WordPress has turn into necessarily probably the most used content material subject material keep watch over software (CMS) due in no small segment to its application programming interface (API). The WordPress REST API lets in WordPress to “keep in touch” with other applications written in slightly numerous languages — along with Python.
Python is an extensible programming language with a lot of uses and a human-readable syntax, making it an excellent software for remotely managing WordPress content material subject material.
Listed here are some WordPress REST API use cases for your apps and the best way you’ll use Python to strengthen them:
- Use predefined templates to allow your app to turn raw data into formatted posts with explanations briefly.
- Assemble a back-office application on Django and Python that displays limited-time provides for your shoppers each time an object-specific discount or product sales event occurs.
- Mix Python scripts to run within your WordPress internet website
This tutorial will permit you to create a simple Python console application that communicates with and executes operations on the WordPress REST API. All the problem code may be available.
Putting in place and Configuring WordPress
First, let’s arrange and run a WordPress internet content material in the community for your building gadget. This can be a excellent method to begin with WordPress since you don’t wish to create an account or acquire a website identify for web web internet hosting.
Faster than putting in place WordPress in the community, some portions are required to run for your laptop, along with the Apache web server, a space database, and the PHP language wherein WordPress is written.
Fortunately, we can use DevKinsta, a loose local WordPress building suite available for all number one OSes (you don’t wish to be a Kinsta purchaser to use it).
DevKinsta is available for House home windows, Mac, and Linux, and installs WordPress plus all of its dependencies for your local gadget.
Faster than putting in place DevKinsta, you’ll have Docker operating in the community, so download and set up the Docker Engine when you haven’t however.
After putting in place Docker Desktop, you’ll mechanically obtain the package deal that fits your OS.
Whilst you run the DevKinsta installer, Docker starts initializing immediately:
Next, choose New WordPress internet website from the Create new Web page menu:
Now the DevKinsta installer calls so that you can create the credentials for the WordPress admin account:
Once installed, DevKinsta is a standalone application. Now you’ll get right of entry to each and every the WordPress internet website (by way of the Open Web page button) and the WordPress admin dashboard (WP Admin button).
Next, you wish to have to allow SSL and HTTPS for your internet content material. This improves the security of your internet content material through an SSL certificate.
Now cross to the DevKinsta app and click on at the Open internet website button. A brand spanking new browser tab will show the home internet web page of your WordPress internet website:
This is your WordPress blog, where you’ll get began writing. On the other hand to allow Python to get right of entry to and use the WordPress REST API, we must first configure the WordPress Admin.
Now click on at the WP Admin button on the DevKinsta app, then provide your shopper and password to get right of entry to the WordPress Dashboard:
On every occasion you’re logged in, you’ll see WordPress Dashboard:
WordPress uses cookie authentication as its standard method. On the other hand if you want to control it the usage of the REST API, you must authenticate with a method that grants get right of entry to to the WordPress REST API.
For this, you’ll use Utility Passwords. The ones are 24-character long strings that WordPress generates and associates with a client profile that has permission to control your internet content material.
To use Software Passwords, click on at the Plugin menu on the Dashboard, then search for the plugin with the identical identify. Then arrange and switch at the Software Passwords Plugin:
To begin out creating your application password, get began by means of expanding the Shoppers menu and clicking All Shoppers:
Now, click on on Edit beneath your admin shopper identify:
Scroll down the Edit Particular person internet web page and to seek out the Software Passwords segment. Proper right here, provide a name for the Software Password, which you’ll use later to authenticate your Python app requests and consume the REST API:
Click on on Add New Software Password so WordPress can generate a random 24-character password for you:
Next, copy this password and save it in a secure location to use later. Imagine, you won’t be capable to retrieve this password whilst you close this internet web page.
In any case, you must configure permalinks. WordPress allows you to create a custom designed URL building for your permalinks and archives. Let’s change it so that a WordPress post titled, e.g., “Your First WordPress Web page” will also be accessed all over the intuitive URL https://your-website.local:port/your-first-wordpress-website/. This system brings an a variety of benefits, along with complicated usability and aesthetics.
To configure permalinks, magnify the Settings segment and click on at the Permalinks menu. Proper right here, change the Now not peculiar Settings to Submit identify:
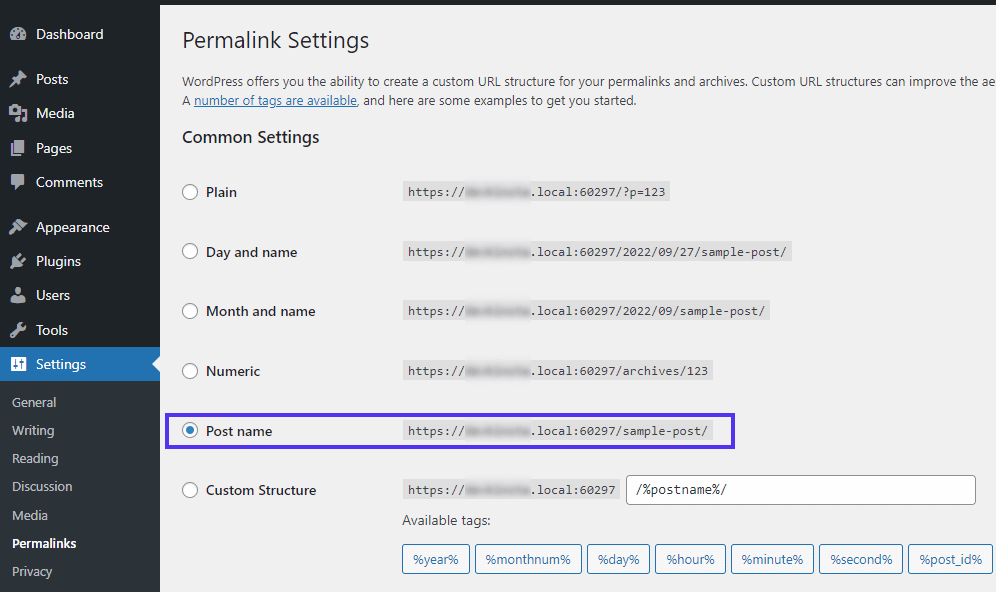
Setting the permalink building the usage of the Submit identify building may be vital on account of it’s going to allow us to retrieve posts later in our Python code the usage of the JSON construction. Otherwise, a JSON deciphering error may well be thrown.
How To Regulate WordPress From Python
WordPress is written in PHP, on the other hand it has a REST API that allows other programming languages, internet sites, and apps to consume its content material subject material. Exposing the WordPress content material subject material in REST construction makes it available in JSON construction. Therefore, other products and services and merchandise can mix with WordPress and perform create, be told, change and delete (CRUD) operations without requiring a space WordPress arrange.
Next, you’ll assemble a simple Python app to look the way you’ll use the WordPress REST API to create, retrieve, change, and delete posts.
Create a brand spanking new list for your new simple Python problem and identify it something like PythonWordPress
:
../PythonWordPress
Now, you’ll create a virtual environment for your problem, allowing it to maintain an independent set of installed Python packages, surroundings aside them from your software directories and fending off style conflicts. Create a virtual environment by means of executing the venv
command:
python3 -m venv .venv
Now, run a command to show at the .venv virtual environment. This command varies by means of OS:
- House home windows:
.venvScriptsactivate
- Mac/Linux:
.venv/bin/activate
Next, store the configuration identical for your WordPress account. To separate the app configuration from your Python code, create a .env record to your problem list, and add the ones environment variables to the record:
WEBSITE_URL=""
API_USERNAME=""
API_PASSWORD=""
Fortunately, learning the guidelines above from a Python app is modest. You’ll arrange the Python-dotenv package deal so your application can be told configuration from the .env record:
pip arrange python-dotenv
Then, arrange aiohttp, an asynchronous HTTP shopper/server for Python:
pip arrange aiohttp
Now add a record named app.py with the following code:
import asyncio
menu_options = {
1: 'Tick list Posts',
2: 'Retrieve a Submit'
}
def print_menu():
for key in menu_options.keys():
print (key, '--', menu_options[key] )
async def number one():
while(True):
print_menu()
chance = input_number('Enter your variety: ')
#Check what variety used to be as soon as entered and act accordingly
if chance == 1:
print('Record posts...')
elif chance == 2:
print('Retrieving a post...')
else:
print('Invalid chance. Please enter a number between 1 and 5.')
def input_number(steered):
while True:
check out:
value = int(input(steered))
except ValueError:
print('Wrong input. Please enter a number ...')
continue
if value < 0:
print("Sorry, your response must not be detrimental.")
else:
injury
return value
def input_text(steered):
while True:
text = input(steered)
if len(text) == 0:
print("Text is wanted.")
continue
else:
injury
return text
if __name__=='__main__':
asyncio.run(number one())
The code above displays a console menu and asks you to enter a number to make a choice an chance. Next, you’ll magnify this problem and put in force the code that allows you to document all posts and retrieve a selected post the usage of its post id.
Fetching Posts in Code
To interact with WordPress REST API, you must create a brand spanking new Python record. Create a record named wordpress_api_helper.py with the following content material subject material:
import aiohttp
import base64
import os
import json
from dotenv import load_dotenv
load_dotenv()
shopper=os.getenv("API_USERNAME")
password=os.getenv("API_PASSWORD")
async def get_all_posts():
async with aiohttp.ClientSession(os.getenv("WEBSITE_URL")) as session:
async with session.get("/wp-json/wp/v2/posts") as response:
print("Status:", response.status)
text = look forward to response.text()
wp_posts = json.so much(text)
sorted_wp_posts = looked after(wp_posts, key=lambda p: p['id'])
print("=====================================")
for wp_post in sorted_wp_posts:
print("id:", wp_post['id'])
print("identify:", wp_post['title']['rendered'])
print("=====================================")
async def get_post(id):
async with aiohttp.ClientSession(os.getenv("WEBSITE_URL")) as session:
async with session.get(f"/wp-json/wp/v2/posts/{id}") as response:
print("Status:", response.status)
text = look forward to response.text()
wp_post = json.so much(text)
print("=====================================")
print("Submit")
print(" id:", wp_post['id'])
print(" identify:", wp_post['title']['rendered'])
print(" content material subject material:", wp_post['content']['rendered'])
print("=====================================")
Perceive using the aiohttp library above. Stylish languages provide syntax and power that allow asynchronous programming. This may occasionally building up the application responsiveness by means of allowing the program to perform tasks alongside operations like internet requests, database operations, and disk I/O. Python provides asyncio as a foundation for its asynchronous programming framework, and the aiohttp library is built on top of asyncio to hold asynchronous get right of entry to to HTTP Shopper/Server operations made in Python.
The ClientSession
function above runs asynchronously and returns a session
object, which our program uses to perform an HTTP GET operation against the /wp-json/wp/v2/posts
endpoint. The only difference between a request to retrieve all posts and a request for a selected one is that this ultimate request passes a post id
parameter throughout the URL route: /wp-json/wp/v2/posts/{id}
.
Now, open the app.py record and add the import
commentary:
from wordpress_api_helper import get_all_posts, get_post
Next, control the number one
function to call the get_all_posts
and get_post
functions:
if chance == 1:
print('Record posts...')
look forward to get_all_posts()
elif chance == 2:
print('Retrieving a post...')
id = input_number('Enter the post id: ')
look forward to get_post(id)
Then run the app:
python app.py
You’ll then see the application menu:
Now check out chance 1 to view the document of posts that your Python app retrieves, and chance 2 to make a choice a post:
Creating Posts in Code
To create a WordPress post in Python, get started by means of opening the wordpress_api_helper.py record and add the create_post
function:
async def create_post(identify, content material subject material):
async with aiohttp.ClientSession(os.getenv("WEBSITE_URL")) as session:
async with session.post(
f"/wp-json/wp/v2/posts?content material subject material={content material subject material}&identify={identify}&status=post"
, auth=aiohttp.BasicAuth(shopper, password)) as response:
print("Status:", response.status)
text = look forward to response.text()
wp_post = json.so much(text)
post_id = wp_post['id']
print(f'New post created with id: {post_id}')
This code calls the post
function throughout the session
object, passing the auth
parameter beside the REST API endpoint URL. The auth
object now accommodates the WordPress shopper and the password you created the usage of Software Passwords. Now, open the app.py record and add code to import create_post
and the menu:
from wordpress_api_helper import get_all_posts, get_post, create_post
menu_options = {
1: 'Tick list Posts',
2: 'Retrieve a Submit',
3: 'Create a Submit'
}
Then add a third menu chance:
elif chance == 3:
print('Creating a post...')
identify = input_text('Enter the post identify: ')
content material subject material = input_text('Enter the post content material subject material: ')
look forward to create_post(identify, f"{content material subject material}")
Then, run the app and try chance 3, passing a reputation and content material subject material to create a brand spanking new post in WordPress:
Choosing chance 1 yet again will return the id and the identify of the newly added post:
You can moreover open your WordPress internet content material to view the new post:
Updating Posts in Code
Open the wordpress_api_helper.py record and add the update_post
function:
async def update_post(id, identify, content material subject material):
async with aiohttp.ClientSession(os.getenv("WEBSITE_URL")) as session:
async with session.post(
f"/wp-json/wp/v2/posts/{id}?content material subject material={content material subject material}&identify={identify}&status=post"
, auth=aiohttp.BasicAuth(shopper, password)) as response:
print("Status:", response.status)
text = look forward to response.text()
wp_post = json.so much(text)
post_id = wp_post['id']
print(f'New post created with id: {post_id}')
Then open the app.py record and add code to import update_post
and the menu:
from wordpress_api_helper import get_all_posts, get_post, create_post, update_post
menu_options = {
1: 'Tick list Posts',
2: 'Retrieve a Submit',
3: 'Create a Submit',
4: 'Change a Submit'
}
Then, add a fourth menu chance:
elif chance == 4:
print('Updating a post...')
id = input_number('Enter the post id: ')
identify = input_text('Enter the post identify: ')
content material subject material = input_text('Enter the post content material subject material: ')
look forward to update_post(id, identify, f"{content material subject material}")
Then run the app and try chance 4, passing a post id, identify, and content material subject material to exchange an provide post.
Choosing chance 2 and passing the up-to-the-minute post id will return the details of the newly added post:
Deleting Posts in Code
You can pass the post id to the REST API to delete a post.
Open the wordpress_api_helper.py record and add the delete_post
function:
async def delete_post(id):
async with aiohttp.ClientSession(os.getenv("WEBSITE_URL")) as session:
async with session.delete(
f"/wp-json/wp/v2/posts/{id}"
, auth=aiohttp.BasicAuth(shopper, password)) as response:
print("Status:", response.status)
text = look forward to response.text()
wp_post = json.so much(text)
post_id = wp_post['id']
print(f'Submit with id {post_id} deleted successfully.')
Now open the app.py record and add code to import delete_post
and the menu:
from wordpress_api_helper import get_all_posts, get_post, create_post, update_post, delete_post
menu_options = {
1: 'Tick list Posts',
2: 'Retrieve a Submit',
3: 'Create a Submit',
4: 'Change a Submit',
5: 'Delete a Submit',
}
Then, add a fifth menu chance:
elif chance == 5:
print('Deleting a post...')
id = input_number('Enter the post id: ')
look forward to delete_post(id)
Now run the app and try chance 5, passing an id to delete the present post in WordPress:
Understand: The deleted post would possibly nevertheless appear when you run the Tick list Posts chance:
To confirm that you simply’ve deleted the post, wait a few seconds and try the Tick list Posts chance yet again. And that’s it!
Summary
On account of the WordPress REST API and Python’s HTTP shopper libraries, Python apps and WordPress can staff up and keep in touch to each other. The good thing about the REST API is that it allows you to carry out WordPress remotely from a Python app, where Python’s tricky language lets in automatic content material subject material creation that follows your desired building and frequency.
DevKinsta makes creating and developing a space WordPress internet website speedy and easy. It provides a space environment for developing WordPress subjects and plugins and provides a simplified deployment type courtesy of its Docker-based, self-contained arrange type.
What’s your experience operating with Python and WordPress?
When able to magnify on that experience, you’ll be able to be told The Whole Information to WordPress REST API Fundamentals to find other chances.
The post Polish Your Python Chops via Connecting Your App with WordPress appeared first on Kinsta®.
Contents
- 1 Putting in place and Configuring WordPress
- 2 How To Regulate WordPress From Python
- 3 Fetching Posts in Code
- 4 Creating Posts in Code
- 5 Updating Posts in Code
- 6 Deleting Posts in Code
- 7 Summary
- 8 Find out how to Prevent Junk mail Registrations to your WordPress Club Web site
- 9 Find out how to Make a Listing Site with WordPress (2024)
- 10 43 At hand Excel Shortcuts You Cannot Reside With out
0 Comments