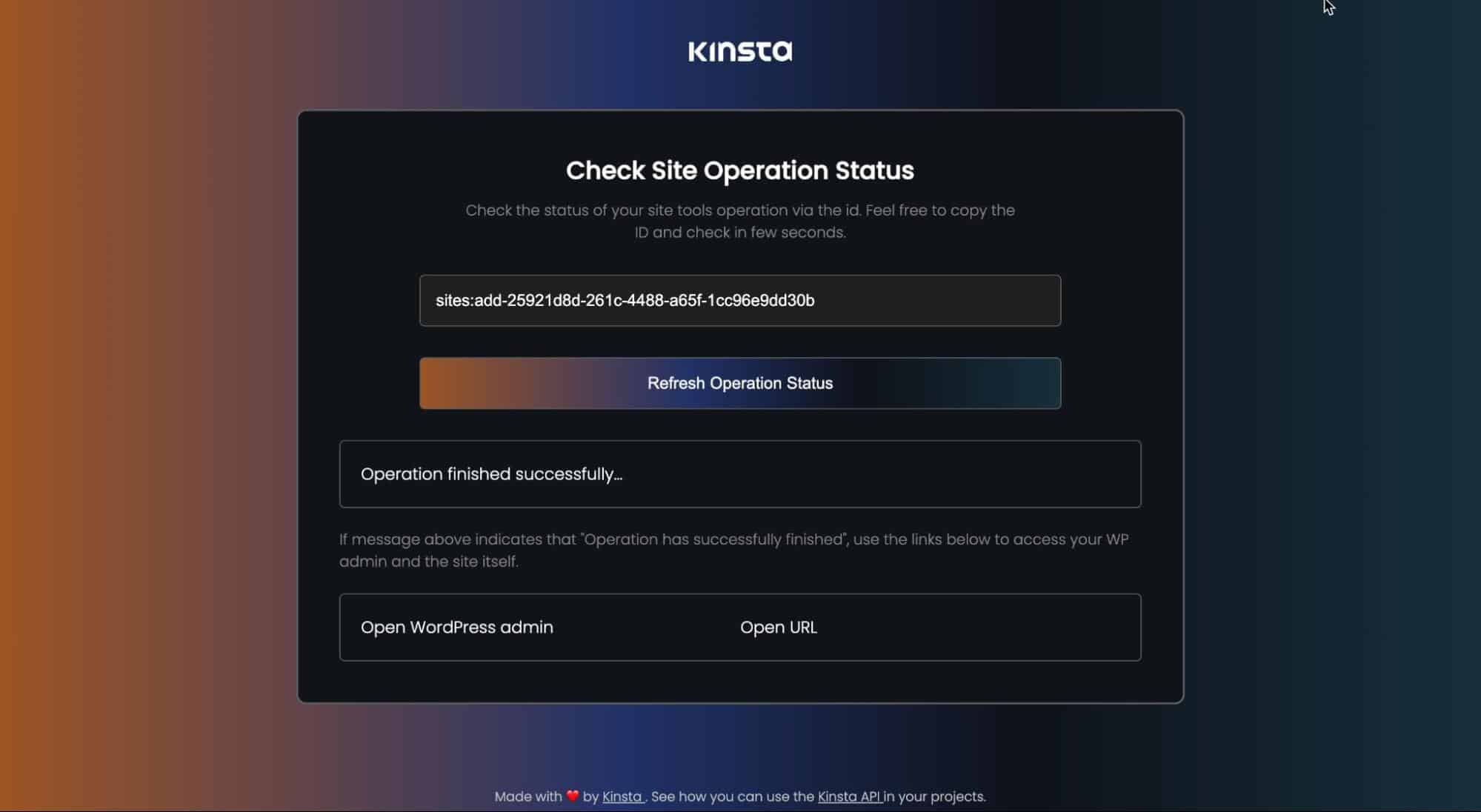
One very last item, you’ll use the redirect solution to navigate to the operations internet web page when a site introduction process starts:
if (wisdom.status === 202) {
fetch(
`https://hooks.slack.com/services and products/${process.env.SLACK_WEBHOOK_ID}`,
{
method: 'POST',
headers: {
'Content material material-Type': 'application/json',
},
body: JSON.stringify(message),
}
);
res.redirect(`/operation/${req.body.displayName}/${wisdom.operation_id}`);
}
The entire provide code for this mission is available on this GitHub repository’s major department.
Deploying Your Node.js Tool to Kinsta
You’ll merely deploy this Node.js application to Kinsta’s Software Webhosting platform. All it’s necessary to do is push your code in your most popular Git provider (Bitbucket, GitHub, or GitLab). Then apply the ones steps:
- Log in in your Kinsta account on the MyKinsta dashboard.
- Click on on Add supplier.
- Make a selection Tool from the dropdown menu.
- Inside the modal that appears, choose the repository you want to deploy. When you have a couple of branches, you’ll make a choice the desired division and gives a name in your application.
- Make a selection one of the most a very powerful available wisdom heart puts. Kinsta will find and arrange your app’s dependencies from package deal.json, then assemble and deploy.
Finally, it’s not safe to push out API keys to public hosts like your Git provider. When internet webhosting, you’ll add them as atmosphere variables the use of the equivalent variable determine and value specified throughout the .env file.
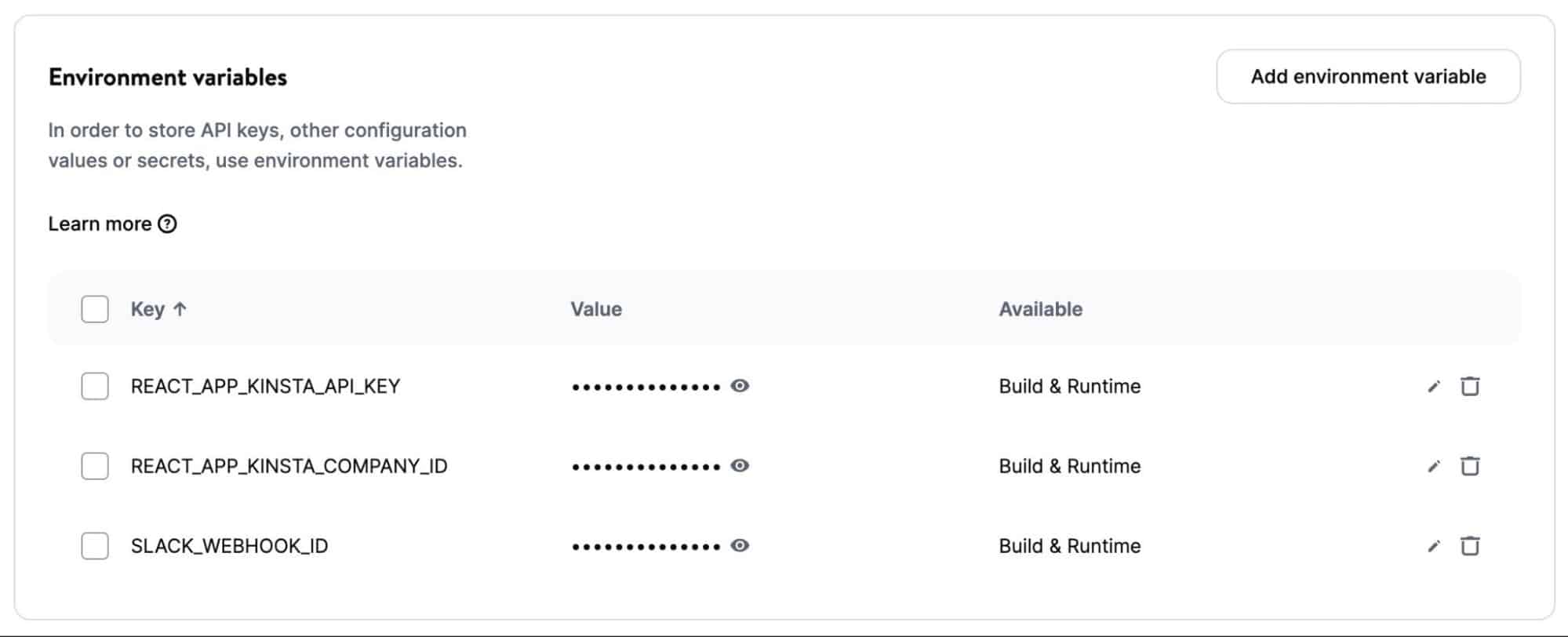
In case you start the deployment of your application, it’ll generally assemble and deploy inside a few minutes. A link in your new application could be supplied, which turns out like this: https://site-builder-nodejs-xvsph.kinsta.app.
Summary
In this instructional, you are going to have found out learn the way to send messages to Slack from a Node.js application the use of Incoming Webhooks and learn the way to customize Slack messages with the Block Bundle Builder.
The probabilities with Slack and the Kinsta API are massive, and this instructional is only the start. By way of integrating the ones tools, you’ll create a continuing workflow that keeps your body of workers well-informed and boosts productivity.
How are you the use of Kinsta API? What choices do you need to see added/exposed next?
The post Managing Kinsta Products and services With Kinsta API and Slack appeared first on Kinsta®.
Contents
- 0.1 What You’re Development
- 0.2 Setting Up the Development Surroundings
- 0.3 Getting Started With Slack Incoming Webhooks
- 0.4 Sending Messages to Slack With Node.js and Kinsta API
- 0.5 Handle Internet web page Creation Operation With Kinsta API
- 1 Check out Internet web page Operation Status
0 Comments