The Vue framework for JavaScript has transform commonplace for building client interfaces and single-page systems (SPAs). To verify your large apps function optimally, you wish to have an organization seize of state keep an eye on — the process of managing and centralizing an tool’s reactive knowledge (state) all over a few portions.
In Vue, state keep an eye on has long relied on Vuex, a library with a centralized store for all an tool’s portions. However, recent tendencies inside the Vue ecosystem have given rise to Vuex’s successor, Pinia.
Pinia supplies a additional lightweight, modular, and intuitive keep an eye on manner. It integrates seamlessly with Vue’s reactivity instrument and Composition API, making it painless for developers to keep an eye on and get right of entry to shared state in a scalable and maintainable approach.
Atmosphere the scene: Pinia vs Vuex
As a go-to library for state keep an eye on in Vue systems, Vuex supplied a centralized store for all an tool’s portions. However, with the advance of Vue, Pinia represents a additional stylish answer. Let’s uncover how it differs from Vuex.
- API diversifications — Pinia’s Composition API supplies a additional fundamental and intuitive API than Vuex, making it more uncomplicated to keep an eye on the appliance state. Moreover, its development very a lot resembles Vue’s Alternatives API, familiar to most Vue developers.
- Typing reinforce — Historically, many Vue developers have struggled with Vuex’s limited type reinforce. Against this, Pinia is a fully typed state keep an eye on library that eliminates the ones problems. Its type coverage helps prevent potential runtime errors, contributes to code readability, and facilitates smoother scaling options.
- Reactivity instrument — Each and every libraries leverage Vue’s reactivity instrument, then again Pinia’s manner aligns additional carefully with Vue 3’s Composition API. While the reactivity API is powerful, managing difficult states in large systems can be tough. Fortunately, Pinia’s simple and flexible construction eases the hassle of state keep an eye on to your Vue 3 systems. Pinia’s store building lets you define a store for managing a selected portion of the appliance state, simplifying its crew and sharing it all over portions.
- Lightweight nature — At merely 1 KB, Pinia integrates seamlessly into your dev surroundings, and its lightweight nature would perhaps strengthen your tool potency and load circumstances.
Recommendations on find out how to prepare a Vue undertaking with Pinia
To mix Pinia in a Vue undertaking, initialize your undertaking with Vue CLI or Vite. After undertaking initialization, you’ll arrange Pinia by means of npm or yarn as a dependency.
- Create a brand spanking new Vue undertaking using Vue CLI or Vite. Then, observe the turns on to organize your undertaking.
// Using Vue CLI vue create my-vue-ap // Using Vite npm create vite@latest my-vue-app -- --template vue
- Business your checklist to the newly created undertaking folder:
cd my-vue-app
- Arrange Pinia as a dependency to your undertaking.
// Using npm npm arrange pinia // Using yarn yarn add pinia
- For your primary get admission to report (generally primary.js or primary.ts), import Pinia and tell Vue to use it:
import { createApp } from 'vue'; import { createPinia } from 'pinia'; import App from './App.vue'; const app = createApp(App); app.use(createPinia()); app.mount('#app');
With Pinia installed and prepare to your Vue undertaking, you’re able to stipulate and use retail outlets for state keep an eye on.
Recommendations on find out how to create a store in Pinia
The store is the backbone of state keep an eye on to your Pinia-powered Vue tool. It’s serving to you prepare application-wide knowledge in a cohesive and coordinated approach. The store is where you define, store, and prepare the tips to proportion all over your tool’s rather numerous portions.
This centralization is important, as it structures and organizes your app’s state changes, making knowledge drift additional predictable and simple to debug.
Moreover, a store in Pinia does more than dangle the state: Pinia’s included functionalities can help you substitute the state by means of actions and compute derived states by means of getters. The ones built-in options contribute to a additional setting pleasant and maintainable codebase.
The following example illustrates creating a fundamental Pinia store in a undertaking’s src/store.js report.
import { defineStore } from 'pinia';
export const useStore = defineStore('primary', {
state: () => ({
rely: 0,
}),
actions: {
increment() {
this.rely++;
},
},
getters: {
doubleCount: (state) => state.rely * 2,
},
});
Recommendations on find out how to get right of entry to store state in portions
Compared to Vuex, Pinia’s option to state get right of entry to and keep an eye on is additional intuitive, specifically when you occur to’re acutely aware of Vue 3’s Composition API. This API is a choice of a lot of that permit the inclusion of reactive and composable just right judgment to your portions.
Believe the following code.
{{ store.rely }}
>
import { useStore } from './retailer';
export default {
setup() {
const retailer = useStore();
go back { retailer };
},
}
Throughout the above snippet, the tag accommodates your component’s defined HTML markup. To turn the cost of the
rely
belongings from the Pinia store, you employ Vue’s knowledge binding syntax, expressed as {{ rely }}
.
The useStore
function provides get right of entry to to the Pinia store, in order that you import it from store.js using import { useStore } from './store';
.
A feature of Vue 3’s Composition API, the setup
function defines your component’s reactive state and just right judgment. During the function, then you definately identify useStore()
to get right of entry to the Pinia store.
Next, const rely = store.rely
accesses the store’s rely
belongings, making it available inside the component.
After all, setup
returns the rely
, allowing the template to render it. Vue’s reactivity instrument means that your component’s template will substitute the cost of rely
each time it changes inside the store.
Underneath is a screenshot of the output.
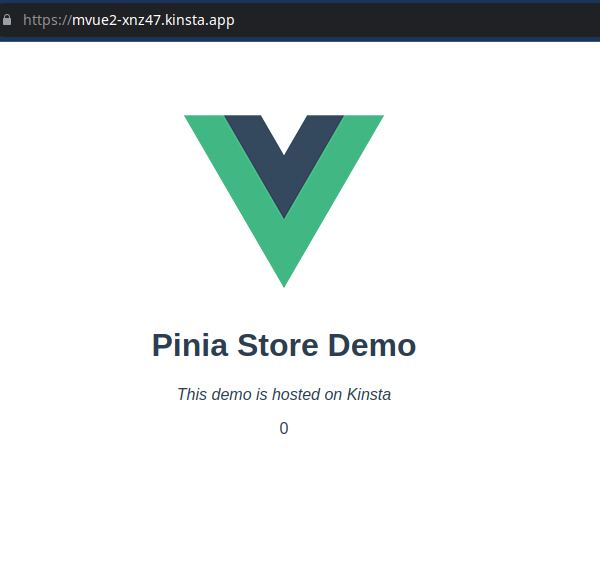
This case illustrates Pinia’s advantages:
- Simplicity — Pinia allows direct get right of entry to to the store’s state without mapping functions. Against this, Vuex needs
mapState
(or identical helpers) to achieve the identical get right of entry to. - Direct store get right of entry to — Pinia lets you get right of entry to state properties (like
store.rely
) without delay, making your code additional readable and comprehensible. Within the intervening time, Vuex incessantly requires getters to get right of entry to even fundamental properties, together with complexity that diminishes readability. - Composition API compatibility — Since the setup manner demonstrates, Pinia’s integration with the Composition API aligns specifically neatly with stylish Vue development, providing a additional uniform coding revel in.
Recommendations on find out how to adjust state with Pinia
In Pinia, you adjust a store’s state using actions, which might be additional flexible than Vuex mutations. Believe the following function identify, which increments the state’s rely
belongings:
store.increment(); // Increments the rely
On the other hand, a Vuex an similar involves defining a mutation at the side of no less than one movement:
mutations: {
increment(state) {
state.rely++;
},
},
actions: {
increment({ commit }) {
commit('increment');
},
}
The Pinia movement and its an similar Vuex code exemplify a the most important difference between the libraries’ code complexity. Let’s uncover the ones diversifications further:
- Direct versus indirect state mutation — Since the
increment
movement demonstrates, Pinia actions without delay mutate the store’s state. In Vuex, you’ll absolute best exchange the state using mutations, which you will have to commit with actions. This process separation promises your state changes are trackable, then again it’s additional difficult and rigid than similar Pinia actions. - Asynchronous versus synchronous operations — Whilst Vuex mutations are all the time synchronous and can’t come with asynchronous processes, Pinia actions can come with synchronous and asynchronous code. Consequently, you’ll perform API calls or other asynchronous operations without delay within actions, making for a leaner and further concise codebase.
- Simplified syntax — Vuex incessantly calls for outlining mutations and calling actions to commit them. Pinia does away with this need. The ability to mutate the state within actions reduces boilerplate code and keeps your provide code more uncomplicated. In Vuex, fundamental state updates require defining an movement and a mutation, despite the fact that the movement is just committing the mutation.
The transition from Vuex to Pinia
Transitioning to Pinia can provide a lot of benefits in simplicity, flexibility, and maintainability, but it requires wary planning and a focus to make sure a a success implementation.
Previous than making the switch, just be sure you:
- Familiarize yourself with the variations between Pinia’s and Vuex’s construction, state keep an eye on patterns, and APIs. Working out the ones diversifications is the most important to effectively refactor your code and take whole good thing about Pinia’s choices.
- Analyze and refactor your Vuex state, actions, mutations, and getters to fit into Pinia’s development. Bear in mind, in Pinia, you define state as a function. You’ll be capable to without delay mutate states with actions and can enforce getters additional simply.
- Plan transition your Vuex store modules. Pinia doesn’t use modules within the identical approach as Vuex, then again you’ll however development your retail outlets to serve identical purposes.
- Leverage Pinia’s improved TypeScript reinforce. If your undertaking uses TypeScript, consider Pinia’s enhanced type inference and typing options for upper type coverage and developer revel in.
- Revise your trying out how to care for changes in state keep an eye on. This process might include updating the way in which you mock retail outlets or actions to your unit and integration tests.
- Believe how the transition affects your undertaking’s development and crew. Account for components like naming conventions and the way in which you import and use retail outlets all over portions.
- Be sure that compatibility with other libraries. Check out for any required updates or dependency changes that the changeover might affect.
- Analysis any potency changes. Pinia is normally additional lightweight than Vuex, then again continue monitoring your tool’s potency all over and after the transition to make sure there don’t seem to be any issues.
Converting a store from Vuex to Pinia involves a lot of steps to care for diversifications in their structures and APIs. Believe the Pinia store from earlier.
The identical store in a Vuex store.js report turns out as follows.
import Vue from 'vue';
import Vuex from 'vuex';
Vue.use(Vuex);
export default new Vuex.Store({
state: {
rely: 0,
},
mutations: {
increment(state) {
state.rely++;
},
},
actions: {
increment(context) {
context.commit('increment');
},
},
getters: {
doubleCount(state) {
return state.rely * 2;
},
},
});
As with the previous Pinia store, this Vuex example accommodates a state
object with a single rely
belongings initialized to 0
.
The mutations
object accommodates how you’ll without delay mutate the state, while the actions
object’s methods commit the increment
mutation.
Then, the getters
object holds the doubleCount
manner, which multiplies the rely
state via 2
and returns the end result.
As this code demonstrates, implementing a store in Pinia involves a lot of noticeable diversifications to Vuex:
- Initialization — Pinia does not require
Vue.use()
. - Building — In Pinia, the state is a function returning an object, taking into account upper TypeScript reinforce and reactivity.
- Actions — Actions in Pinia are methods that without delay mutate the state without having mutations, simplifying the code.
- Getters — While similar to Vuex, Getters in Pinia are defined inside the store definition and can without delay get right of entry to the state.
Recommendations on find out how to use the store in portions
With Vuex, chances are high that you’ll use the store like this:
{{ doubleCount }}
import { mapGetters, mapActions } from 'vuex';
export default {
computed: {
...mapGetters(['doubleCount']),
},
strategies: {
...mapActions(['increment']),
},
};
For Pinia, the usage becomes:
{{ store.doubleCount }}
>
import { useStore } from '/src/retailer';
export default {
setup() {
const retailer = useStore();
go back {
retailer,
};
},
};
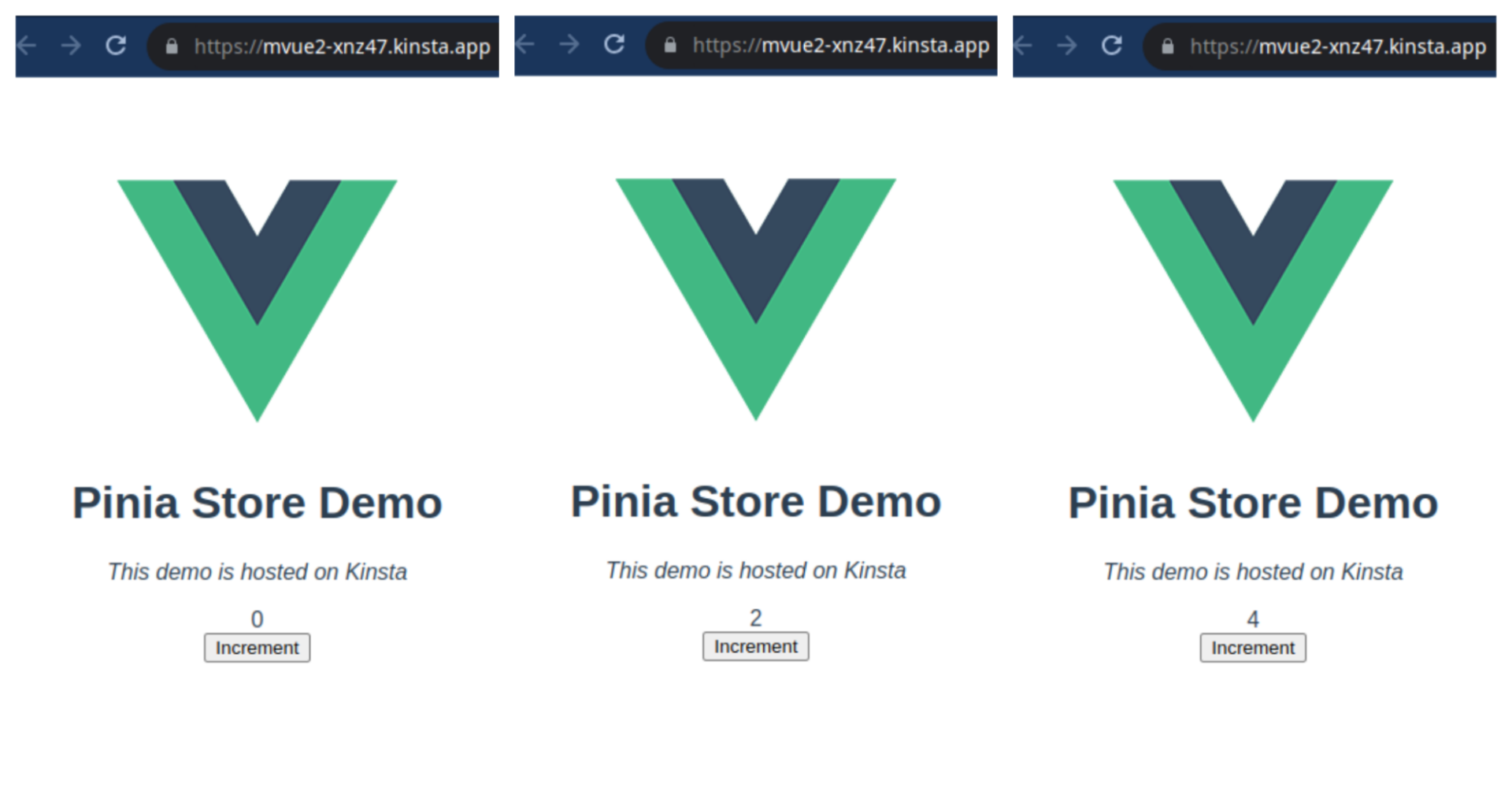
This case covers a fundamental conversion. For additonal difficult Vuex retail outlets, specifically those using modules, the conversion would include a additional detailed restructuring to align with Pinia’s construction.
Recommendations on find out how to deploy your Vue tool
Previous than deploying, sign up for a loose trial of Kinsta’s Utility Webhosting carrier. You’ll deploy the appliance with the assistance of a Dockerfile.
Create a Dockerfile at your undertaking’s root and paste inside the following contents:
FROM node:latest
WORKDIR /app
COPY package deal*.json ./
RUN npm arrange
COPY ./ .
CMD ["npm", "run", "start"]
This code instructs Kinsta’s Docker engine to place in Node.js (FROM node:latest
), create the working checklist (WORKDIR /app
), arrange the node modules from the package deal.json report (RUN npm arrange
) and set the get started (CMD ["npm", "run", "start"]
) command that may be invoked when the Vue app starts. The COPY
directions reproduction the required knowledge or directories to the working checklist.
After that, push your code to a most popular Git provider (Bitbucket, GitHub, or GitLab). Once your repo is able, observe the ones steps to deploy your app to Kinsta:
- Log in or create an account to view your MyKinsta dashboard.
- Authorize Kinsta along side your Git provider.
- Make a choice Application on the left sidebar and click on at the Add Application button.
- Throughout the modal that appears, choose the repository you want to deploy. When you’ve got a few branches, you’ll make a choice the desired division and gives a name on your tool.
- Make a choice one of the most available knowledge center puts.
- Select your assemble surroundings and make a choice Use Dockerfile to organize container image.
- If your Dockerfile isn’t to your repo’s root, use Context to indicate its path and click on on Continue.
- You’ll be capable to go away the Get began command get admission to empty. Kinsta uses
npm get began
to start out your tool. - Make a choice the pod measurement and choice of instances absolute best imaginable suited on your app and click on on Continue.
- Fill out your credit card information and click on on Create tool.
As an alternative choice to tool web website hosting, you’ll opt for deploying your Vue tool as a static internet web page with Kinsta’s static web site internet hosting without spending a dime.
Summary
The transition from Vuex to Pinia marks the most important evolution in state keep an eye on within the Vue ecosystem. Pinia’s simplicity, improved TypeScript reinforce, and alignment with Vue 3’s Composition API make it a compelling variety for modern Vue systems.
Whilst you’re able to host your Vue tool with Kinsta, you’ll get right of entry to a snappy, protected, and loyal infrastructure. Join Kinsta and use our software internet hosting service.
The publish Grasp state control in Vue.js appeared first on Kinsta®.
Contents
- 1 Atmosphere the scene: Pinia vs Vuex
- 2 Recommendations on find out how to prepare a Vue undertaking with Pinia
- 3 Recommendations on find out how to create a store in Pinia
- 4 Recommendations on find out how to get right of entry to store state in portions
- 5 Recommendations on find out how to adjust state with Pinia
- 6 The transition from Vuex to Pinia
- 7 Recommendations on find out how to deploy your Vue tool
- 8 Summary
- 9 6 Commercial Advertising Methods to Make Your Trade A success
- 10 5 Fast Fixes for Sluggish Wi-Fi Connection on iPhone
- 11 Why is Neighborhood Control Essential?
0 Comments