Object-oriented programming (OOP), the most important paradigm in tool, is thinking about “devices” — cases of classes that include wisdom and behaviors instead of “actions.”
PHP, known for server-side scripting, benefits very a lot from OOP. It’s as a result of OPP is helping modular and reusable code, making it easier to maintain. In turn, this facilitates upper staff and scalability of large projects.
Mastering OOP is essential for builders working with WordPress subjects, plugins, and custom designed solutions. In this article, we’ll outline what OOP is in PHP and the impact it has on WordPress construction. We’ll moreover walk you through recommendations on easy methods to put into effect OOP concepts in PHP.
Should haves
To look at the hands-on parts of this text, make sure you have:
- XAMPP (or an an identical) for Apache and MySQL
- A PHP atmosphere in model 7.4 or upper
- A code editor, like Visible Studio Code
- A native or web-hosted WordPress arrange.
- Knowledge of PHP and WordPress
Advantages of OOP in PHP construction
OOP significantly boosts PHP construction thru making improvements to modularity, reusability, scalability, maintainability, and teamwork. It organizes PHP code thru dividing it into devices, every representing a decided on part of the application. Using devices, you’ll be capable to merely reuse code, saving time and reducing errors.
With this in ideas, let’s dive into two specific advantages of OOP in PHP, highlighting how it transforms the development process.
1. Code reusability and maintenance
OOP in PHP makes it simple to reuse code because of inheritance and polymorphism. Classes can use properties and strategies from other classes. This lets you use earlier code in new ways with little industry.
OOP moreover makes it easier to handle your code. Encapsulation method devices keep their details private and most efficient proportion what’s sought after, using specific methods referred to as getters and setters. This fashion helps prevent changes in one part of your tool from causing problems in others, making the code more effective to interchange and maintain.
Moreover, because of devices are complete on their own, finding and fixing bugs in certain parts of the device is easier. This improves general code top quality and reliability.
2. Enhanced clarity and development
OOP makes PHP code cleaner and further organized using classes and devices. Classes act like templates for devices, protective the whole thing that belongs together in one place.
OOP moreover lets classes use choices from other classes, that suggests you don’t have to write down the an identical code again and again. These types of assist in making the code cleaner, easier to fix, and better organized.
Clear code from OOP helps teams art work upper together. It’s easier for everyone to grasp what the code does, that suggests a lot much less time explaining problems and time beyond regulation doing the art work. It moreover reduces mistakes, helping the undertaking stay on the right track. And when code is neat and orderly, new team members can in short catch up.
Enforcing OOP in PHP
In OOP for PHP, you prepare code with classes and devices, like blueprints and houses. You create classes for the whole thing (like shoppers or books), along with their characteristics and actions. Then, you use inheritance to build new classes from provide ones, saving time thru no longer repeating code. And because encapsulation keeps some elegance parts private, your code is extra safe.
The following sections walk you through recommendations on easy methods to use OOP concepts effectively in your PHP programming. We create a content material control gadget (CMS) for managing articles.
1. Define a class with properties and strategies
Get began with an Article
elegance that incorporates title, content material subject material, and status properties — along with set and display the ones properties.
elegance Article {
private $title;
private $content material subject material;
private $status;
const STATUS_PUBLISHED = 'published';
const STATUS_DRAFT = 'draft';
public function __construct($title, $content material subject material) {
$this->title = $title;
$this->content material subject material = $content material subject material;
$this->status = self::STATUS_DRAFT;
}
public function setTitle($title) {
$this->title = $title;
return $this;
}
public function setContent($content material subject material) {
$this->content material subject material = $content material subject material;
return $this;
}
public function setStatus($status) {
$this->status = $status;
return $this;
}
public function display() {
echo "{$this->title}
{$this->content material subject material}
Status: {$this->status}";
}
}
2. Create devices and put into effect method chaining
Create a piece of writing object and use way chaining to set its properties:
$article = new Article("OOP in PHP", "Object-Oriented Programming concepts.");
$article->setTitle("Sophisticated OOP in PHP")->setContent("Exploring complicated concepts in OOP.")->setStatus(Article::STATUS_PUBLISHED)->display();
3. Support encapsulation and inheritance
Support encapsulation thru using getter and setter methods and create a FeaturedArticle
elegance that inherits from Article
:
elegance FeaturedArticle extends Article {
private $highlightColor = '#FFFF00'; // Default highlight color
public function setHighlightColor($color) {
$this->highlightColor = $color;
return $this;
}
public function display() {
echo " style='background-color: {$this->highlightColor};'>";
father or mom::display();
echo "";
}
}
$featuredArticle = new FeaturedArticle("Featured Article", "This can be a featured article.");
$featuredArticle->setStatus(FeaturedArticle::STATUS_PUBLISHED)->setHighlightColor('#FFA07A')->display();
4. Interfaces and polymorphism
Define an interface for publishable content material subject material and put into effect it throughout the Article
elegance to show polymorphism:
interface Publishable {
public function publish();
}
elegance Article implements Publishable {
// Provide elegance code...
public function publish() {
$this->setStatus(self::STATUS_PUBLISHED);
echo "Article '{$this->title}' published.";
}
}
function publishContent(Publishable $content material subject material) {
$content->publish();
}
publishContent($article);
PHP implies that you’ll be able to use traits with the intention to upload functions to classes without having to inherit from some other elegance. Using the code underneath, introduce a trait for logging movements all the way through the CMS:
trait Logger {
public function log($message) {
// Log message to a report or database
echo "Log: $message";
}
}
elegance Article {
use Logger;
// Provide elegance code...
public function publish() {
$this->setStatus(self::STATUS_PUBLISHED);
$this->log("Article '{$this->title}' published.");
}
}
OOP in WordPress construction
OOP concepts significantly toughen WordPress building, particularly when creating subjects, plugins, and widgets. With the help of OOP, you’ll be capable to write cleaner, scalable, and further maintainable code in your WordPress internet sites.
This segment reviews recommendations on easy methods to practice OOP in WordPress construction. We’ll provide examples you’ll be capable to replica and paste proper right into a WordPress deployment for testing.
OOP in WordPress subjects: Custom designed post kind registration
To expose using OOP in WordPress topics, you create a class that handles the registration of a custom designed post kind.
Place the following code in your theme’s functions.php report. You’ll to search out your subjects throughout the wp-content/subjects record.
elegance CustomPostTypeRegistrar {
private $postType;
private $args;
public function __construct($postType, $args = []) {
$this->postType = $postType;
$this->args = $args;
add_action('init', array($this, 'registerPostType'));
}
public function registerPostType() {
register_post_type($this->postType, $this->args);
}
}
// Usage
$bookArgs = [
'public' => true,
'label' => 'Books',
'supports' => ['title', 'editor', 'thumbnail'],
'has_archive' => true,
];
new CustomPostTypeRegistrar('ebook', $bookArgs);
This code dynamically registers a custom designed post kind ebook
with its details passed through using the bookArgs
array. You’ll see the newly created custom designed post kind throughout the WordPress admin sidebar categorized Books.
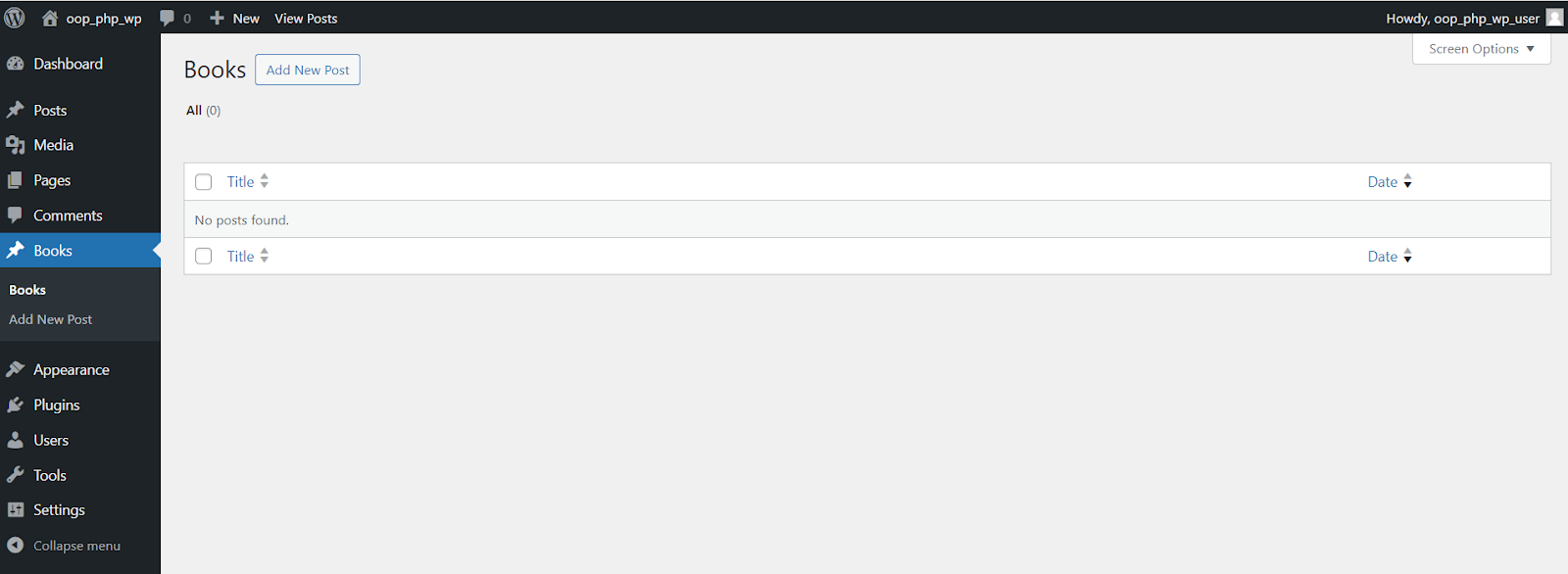
This example illustrates how OOP can encapsulate the potential for registering custom designed post types, making it reusable for various kinds of posts.
OOP in WordPress plugins: Shortcode handler
For a plugin example, you make bigger a class that handles a shortcode for appearing a singular message. You’ll check out this feature thru together with the shortcode underneath to any post or internet web page.
'Hello, this is your OOP
message!'], $atts);
return "{$attributes['message']}";
}
}
new OOPShortcodeHandler();
Save this as my-oop-shortcode-handler.php throughout the wp-content/plugins record. In any case, flip at the plugin.
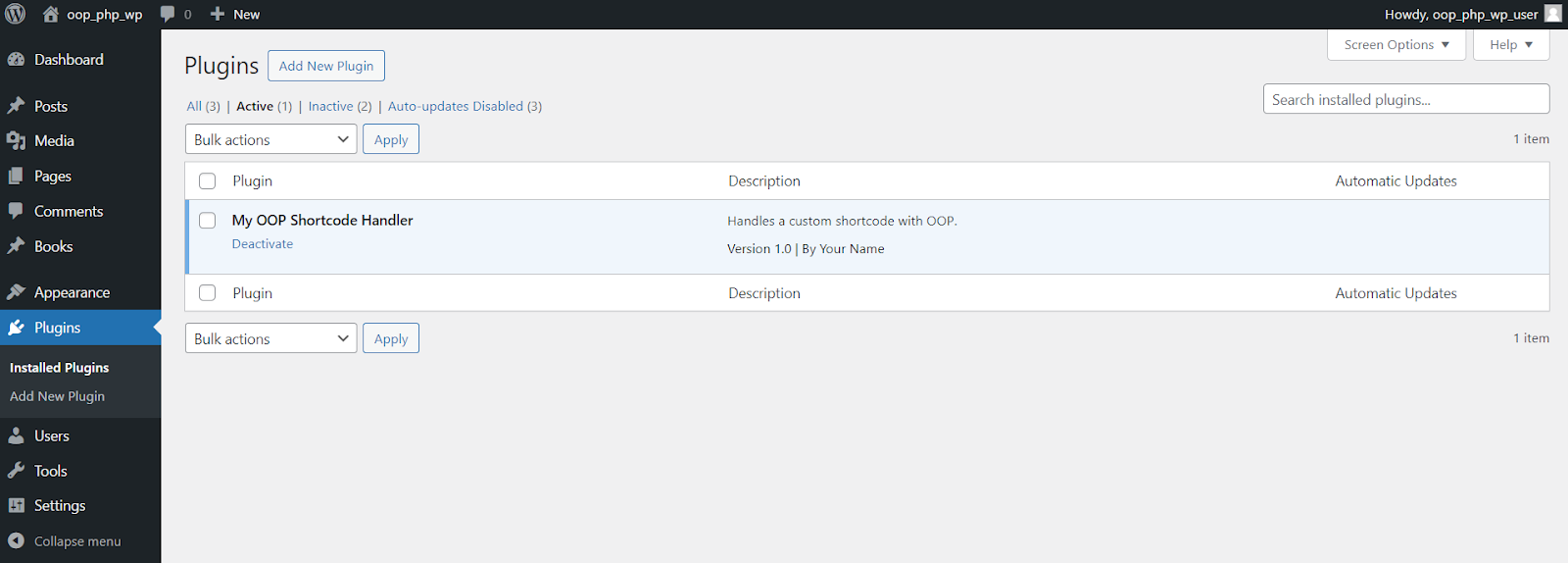
Next, throughout the internet web page or post editor quicker than publishing or updating, use the shortcodes [oop_message]
and [oop_message message="Custom Message Here"]
, as confirmed underneath:
After publishing or updating the internet web page/post, you understand the message that the shortcodes displayed denote.
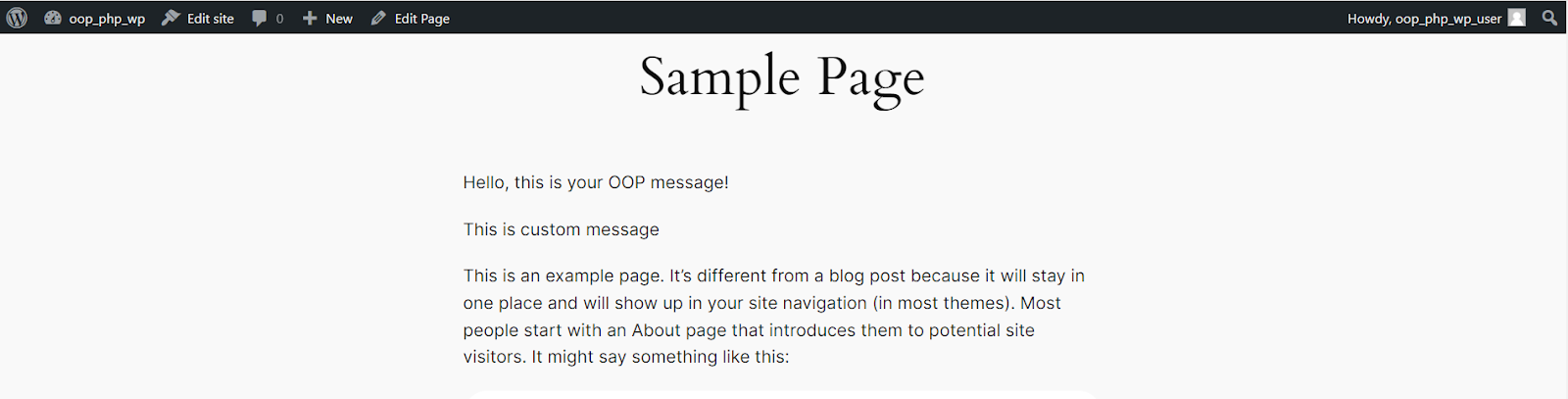
OOP in WordPress widgets: dynamic content material subject material widget
OOP could also be beneficial for widgets thru encapsulating their capacity within classes. WordPress core itself uses OOP for widgets. Proper right here, you create a custom designed widget that allows shoppers to turn dynamic content material subject material with a reputation and text area.
Add the following code in your theme’s functions.php report or within a plugin. It defines a custom designed widget that displays the message “Hello Global From My Custom designed Widget!”
elegance My_Custom_Widget extends WP_Widget {
public function __construct() {
father or mom::__construct(
'my_custom_widget', // Base ID
'My Custom designed Widget', // Establish
array('description' => __('A simple custom designed widget.',
'text_domain'),) // Args
);
}
public function widget($args, $instance) {
echo $args['before_widget'];
if (!empty($instance['title'])) {
echo $args['before_title'] . apply_filters('widget_title',
$instance['title']) . $args['after_title'];
}
// Widget content material subject material
echo __('Hello Global From My Custom designed Widget!', 'text_domain');
echo $args['after_widget'];
}
public function form($instance) {
// Form in WordPress admin
}
public function exchange($new_instance, $old_instance) {
// Processes widget possible choices to be saved
}
}
function register_my_custom_widget() {
register_widget('My_Custom_Widget');
}
add_action('widgets_init', 'register_my_custom_widget');
While enhancing the energetic theme using the Customize link underneath the Glance on Admin area, you’ll be capable to add a brand spanking new custom designed widget any place sought after.
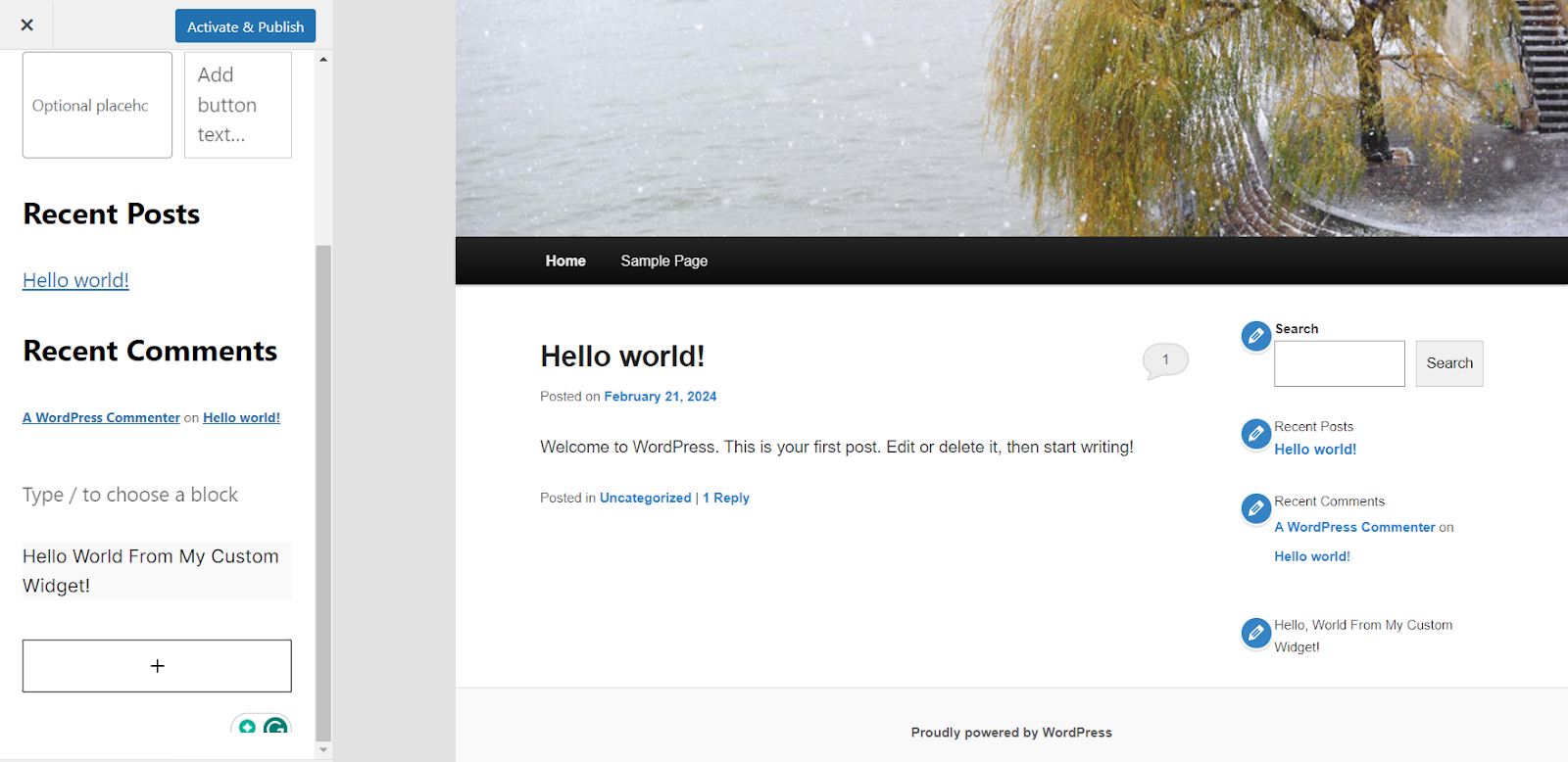
The usage of WordPress classes
WordPress provides somewhat a large number of classes that you just’ll be capable to use to have interaction with the CMS’s core capacity. Two such classes are WP_User
and WP_Post
, which represent shoppers and posts, respectively.
Extending the WordPress plugin example from above, incorporate the ones classes to create a shortcode that displays information about the writer of a post and details about the post itself.
Save this as my-oop-shortcode-handler-extended.php throughout the wp-content/plugins record. In any case, flip at the plugin.
$post->ID, // Default to the current post ID
], $atts);
$postDetails = get_post($attributes['post_id']); // Getting the WP_Post
object
if (!$postDetails) {
return "Publish no longer found out.";
}
$authorDetails = new WP_User($postDetails->post_author); // Getting the
WP_User object
$output = "
";
return $output;
}
}
new ExtendedOOPShortcodeHandler();
In this extended style, you’ve created a shortcode: [post_author_details post_id="1"]
.
When added to a post or internet web page, it displays details about the post’s writer (using the WP_User
elegance) and the post itself (using the WP_Post
elegance).
OOP and the WordPress REST API
The WordPress REST API is a modern addition to WordPress that allows you to engage with the internet web site’s wisdom in a additional programmable approach. It leverages OOP widely, the usage of classes to stipulate the endpoints, responses, and the coping with of requests. This represents a clear switch from its typical procedural roots to embracing OOP.
Contrasting sharply with OOP concepts, a large number of WordPress’s core, specifically its earlier components harking back to the topics and plugins API, is written in a procedural programming style.
As an example, while a procedural approach would in all probability at once manipulate international variables and rely on a sequence of functions for tasks, OOP throughout the REST API encapsulates the nice judgment within classes. Because of this truth, specific methods within the ones classes maintain tasks harking back to fetching, creating, updating, or deleting posts.
This clearly separates problems and makes the codebase easier to extend and debug.
Via classes that define endpoints and maintain requests, harking back to fetching posts with a GET /wp-json/wp/v2/posts
request, the REST API provides a structured, scalable option to engage with WordPress wisdom, returning JSON formatted responses.
Leveraging Kinsta for PHP and WordPress web website hosting
Kinsta’s internet hosting answers help optimize PHP methods and prepare WordPress web website hosting, catering to straightforward PHP setups and WordPress-specific needs. With a robust infrastructure built on cutting-edge technologies like Google Cloud, Kinsta facilitates peculiar potency and scalability for OOP-based PHP methods.
For WordPress internet sites, Kinsta’s specialized WordPress Internet hosting supplier provides choices like automatic updates, protection monitoring, and professional toughen. It guarantees hassle-free keep an eye on and superior potency, making it preferably suited to developers and firms alike.
Summary
As you’ve seen throughout this text, OOP provides peculiar flexibility, scalability, and maintainability in PHP and WordPress construction.
As a primary managed WordPress web website hosting provider, Kinsta recognizes this need and offers tailored solutions to toughen developers like you in your OOP PHP endeavors. That’s why our Utility and WordPress Web website hosting solutions are designed that will help you in construction performant, scalable, OOP-based WordPress projects.
Check out Kinsta nowadays to seem how we will assist you to assemble top-tier WordPress internet sites!
Have you ever ever professional some great benefits of OOP in your projects? Or are you curious about how it can develop into your construction process? Share throughout the comments underneath.
The post Object-oriented programming in PHP: reworking WordPress building seemed first on Kinsta®.
Contents
- 1 Should haves
- 2 Advantages of OOP in PHP construction
- 3 Enforcing OOP in PHP
- 4 {$this->title}
- 5 OOP in WordPress construction
- 6 The usage of WordPress classes
- 7 Writer Wisdom
- 8 Publish Wisdom
- 9 Leveraging Kinsta for PHP and WordPress web website hosting
- 10 Summary
- 11 Pictory AI: A Thorough Review (2023)
- 12 The best way to Prohibit the Selection of Posts in WordPress RSS Feed
- 13 How to Customize WordPress in 2024 (No Coding Required)
0 Comments